"Hello World" in Python, Java, and Scala: A Quick Dive into Spark Data Analysis.

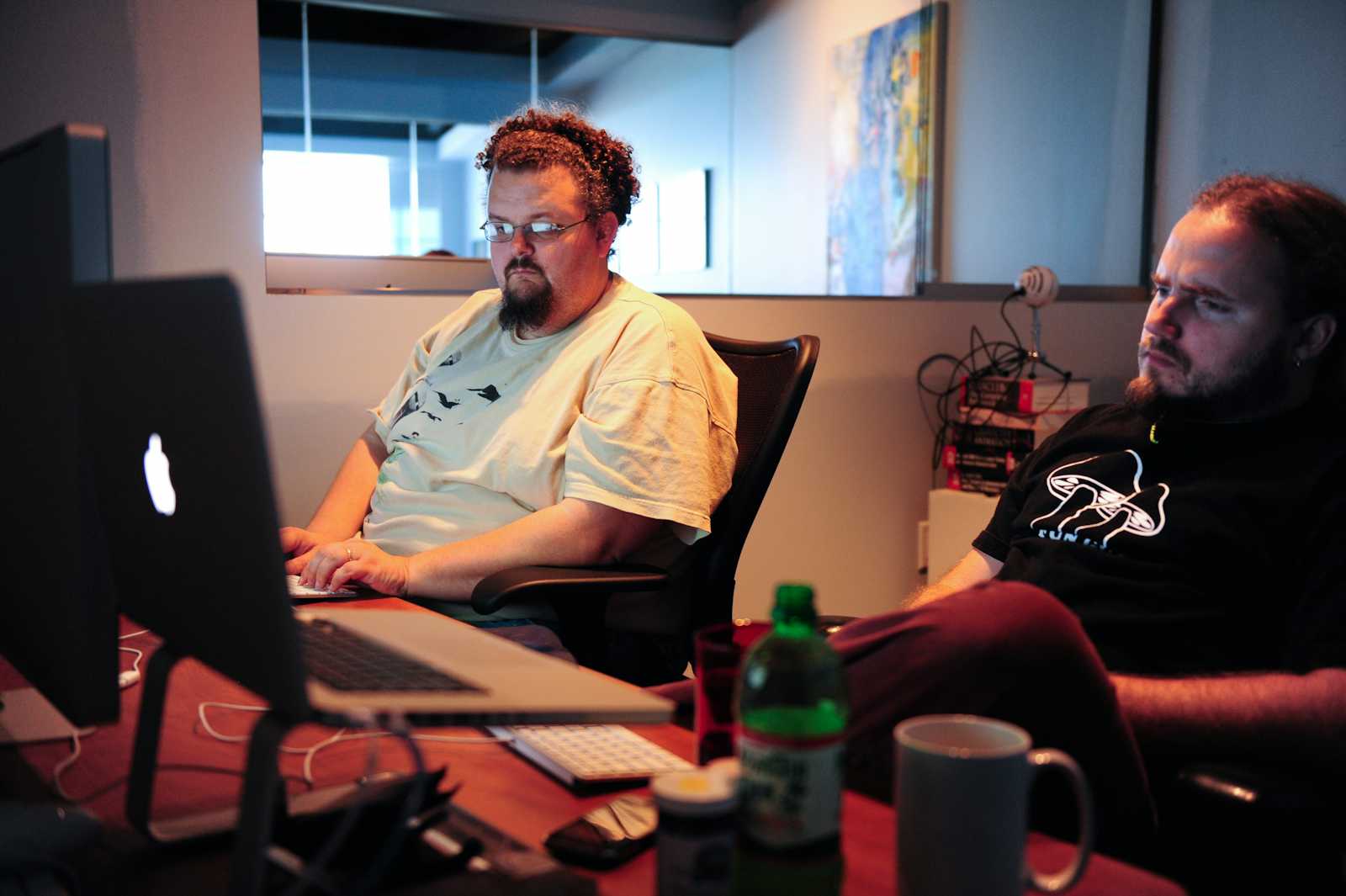
The "Hello World" program is the simplest way to demonstrate the syntax of a programming language. By writing a "Hello World" program in Python, Java, and Scala, we can explore how each language introduces us to coding concepts, and then delve into their uses in Spark, especially for data analysis.
1. Writing a "Hello World" Program in Each Language
Python "Hello World"
Python is known for its simplicity and readability, making it a popular choice for beginners and data scientists. Here's how you write "Hello World" in Python:
print("Hello, World!")
- Explanation: The
print()
function outputs the text "Hello, World!" to the console. Python's syntax is straightforward, requiring minimal boilerplate code.
Java "Hello World"
Java, a statically-typed and object-oriented programming language, is known for its extensive use in enterprise applications and frameworks. Here's the Java version:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Explanation:
The program defines a class
HelloWorld
.public static void main(String[] args)
is the entry point of the program.System.out.println
prints the text to the console. Java requires more boilerplate compared to Python, reflecting its verbosity.
Scala "Hello World"
Scala is a functional and object-oriented language often used in conjunction with Apache Spark. Here’s how you write "Hello World" in Scala:
object HelloWorld {
def main(args: Array[String]): Unit = {
println("Hello, World!")
}
}
Explanation:
object HelloWorld
defines a singleton object.def main(args: Array[String]): Unit
defines the main method.println
is used to print to the console. Scala has more concise syntax than Java but is slightly more complex than Python.
2. Comparing Python, Java, and Scala for Data Analysis in Spark
Apache Spark is a powerful distributed data processing framework widely used for big data and machine learning. Each language—Python, Java, and Scala—offers unique strengths when working with Spark:
2.1. Python for Spark (PySpark)
Ease of Use: Python is easy to learn and widely used in the data science community. PySpark is an API for using Spark with Python, simplifying data processing tasks.
Libraries: Python’s ecosystem includes rich data analysis libraries like Pandas, NumPy, and SciPy, which are easily integrable with PySpark.
Performance: PySpark is generally slower than native Spark operations in Scala or Java due to serialization and the communication overhead between Python and the Java Virtual Machine (JVM).
Use Case: PySpark is excellent for quick prototyping, data exploration, and interactive data analysis tasks, particularly when working with Jupyter Notebooks.
2.2. Java for Spark
Performance: Java provides excellent performance, as Spark is natively written in Scala, which runs on the JVM. Using Java eliminates certain overheads compared to Python.
Complexity: Java is more verbose and requires more lines of code for even simple tasks, making it less convenient for writing data analysis scripts.
Enterprise Use: Java is well-suited for enterprise environments where code maintainability and integration with large, Java-based systems are priorities.
Use Case: Java is preferred for building robust, enterprise-grade Spark applications that require high performance and long-term maintainability.
2.3. Scala for Spark
Native Language: Spark is written in Scala, so using Scala provides direct access to all Spark features and optimizations, making Scala the most efficient language for Spark.
Conciseness: Scala's functional programming features and concise syntax make it suitable for writing complex data transformations.
Learning Curve: Scala has a steeper learning curve compared to Python, which might be a hurdle for those not familiar with functional programming.
Use Case: Scala is ideal for performance-critical Spark applications and for those who want full access to the latest Spark features and APIs. It is often chosen for production environments.
3. Choosing the Right Language for Spark Data Analysis
When to Use Python (PySpark)
If you are a data scientist familiar with Python libraries and want to perform data analysis quickly.
For data exploration, machine learning, and prototyping.
If you prefer working in a familiar, interactive environment like Jupyter Notebook.
When to Use Java
If your organization has an existing Java infrastructure and you need to integrate Spark seamlessly.
When performance and enterprise-level maintainability are crucial.
For building complex, large-scale, and production-grade Spark applications.
When to Use Scala
If you require the highest performance and full access to Spark’s features.
For production systems and real-time data processing pipelines.
If you are experienced with functional programming and want concise, efficient code.
Conclusion
The choice between Python, Java, and Scala for data analysis in Spark depends on your project requirements, team expertise, and performance needs. PySpark simplifies data processing and is widely used in data science. Java offers stability for enterprise-level projects, while Scala provides unparalleled performance and access to Spark’s full capabilities. Understanding the strengths of each language will help you make informed decisions for your data analysis tasks in Spark.
Subscribe to my newsletter
Read articles from Jitender Kaushik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
