My Python Learning Journey: Week 1 - Steps I Took, Problems I Faced, and How I Solved Them.

Table of contents
- Hey everyone, in this week, I learned about the following Python concepts:
- These are the steps I took to grasp the concepts:
- These are the problems that I encountered:
- This is how I solved those problems:
- 1. Resolving Import Errors when Working with Modules
- 2. Understanding between Static and Dynamic Typing
- 3. Confusion between single equal to (=) and double equal to (==) operators.
- 4. Python Error Encountered: TypeError - Combining Integers and Strings.
- 5. Missing f Prefix: A Common f-String (formatted string) Mistake.
- 6. Tuples cannot be changed after creation but the Lists can be modified.
- 7. List Sorting Error when inputs are strings instead of integers.
- 8. Error while trying to add a List to a Set in Python.
- These are the resources that helped me learn:
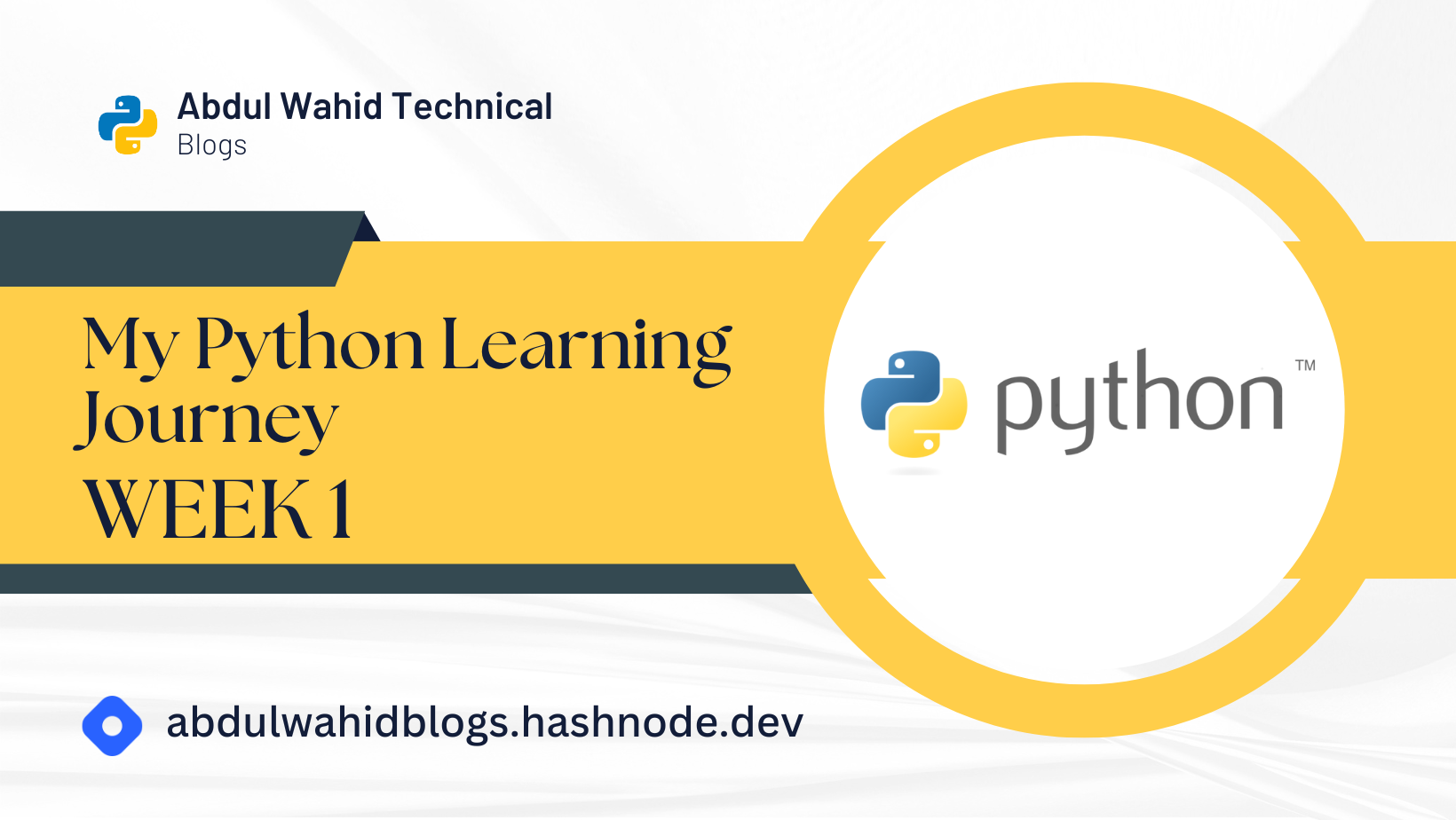
Hey everyone, in this week, I learned about the following Python concepts:
Variables & Data Types.
Modules, Comments & PIP.
Strings.
Lists & Tuples.
Dictionaries & Sets.
These are the steps I took to grasp the concepts:
Explored Modules, learning how to import and utilize external libraries.
Understood the importance of Comments for readable and maintainable code.
Installed packages using pip, Python's package manager.
Learned rules to define variable names, and how to assign values.
Studied Data Types, including Integers, Floats, Strings, Booleans, and None.
Worked with Strings, Mastering Concatenation, Slicing, and Formatted Strings.
Practiced creating and manipulating Lists, Tuples, Dictionaries, and Sets.
These are the problems that I encountered:
Resolving Import Errors when Working with Modules.
Understanding between Static and Dynamic Typing.
Confusion between single equal to (=) and double equal to (==) operators.
Python Error Encountered: TypeError - Combining Integers and Strings
Missing f Prefix: A Common f-String (formatted string) Mistake.
Tuples cannot be changed after creation but the Lists can be modified.
List Sorting Error when inputs are strings instead of integers.
Error while trying to add a List to a Set in Python.
This is how I solved those problems:
1. Resolving Import Errors when Working with Modules
Problem 1: Importing a module fails due to an incorrect module name or path.
Solution: Ensure the module is installed properly & verify the module name is correct.
# Incorrect import
import maths # "Error: Module 'maths' not found. Did you mean 'math'?"
# Correct import
import math
print(math.pi) # Output:3.141592653589793
Problem 2: Understanding Module Import Syntax.
Using math.pi
in Python without importing the math
module correctly.
Solution: To fix this error, you need to import the math
module before using its constants or functions
# Printing Module without Import
print(math.pi) #NameError: name 'math' is not defined
# Import entire module
import math
print(math.pi) # Output:3.141592653589793
# Import specific function or constant
from math import pi
print(pi) # Output:3.141592653589793
# Import with alias
import math as m
print(m.pi) # Output:3.141592653589793
2. Understanding between Static and Dynamic Typing
Problem: Confusion between static and dynamic typing in Python.
Solution: Python is dynamically typed which means variable types are determined at runtime.
# Dynamic typing
x = 5 # integer
print(type(x)) # Output:<class 'int'>
x = "hello" # string
print(type(x)) # Output:<class 'str'>
3. Confusion between single equal to (=)
and double equal to (==)
operators.
Problem: Beginners usually confuse between the Assignment operator & the Equality operator.
Solution:
Use the Assignment operator (=)
if you have to put a value inside a variable.
# Assign value 10 to variable x
x = 10
print(x) # Output: 10
# Assign string "Hello" to variable greeting
greeting = "Hello"
print(greeting) # Output: Hello
Use the Equality operator (==)
to check if two values are equal.
# Compare integers
x = 5
y = 10
print(x == y) # Output: False
# Compare strings
greeting1 = "Hello"
greeting2 = "Hello"
print(greeting1 == greeting2) # Output: True
4. Python Error Encountered: TypeError - Combining Integers and Strings.
Problem: TypeError When Adding Integers and Strings in Python.
Solution: Convert Integers to Strings and then Concatenate.
# Define variables: two integers and one string
a = 1
b = 2
c = "Abdul"
print(a + b + c) # TypeError: unsupported operand type(s) for +: 'int' & 'str'
# Correct Method
a = 1
b = 2
c = "abdul"
result = str(a) + str(b) + c # Convert integers to strings before concatenation
print(result) # Output: 12abdul
5. Missing f Prefix: A Common f-String (formatted string) Mistake.
Problem: When using f-strings, forgetting the f
prefix can lead to unexpected output.
Solution: Add the f
prefix before the string.
# Missing 'f' for f-string formatting
name = "John"
age = 30
print("I am {age} years old.") # Output: I am {age} years old.
# Correct use of f-string for formatting
name = "John"
age = 30
print(f"I am {age} years old.") # Output: I am 30 years old.
6. Tuples cannot be changed after creation but the Lists can be modified.
Problem: Trying to modify a tuple after creation, which led to an error.
Solution:
Tuples cannot be changed after creation. Tuples are immutable.
If you need a collection that can be modified, use a List instead.
my_tuple = (1, 2, 3) # Creating a tuple
# Trying to modify a tuple (this will raise an error)
my_tuple[0] = 10
print(my_tuple) #Error: 'tuple' object does not support item assignment
# Correct approach if you need a modifiable collection
my_list = [1, 2, 3]
my_list[0] = 10 # Lists can be modified
print(my_list) # Output: [10, 2, 3]
7. List Sorting Error when inputs are strings instead of integers.
Problem: I wrote a program to sort user inputs, but the sort () function treated the inputs as strings instead of numbers, causing incorrect alphabetical sorting.
marks = [] # Creating Empty List
m1 = input("Enter marks of first student: ")
marks.append(m1) # Add first student's marks to the list
m2 = input("Enter marks of second student: ")
marks.append(m2) # Add second student's marks to the list
m3 = input("Enter marks of third student: ")
marks.append(m3) # Add third student's marks to the list
# Sort the marks in ascending order
marks.sort() # Sorts alphabetically, not numerically due to string input
# Print the sorted marks
print("Marks: ", marks) # Output: Marks: ['36', '65', '9']
Solution: To fix this, I used int ()
while taking input, ensuring each mark was stored as an integer.
marks = []
m1 = int(input("Enter marks of first student: ")) # Using int() function
marks.append(m1) # Add first student's marks to the list
m2 = int(input("Enter marks of second student: "))
marks.append(m2) # Add second student's marks to the list
m3 = int(input("Enter marks of third student: "))
marks.append(m3) # Add third student's marks to the list
# Sort the marks in ascending order
marks.sort() # Sorts numerically
# Print the sorted marks
print("Marks: ", marks) # Marks: [9, 36, 65]
8. Error while trying to add a List to a Set in Python.
Problem: While working with sets, I encountered an error when attempting to add a list as an element.
# Attempting to add a list to a set
s = {8, 7, 12, "Abdul", [1, 2]} # This will raise an error
# TypeError: unhashable type: 'list'
Solution:
Lists are mutable, so they cannot be added to sets. Instead, use a tuple to store sequences in a set, as tuples are immutable and compatible with sets.
# Using a tuple instead of a list
s = {8, 7, 12, "Abdul", (1, 2)}
print(s) # Output: {8, 7, 12, 'Abdul', (1, 2)}
If you need a different tuple, you can remove the existing one and add a new tuple.
# Using a tuple instead of a list
s = {8, 7, 12, "Abdul", (1, 2)}
s.remove((1, 2)) # Remove the original tuple
s.add((3, 4)) # Add a new tuple with modified values
print(s) # Output: {'Abdul', (3, 4), 7, 8, 12}
These are the resources that helped me learn:
Some valuable resources I'd like to share:
Subscribe to my newsletter
Read articles from Sheikh Abdul Wahid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
