JavaScript Functions: Regular vs Constructor Functions Explained

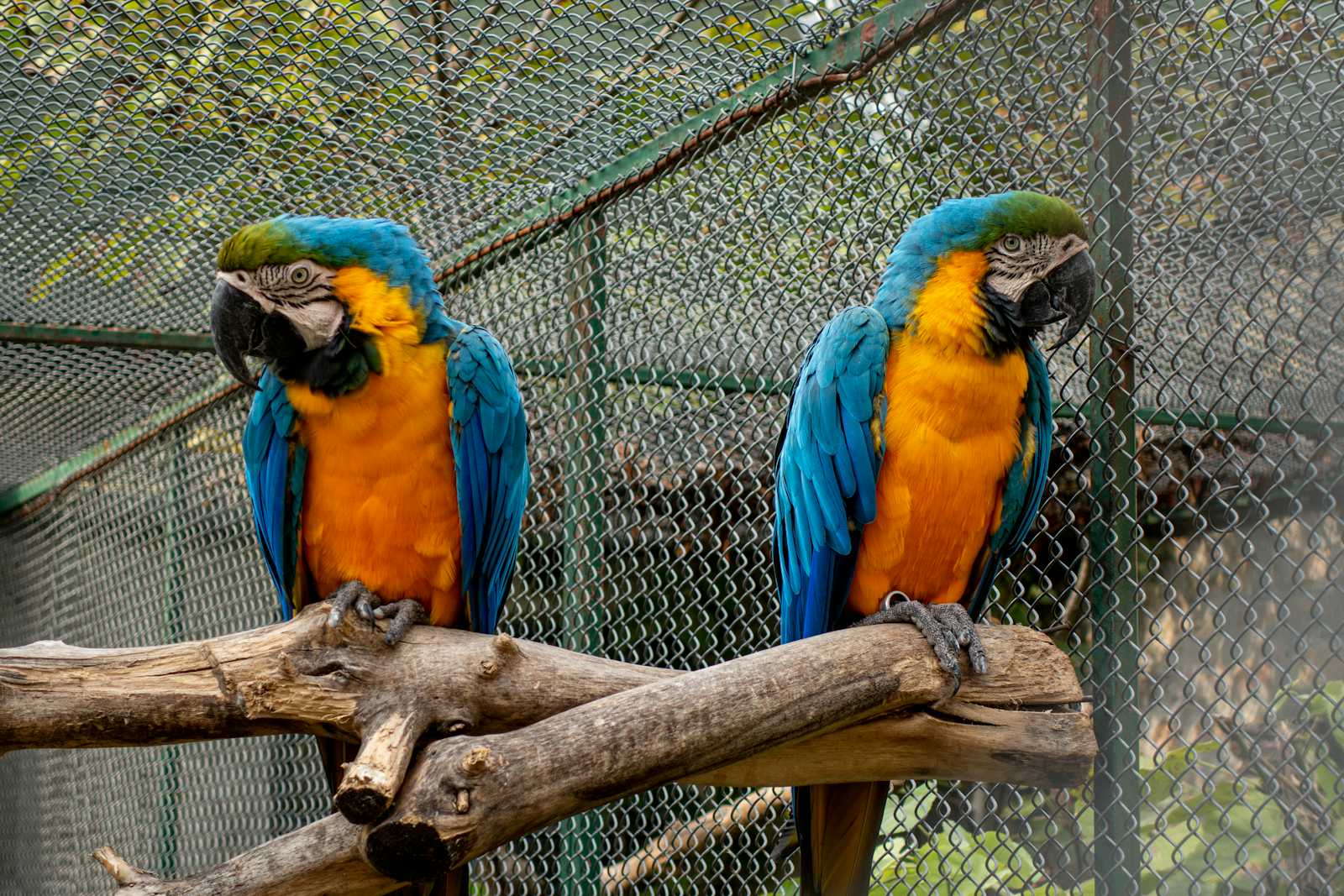
1. Purpose
Function: A regular function is used to define reusable blocks of code, perform specific tasks, or calculate values. It’s called simply by its name.
Constructor Function: A constructor function is intended to create instances of objects with similar properties and methods, acting as a "blueprint" for objects.
2. Naming Conventions
Function: Regular functions typically follow camelCase or snake_case naming conventions.
function myFunction() { /* ... */ }
Constructor Function: Constructor functions follow PascalCase (first letter of each word capitalized) by convention, to signal that they should be used with
new
.function Person() { /* ... */ }
3. Usage with new
Function: Regular functions are called directly without
new
.myFunction();
Constructor Function: Constructor functions are used with the
new
keyword to create an instance.new
sets up a new context (an empty object) and assignsthis
to that new object.const person = new Person();
4. this
Binding
Function: In regular functions,
this
is determined by how the function is called. It could refer to the global object (in non-strict mode),undefined
(in strict mode), or a specified context in methods or event handlers.Constructor Function: When called with
new
, the constructor function’sthis
automatically refers to the new instance being created. This lets you add properties and methods directly tothis
, which becomes the new instance.
5. Returning Values
Function: Regular functions can return any value. If no return statement is specified, they return
undefined
by default.Constructor Function: Constructor functions typically do not have a return statement. If they return a primitive (like
string
,number
, etc.), it’s ignored, and the new object is returned. However, if a constructor explicitly returns an object, that object will be returned instead of the new instance.function Person(name) { this.name = name; return { age: 25 }; // This replaces the new instance } const person = new Person('Alice'); console.log(person); // { age: 25 }
6. Prototype
Function: Regular functions don’t have a prototype object associated with them in the same way. You typically don’t use
prototype
for regular functions.Constructor Function: Constructor functions have a
prototype
property, which can be used to add methods that will be shared across all instances created with that constructor. This is key for memory efficiency, as methods defined on the prototype are not duplicated for each instance.function Person(name) { this.name = name; } Person.prototype.greet = function() { console.log(`Hello, my name is ${this.name}`); }; const person = new Person('Alice'); person.greet(); // Hello, my name is Alice
7. Intended Use
Function: Regular functions are used for any block of code that you want to execute, perform tasks, or calculate results without creating an instance of an object.
Constructor Function: Constructor functions are used specifically for creating multiple instances of similar objects, giving each one unique properties while sharing common behaviors via the prototype.
Summary Table
Feature | Regular Function | Constructor Function |
Purpose | Perform tasks, calculate results | Create new object instances |
Naming Convention | camelCase or snake_case | PascalCase |
Usage with new | Not used with new | Always used with new |
this Binding | Depends on how called | Refers to new instance |
Return Value | Returns specified value or undefined if none | Returns new instance by default |
Prototype | Not used or relevant | Has a prototype for shared methods |
Intended Use | Any reusable code | Blueprint for creating objects |
In short, while both regular functions and constructor functions are technically functions, constructor functions are specifically designed to help create instances of objects with shared structure and behavior, making them essential for object-oriented programming in JavaScript.
Subscribe to my newsletter
Read articles from kotesh_Mudila directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
