The Difference Between find() and findById() in MongoDB

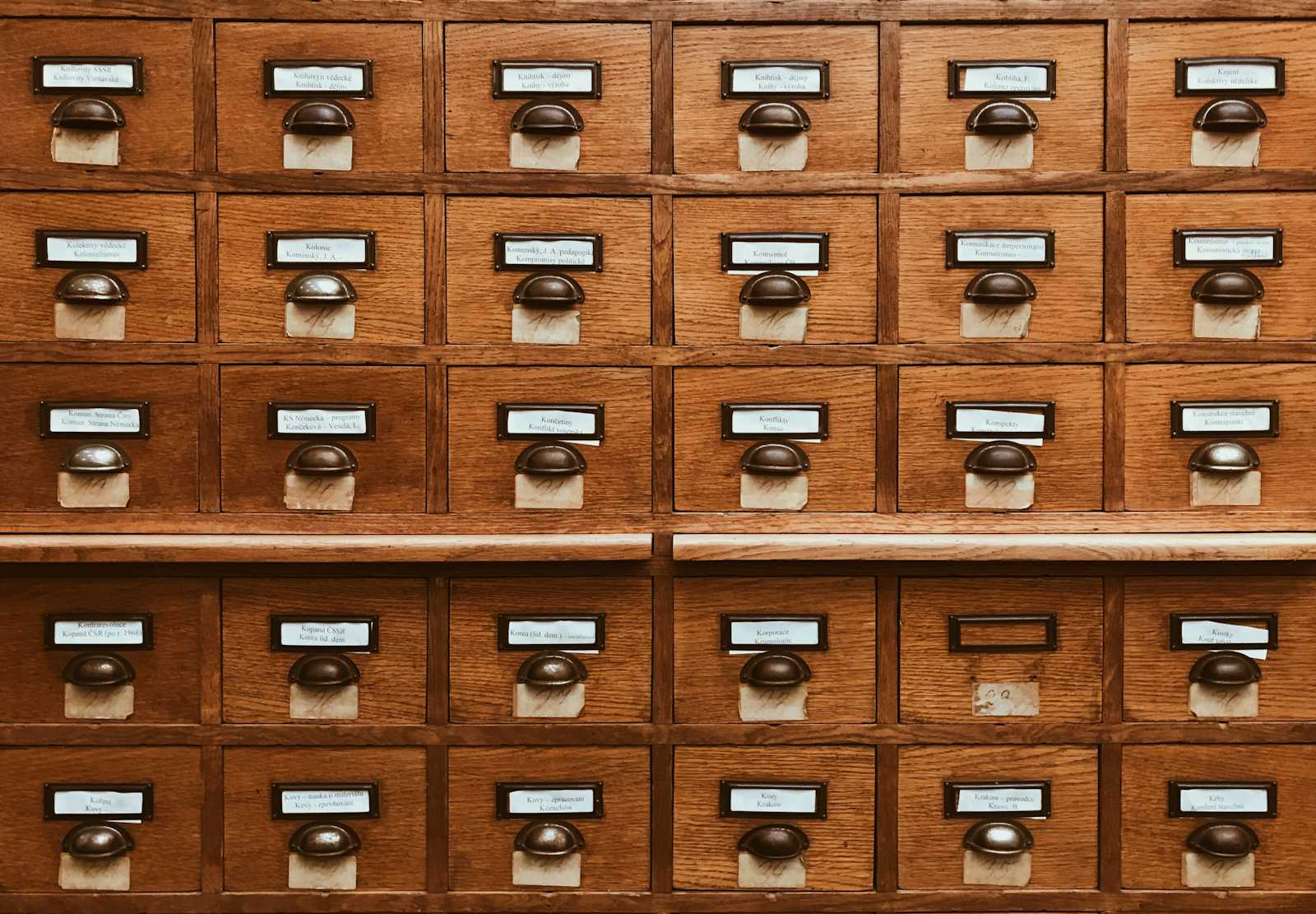
If you're working with MongoDB, you've likely encountered the find()
and findById()
methods. While both are used to retrieve documents from a database, they have some key differences that are important to understand.find({object}) findById(string)
find()
: The General-Purpose Query Method
The find()
method is a versatile way to query a MongoDB collection and retrieve one or more documents that match the specified criteria. You pass a query object to find()
that can contain various query operators, like $gt
, $lte
, $in
, and more. This allows you to construct complex queries to filter the results exactly how you need.
For example, if you wanted to find all users who are over 30 years old and live in the "New York" location, you could use a query like this:
javascriptCopydb.users.find({
age: { $gt: 30 },
location: "New York"
})
The find()
method returns a cursor object, which you can then use to iterate over the matching documents.
findById()
: The Shorthand for Unique IDs
In contrast, the findById()
method is a shorthand way to retrieve a single document by its unique _id
field. This method is faster than find()
because it uses the indexed _id
field, which is an optimized lookup operation.
If you know the unique ID of the document you want to retrieve, you can simply pass that ID to findById()
:
javascriptCopydb.users.findById("6374a2b3c9e7d8c0d1e4b5c6")
The findById()
method will return the single document object (or null
if no match is found).
When to Use Each Method
Use findById()
when you know the unique _id
of the document you want to retrieve. This is the fastest way to get a single document.
Use find()
when you need to perform more complex queries or retrieve multiple documents that match certain criteria. The flexibility of the find()
method makes it useful for a wide variety of query scenarios.
Ultimately, understanding the differences between find()
and findById()
will help you write more efficient and effective MongoDB queries in your applications.
Subscribe to my newsletter
Read articles from Tejas Shinde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
