Building a Distributed Leader Election System with the Bully Algorithm in Node.js and React
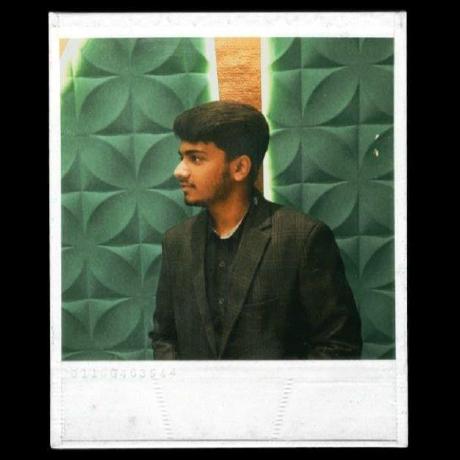
Table of contents
Introduction
In distributed systems, selecting a leader is crucial for coordinating tasks, making decisions, and handling failures gracefully. In this article, we'll walk through building a distributed leader election system using the Bully Algorithm, a classic approach for electing a leader in distributed environments. This project combines Node.js for backend logic and React for real-time visualization, creating an intuitive interface to simulate leader election, detect failures, and demonstrate communication between nodes.
We’ll cover the Bully Algorithm basics, project requirements, the system architecture, key challenges, solutions, and features of our admin panel for controlling nodes.
What is the Bully Algorithm?
The Bully Algorithm is a leader election protocol designed for distributed systems where nodes (or "processes") need to select a single leader. Here’s a high-level overview of how it works:
Trigger Election: When a node detects that the leader has failed, it starts an election.
Message Exchange: The initiating node sends “election” messages to nodes with a higher ID.
Response and Leadership: The highest-ID node responding to the election becomes the leader and announces itself to all nodes.
Fault-Tolerance: If the elected leader fails, another election can be triggered automatically, ensuring a robust and resilient system.
This project allows us to simulate the election process and visualize leader elections in real-time, making it easier to understand and study distributed algorithms in practice.
Project Overview
1. Technology Stack
Frontend: React
Backend: Node.js
Communication: WebSockets (for real-time updates between frontend and backend)
The frontend UI provides a real-time visualization of the network and nodes, including the leader node, active nodes, and inactive ones. The backend manages the nodes' states and implements the Bully Algorithm for leader election.
2. System Requirements and Goals
Automatic Leader Election: If a leader node fails, the system automatically detects the failure and elects a new leader.
Real-Time Visualization: Users can observe the current leader, active nodes, and election process on the frontend.
Admin Panel: A control panel allows users to activate or deactivate nodes, trigger manual elections, and monitor system health.
Scalability: Supports multiple nodes without performance degradation, allowing simulation for larger distributed networks.
Setting Up the Project
Initialize the Backend with Node.js
Useexpress
for handling server requests andws
for WebSocket communication. The server holds the Bully Algorithm logic for election handling and broadcasts updates to connected clients (frontend) via WebSockets.Frontend with React
The frontend is responsible for visualizing the network, nodes, and election status in real-time. We’ll use React to manage the UI and Socket.IO to receive updates from the backend.
Implementing the Bully Algorithm
In a distributed network, the Bully Algorithm is implemented through the following steps:
Node Failure Detection
When a node fails, nodes that recognize the failure initiate an election. Nodes with a higher ID respond to the election message, and the highest-ID node becomes the leader.Election Initiation
The initiating node sends election requests to nodes with higher IDs and waits for their responses. Only nodes with a higher ID can respond.Selecting a New Leader
The highest-ID node that responds is elected as the new leader. This node announces its status to the network, allowing all other nodes to recognize it as the leader.
System Architecture
The system consists of two main components:
Backend
Manages node states and implements the election logic using the Bully Algorithm.
Uses WebSockets to send real-time status updates to the frontend.
Frontend
Displays the current status of nodes, including active/inactive and leader status.
Allows users to simulate failures by activating/deactivating nodes through the admin panel.
Uses WebSockets to receive updates from the backend in real-time.
Building the Core Components
1. Backend Logic: Node.js with the Bully Algorithm
Node.js Server: Handles node states, failure detection, and the election process.
WebSocket Communication: Sends status updates to the frontend, allowing real-time visualization of the election.
2. Frontend Interface: React with Real-Time Visualization
Admin Panel: An interface where users can activate or deactivate nodes and manually start elections.
Visualization of Nodes: Each node is displayed with different icons/colors based on its status (leader, active, inactive).
Election Status: Shows communication between nodes during the election process and updates the leader in real-time.
Real-Time Updates with WebSockets
For real-time feedback on node status and election processes, we use WebSockets for bi-directional communication between the frontend and backend. Whenever a node’s status changes or an election concludes, the backend broadcasts an update to the frontend.
Handling Challenges
Synchronization Issues in Simultaneous Elections
Challenge: Multiple nodes initiating an election at the same time can create conflicts.
Solution: Introduced delays and locking mechanisms to ensure only one election runs at a time, preventing race conditions.
Fault Tolerance During Election Process
Challenge: A node failing during an election could disrupt the entire process.
Solution: Implemented a timeout mechanism to detect unresponsive nodes. If a timeout occurs, a new election is initiated.
Real-Time UI Updates
Challenge: Keeping the frontend in sync with the backend during rapid node status changes and elections.
Solution: WebSocket updates provide immediate feedback on election results and node statuses, ensuring the UI stays current.
Testing the System
Unit Tests
Tested election logic to ensure the Bully Algorithm consistently selects the correct leader.
Verified that nodes correctly detect leader failures and initiate elections.
Integration Testing
Ensured WebSocket communication updates the frontend accurately.
Tested node failure scenarios and verified the election process through the frontend.
User Acceptance Testing
Tested usability of the frontend to ensure it provides meaningful feedback on election processes.
Verified the admin panel's effectiveness in controlling node states and visualizing the distributed network.
Example Scenario: Simulating a Leader Election
To see the Bully Algorithm in action, we can simulate a scenario:
A node detects that the current leader has failed and initiates an election.
The frontend displays the communication between nodes, showing which nodes respond and which are participating.
The node with the highest ID responds, and the UI updates to display the new leader.
Future Improvements
This project demonstrates the basic functionality of the Bully Algorithm in a distributed system. Potential future improvements could include:
Implementing Alternative Election Algorithms: Comparing different leader election algorithms, like the Ring Algorithm, to understand their behavior.
Scaling Up: Expanding the simulation to handle larger networks with more complex node interactions.
Enhanced UI Metrics: Adding additional metrics for each node, such as communication latency and response times, to provide deeper insights into the system’s performance.
Conclusion
The Bully Algorithm provides a straightforward yet effective approach for leader election in distributed systems. Through this project, we explored how the Bully Algorithm can be implemented using Node.js and React, creating a robust and interactive simulation of distributed leader election. Our UI not only allows real-time observation of node statuses and leader transitions but also gives users control over node states, enhancing understanding of distributed algorithms.
This project serves as a hands-on guide to implementing fault tolerance and leader election, equipping developers and learners with essential concepts and tools for building resilient distributed systems. Happy coding, and may the best node win!
Subscribe to my newsletter
Read articles from Dhyan Amit Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
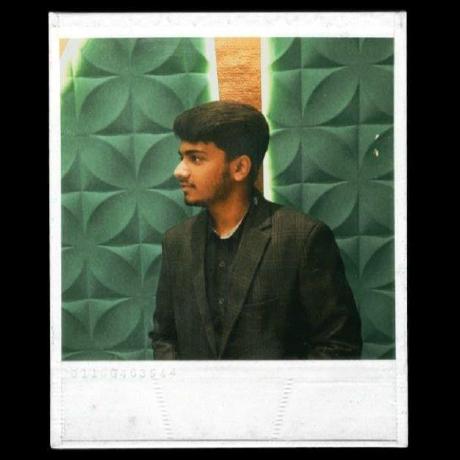
Dhyan Amit Shah
Dhyan Amit Shah
I am a developer and programmer from India, I am a Backend Developer, and a ML enthusiast.