Understanding Global and Local Scope in JavaScript

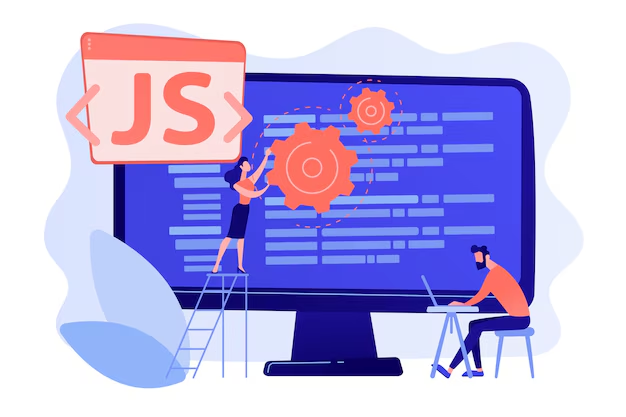
JavaScript is a powerful and versatile programming language, and one of its most important features is how it handles scope. Understanding the difference between global and local scope is crucial for writing clean, efficient, and bug-free code. In this article, we'll explore what global and local scope are, how they work in JavaScript, and how they impact your code.
What is Scope in JavaScript?
In programming, scope refers to the context in which a variable or function is accessible. In JavaScript, scope determines where a variable or function can be referenced or modified. JavaScript uses two main types of scope: global scope and local scope.
Global Scope
Variables and functions that are defined in the global scope are accessible from anywhere in your JavaScript code. They exist outside of any function or block and can be referenced and manipulated throughout the entire program.
In the browser, the global scope is represented by the window
object, whereas in Node.js, it is the global
object.
Example of Global Scope:
javascriptCopy codevar globalVar = "I'm a global variable";
function showGlobalVar() {
console.log(globalVar); // Can access the global variable
}
showGlobalVar(); // Output: "I'm a global variable"
In the example above, the variable globalVar
is declared in the global scope, so it can be accessed both inside the showGlobalVar()
function and outside of it.
However, be cautious when using global variables. If a global variable is modified or overwritten somewhere in your code, it could lead to bugs that are difficult to trace. This is why limiting the use of global variables is often considered a good practice in JavaScript.
Local Scope
On the other hand, local scope refers to variables that are only accessible within a specific block of code—usually inside a function. These variables are created when the function is invoked and are destroyed once the function finishes executing.
Variables declared inside a function with var
, let
, or const
are local to that function. Local scope can also be nested, meaning a function inside another function can access its parent function's variables, but not vice versa.
Example of Local Scope:
javascriptCopy codefunction localScopeExample() {
var localVar = "I'm a local variable";
console.log(localVar); // Accessible inside the function
}
localScopeExample(); // Output: "I'm a local variable"
console.log(localVar); // Error: localVar is not defined
In this example, localVar
is only accessible inside the localScopeExample()
function. Trying to access it outside the function will result in a ReferenceError
, as it is out of scope.
Block Scope (ES6 and Beyond)
Before ES6 (ECMAScript 2015), JavaScript had function-level scope, meaning variables declared with var
were scoped to the function they were declared in, not the block. This could lead to unexpected behavior, especially inside loops or conditional statements.
With the introduction of ES6, the let
and const
keywords were introduced to provide block-level scope. Variables declared with let
or const
are only accessible within the block (enclosed by curly braces {}
) in which they are defined.
Example of Block Scope with let
and const
:
javascriptCopy codeif (true) {
let blockScopedVar = "I'm scoped to this block";
const blockScopedConst = "I cannot be reassigned";
console.log(blockScopedVar); // Accessible here
}
console.log(blockScopedVar); // Error: blockScopedVar is not defined
console.log(blockScopedConst); // Error: blockScopedConst is not defined
In this case, blockScopedVar
and blockScopedConst
are only accessible inside the if
block. Once the block is exited, they are no longer available.
Global vs. Local Scope: Key Differences
Accessibility:
Global Scope: Variables are accessible throughout the entire program.
Local Scope: Variables are only accessible within the function or block in which they are defined.
Lifetime:
Global Scope: Variables exist for the duration of the program.
Local Scope: Variables are created when the function or block is executed and are destroyed when the execution context ends.
Namespace Pollution:
Global Scope: If too many variables are declared in the global scope, it can lead to namespace pollution, making it harder to track and manage variable names and values.
Local Scope: Variables in local scope are confined to their respective functions or blocks, reducing the risk of conflicts.
Performance:
Global Scope: Overusing global variables can negatively impact performance, as they are always available and might lead to unnecessary lookups or overwriting.
Local Scope: Local variables are typically faster to access and cleaner to use, as they are discarded after execution.
Best Practices for Managing Scope
Minimize the Use of Global Variables: Avoid creating too many global variables. Instead, try to encapsulate your logic inside functions or modules. This minimizes the risk of accidental overwrites and keeps your code organized.
Use
let
andconst
for Local Variables: Always preferlet
andconst
for declaring variables inside functions and blocks. They provide block-level scoping and avoid the pitfalls ofvar
, which can have function-level scope.Use Closures to Control Scope: JavaScript closures allow you to create private variables that are inaccessible from outside a function. This can help with data encapsulation and reducing the risk of accidental manipulation.
Modularize Your Code: By using JavaScript modules (ES6 modules or CommonJS in Node.js), you can create separate scopes for different parts of your application, preventing variable conflicts and promoting better code organization.
Subscribe to my newsletter
Read articles from shubham kabsuri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
