Random Forest
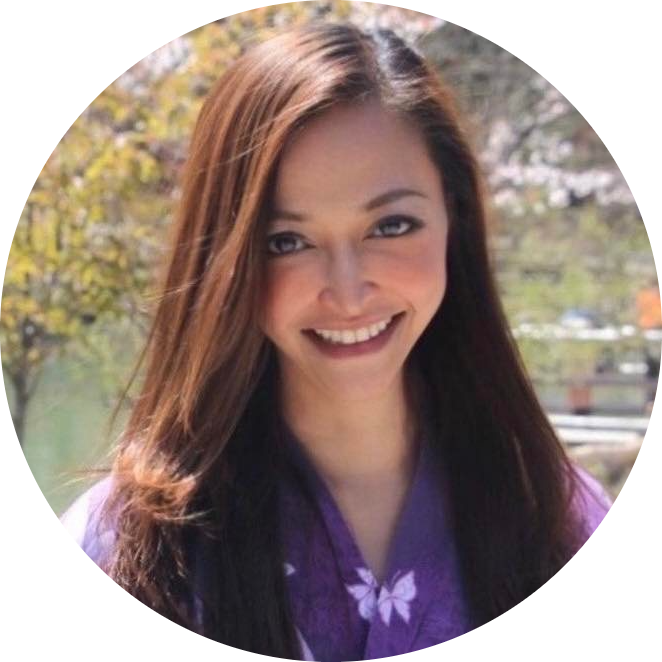
Suppose we have a dataset about students, with features like study hours ( \( x_1 \) ), attendance rate ( \( x_2 \) ), and previous grades ( \( x_3 \) ), and we want to predict whether they will pass an exam ( \( y = 1 \) for "pass" and \( y = 0 \) for "fail").
Study Hours \( x_1 \) | Attendance Rate \( x_2 \) | Previous Grades \( x_3 \) | Pass/Fail \( y \) |
2 | 60 | 70 | 0 |
4 | 85 | 80 | 1 |
3 | 70 | 60 | 1 |
1 | 50 | 55 | 0 |
5 | 90 | 85 | 1 |
We will use this example dataset to walk through each step of how Random Forest works.
Step 1: Decision Trees in Random Forest
A Random Forest is an ensemble of multiple Decision Trees. Each decision tree makes predictions (pass or fail) based on different subsets of the data and features.
How Decision Trees Split Data: Each tree splits the data into branches by identifying conditions on features like "Study Hours > 2.5." This creates smaller, more homogeneous groups where one outcome is more common (e.g., passing or failing).
Impurity: A tree tries to reduce impurity with each split. Impurity measures how mixed the outcomes are in a group. For example, if a node contains 3 "pass" and 1 "fail," it has a Gini Impurity calculated as: \( \text{Gini} = 1 - \left(\frac{3}{4}\right)^2 - \left(\frac{1}{4}\right)^2 = 0.375 \) The lower the impurity, the clearer the separation between "pass" and "fail."
Each tree in a Random Forest will learn different patterns in the data because it trains on slightly different samples.
Step 2: Bagging (Bootstrap Aggregation)
In Bagging, each decision tree in the Random Forest is trained on a different bootstrap sample of the data. For example:
If we have five students in the dataset, each tree might randomly select a different subset with replacement:
Tree 1: Students 1, 2, 3, 2, 4
Tree 2: Students 2, 4, 3, 5, 5
These random samples (with replacement) help create variation across trees, reducing the chance of overfitting to any particular pattern. Even if one student’s data is heavily represented in one tree, another tree might give more focus to different students.
Step 3: Feature Randomness
Random Forests add an extra layer of randomness by selecting a random subset of features (like study hours, attendance, or grades) at each split within each tree.
For example, a tree might consider only "Study Hours" and "Attendance Rate" for the first split, ignoring "Previous Grades." This randomness reduces the dominance of any single feature across all trees, making the model more robust.
Why Feature Randomness Matters: If "Study Hours" were the only dominant feature, every tree might heavily rely on it. By randomizing the features, Random Forest ensures that each tree looks at various combinations of features, which can lead to a more balanced model.
Step 4: Making Predictions
After training, each tree in the forest makes an independent prediction about whether a student will pass or fail. For instance:
Tree 1 might predict "fail" for a student with 2 study hours and a 60% attendance rate.
Tree 2 might predict "pass" for the same student.
To make a final prediction, Random Forest takes a majority vote across all trees. If most trees predict "pass," the Random Forest predicts "pass." Mathematically, if there are \( T \) trees, each giving a prediction \( \hat{y}^{(t)} \) , the overall prediction \( \hat{y} \) is: \( \hat{y} = \text{mode}(\\{\hat{y}^{(t)}\\}_{t=1}^T) \)
In this way, if 6 out of 10 trees predict "pass," the final decision is "pass."
Step 5: Feature Importance
Feature importance in a Random Forest shows how much each feature contributes to predictions. For instance, in our student dataset:
The model might determine that "Study Hours" has the highest feature importance, indicating it's the most relevant for predicting exam success.
"Attendance Rate" could have a moderate importance, while "Previous Grades" might be less influential.
This importance is calculated based on how much each feature reduces impurity across all trees: \( \text{Importance}(x_j) = \frac{1}{T} \sum_{t=1}^T \sum_{\text{split on } x_j} \Delta \text{Impurity} \)
For example, if "Study Hours" consistently creates splits that reduce impurity more than other features, it will have the highest importance score.
Python Code Example
Here’s an example code to apply Random Forest using Python and analyze feature importance.
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
import pandas as pd
# Example dataset
data = pd.DataFrame({
'Study Hours': [2, 4, 3, 1, 5],
'Attendance Rate': [60, 85, 70, 50, 90],
'Previous Grades': [70, 80, 60, 55, 85],
'Pass': [0, 1, 1, 0, 1]
})
X = data[['Study Hours', 'Attendance Rate', 'Previous Grades']]
y = data['Pass']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Initialize and train the Random Forest
rf_model = RandomForestClassifier(n_estimators=10, random_state=42)
rf_model.fit(X_train, y_train)
# Make predictions
y_pred = rf_model.predict(X_test)
# Feature importance
importances = rf_model.feature_importances_
feature_importances = pd.DataFrame({'Feature': X.columns, 'Importance': importances})
feature_importances = feature_importances.sort_values(by='Importance', ascending=False)
print(feature_importances)
Summary
Random Forest combines predictions from multiple decision trees, each trained on a different sample of the data.
Trees vote on each prediction, and Random Forest aggregates these votes to make a final decision.
Adding randomness in data sampling and feature selection improves robustness and prevents overfitting.
Feature importance highlights which features (e.g., Study Hours, Attendance Rate) are most influential in predictions.
This version integrates the student example into each step to connect the theory with practical application. Let me know if further clarification is needed!
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
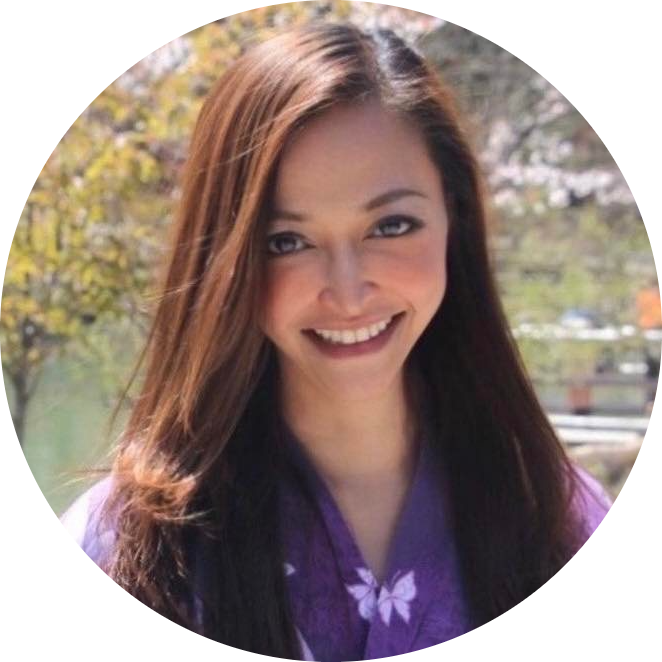