JavaScript Promises Explained: The Easiest Guide You’ll Ever Need!
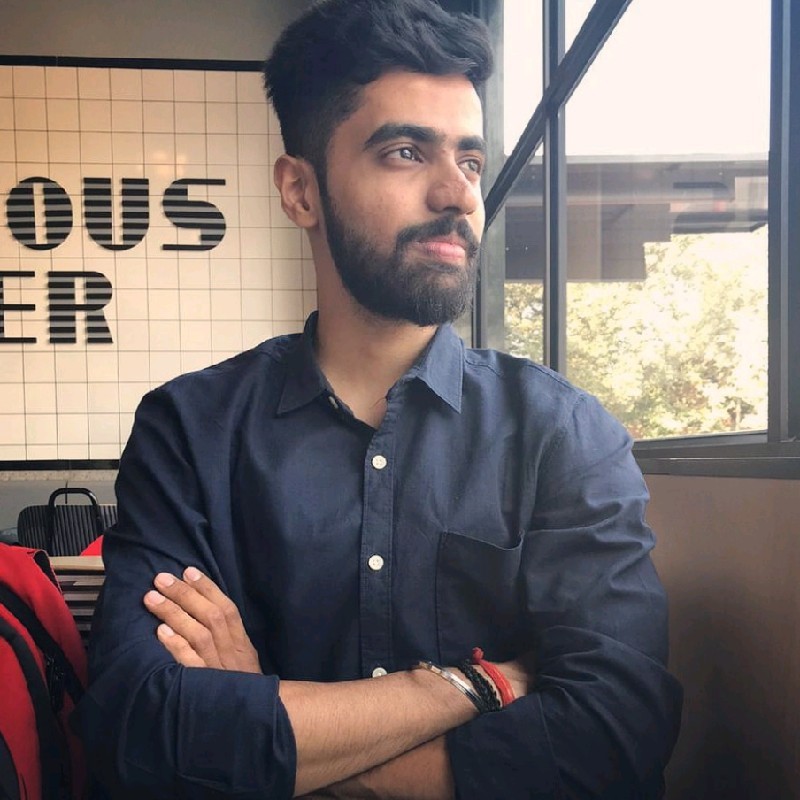
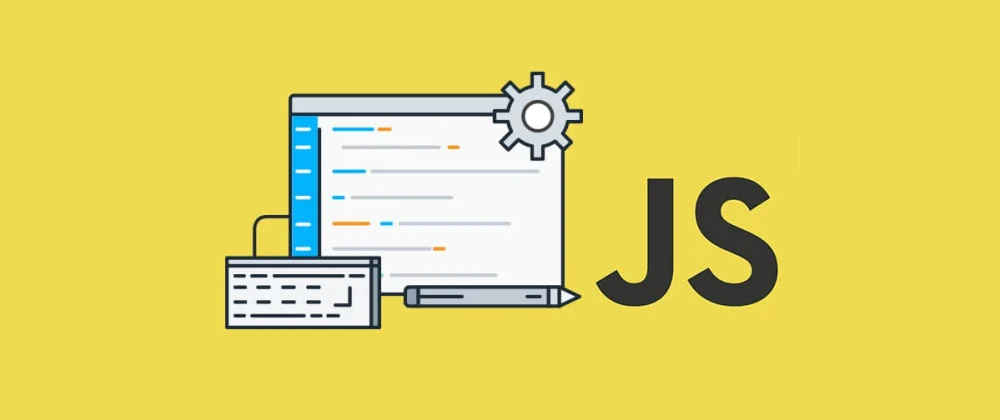
Introduction
Promises in JavaScript can feel a bit like magic—but once you get the hang of them, they’re incredibly useful! In this guide, we’ll break down JavaScript promises in a way that’s easy to understand and remember. By the end, you’ll have a clear picture of how promises work, why they’re so important, and how to use async
and await
for even cleaner, more readable code.
What Exactly is a Promise?
A promise in JavaScript is like placing an order at a restaurant. You know your food will be ready at some point, but it’s not there instantly. Instead, the restaurant "promises" that it will serve you as soon as it’s done.
A JavaScript promise is similar: it represents an operation that hasn’t completed yet but will do so in the future.
The 3 States of a Promise
Pending: This is the initial state of a promise—like when you’ve placed your order and are waiting for the food. The promise is in progress, and you don’t know the outcome yet.
Fulfilled (Resolved): Your food is finally ready! The promise successfully completes, giving you a result (like “Your food is ready!”).
Rejected: The restaurant can’t fulfill your order due to an issue, such as missing ingredients. This means the promise is rejected and won’t deliver what you asked for.
How to Use Promises
In JavaScript, promises are created with the Promise
constructor. Here’s a quick example that follows the restaurant analogy:
let foodPromise = new Promise((resolve, reject) => {
let foodReady = true; // Change this to false to test rejection
if (foodReady) {
resolve("Your food is ready!");
} else {
reject("Sorry, we’re out of ingredients.");
}
});
In this example, we create a new foodPromise
that takes two callbacks: resolve
(fulfilled) and reject
(rejected). If foodReady
is true, it resolves with "Your food is ready!" Otherwise, it rejects with "Sorry, we’re out of ingredients."
Handling Promises with .then()
and .catch()
JavaScript gives us .then()
and .catch()
to handle promises:
.then()
is called when the promise is fulfilled..catch()
is called when there’s a rejection.
foodPromise
.then((message) => console.log(message)) // When food is ready
.catch((error) => console.log(error)); // When food is out of stock
The code above will print "Your food is ready!" if the promise is fulfilled, or "Sorry, we’re out of ingredients" if it’s rejected.
Enter async
and await
– A Better Way to Handle Promises
Handling promises with .then()
and .catch()
is fine, but sometimes it can get messy when you have multiple promises or long chains of .then()
calls. This is where async
and await
come to the rescue, allowing us to write asynchronous code that looks and behaves more like synchronous code.
How async
and await
Work
async
: Use this keyword to define a function that will return a promise.await
: This pauses the execution inside theasync
function until the promise resolves, allowing you to write cleaner code.
Here’s our earlier foodPromise
example, but now using async
and await
:
async function orderFood() {
try {
let message = await foodPromise;
console.log(message); // If resolved, prints "Your food is ready!"
} catch (error) {
console.log(error); // If rejected, prints "Sorry, we’re out of ingredients."
}
}
orderFood();
In this example:
The
await
keyword pauses theorderFood
function untilfoodPromise
resolves.If the promise is fulfilled,
message
contains the result, and we log "Your food is ready!".If the promise is rejected, the
catch
block handles it, logging "Sorry, we’re out of ingredients."
This syntax makes code much more readable by removing the need for multiple .then()
and .catch()
calls.
Real-World Example with async
and await
: Fetching Data from an API
To show you the true power of async
and await
, let’s write our API call example using them:
async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchData();
With async
and await
, this code is both simpler and easier to follow. It does the same thing as before, but now the await
keywords pause execution until each promise resolves. If there’s an error at any step, the catch
block handles it.
Wrapping Up
Promises make JavaScript a lot more efficient by allowing it to handle multiple tasks without waiting around. By understanding the basics of pending, fulfilled, and rejected states, plus .then()
, .catch()
, async
, and await
, you’re now ready to handle asynchronous tasks with confidence.
Closing Call-to-Action
If you found this article helpful, please leave a comment or share your thoughts below!
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
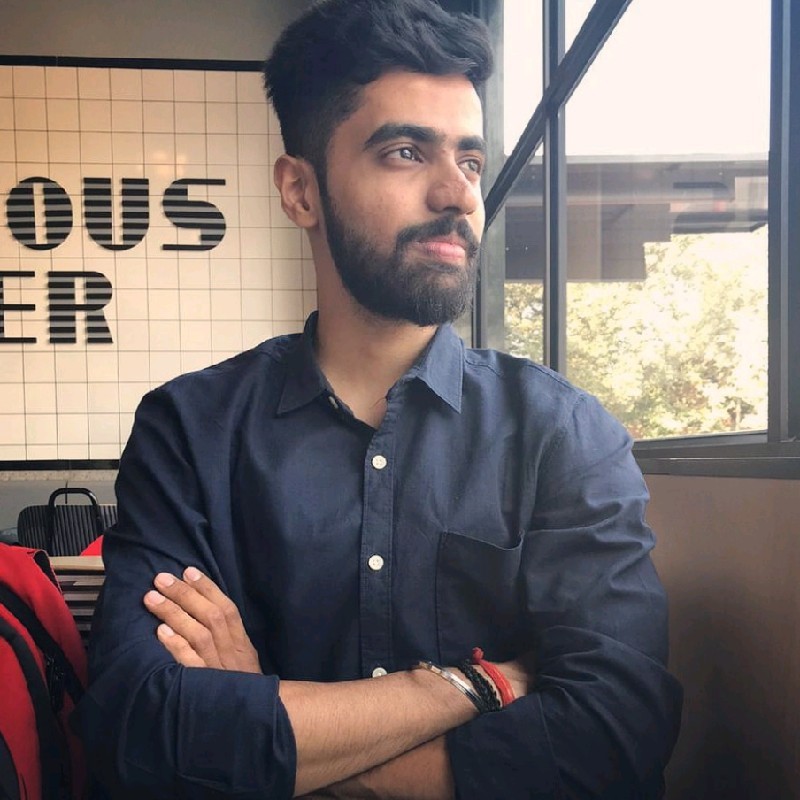