Learn to Create Basic Windows Forms in C#: A Guide for HNDIT Students

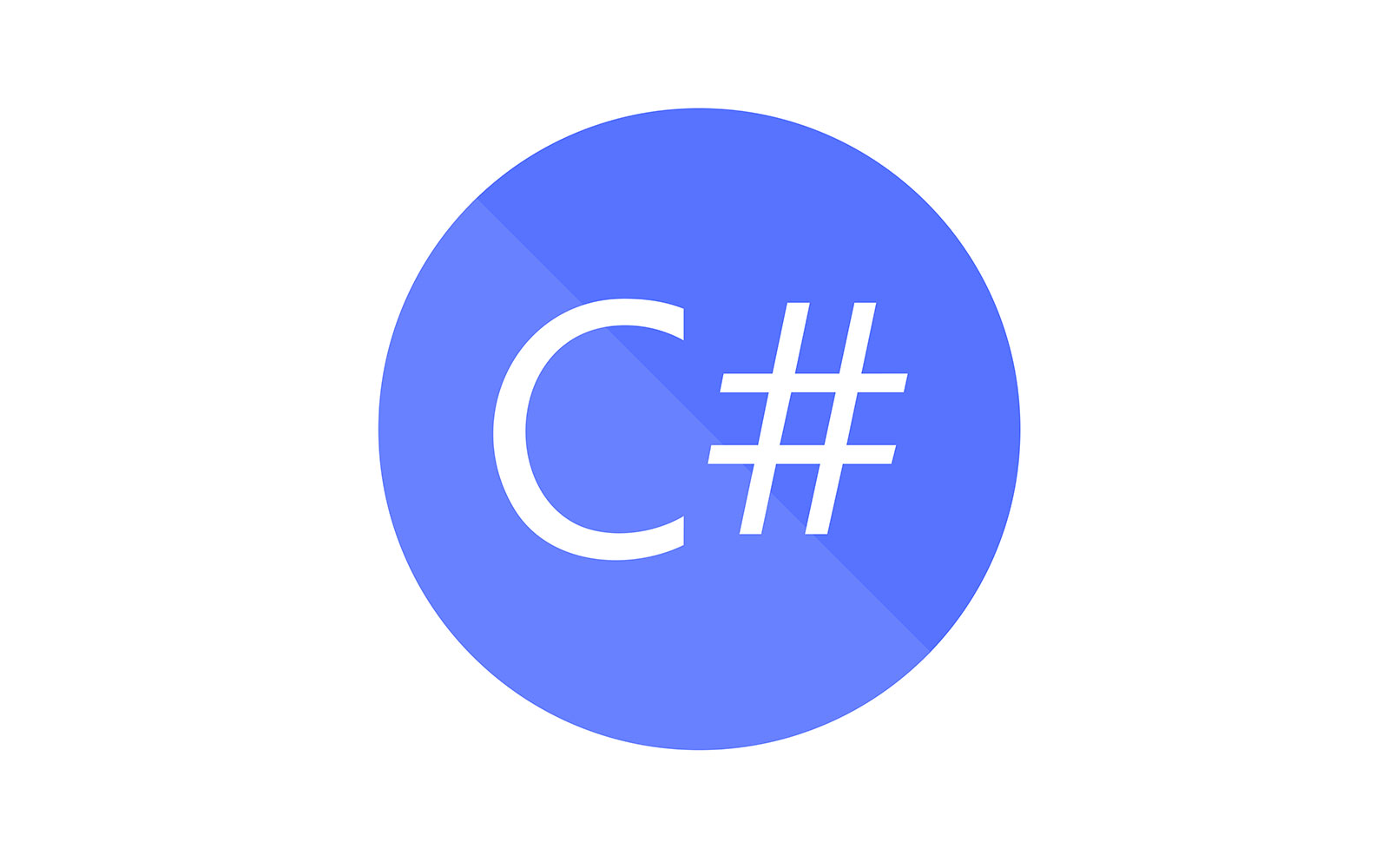
Objective: Create a calculator that performs basic arithmetic operations (addition, subtraction, multiplication, division).
Steps:
Add four
Button
controls for each operation.Add two
TextBox
controls for input numbers and oneLabel
for displaying the result.Write code in the
Button
click events to perform calculations and display results in theLabel
.
private void btnAdd_Click(object sender, EventArgs e)
{
int num1 = int.Parse(txtNumber1.Text);
int num2 = int.Parse(txtNumber2.Text);
lblResult.Text = (num1 + num2).ToString();
}
2. Temperature Converter
Objective: Convert temperatures from Celsius to Fahrenheit and vice versa.
Steps:
Add a
TextBox
for input and aLabel
to display the converted temperature.Add two
RadioButton
controls, one for Celsius to Fahrenheit and the other for Fahrenheit to Celsius.Add a
Button
for the conversion.In the button's click event, check the selected
RadioButton
and perform the conversion.
private void btnConvert_Click(object sender, EventArgs e)
{
double temp = double.Parse(txtTemperature.Text);
if (rbtnCtoF.Checked)
{
lblResult.Text = ((temp * 9 / 5) + 32).ToString("0.##") + " °F";
}
else if (rbtnFtoC.Checked)
{
lblResult.Text = ((temp - 32) * 5 / 9).ToString("0.##") + " °C";
}
}
3. Basic Login Form
Objective: Create a login form with username and password verification.
Steps:
Add
TextBox
controls for username and password, and aButton
for login.Write code to verify the username and password when the button is clicked.
Display a success message if the username and password are correct, or an error if they are incorrect.
private void btnLogin_Click(object sender, EventArgs e)
{
string username = txtUsername.Text;
string password = txtPassword.Text;
if (username == "admin" && password == "12345")
{
MessageBox.Show("Login Successful!");
}
else
{
MessageBox.Show("Invalid username or password");
}
}
4. Simple To-Do List
Objective: Allow the user to add tasks to a list, mark them as completed, and delete them.
Steps:
Add a
TextBox
for input and aButton
to add tasks.Add a
ListBox
to display the tasks.Add two more buttons to mark tasks as completed and to delete selected tasks.
private void btnAddTask_Click(object sender, EventArgs e)
{
listBoxTasks.Items.Add(txtTask.Text);
txtTask.Clear();
}
private void btnMarkComplete_Click(object sender, EventArgs e)
{
if (listBoxTasks.SelectedItem != null)
{
int index = listBoxTasks.SelectedIndex;
listBoxTasks.Items[index] = "[Completed] " + listBoxTasks.Items[index];
}
}
private void btnDeleteTask_Click(object sender, EventArgs e)
{
listBoxTasks.Items.Remove(listBoxTasks.SelectedItem);
}
5. Change Background Color
Objective: Create a form where users can change the background color.
Steps:
Add several
Button
controls, each representing a different color.In each button's click event, change the form's
BackColor
.
private void btnRed_Click(object sender, EventArgs e)
{
this.BackColor = Color.Red;
}
private void btnGreen_Click(object sender, EventArgs e)
{
this.BackColor = Color.Green;
}
Subscribe to my newsletter
Read articles from Arzath Areeff directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arzath Areeff
Arzath Areeff
I co-founded digizen.lk to promote online safety and critical thinking. Currently, I’m developing an AI app to fight misinformation. As Founder and CEO of ideaGeek.net, I help turn startup dreams into reality, and I share tech insights and travel stories on my YouTube channels, TechNomad and Rz Omar.