Setting Up a Jenkins Pipeline for Automated Testing and Deployment
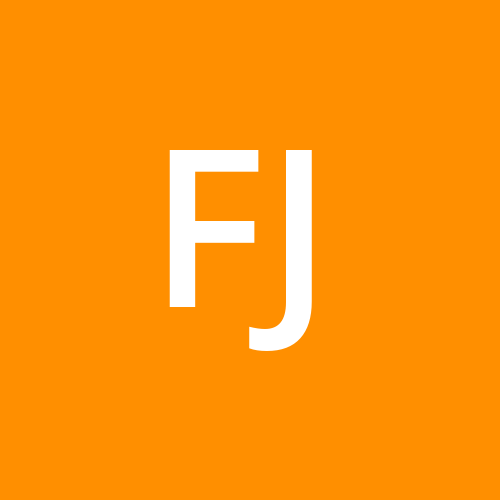
Automation is at the heart of modern software development, enabling teams to deploy faster and with higher confidence. Jenkins, a widely-used CI/CD tool, simplifies the automation process, making it an ideal choice for setting up a reliable pipeline that handles everything from testing to deployment. This step-by-step guide will walk you through setting up a Jenkins pipeline for automated testing and deployment—a valuable skill if you’re considering a cloud computing course in Mumbai or diving into the DevOps world.
Why Use Jenkins for CI/CD?
Jenkins offers extensive support for building CI/CD pipelines. With it, you can automate code integration, testing, and deployment, reducing manual errors and accelerating release cycles. Its flexibility to integrate with other tools, plugins, and cloud platforms makes it ideal for cloud-based applications.
Step-by-Step Guide: Setting Up a Jenkins Pipeline for Automated Testing and Deployment
Here’s how to set up an automated pipeline in Jenkins to streamline testing and deployment. We’ll assume that Jenkins is already installed and configured.
Step 1: Create a New Pipeline Project in Jenkins
Login to Jenkins Dashboard: Open your Jenkins dashboard and navigate to the main page.
Create a New Item: Click on "New Item" and enter a name for your pipeline (e.g., "Automated-Test-Deploy-Pipeline").
Select Pipeline: Choose "Pipeline" as the project type and click "OK" to create it.
This setup allows you to configure a pipeline that Jenkins will execute automatically based on the instructions you provide in the Jenkinsfile
.
Step 2: Write Your Jenkinsfile
The Jenkinsfile
is a script that defines each step of your pipeline. This file uses either declarative or scripted syntax; here, we’ll focus on a declarative pipeline, which is simpler and more maintainable.
Here’s a sample Jenkinsfile
that covers building, testing, and deploying a Java application:
groovyCopy codepipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-repository-url.git'
}
}
stage('Build') {
steps {
sh './gradlew build' // Use gradle or maven for Java apps, or modify for other languages
}
}
stage('Test') {
steps {
sh './gradlew test' // Modify as per your testing command
}
}
stage('Deploy') {
steps {
sh './deploy.sh' // This can be a script for deploying to a server
}
}
}
post {
success {
echo 'Pipeline completed successfully!'
}
failure {
echo 'Pipeline failed!'
}
}
}
Checkout Stage: Retrieves the latest code from the specified repository.
Build Stage: Builds the application using a command (
./gradlew build
in this case).Test Stage: Executes tests, ensuring code functionality.
Deploy Stage: Deploys the code to your preferred server or environment.
Step 3: Configure Jenkins Pipeline Triggers
To make your Jenkins pipeline truly automated, set up triggers so it runs on code changes or scheduled intervals.
Navigate to Pipeline Configuration: In your Jenkins project, go to "Configure."
Build Triggers: Under the “Build Triggers” section, you have options such as:
Poll SCM: Jenkins will check the repository for changes on a specified interval.
GitHub Hook Trigger for GITScm Polling: Automatically triggers the pipeline when a GitHub push is detected.
Selecting either of these options allows Jenkins to react to code changes or execute pipelines on a regular schedule.
Step 4: Add Automated Testing
Automated testing is crucial for CI/CD pipelines, as it validates code changes before deploying to production. Jenkins supports various testing frameworks, and you can configure your Jenkinsfile
to run them automatically.
Unit Testing: Add testing frameworks like JUnit (for Java) or PyTest (for Python) to your build command.
Integration Testing: For more complex testing, include integration tests in the pipeline to validate interactions between components.
In our Jenkinsfile
example, we used ./gradlew test
to execute tests. Jenkins will collect and display test results, giving you insights into test success rates and any failures.
Step 5: Configure Deployment Automation
Automated deployment allows the pipeline to deliver changes directly to staging or production environments. This process might vary depending on your deployment platform, whether it’s cloud-based, on-premises, or hybrid.
Scripted Deployments: Create a deployment script (e.g.,
deploy.sh
) that handles pushing your application to the target environment.Use Jenkins Plugins for Deployment: Jenkins has plugins like AWS CodeDeploy and Kubernetes that can simplify cloud deployments. If you’re deploying to a cloud environment, these plugins can streamline integration with cloud services.
For example, a deployment script might include commands to transfer files to a server or initiate a Kubernetes rollout.
bashCopy code#!/bin/bash
echo "Deploying application..."
scp -r ./build user@yourserver:/path/to/deploy
Ensure you include this script in your Jenkinsfile
under the deployment stage.
Step 6: Configure Notifications
Keeping track of pipeline execution is easier with notifications. Jenkins supports integrations with Slack, email, and other communication tools to alert your team about build results.
Install Notifications Plugin: Go to “Manage Jenkins” > “Manage Plugins” and install plugins for your preferred notification service (e.g., Slack Notification Plugin).
Configure Notifications: In your pipeline, add a post-build action to send notifications upon success or failure. This helps keep everyone updated about the status of builds and deployments.
Example notification configuration in Jenkinsfile
:
groovyCopy codepost {
success {
slackSend(channel: '#deployments', message: "Pipeline completed successfully!")
}
failure {
slackSend(channel: '#deployments', message: "Pipeline failed. Check logs for details.")
}
}
Benefits of Using Jenkins CI/CD for Cloud-Based Applications
For those interested in a cloud computing course in Mumbai, building Jenkins CI/CD pipelines with automated testing and deployment is essential for cloud deployments. Here’s why:
Scalability: Cloud-native deployments allow Jenkins to scale seamlessly, running builds on cloud instances or containers.
Efficiency: CI/CD pipelines in Jenkins reduce the time and manual effort required to test and deploy, allowing for rapid iteration.
Flexibility: With Jenkins’ wide range of plugins and integrations, it’s easy to connect with cloud services, making it ideal for cloud-based applications.
Advanced Tips for Jenkins CI/CD
Implement Environment-Specific Pipelines: Use different pipelines for development, staging, and production to control the release process.
Parallelize Stages: Jenkins allows running stages in parallel, which can speed up pipelines by testing or building different components simultaneously.
Artifact Management: Use artifact repositories like JFrog or Nexus to store and manage build artifacts, especially if you’re deploying across different environments.
Conclusion
Setting up an automated CI/CD pipeline with Jenkins for testing and deployment is an invaluable skill for modern developers and DevOps professionals. This pipeline not only speeds up software delivery but also reduces the chances of manual errors, ensuring higher code quality and quicker time-to-market. By integrating testing and automated deployments into Jenkins, you can establish a streamlined, consistent, and efficient delivery process.
If you’re considering a cloud computing course in Mumbai, understanding Jenkins and CI/CD automation will prepare you for real-world cloud and DevOps challenges, giving you a solid foundation in automation best practices. Start exploring Jenkins today and experience the efficiency of automated pipelines in your development workflow!
Subscribe to my newsletter
Read articles from Fizza Jatniwala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
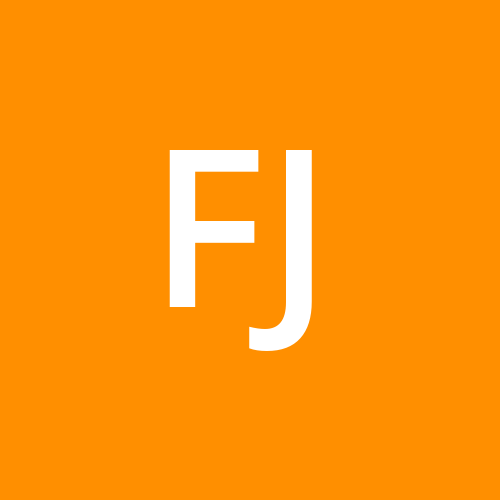