Remote Procedure Call

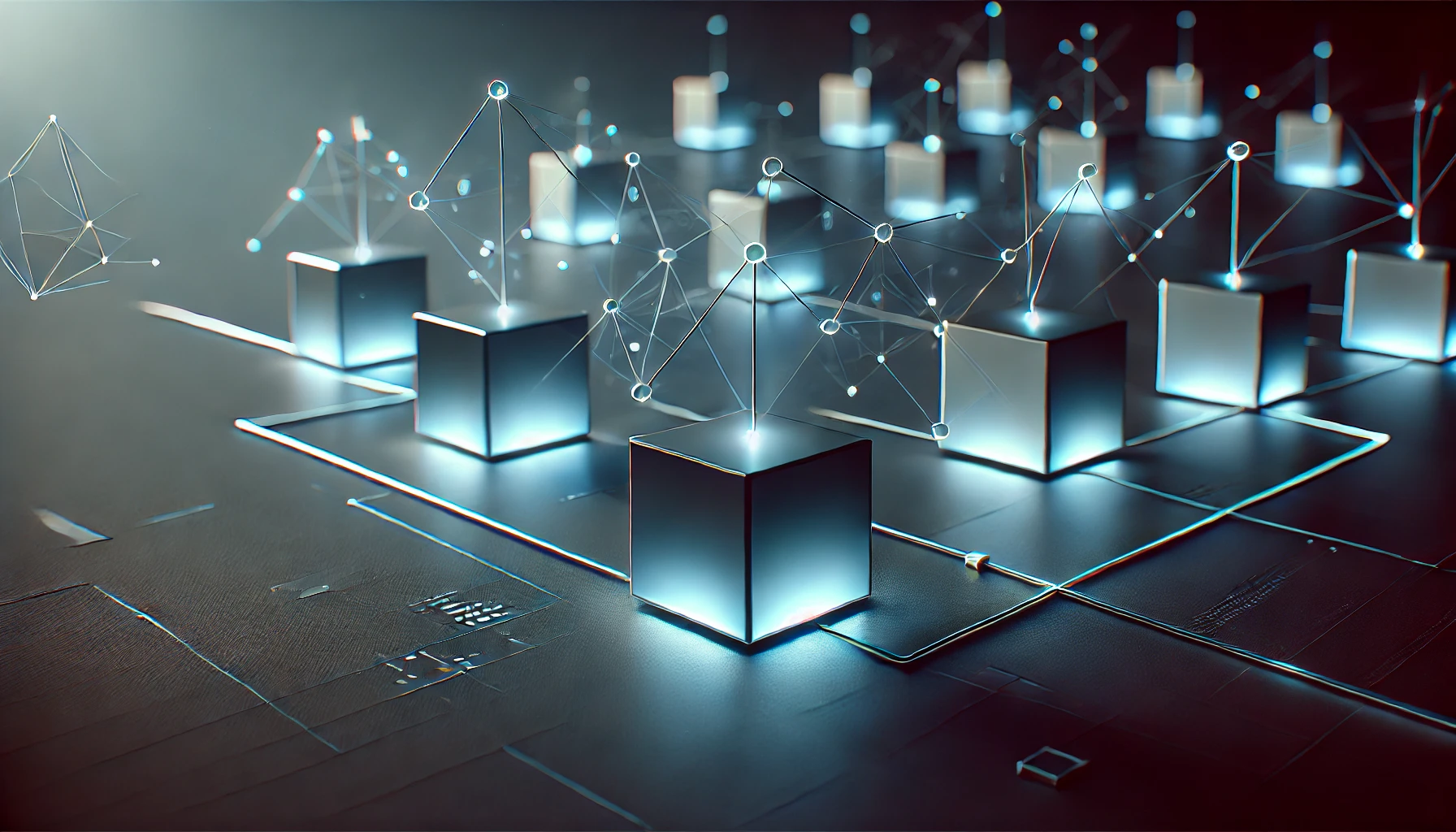
Hello everyone..
Welcome to another day of exploring Web3 Engineering.
We have been talking about blockchain and developing smart contracts on them for a while and also writing scripts on how to interact with the blockchain for a while. But have you ever wondered on how actually your script communicates with the blockchain ?? Of course it uses RPC .. Duh..!! But what is this RPC ? How is it different from other communication protocols ? Where it is used ? So without any further ado, let’s get answers to these questions.
Remote Procedure Call aka RPC protocol
Remote Procedure Call (RPC) protocol is a communication protocol that allows a service (or an entity) to invoke a certain procedure on a remote service as if it is a local procedure.
In common language, it is a protocol that allows you to call a function on a different server as a local function in the code. For example, we are calling eth_getBalance
function in our local javascript file as a local function but it is implemented on the blockchain node provided by our RPC provider.
Structure of RPC method
An rpc request contains 4 parameters. The version of the rpc, method name to be called, params if any, and finally a request identifier id. The structure looks as follows
{
"jsonrpc": "2.0",
"method": "getBlock",
"params": [1234],
"id": 1
}
We can also add any metadata if needed for our procedure.
How is it different from Other
Other communication protocols like REST-API follows resource based design and uses URLs to provide different functionalities. Whereas, the RPC is designed around the procedures rather than resources.
For example, if we want to get all the users using REST - API, the code looks like this
const url = 'https://example.com/users/getUsers';
try {
const response = await fetch(url, {
method: 'GET', // RESTful GET request
headers: {
'Content-Type': 'application/json',
},
});
if (!response.ok) {
throw new Error(`Error: ${response.statusText}`);
}
const data = await response.json();
But if we want to implement the same thing using RPC methods, it would like this
const url = 'https://example.com/rpc'; // The endpoint for RPC requests
const rpcRequest = {
jsonrpc: '2.0',
method: 'getUsers', // The remote procedure call method
params: [], // Parameters required for the method (empty in this case)
id: 1, // Request ID
};
try {
const response = await fetch(url, {
method: 'POST', // POST request for RPC
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(rpcRequest), // Sending the request body
});
const data = await response.json();
Where it is used
RPC is mainly used for communication between micro services and distributed systems. It is also used in systems where a real time communication is needed such as IoT, blockchain and smart contracts, online multiplayer games etc.
What are the popular implementations of RPC:
Due to its flexible architecture and compatibility with different formats, RPC is implemented to support various data formats. Such as
gRPC - The gRPC is developed by Google where RPC is implemented to transport binary data for communication between entities which is mainly used in cloud-native applications
JSON-RPC : The JSON-RPC format transport data using JSON format widely used in blockchain communication
XML-RPC: The XML data formatted RPC calls are used in older system where XML format is used data exchange.
SOAP: While more of a web service protocol, SOAP can be considered an RPC mechanism, as it invokes remote operations with an XML-based request and response.
Along with the data format used, we can also use RPC with different transfer protocols such as https, web sockets etc
That’s all for today.
Feel free to comment down your questions and don’t forget to subscribe to the newsletter.
Subscribe to my newsletter
Read articles from Jay Nalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jay Nalam
Jay Nalam
Hi, I'm Jay Nalam, a seasoned Web3 Engineer committed to advancing decentralized technologies. Specializing in EVM-based blockchains, smart contracts, and web3 protocols, I've developed NFTs, DeFi protocols, and more, pushing boundaries in the crypto realm.