Day 19 Task: Docker for DevOps Engineers – Mastering Docker Volume & Docker Network
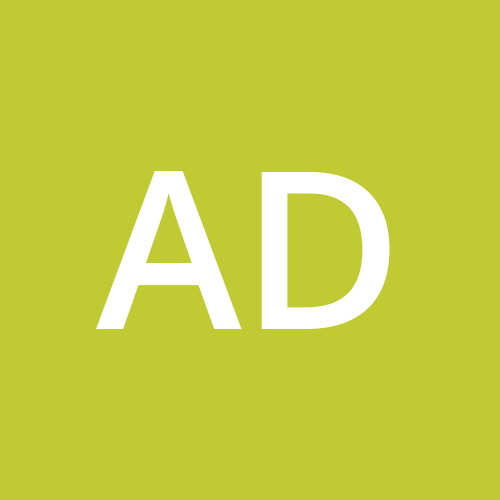
Docker Volumes
Docker Volumes are essential for persisting data that lives outside a container’s lifecycle. Here’s why they’re a powerful feature:
Persistent Storage: By default, when a container is removed, all data inside it is lost. Volumes allow us to store data outside containers, so it persists even if the container is deleted or stopped.
Data Sharing Across Containers: We can mount the same volume to multiple containers to allow them to share data seamlessly.
Examples of Use: Storing database data in volumes is a common practice so that data is saved even when the database container is updated or restarted.
Docker Networks
Docker Networks enable containers to communicate with each other as well as the host machine. They offer several networking capabilities:
Virtual Networks: Containers connected to the same network can communicate directly, making it easier to connect applications with their services (like a database).
Isolation: By isolating containers on separate networks, you can add a layer of security and control.
Use Cases: Docker networks are commonly used for multi-container applications, where services like web servers, databases, and application backends need to communicate seamlessly.
Task 1: Create a Multi-Container Docker-Compose File
Example docker-compose.yml
Below is an example of a docker-compose.yml
file to set up a Node.js application and a MySQL database using Docker Compose.
version: '3.8'
services:
app:
image: node:14
container_name: node_app
ports:
- "3000:3000"
environment:
DB_HOST: db
DB_USER: root
DB_PASSWORD: example
DB_NAME: mydb
volumes:
- app-data:/usr/src/app
depends_on:
- db
networks:
- app-network
db:
image: mysql:5.7
container_name: mysql_db
environment:
MYSQL_ROOT_PASSWORD: example
MYSQL_DATABASE: mydb
volumes:
- db-data:/var/lib/mysql
networks:
- app-network
volumes:
app-data:
db-data:
networks:
app-network:
Command Breakdown
Bring Up Containers in Detached Mode:
docker-compose up -d
Scale the App Service:
docker-compose up -d --scale app=3
Check Container Status:
docker-compose ps
View Logs:
docker-compose logs app
Bring Down Containers and Clean Up:
docker-compose down
Task 2: Using Docker Volumes and Named Volumes
Create Containers with Shared Volume Run two containers that share the same volume to read and write data.
docker run -d --name container1 --mount source=shared-data,target=/data alpine docker run -d --name container2 --mount source=shared-data,target=/data alpine
Verify Data Sharing Across Containers Use the
docker exec
command to write and verify data across containers:docker exec container1 sh -c "echo 'Hello from container1' > /data/shared-file.txt" docker exec container2 cat /data/shared-file.txt
If data is shared correctly, container2 should output:
Hello from container1
List and Remove Volumes
List All Volumes:
docker volume ls
Remove a Volume:
docker volume rm shared-data
Project Opportunity
Use this task to build a portfolio project by combining Docker Volumes and Networks in a real-world scenario. Document your work and post on LinkedIn with #90DaysOfDevOps to showcase your skills to the community!
Subscribe to my newsletter
Read articles from Amit singh deora directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
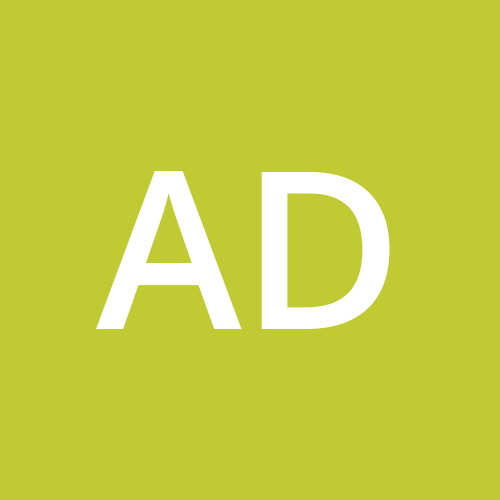