Distributed Caching Architectures in Laravel: A Beginner's Guide

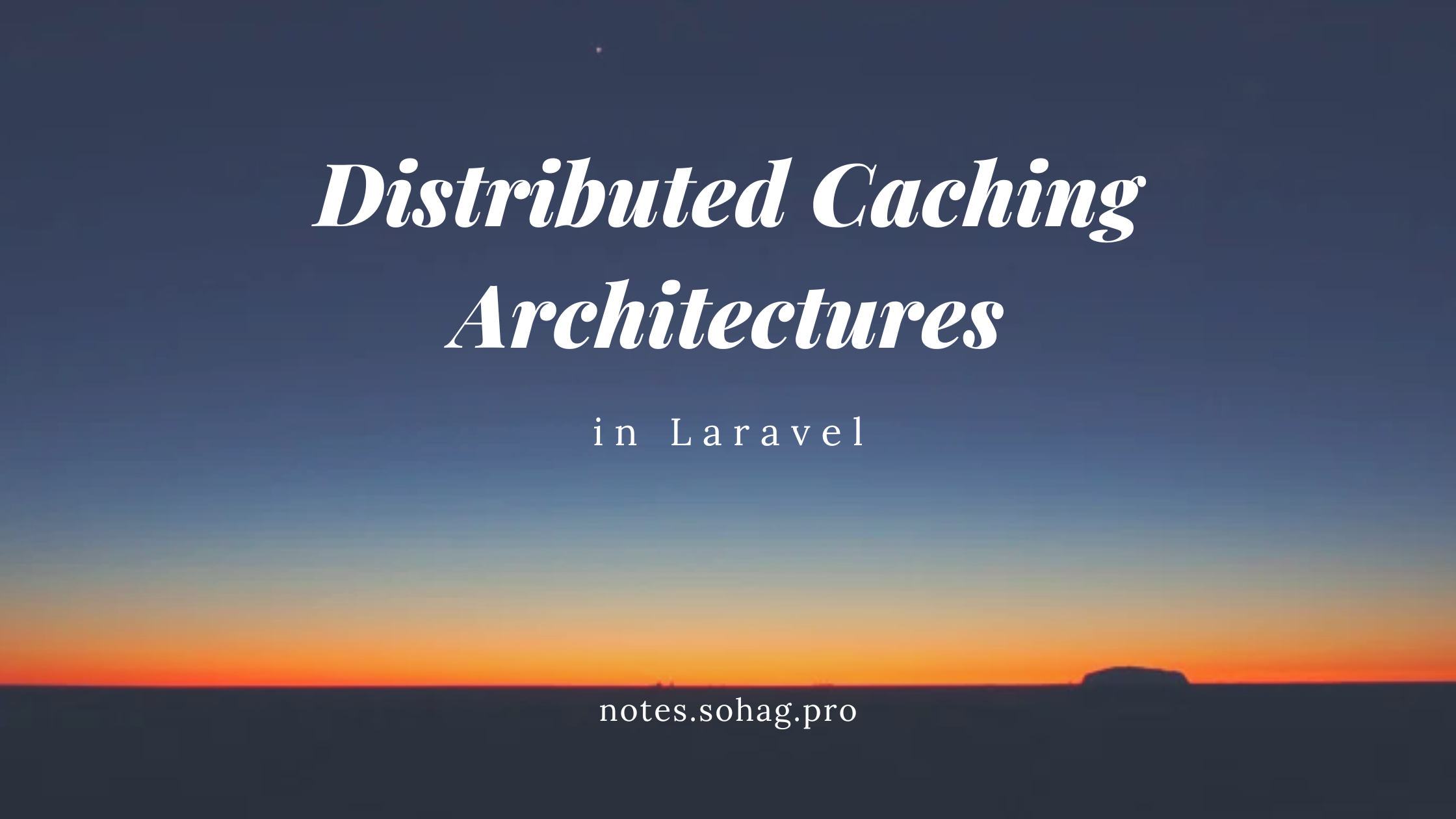
Distributed Caching Architectures in Laravel
Introduction
Imagine you're preparing breakfast every morning. Instead of taking out all the ingredients from different cabinets each time, you keep frequently used items like coffee, sugar, and bread on the counter for quick access. This is exactly how caching works in web applications – keeping frequently accessed data readily available to serve requests faster.
In Laravel applications, caching becomes crucial as your application grows and handles more users. Just as a busy café can't rely on a single coffee machine during rush hour, your application can't depend on a single cache store when scaling up. This is where distributed caching comes into play.
Understanding Basic Caching in Laravel
Before diving into distributed patterns, let's understand how basic caching works in Laravel:
// Storing data in cache
Cache::put('user_profile', $userProfile, now()->addHours(24));
// Retrieving data from cache
$userProfile = Cache::get('user_profile');
// Store if not exists
$value = Cache::remember('users', 3600, function () {
return DB::table('users')->get();
});
Distributed Caching Patterns
1. Replication Pattern
Think of replication like having multiple copies of your favorite recipe book in different locations. If one gets damaged, you can still access the recipes from another copy.
Implementation Example:
// config/cache.php
'stores' => [
'distributed' => [
'driver' => 'redis',
'connection' => 'cache',
'replicas' => [
['host' => 'redis-1.example.com', 'port' => 6379],
['host' => 'redis-2.example.com', 'port' => 6379],
],
],
],
Usage:
class UserController extends Controller
{
public function show($id)
{
return Cache::store('distributed')->remember(
"user.{$id}",
3600,
function () use ($id) {
return User::find($id);
}
);
}
}
2. Sharding Pattern
Sharding is like organizing your wardrobe by categories – putting shirts in one drawer, pants in another. Each cache server handles specific types of data.
Implementation:
class ShardedCache
{
protected $shards = [
'users' => 'redis-1',
'products' => 'redis-2',
'orders' => 'redis-3'
];
public function get($key)
{
$shard = $this->determineShardByKey($key);
return Cache::store($shard)->get($key);
}
protected function determineShardByKey($key)
{
$prefix = explode('.', $key)[0];
return $this->shards[$prefix] ?? 'default';
}
}
3. Partitioning Pattern
Partitioning distributes data across servers based on a consistent hashing algorithm, like dividing students into different classrooms based on their last names.
class PartitionedCache
{
protected $nodes = [
'cache-1' => ['start' => 0, 'end' => 1000],
'cache-2' => ['start' => 1001, 'end' => 2000],
'cache-3' => ['start' => 2001, 'end' => 3000],
];
public function set($key, $value, $ttl = 3600)
{
$node = $this->getNodeForKey($key);
return Cache::store($node)->put($key, $value, $ttl);
}
protected function getNodeForKey($key)
{
$hash = crc32($key) % 3000;
foreach ($this->nodes as $node => $range) {
if ($hash >= $range['start'] && $hash <= $range['end']) {
return $node;
}
}
}
}
Best Practices
- Cache Invalidation Strategy
// Use tags for easier cache management
Cache::tags(['users', "user.{$id}"])->put("user.{$id}", $userData, 3600);
// Invalidate all user-related caches
Cache::tags(['users'])->flush();
- Handle Cache Failures Gracefully
public function getData($key)
{
try {
return Cache::get($key, function() {
return $this->fetchFromDatabase();
});
} catch (Exception $e) {
Log::error("Cache error: " . $e->getMessage());
return $this->fetchFromDatabase();
}
}
- Implement Cache Warming
class WarmCache
{
public function handle()
{
// Pre-cache frequently accessed data
$popularProducts = Product::popular()->get();
Cache::tags(['products'])->put('popular_products', $popularProducts, now()->addDay());
}
}
Monitoring and Debugging
// Add cache hit/miss monitoring
Cache::extend('monitored', function ($app) {
return Cache::repository(new MonitoredStore(
new RedisStore($app['redis']),
$app['events']
));
});
// Listen for cache events
Event::listen('cache.hit', function ($key) {
Log::info("Cache hit for key: {$key}");
});
Conclusion
Distributed caching is essential for scaling Laravel applications effectively. By understanding and implementing these patterns correctly, you can significantly improve your application's performance and reliability. Remember:
Use replication for high availability
Implement sharding for better resource utilization
Consider partitioning for balanced data distribution
Always implement proper monitoring and fallback mechanisms
Further Learning
To deepen your understanding of distributed caching, explore these topics:
Redis Cluster Configuration and Management
Cache Coherency Protocols
Event-Driven Cache Invalidation
Cache-Aside vs. Write-Through Patterns
Laravel Horizon for Redis Queue Management
Remember that caching is an optimization technique that requires careful consideration of your specific use case. Start with simple implementations and gradually move to more complex patterns as your application's needs grow.
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author