Angular Cheat Sheet
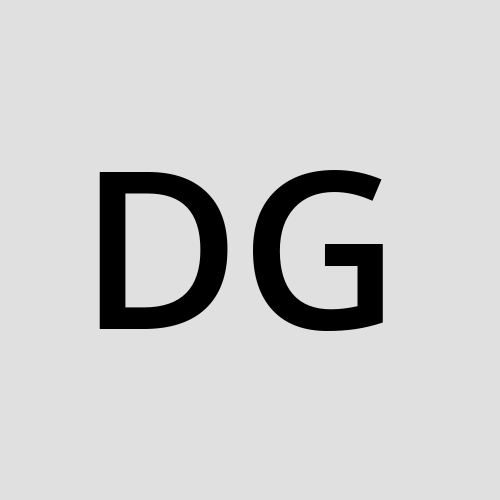

Here’s an Angular cheat sheet with key commands, concepts and syntax:
Basic Setup
Install Angular CLI:
npm install -g @angular/cli
Create a New Angular Project:
ng new project-name
Serve the Application:
ng serve
Build the Application:
ng build
Angular CLI Commands
Generate a Component:
ng generate component component-name
Generate a Service:
ng generate service service-name
Generate a Module:
ng generate module module-name
Generate a Directive:
ng generate directive directive-name
Generate a Pipe:
ng generate pipe pipe-name
Run Tests:
ng test
Run End-to-End (E2E) Tests:
ng e2e
App Structure
src/app:
app.component.ts: Main app component.
app.module.ts: Root module file.
app-routing.module.ts: Manages app routes (generated with
--routing
flag).
Components
Basic Component Structure:
import { Component } from '@angular/core'; @Component({ selector: 'app-component-name', templateUrl: './component-name.component.html', styleUrls: ['./component-name.component.css'] }) export class ComponentNameComponent { title = 'Hello, Angular!'; }
Component Binding:
Interpolation:
{{ variable }}
Property Binding:
[property]="value"
Event Binding:
(event)="function()"
Two-Way Binding:
[(ngModel)]="value"
Directives
Built-in Structural Directives:
<div *ngIf="condition">Content</div> <div *ngFor="let item of items">{{ item }}</div>
Built-in Attribute Directives:
<div [ngClass]="{'class-name': condition}">Content</div> <div [ngStyle]="{'background-color': color}">Content</div>
Pipes
Using Pipes:
<p>{{ dateValue | date:'fullDate' }}</p> <p>{{ amount | currency:'USD' }}</p>
Custom Pipe:
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'customPipe' }) export class CustomPipe implements PipeTransform { transform(value: string): string { return value.toUpperCase(); } }
Services & Dependency Injection
Generate a Service:
ng generate service service-name
Inject a Service in a Component:
import { Component } from '@angular/core'; import { ExampleService } from './example.service'; @Component({ /* component metadata */ }) export class ExampleComponent { constructor(private exampleService: ExampleService) {} }
Creating an HTTP Service with HttpClient:
Import
HttpClientModule
inapp.module.ts
:import { HttpClientModule } from '@angular/common/http'; @NgModule({ imports: [HttpClientModule], // other imports }) export class AppModule {}
Inject
HttpClient
in the service:import { HttpClient } from '@angular/common/http'; export class ExampleService { constructor(private http: HttpClient) {} getData() { return this.http.get('api/url'); } }
Routing
Define Routes in app-routing.module.ts:
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, { path: '**', redirectTo: '' } // wildcard route for 404 ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule {}
Router Outlet in Template:
<router-outlet></router-outlet>
Router Link:
<a routerLink="/about">About</a>
Forms
Template-Driven Form:
<form #myForm="ngForm" (ngSubmit)="onSubmit(myForm)"> <input name="name" ngModel required> <button type="submit">Submit</button> </form>
Reactive Form:
import { FormGroup, FormControl, Validators } from '@angular/forms'; export class ExampleComponent { form = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]) }); onSubmit() { console.log(this.form.value); } }
Observables & RxJS
Basic Observable:
import { Observable } from 'rxjs'; const observable = new Observable(observer => { observer.next('Hello'); observer.complete(); }); observable.subscribe(value => console.log(value));
Using Operators (e.g.,
map
andfilter
):import { of } from 'rxjs'; import { map, filter } from 'rxjs/operators'; of(1, 2, 3, 4) .pipe( filter(value => value % 2 === 0), map(value => value * 10) ) .subscribe(result => console.log(result));
HttpClient with Observable:
import { HttpClient } from '@angular/common/http'; import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class DataService { constructor(private http: HttpClient) {} fetchData() { return this.http.get('api/data'); } }
Lifecycle Hooks
Common Lifecycle Hooks:
import { Component, OnInit, OnDestroy, AfterViewInit } from '@angular/core'; @Component({ /* component metadata */ }) export class ExampleComponent implements OnInit, OnDestroy, AfterViewInit { ngOnInit() { console.log('OnInit called'); } ngAfterViewInit() { console.log('AfterViewInit called'); } ngOnDestroy() { console.log('OnDestroy called'); } }
Angular Modules
Declare Components and Services in Modules:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { ExampleComponent } from './example/example.component'; @NgModule({ declarations: [AppComponent, ExampleComponent], imports: [BrowserModule], providers: [], bootstrap: [AppComponent] }) export class AppModule {}
Feature Modules with Routing:
import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { FeatureComponent } from './feature/feature.component'; import { RouterModule } from '@angular/router'; @NgModule({ declarations: [FeatureComponent], imports: [ CommonModule, RouterModule.forChild([{ path: '', component: FeatureComponent }]) ] }) export class FeatureModule {}
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
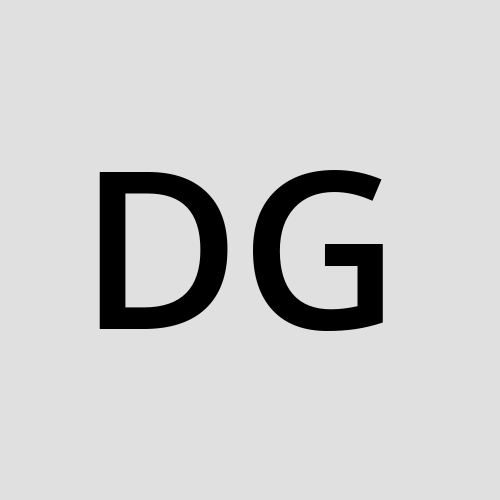
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.