Understanding PHP Caching: A Comprehensive Guide for Beginners

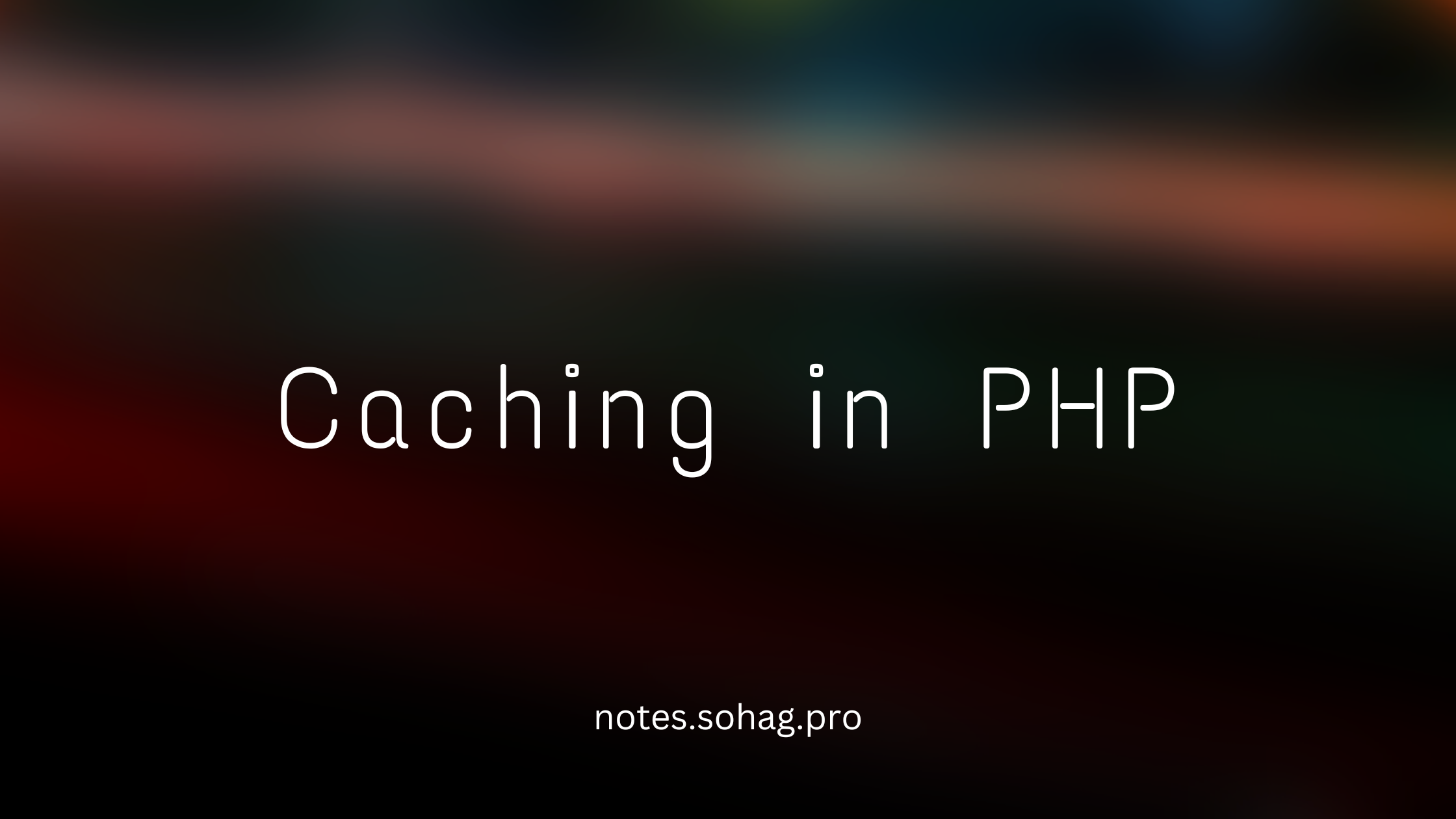
Caching in PHP and Laravel
Introduction
Imagine walking to your kitchen every time you need a glass of water versus keeping a water bottle at your desk. That's essentially what caching is in programming - keeping frequently used data closer for quick access. In this guide, we'll explore caching in PHP, from basic concepts to advanced implementation with Laravel, making it relatable and practical for everyday development.
Why You Should Care
Speed: Your applications can run up to 1000% faster with proper caching
Cost Savings: Reduced server load means lower hosting costs
User Experience: Faster response times = happier users
Scalability: Handle more users without proportionally increasing resources
Think about it: Netflix doesn't re-encode a movie every time someone wants to watch it. They cache the encoded versions. Similarly, your PHP application shouldn't recalculate or refetch the same data repeatedly.
Basic Caching Concepts
1. Application-Level Caching
Think of this as your personal notepad where you jot down important information for quick reference.
// Without caching
function calculateComplexValue($input) {
// Complex calculation that takes time
sleep(2); // Simulating complex calculation
return $input * 2;
}
// With caching
function calculateComplexValueCached($input) {
static $cache = [];
if (isset($cache[$input])) {
return $cache[$input]; // Return cached result
}
// Calculate and cache result
$cache[$input] = $input * 2;
return $cache[$input];
}
Real-life example: Like keeping your frequently used cooking ingredients on the counter instead of the storage room.
2. Database Query Caching with Laravel
Similar to how a barista remembers regular customers' orders, Laravel can remember database query results.
// Without caching
$users = DB::table('users')
->where('active', 1)
->get();
// With caching
$users = Cache::remember('active_users', 3600, function () {
return DB::table('users')
->where('active', 1)
->get();
});
Real-life example: A restaurant keeping its most-ordered dishes pre-prepared during rush hour.
3. View Caching
Think of this as taking a snapshot of your finished artwork instead of redrawing it every time.
// In your blade template
@cache('unique_key', 3600)
@foreach($products as $product)
<div class="product-card">
{{ $product->name }}
</div>
@endforeach
@endcache
Advanced Caching Techniques
1. Redis Implementation
Redis is like having a super-fast assistant who remembers everything.
// Configure Redis in .env
REDIS_HOST=127.0.0.1
REDIS_PORT=6379
// Using Redis in Laravel
use Illuminate\Support\Facades\Redis;
Redis::set('user:1', json_encode($userData));
$userData = json_decode(Redis::get('user:1'));
2. Custom Cache Driver
Creating your own cache driver is like building a custom storage solution for specific needs.
namespace App\Cache;
use Illuminate\Contracts\Cache\Store;
class CustomCache implements Store
{
public function get($key)
{
// Custom implementation
}
public function put($key, $value, $seconds)
{
// Custom implementation
}
// Other required methods...
}
3. Cache Tags
Like organizing your files with labels for easy access.
// Storing with tags
Cache::tags(['users', 'profiles'])->put('user:1', $userData, 3600);
// Retrieving tagged cache
$userData = Cache::tags(['users'])->get('user:1');
// Flush specific tags
Cache::tags(['users'])->flush();
Best Practices
Cache Invalidation Strategy
Set appropriate TTL (Time To Live)
Use cache tags for grouped invalidation
Implement versioning for cache keys
// Using cache versioning
$cacheKey = "users_list_v" . config('cache.version');
Security Considerations
Never cache sensitive data
Implement cache key prefixes
Use encryption when necessary
// Secure caching
Cache::tags(['secure'])->put(
'user_data_' . hash('sha256', $userId),
encrypt($userData),
3600
);
Performance Monitoring
Monitor cache hit/miss ratios
Implement cache warming strategies
Use cache race condition prevention
// Atomic cache operations
Cache::lock('processing_data')->get(function () {
// Process and cache data
});
Topics to Explore Further
Cache Backends
Memcached vs Redis
File-based caching
Database caching
Advanced Laravel Caching
Queue workers with cache
Broadcasting with cache
Rate limiting using cache
Distributed Caching
Cache synchronization
Cache replication
Cache clustering
Common Caching Patterns and Real-Life Analogies
Cache-Aside (Lazy Loading)
- Like checking your pocket before going to the ATM
function getData($key) {
if (!Cache::has($key)) {
Cache::put($key, fetchFromDatabase(), 3600);
}
return Cache::get($key);
}
Write-Through
- Like updating both your digital and paper calendar simultaneously
function saveData($key, $value) {
Database::save($value);
Cache::put($key, $value, 3600);
}
Write-Behind
- Like collecting dishes in a bin and washing them all at once
function saveData($key, $value) {
Cache::put($key, $value, 3600);
dispatch(new UpdateDatabaseJob($key, $value));
}
Conclusion
Caching is not just a performance optimization technique; it's a fundamental concept that can make or break your application's success. Like a well-organized kitchen where everything has its place, proper caching ensures your PHP application runs smoothly and efficiently. Start with basic caching techniques and gradually move to more advanced patterns as your application grows.
Remember: The best caching strategy is the one that fits your specific needs. Don't overcomplicate it initially - start simple and optimize based on real usage patterns.
Further Reading:
Laravel Official Documentation on Caching
Redis Documentation
PHP OpCache Configuration
Memcached vs Redis Comparison
Distributed Caching Architectures
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author