[Python] How to Add Hyperlink to PDF without Acrobat | Detailed Instructions

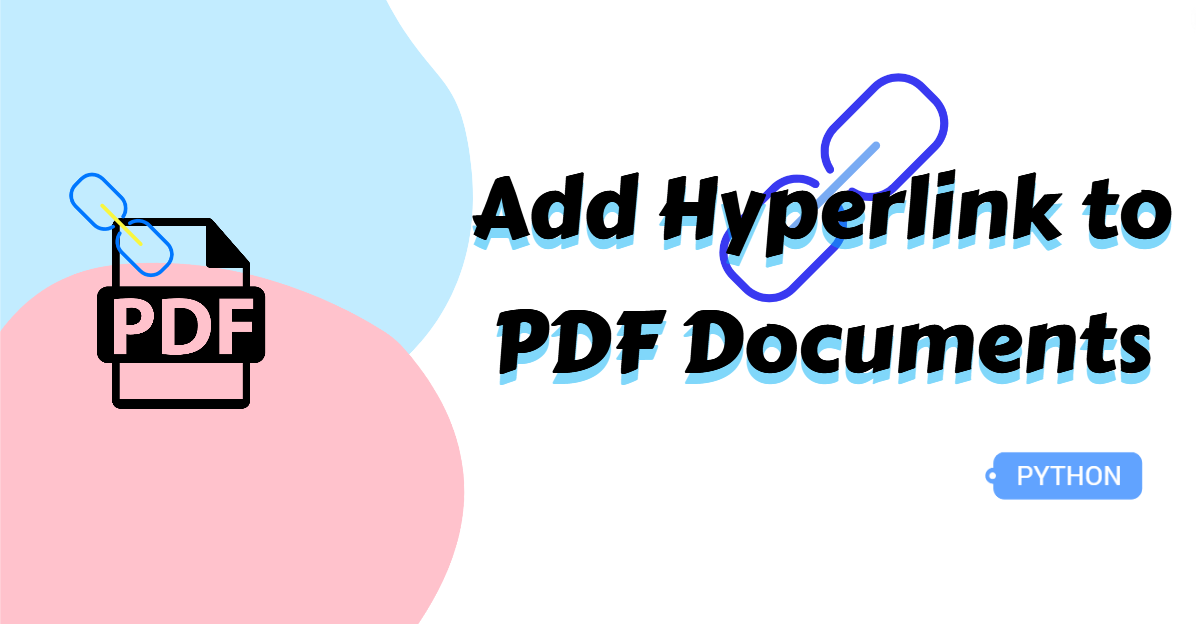
Adding hyperlinks to PDF documents can greatly enhance their interactivity, allowing readers to easily access external websites or other related documents. However, embedding links in a PDF can be trickier than in Word documents. Fortunately, this article introduces a quick and effective approach to help you complete this task seamlessly. Read on to discover how to use Python to add hyperlinks to PDF files, creating a more engaging and resourceful document for your audience.
Python Library to Add Links to PDFs
To wrap up the assignment, we will use Spire.PDF for Python to demonstrate. This tool is one of the best alternatives to Adobe Acrobat with comprehensive features. It allows users to edit PDF documents and insert links without hassle.
You can install it using the pip command: pip install Spire.PDF
Add Hyperlinks to PDF Files Directly
Suppose you are now fully prepared. Let’s get down to the key point: inserting hyperlinks to PDF documents. In this section, we will guide you through adding four types of common links in PDF; they are basic text links, hypertext links, email links, and file links. Check out the details below and make your PDFs not only informative but interactive as well.
Steps to add hyperlinks to PDFs:
Create an object for the PdfDocument class.
Add a blank page to it using the PdfDocument.Pages.Add() method.
Initialize the x and y coordinates to determine where the text be placed.
Create objects of the PdfTrueTypeFont class to customize font style for links.
Add a link to the page: (here take the hypertext link as an example.) Create the PdfTextWebLink object. Then set the displayed text, link URL, font, and color for the link. Insert the link in the PDF page by calling the PdfTextWebLink.DrawTextWebLink() method.
Save the resulting PDF file with the PdfDocument.SaveToFile() method and close the document.
Here is the code example of adding simple text links, hypertext links, email links and a file link to the blank page in PDF:
from spire.pdf.common import *
from spire.pdf import *
# Create a PdfDocument instance
pdf = PdfDocument()
# Add a page to the PDF document
page = pdf.Pages.Add()
# Initialize x, y coordinates
y = 30.0
x = 10.0
# Create true type fonts
font = PdfTrueTypeFont("Arial", 14.0,PdfFontStyle.Regular,True)
font1 = PdfTrueTypeFont("Arial", 14.0, PdfFontStyle.Underline,True)
# Add a basic text link
label = "Simple Text Link: "
format = PdfStringFormat()
format.MeasureTrailingSpaces = True
page.Canvas.DrawString(label, font, PdfBrushes.get_Orange(), 0.0, y, format)
x = font.MeasureString(label, format).Width
url = "http://www.e-iceblue.com"
page.Canvas.DrawString(url, font1, PdfBrushes.get_Blue(), x, y)
y = y + 28
# Add a hypertext link
label = "Hypertext Link: "
page.Canvas.DrawString(label, font, PdfBrushes.get_Orange(), 0.0, y, format)
x = font.MeasureString(label, format).Width
webLink = PdfTextWebLink()
webLink.Text = "Home Page"
webLink.Url = url
webLink.Font = font1
webLink.Brush = PdfBrushes.get_Blue()
webLink.DrawTextWebLink(page.Canvas, PointF(x, y))
y = y + 28
# Add an Email link
label = "Email Link: "
page.Canvas.DrawString(label, font, PdfBrushes.get_Orange(), 0.0, y, format)
x = font.MeasureString(label, format).Width
link = PdfTextWebLink()
link.Text = "Contact Us"
link.Url = "mailto:support@e-iceblue.com"
link.Font = font1
link.Brush = PdfBrushes.get_Blue()
link.DrawTextWebLink(page.Canvas, PointF(x, y))
y = y + 28
# Add a file link
label = "Document Link: "
page.Canvas.DrawString(label, font, PdfBrushes.get_Orange(), 0.0, y, format)
x = font.MeasureString(label, format).Width
text = "Open File"
location = PointF(x, y)
size = font1.MeasureString(text)
linkBounds = RectangleF(location, size)
fileLink = PdfFileLinkAnnotation(linkBounds,"C:\\Users\\Administrator\\Desktop\\Report.xlsx")
fileLink.Border = PdfAnnotationBorder(0.0)
page.AnnotationsWidget.Add(fileLink)
page.Canvas.DrawString(text, font1, PdfBrushes.get_Blue(), x, y)
# Save the result pdf file
pdf.SaveToFile("AddLinkstoPDF.pdf")
# Close the document
pdf.Close()
Add Hyperlinks to Existing Text in PDF Documents
Adding hyperlinks to existing text in a PDF is another essential capability, especially when you’re working with pre-written documents that require additional external references. Unlike adding hyperlinks to new content, this process involves locating the text where the link is needed and then applying the AnnotationsWidget.Add() method to insert the link. Let’s walk through the exact steps for accomplishing this.
Steps to add hyperlinks to existing text in PDF:
Create a PdfDocument instance, and specify the file path to load a PDF document using the PdfDocument.LoadFromFile() method.
Get a certain page with the PdfDocument.Pages[] method.
Find the text on the page using the Pages.FindText() method.
Instantiate a PdfUriAnnotation class.
Set the URL, and configure the border and color of the hyperlink by creating instances of the PdfAnnotationBorder and PdfRGBColor classes, respectively.
Add the link to the text by calling the AnnotationsWidget.Add() method.
Save the updated PDF as a new file with the PdfDocument.SaveToFile() method and release the resource.
Below is a code example that demonstrates how to add hyperlinks to every instance of the term “big O notation” found on the first page:
from spire.pdf.common import *
from spire.pdf import *
# Create a PdfDocument instance
pdf = PdfDocument()
# Load a PDF file
pdf.LoadFromFile("input.pdf")
# Get the first page
page = pdf.Pages[0]
# Find all occurrences of the specified text on the page
collection = page.FindText("big O notation", TextFindParameter.IgnoreCase)
# Loop through all occurrences of the specified text
for find in collection.Finds:
# Create a hyperlink annotation
uri = PdfUriAnnotation(find.Bounds)
# Set the URL of the hyperlink
uri.Uri = "https://en.wikipedia.org/wiki/Big_O_notation"
# Set the border of the hyperlink annotation
uri.Border = PdfAnnotationBorder(1.0)
# Set the color of the border
uri.Color = PdfRGBColor(Color.get_Blue())
# Add the hyperlink annotation to the page
page.AnnotationsWidget.Add(uri)
#Save the result file
pdf.SaveToFile("SearchTextAndAddHyperlink.pdf")
# Release the resource
pdf.Close()
The Conclusion
This guide covers how to add hyperlinks to PDFs using Python, including instructions for inserting links both on a blank page and directly onto existing text. With detailed steps and code examples, you’ll have a clear understanding of each process. We hope this helps you add interactive elements to your PDFs with ease!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
