Day 16: Mastering Modern JavaScript - Key Features of ES6
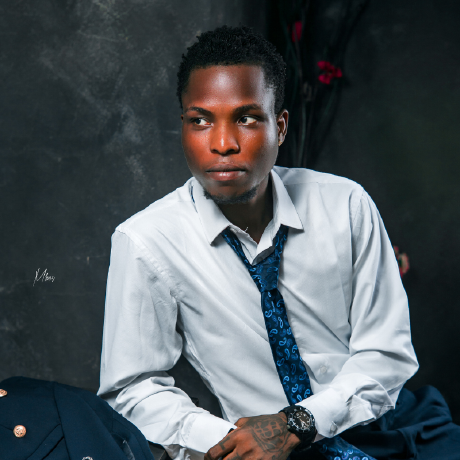
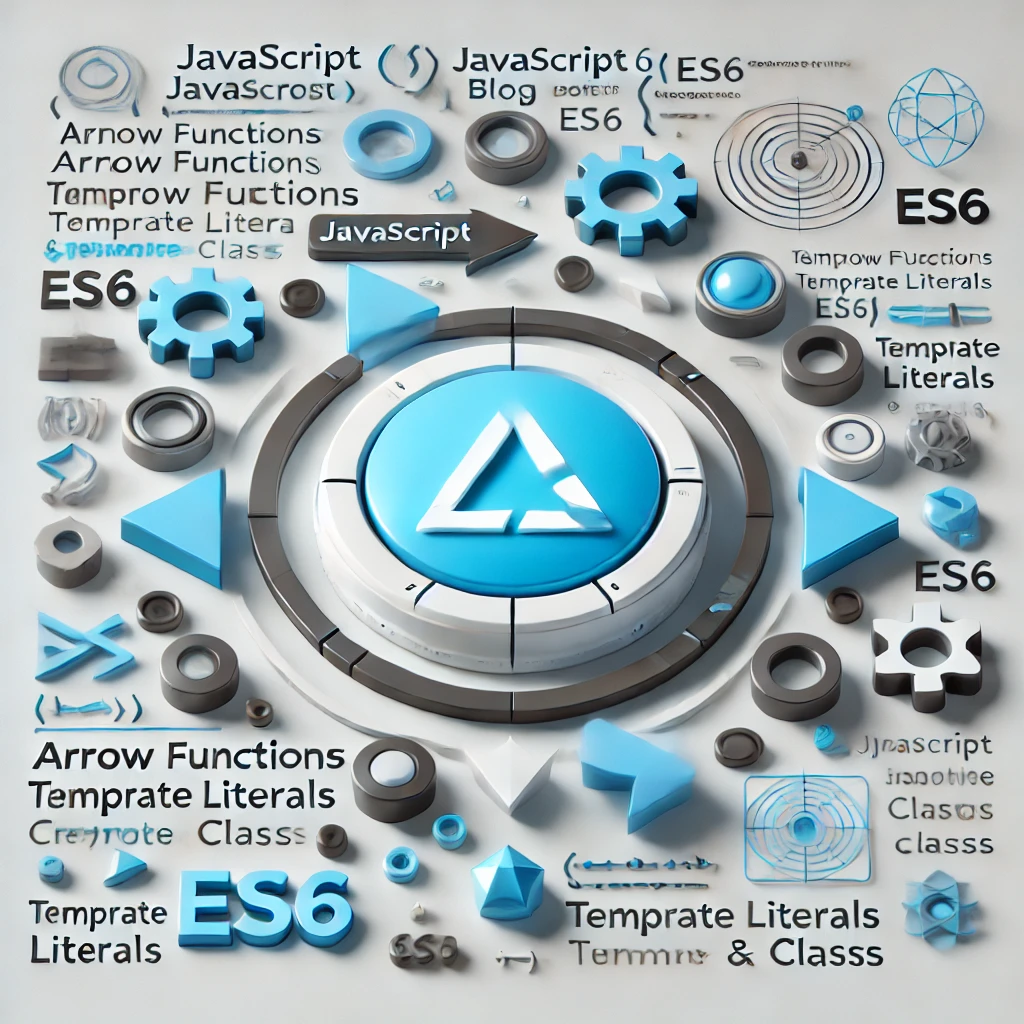
Today was meant to be a relaxed writing session for our Day 16 blog post, complete with a glass of white wine. But after a quick stop at the ShopRite mall to pick up a new facial product (as you may have seen in my latest tweet on X), I missed the chance to grab the wine before the store closed! Since I’m not one for fake wine substitutes, I’ll save that glass for tomorrow’s post.
In the meantime, join me as we dive into some of the most game-changing features of ECMAScript 6 (ES6). In 2015, ES6 brought several groundbreaking updates that transformed JavaScript into a powerhouse for modern development. From cleaner syntax to new data structures, let’s explore these key features and see how they can make our code more efficient, readable, and fun to write. Enjoy exploring JavaScript ES6, and stay tuned for tomorrow’s post—this time, with that well-deserved wine in hand!
1. Block-Scoped Declarations with let
and const
Before ES6, JavaScript used var
for variable declarations, which had function scope. ES6 introduced let
and const
, which provide block scope — meaning they are only accessible within the nearest curly braces {}
.
let
allows reassignment within the block.const
creates constants whose values cannot be reassigned.
function scopeExample() {
var a = 10;
let b = 20;
const c = 30;
console.log(a); // 10
console.log(b); // 20
console.log(c); // 30
}
scopeExample();
console.log(a); // ReferenceError: a is not defined
2. Arrow Functions
Arrow functions offer a cleaner syntax and have a lexically bound this
context, meaning they inherit this
from the surrounding code. If the function body is a single expression, we can even omit the return
statement.
const greet = () => console.log('Hello!');
greet(); // Output: Hello!
3. Template Literals
Template literals allow for easy string interpolation and multi-line strings. They use backticks (`
) and ${expression}
to embed variables directly within strings.
const name = "JavaScript";
console.log(`Welcome to ${name} learning!`);
4. Destructuring Assignment
Destructuring lets us extract values from arrays or objects into variables, simplifying code and improving readability.
const user = { name: "Alice", age: 25 };
const { name, age } = user;
console.log(name); // Output: Alice
5. Default Parameters
Default parameters allow functions to initialize parameters with default values if they’re not provided, reducing the need for additional checks.
function greet(name = "Guest") {
return `Hello, ${name}!`;
}
console.log(greet()); // Output: Hello, Guest!
6. Rest and Spread Operators
The rest operator (...args
) collects arguments into an array, while the spread operator (...arr
) expands elements of an iterable (like an array) into individual elements.
const numbers = [1, 2, 3];
console.log(...numbers); // Output: 1 2 3
7. Classes
JavaScript introduced a class syntax in ES6, making object-oriented programming more accessible. Classes in JavaScript support constructors, methods, inheritance, and super
calls.
class Person {
constructor(name) {
this.name = name;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
const person = new Person("Alice");
person.greet(); // Output: Hello, my name is Alice
8. Modules
Modules help organize JavaScript code into reusable components. They allow you to export variables, functions, or classes from one file and import them into another, making code modular and maintainable.
// math.js
export function add(a, b) {
return a + b;
}
// main.js
import { add } from './math.js';
console.log(add(2, 3)); // Output: 5
Why These ES6 Features Matter
These features have made JavaScript more powerful and accessible, providing developers with tools to write cleaner, more maintainable, and efficient code. As we continue our journey, these ES6 updates will become essential tools in our JavaScript toolkit.
Special Thanks and Closing Thoughts
A big thank you to Monali Bedre, Board Infinity, and Divami for their incredible content on modern JavaScript features. Their articles have been invaluable in shaping today’s insights:
Mastering Modern JavaScript: Exploring the Key Features of ES6 by Monali Bedre
Top 10 Features of ES6 - Board Infinity
Top ECMAScript (ES6) Features Every JavaScript Developer Should Know - Divami
As we continue our journey through the exciting world of JavaScript and React, I encourage you to keep exploring, learning, and growing alongside me.
Your support and feedback mean the world, and I would love to hear your thoughts and experiences as we tackle this challenge together.
Let’s push forward, take on new challenges, and celebrate our progress—because each day brings us closer to mastering these essential technologies. Stay tuned for tomorrow’s post, and let’s keep the momentum going!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
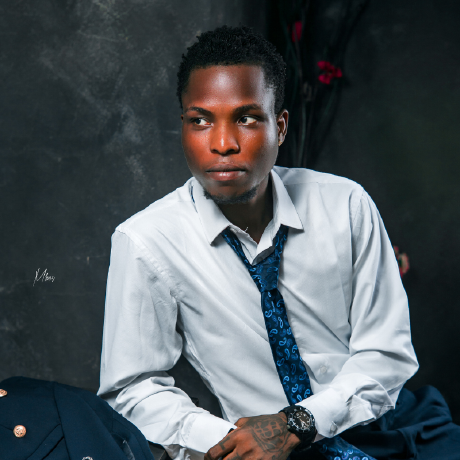
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com