๐ Exploring new Map() in JavaScript: A Powerful Data Structure for Developers ๐บ๏ธ

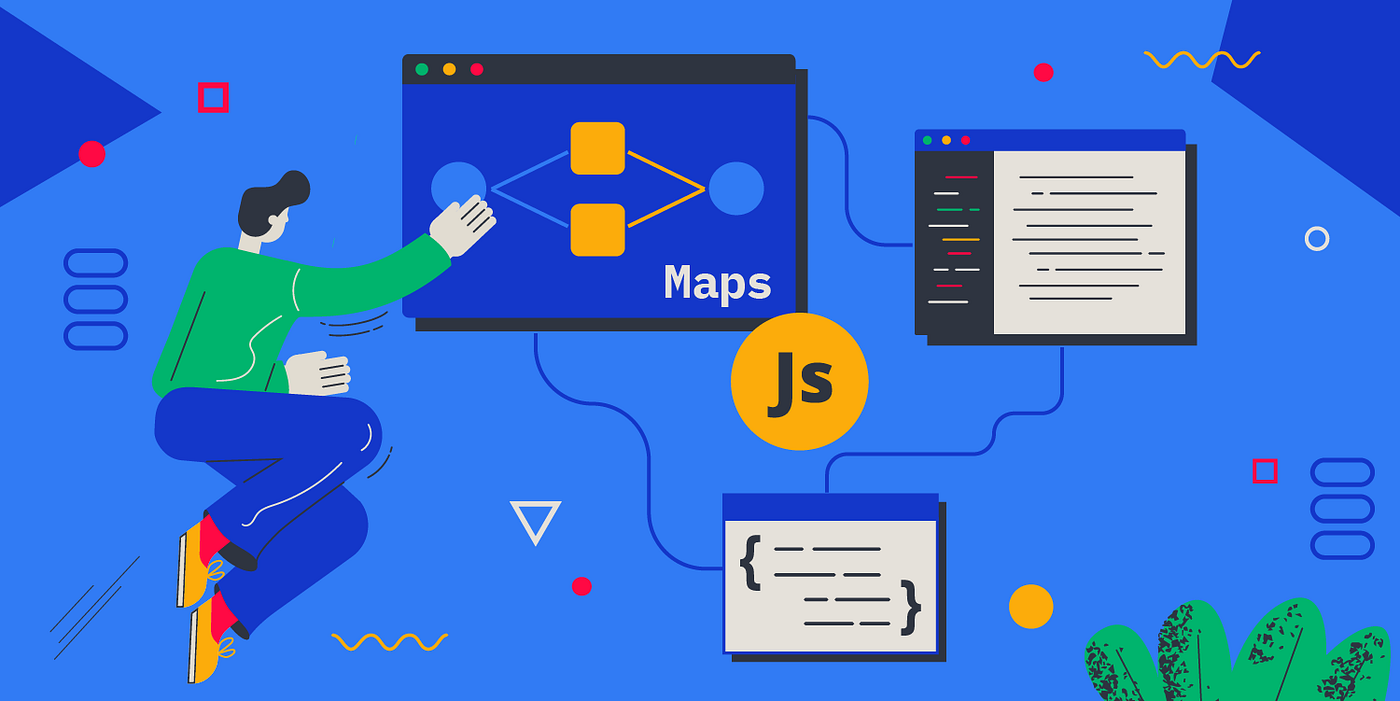
JavaScript offers a variety of ways to store data, and while arrays and objects are great, sometimes you need something even more powerful and flexible. Enter Map
! ๐ก
In this article, weโll break down what the Map
object is, why itโs useful, and how you can leverage it for more efficient data management. Letโs dive in! ๐
๐ What is a Map
?
A Map
in JavaScript is a special object that lets you store key-value pairs. ๐๏ธโก๏ธ๐ฆ Unlike regular objects where keys are always strings or symbols, a Map
allows you to use any type of key, including objects, numbers, and even functions! ๐ฒ
Think of Map
as an enhanced object that comes with some nifty features, such as maintaining insertion order and supporting complex keys.
๐ Key Features of Map
Any Data Type as a Key ๐: Whether it's a string, number, object, or functionโ
Map
lets you use anything as a key! ๐๏ธKeeps Insertion Order ๐งฉ: When you iterate over a
Map
, it keeps the order in which the items were added, unlike regular objects, which donโt guarantee order. โณImproved Performance โก:
Map
performs better for frequent additions and deletions compared to objects, especially with large data sets. ๐Easy Size Property ๐: Want to know how many key-value pairs are in your
Map
? Just use.size
โno need to count manually! ๐ข
๐ ๏ธ How to Create a Map
Creating a Map
is super easy! You just use the new Map()
constructor:
javascriptCopy codeconst myMap = new Map();
You can also initialize it with key-value pairs right from the start:
javascriptCopy codeconst userRoles = new Map([
['Alice', 'admin'],
['Bob', 'editor'],
['Charlie', 'viewer']
]);
In this example, we created a Map
called userRoles
, where the keys are usersโ names and the values are their roles. ๐ฉโ๐ป๐จโ๐ป
โ๏ธ Common Operations with Map
Letโs look at some common operations youโll perform with Map
:
1. Adding Values with set()
๐
You can add key-value pairs to a Map
using .set()
:
javascriptCopy codemyMap.set('name', 'Yasin');
myMap.set('age', 25);
myMap.set(true, 'Boolean key');
Here, we added three key-value pairs, and notice how the keys can be of different types! ๐ฏ
2. Accessing Values with get()
๐
Want to get the value for a specific key? Use .get()
:
javascriptCopy codeconsole.log(myMap.get('name')); // Output: Yasin
If the key isnโt in the Map
, it will return undefined
. ๐คทโโ๏ธ
3. Checking for Keys with has()
โ
Need to check if a key exists in the Map
? .has()
has you covered:
javascriptCopy codeconsole.log(myMap.has('name')); // Output: true
4. Deleting Entries with delete()
๐๏ธ
To remove a key-value pair, use .delete()
:
javascriptCopy codemyMap.delete('age');
console.log(myMap.has('age')); // Output: false
5. Clearing All Entries with clear()
๐งน
Want to remove everything from the Map
? Use .clear()
:
javascriptCopy codemyMap.clear();
console.log(myMap.size); // Output: 0
6. Checking the Size with .size
๐
To find out how many items are in your Map
, use .size
:
javascriptCopy codeconsole.log(userRoles.size); // Output: 3
๐ Iterating Over a Map
Map
provides several methods to loop through its key-value pairs. Letโs explore a few:
forEach()
๐:
javascriptCopy codeuserRoles.forEach((value, key) => {
console.log(`${key} => ${value}`);
});
keys()
๐: To get all keys:
javascriptCopy codefor (let key of userRoles.keys()) {
console.log(key);
}
values()
๐งณ: To get all values:
javascriptCopy codefor (let value of userRoles.values()) {
console.log(value);
}
entries()
๐: To get both keys and values:
javascriptCopy codefor (let [key, value] of userRoles.entries()) {
console.log(`${key} => ${value}`);
}
๐ค When to Use Map
Over Objects?
So when should you pick Map
over a regular object? Here are some key scenarios:
When Keys Arenโt Just Strings ๐๏ธ: If you need to use objects, functions, or other types as keys,
Map
is your friend.When Order Matters ๐งฎ: If you want to ensure that your key-value pairs stay in the order you added them, use
Map
.Better Performance โก: If youโre working with a large dataset where keys are frequently added or removed,
Map
can outperform objects.Quick Size Checks ๐ข: Need to check the size often?
Map
โs.size
is easier and faster than counting object properties.
๐ Conclusion
The Map
object in JavaScript is a powerful tool for managing collections of key-value pairs. It offers flexibility with key types, maintains insertion order, and can be more efficient than traditional objects in many cases.
Whether youโre building web apps or handling complex data structures, Map
is an excellent choice for clean, maintainable code! ๐ปโจ
Happy coding! ๐
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.