Memory and Resource Management in PHP: A Beginner's Guide

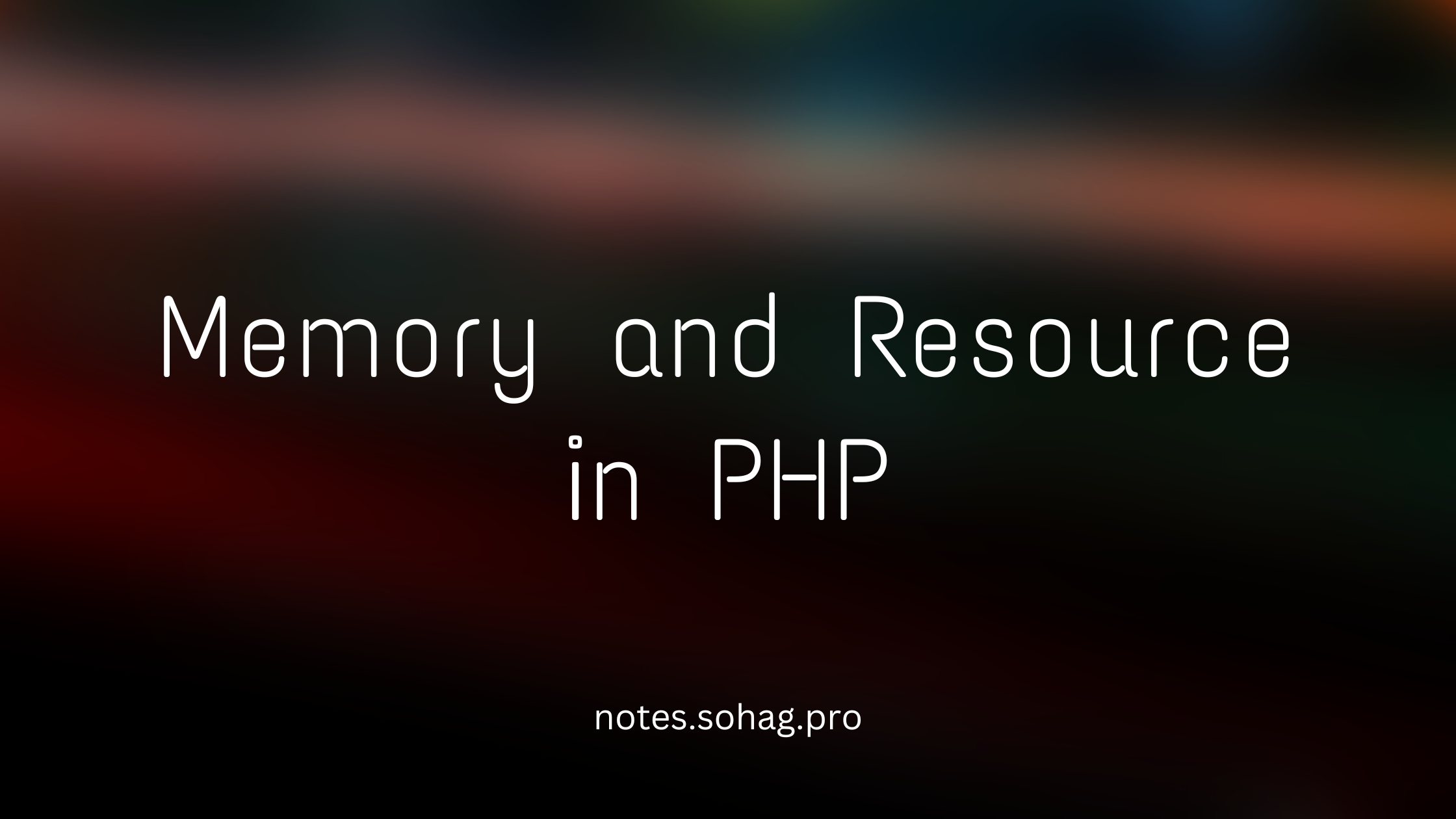
Memory and Resource Management in PHP
Introduction
Just like managing household expenses and organizing your closet, managing memory and resources in PHP is crucial for maintaining a healthy application. Imagine your computer's memory as your home - you need to keep it clean, organized, and efficient to live comfortably. Let's dive into how PHP handles memory and resources, using real-life examples to make these concepts easier to understand.
Understanding Memory Management in PHP
Garbage Collection
Think of PHP's garbage collector as your household cleaning service. Just like how you don't need to manually clean every dish after a meal if you have a dishwasher, PHP automatically cleans up memory that's no longer being used.
function createLargeArray() {
$largeArray = range(1, 10000);
// When this function ends, $largeArray will be automatically cleaned up
}
createLargeArray();
// Memory is freed here automatically
This is similar to how you might clear out your closet - items you no longer use (variables that are out of scope) are removed to make space for new ones.
Memory Limits
Just like how your house has a finite amount of space, PHP has memory limits. You can check and modify these limits:
// Check current memory limit
echo ini_get('memory_limit'); // Usually "128M"
// Set new memory limit (like expanding your house)
ini_set('memory_limit', '256M');
Real-life example: Think of this as managing storage space in your smartphone. When you're running out of space, you either need to delete some apps (free memory) or upgrade to a phone with more storage (increase memory limit).
Resource Management
File Handles
Managing file handles is like managing doors in your house - you need to close them when you're done:
// Bad practice (leaving the door open)
$file = fopen('important.txt', 'r');
// ... do something ...
// Forgot to close!
// Good practice (always close the door)
$file = fopen('important.txt', 'r');
try {
// ... do something ...
} finally {
fclose($file);
}
// Best practice (automatic door closer)
$contents = file_get_contents('important.txt');
Database Connections
Like maintaining relationships with friends, database connections need proper management:
// Using PDO with proper connection management
try {
$pdo = new PDO("mysql:host=localhost;dbname=test", "user", "password");
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
// Use the connection
$stmt = $pdo->query("SELECT * FROM users");
} catch(PDOException $e) {
echo "Connection failed: " . $e->getMessage();
} finally {
// Close the connection explicitly
$pdo = null;
}
Best Practices for Memory Optimization
Use References for Large Objects Like sharing a book instead of making copies:
// Without reference (makes a copy) function processLargeArray($array) { // Processes array } // With reference (shares the original) function processLargeArray(&$array) { // Processes array }
Unset Variables When No Longer Needed Like donating items you no longer use:
$largeData = getLargeDataSet(); processData($largeData); unset($largeData); // Free up memory explicitly
Use Generators for Large Datasets Like getting groceries one bag at a time instead of all at once:
function getNumbers($max) { for ($i = 1; $i <= $max; $i++) { yield $i; } } foreach (getNumbers(1000000) as $number) { // Process one number at a time }
Compiler Customization
PHP.ini Configuration
Like customizing your home's settings:
; Memory settings
memory_limit = 256M
max_execution_time = 30
; Error reporting for development
error_reporting = E_ALL
display_errors = On
; Production settings
error_reporting = E_ALL & ~E_DEPRECATED & ~E_STRICT
display_errors = Off
OPcache Settings
Think of OPcache as your kitchen's pantry - storing frequently used items for quick access:
opcache.enable=1
opcache.memory_consumption=128
opcache.max_accelerated_files=10000
Topics to Explore Further
Memory Profiling Tools
Xdebug
PHP Meminfo
Advanced Garbage Collection
Circular References
Reference Counting
Generational Garbage Collection
Caching Strategies
Redis
Memcached
APCu
Performance Monitoring
New Relic
Datadog
Custom Monitoring Solutions
Conclusion
Managing memory and resources in PHP is like maintaining a well-organized household. By following best practices and understanding how PHP handles memory, you can create efficient, scalable applications. Remember to:
Clean up after yourself (close connections and files)
Don't waste resources (use memory efficiently)
Organize your space (manage memory limits)
Use tools wisely (leverage PHP's built-in features)
Just as you wouldn't leave your house in disarray, keeping your PHP application's memory and resources well-managed ensures smooth operation and optimal performance. Continue exploring the suggested topics to deepen your understanding and become a better PHP developer.
Remember, good memory management habits in programming, like good habits in daily life, take time to develop but pay off tremendously in the long run.
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author