Arrow Functions and More: Modern JavaScript Syntax!
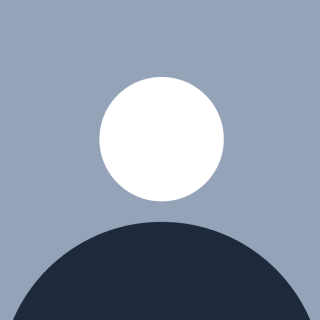
Table of contents
- Defining Arrow Functions
- Understanding the 'this' Keyword in Arrow Functions
- Arrow Functions and 'this'
- Flowchart: How 'this' Works in Different Contexts
- Unveiling the Beauty of Arrow Functions
- Rewriting Classic Functions as Arrow Functions
- Arrow Functions in Practice — Tackling Real Challenges
- Mastering the 'this' Keyword with Arrow Functions
- Mastering Modern JavaScript Syntax
- Let’s explore Memory Management!
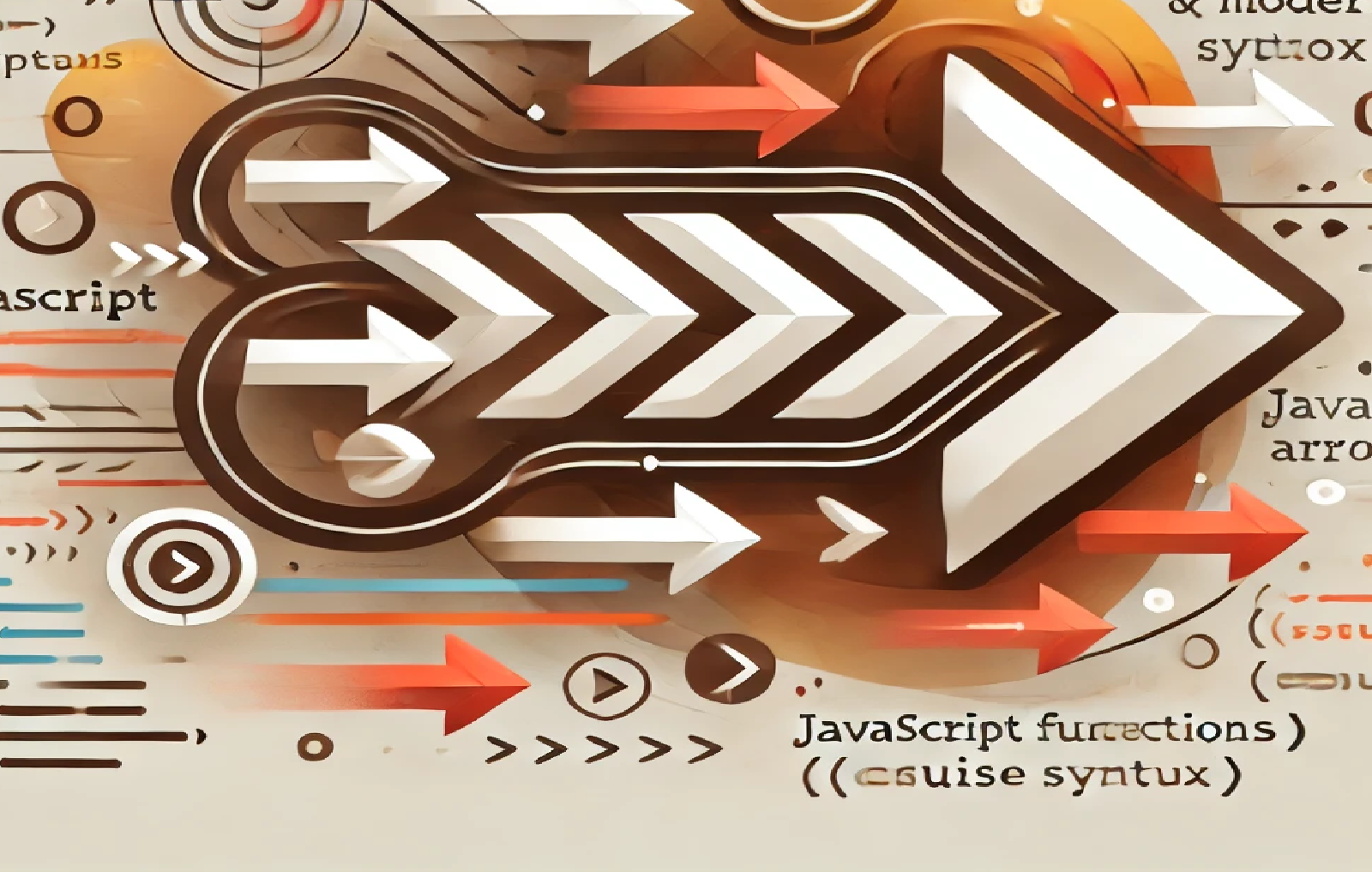
Imagine walking through a modern art gallery where every piece of art is minimal, clean, and elegant. Just as modern artists use simple forms to convey powerful ideas, JavaScript’s arrow functions are a sleek and concise way to write functions—stripping away unnecessary syntax and making your code more readable and expressive.
Arrow functions were introduced in ES6 (ECMAScript 2015) and have since become a popular feature in modern JavaScript, offering a shorthand syntax for defining functions while also addressing some peculiarities with the this
keyword.
In this article, we’ll explore how arrow functions work, how to define them, and why they’re such a powerful tool for writing cleaner, more efficient code in JavaScript.
Defining Arrow Functions
Arrow functions are a more concise way to define functions. They have a simpler syntax compared to traditional function expressions and eliminate the need for the function
keyword.
Syntax of an Arrow Function
The basic syntax of an arrow function looks like this:
(parameter) => {
// Code block
}
For a single line of code, you can even omit the curly braces and return value explicitly:
(parameter) => expression
If you have no parameters, simply use empty parentheses:
() => console.log("Hello, World!");
Code Snippet: Arrow Function Syntax
Let’s start by rewriting a classic function as an arrow function. Below is a comparison between a traditional function expression and an arrow function.
Traditional Function Expression:
const sayHello = function(name) {
return "Hello, " + name;
};
console.log(sayHello("Alice")); // Output: "Hello, Alice"
Arrow Function:
const sayHello = (name) => "Hello, " + name;
console.log(sayHello("Alice")); // Output: "Hello, Alice"
Explanation:
The arrow function syntax removes the
function
keyword and simplifies the structure.If the function body contains only a single line that returns a value, you can omit the curly braces and the
return
keyword.
Arrow functions not only streamline your code, but they also behave differently in terms of the this
keyword, which we’ll explore in the next section.
Understanding the 'this' Keyword in Arrow Functions
One of the most important distinctions between arrow functions and traditional functions lies in how they handle the this
keyword. In a traditional function, this
refers to the object that called the function. However, in an arrow function, this
is lexically bound, meaning it takes the value of this
from the surrounding code context.
Traditional Functions and 'this'
In regular functions, this
can be tricky, especially when you try to use it inside another function or callback.
function traditionalFunction() {
console.log(this); // Refers to the object that called the function
}
const myObj = {
name: "Object",
getName: function() {
traditionalFunction(); // 'this' will refer to the global object or undefined in strict mode
}
};
myObj.getName(); // Logs the global object
Arrow Functions and 'this'
Arrow functions behave differently. They do not have their own this
, which means they inherit this
from their surrounding scope.
const myObj = {
name: "Object",
getName: function() {
const arrowFunction = () => console.log(this.name);
arrowFunction(); // 'this' refers to myObj, not the global object
}
};
myObj.getName(); // Output: "Object"
Explanation:
- In the example above, the arrow function inherits
this
from its surrounding context, which is themyObj
object. This makes arrow functions a great choice for callbacks and event handlers where maintaining the correctthis
context can be challenging.
Flowchart: How 'this' Works in Different Contexts
Let’s visualize how this
behaves differently in traditional functions versus arrow functions:
[ Traditional Function ] [ Arrow Function ]
| |
'this' refers to: 'this' refers to:
- Calling object - Lexically scoped (inherited from outer scope)
- Undefined (in strict mode) - Remains the same inside function
This flowchart helps illustrate that this
in arrow functions is always determined by the surrounding context, which avoids many of the confusing behaviors seen in traditional functions.
Unveiling the Beauty of Arrow Functions
In this part, we’ve explored how arrow functions offer a clean and concise syntax for defining functions, making them a key component of modern JavaScript. With their simplified structure and lexical handling of this
, arrow functions are perfect for creating elegant, readable, and efficient code—like modern art pieces in the gallery of JavaScript.
In the next part, we’ll dive deeper into practical challenges, including rewriting classic functions as arrow functions and exploring more complex use cases.
Rewriting Classic Functions as Arrow Functions
To truly understand the elegance of arrow functions, it’s helpful to practice converting traditional function expressions into arrow functions. This will give you hands-on experience with how arrow functions streamline your code while maintaining the same functionality.
Let’s rewrite a few common examples, demonstrating how arrow functions can make your code cleaner.
Example 1: Summing Two Numbers
Traditional Function:
const sum = function(a, b) {
return a + b;
};
console.log(sum(3, 5)); // Output: 8
Arrow Function:
const sum = (a, b) => a + b;
console.log(sum(3, 5)); // Output: 8
Explanation:
- We removed the
function
keyword and reduced the syntax to a single line. Since there is only one expression, there’s no need for curly braces or thereturn
keyword.
Example 2: Finding Even Numbers in an Array
Traditional Function:
const isEven = function(number) {
return number % 2 === 0;
};
console.log(isEven(4)); // Output: true
Arrow Function:
const isEven = (number) => number % 2 === 0;
console.log(isEven(4)); // Output: true
Explanation:
- Again, the arrow function condenses the syntax while keeping the logic the same, making the code more readable and concise.
Example 3: Greeting Someone
Traditional Function:
const greet = function() {
return "Hello!";
};
console.log(greet()); // Output: "Hello!"
Arrow Function:
const greet = () => "Hello!";
console.log(greet()); // Output: "Hello!"
Explanation:
- With no parameters, the arrow function uses empty parentheses. The result is the same, but the syntax is more compact.
Arrow Functions in Practice — Tackling Real Challenges
Now that you understand how to convert traditional functions into arrow functions, let’s dive into some challenges where you’ll put arrow functions to work.
Challenge 1: Rewrite Array Methods with Arrow Functions
Arrays in JavaScript come with built-in methods like map()
, filter()
, and reduce()
, and these methods often benefit from using arrow functions for brevity and readability. Your task is to rewrite the following functions using arrow functions.
Example:
let numbers = [1, 2, 3, 4, 5];
// Traditional map function
let doubled = numbers.map(function(num) {
return num * 2;
});
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Rewrite as Arrow Function:
let doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Challenge 2: Create a List of Names and Use Arrow Functions
Scenario: You have an array of people’s names, and you need to filter out names shorter than 4 characters and then capitalize them. Use arrow functions to accomplish this.
let names = ["Amy", "Bob", "Charlie", "Dan", "Eve"];
// Filter and map using arrow functions
let filteredAndCapitalized = names
.filter(name => name.length >= 4)
.map(name => name.toUpperCase());
console.log(filteredAndCapitalized); // Output: ["CHARLIE"]
Explanation:
- The arrow functions are used within the
filter()
andmap()
methods to both filter and transform the data in a concise manner.
Challenge 3: Create a Timer Function with Arrow Functions
Scenario: Write a timer function that counts down from a given number of seconds, printing each second. Use arrow functions within setTimeout()
to handle the countdown.
let countdown = (seconds) => {
let interval = setInterval(() => {
console.log(seconds);
if (seconds === 0) {
clearInterval(interval);
console.log("Time’s up!");
}
seconds--;
}, 1000);
};
countdown(5); // Countdown from 5 seconds
Explanation:
- Arrow functions are used within
setInterval()
to ensure the correct handling ofthis
and to keep the syntax compact.
Mastering the 'this' Keyword with Arrow Functions
As we discussed earlier, one of the most powerful features of arrow functions is how they handle the this
keyword. In complex applications, the behavior of this
can be confusing, especially when functions are nested or used as callbacks. Arrow functions simplify this by inheriting this
from their lexical context, making your code more predictable.
Let’s take a look at a real-world scenario where using arrow functions resolves issues with this
.
Code Snippet: 'this' in a Class with Traditional Functions
In traditional functions, this
can sometimes refer to the global object or become undefined
, especially in event handlers or callbacks.
class Car {
constructor(model) {
this.model = model;
}
startEngine() {
setTimeout(function() {
console.log(this.model + " engine started.");
}, 1000);
}
}
let myCar = new Car("Tesla");
myCar.startEngine(); // Output: undefined engine started.
In this example, this
inside the setTimeout()
function does not refer to the car object, but rather to the global object, leading to an incorrect output.
Code Snippet: Fixing 'this' with Arrow Functions
By using arrow functions, this
remains lexically bound to the surrounding scope (the Car
object), resolving the issue.
class Car {
constructor(model) {
this.model = model;
}
startEngine() {
setTimeout(() => {
console.log(this.model + " engine started.");
}, 1000);
}
}
let myCar = new Car("Tesla");
myCar.startEngine(); // Output: Tesla engine started.
Explanation:
- The arrow function in
setTimeout()
inheritsthis
from thestartEngine
method, correctly referring to theCar
instance.
Mastering Modern JavaScript Syntax
Arrow functions are one of the most significant features introduced in modern JavaScript, simplifying function definitions and improving how this
is handled in callbacks and event handlers. By adopting arrow functions, you not only make your code more readable but also avoid many common pitfalls associated with traditional functions.
From rewriting classic functions to handling more complex scenarios involving this
, arrow functions are a vital tool in your JavaScript toolkit—much like how modern art simplifies forms to their essence, allowing the beauty of simplicity to shine through.
Let’s explore Memory Management!
In the next article, we’ll dive into memory management in JavaScript—understanding how memory is allocated, used, and freed up during program execution. Get ready to optimize your code by learning how to manage memory efficiently!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by