Boost Your Next.js Workflow with Prettier: A Quick Setup Guide
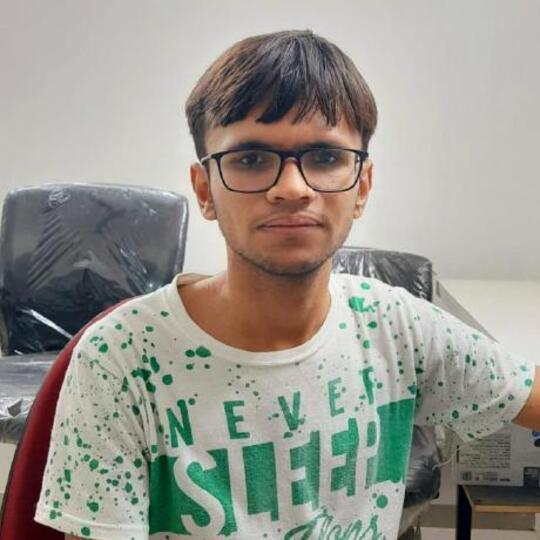
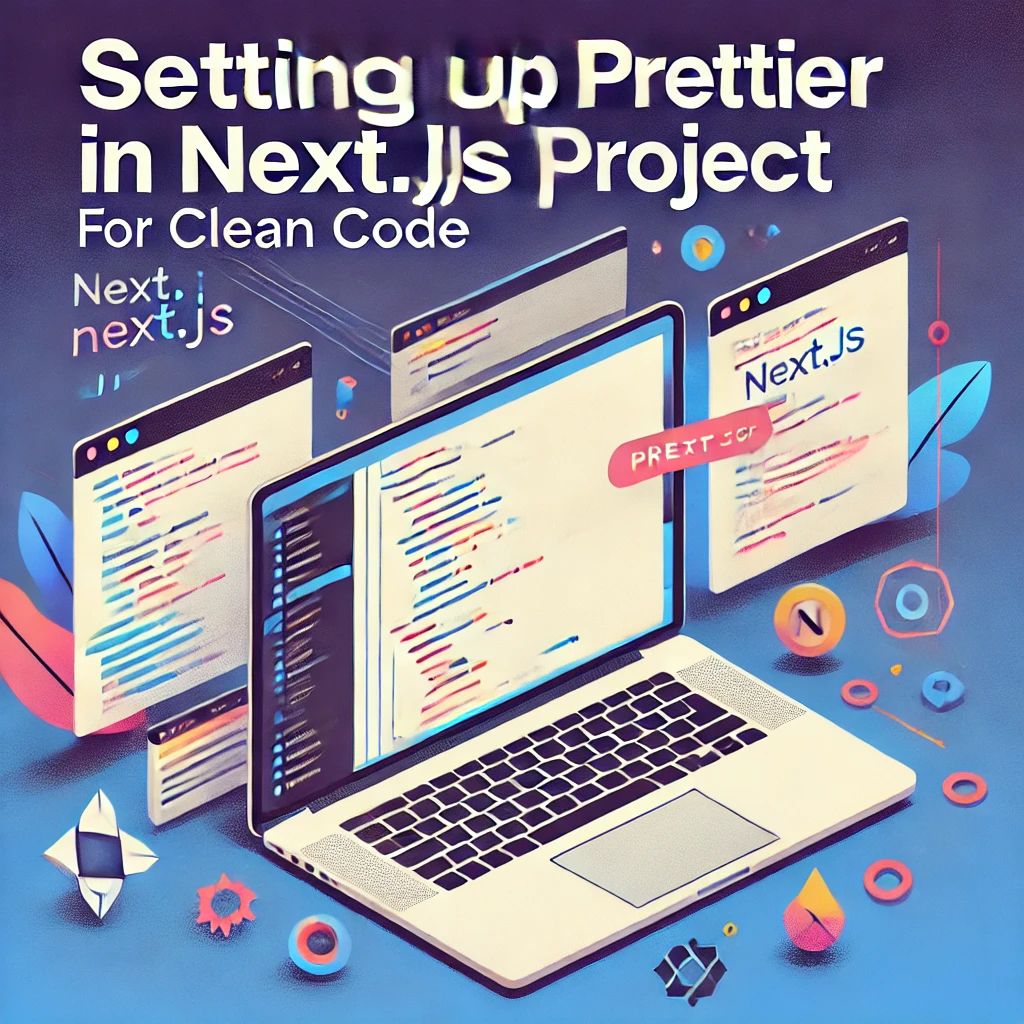
What is Prettier?
Prettier is an automatic code formatting tool. It takes your code and applies a consistent style, such as indentation, spacing, and line breaks, based on rules you set or its defaults. This helps make your code look clean and uniform, so everyone on a team writes in the same style.
Why use Prettier?
Consistent Style: Prettier formats code the same way every time, making everything look neat and uniform.
Automatic Formatting: It takes care of things like indentation, spacing, and line breaks, saving you time.
Easier to Read: Clean, consistently styled code is easier for everyone to read and understand.
Reduces Errors: By keeping code organized, Prettier helps prevent small mistakes.
Improves Teamwork: Everyone on a team follows the same style, making it easier to work together.
Saves Time: You don’t have to manually fix formatting issues; Prettier does it for you automatically.
Setting Up Prettier in Next.js
Step 1: Install Prettier and Required Packages
npm install --save-dev prettier eslint-config-prettier eslint-plugin-prettier
Step 2: Configure Prettier
Create a .prettierrc
file in the root of your project with your preferred formatting options. Below is an example configuration used in real-world projects:
{
"semi": true,
"trailingComma": "es5",
"singleQuote": true,
"printWidth": 80,
"tabWidth": 4,
"useTabs": false,
"bracketSpacing": true,
"jsxBracketSameLine": false,
"arrowParens": "always",
"endOfLine": "lf"
}
Step 3: Configure ESLint to Work with Prettier
Ensure that ESLint does not conflict with Prettier by modifying your .eslintrc.json
file:
{ "extends": [ "next", "next/core-web-vitals", "prettier" ],
"plugins": ["prettier"],
"rules": { "prettier/prettier": "error" }}
Step 4: Create a Prettier Ignore File
Add a .prettierignore
file to prevent Prettier from formatting specific files or directories, similar to .gitignore
. This helps keep unnecessary files
node_modules
build
dist
.next
out
coverage
public
Step 5: Configure Scripts for Formatting
Add a script to your package.json
to format the codebase easily:
"scripts": {
"format": "prettier --write ."
}
Step 6: Run Prettier
npm run format
Summary
In a Next.js project, Prettier automatically formats the code to keep it neat and consistent. It manages spacing, indentation, and line breaks, making the code easier to read and reducing mistakes. Prettier ensures that everyone on the team writes code in the same style, saving time and making teamwork easier.
Subscribe to my newsletter
Read articles from Nayan Radadiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
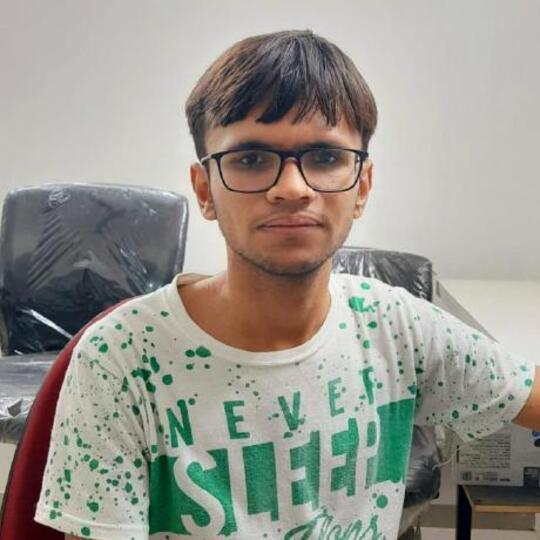
Nayan Radadiya
Nayan Radadiya
Experienced Frontend Developer with 2.5 years of expertise in Next.js, React.js, TypeScript, Javascript (ES6+), Redux, and React Native. Self-taught in blockchain development with a focus on pursuing a career as a Web3 Fronted developer. Proficient in reading smart contracts and integrating them with the frontend side.