Chapter 44: Dynamic Programming (Part 4) - Mastering Complex String Problems in DSA
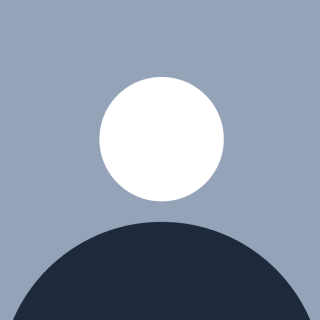
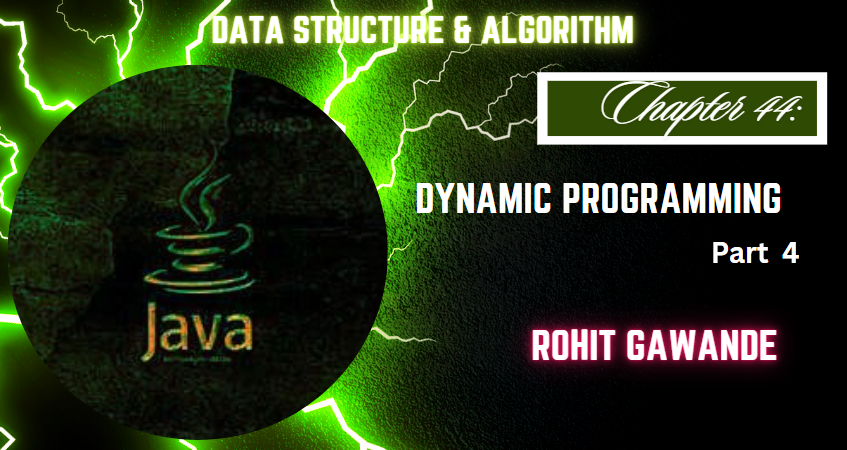
Introduction
In this chapter, I delved into advanced dynamic programming concepts, specifically focusing on string-based problems that are essential for solving complex data structure and algorithm (DSA) challenges. This part of the journey, guided by Apna College and Shradha Khapra’s Alpha Plus Course, has been a deep dive into efficiently solving some of the most challenging string-based dynamic programming problems.
Here's what I learned:
1. Longest Common Substring
The Longest Common Substring problem involves finding the longest substring that two given strings share. Unlike the Longest Common Subsequence problem, which allows characters to be non-contiguous, this problem requires characters to appear consecutively.
Key Concepts
Dynamic Programming Table Setup: Using a 2D DP table where each cell tracks the length of the longest common substring ending at that character.
State Transition: We increase the count if two characters from both strings match; otherwise, we reset it to zero.
Why It's Important
This problem demonstrates how dynamic programming can help with substring comparison, which is especially useful for text matching and pattern recognition applications.
2. Longest Increasing Substring
In the Longest Increasing Substring problem, the objective is to find a substring within a sequence where each successive element is greater than the preceding one.
Approach
We track the length of increasing substrings as we iterate through the string.
The longest length recorded gives us the desired substring length.
Applications
This type of problem is helpful in scenarios where pattern recognition of consecutive sequences is required, such as identifying trends or streaks in data.
3. Edit Distance (Explanation)
The Edit Distance problem is all about transforming one string into another by performing a minimum number of operations. These operations typically include insertions, deletions, and substitutions.
Dynamic Programming Approach
Define a DP Table: The rows represent characters of one string, and the columns represent characters of the other string.
State Transition: For each character pair, choose the minimum number of operations required to make the two strings identical up to that point.
Real-World Use Case
Edit distance is widely used in applications like spell checkers, DNA sequence alignment, and natural language processing.
4. Edit Distance (Code)
After understanding the theory, I implemented the code for the Edit Distance problem to see the dynamic programming approach in action. Here’s a simplified version of the code:
public class EditDistance {
public int minDistance(String word1, String word2) {
int m = word1.length(), n = word2.length();
int[][] dp = new int[m + 1][n + 1];
// Initialize dp table
for (int i = 0; i <= m; i++) dp[i][0] = i;
for (int j = 0; j <= n; j++) dp[0][j] = j;
// Fill dp table
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (word1.charAt(i - 1) == word2.charAt(j - 1))
dp[i][j] = dp[i - 1][j - 1];
else
dp[i][j] = 1 + Math.min(dp[i - 1][j - 1], // Replace
Math.min(dp[i - 1][j], // Remove
dp[i][j - 1])); // Insert
}
}
return dp[m][n];
}
}
This solution fills out the DP table and returns the minimum operations required to convert one string into another.
5. String Conversion
Building on the Edit Distance problem, String Conversion explores how to modify a source string into a target string with the minimum set of insertions, deletions, or replacements.
Steps to Solve:
Use the edit distance calculation to identify the number of edits.
Track each operation (insertion, deletion, or replacement) to understand how the source string transforms into the target.
Applications
String conversion methods are crucial in applications like version control, spell-check engines, and DNA sequence analysis, where efficiently transforming one sequence to another is key.
Related Posts
Related Series
Connect With Me
Feel free to connect and follow me on:
Stay tuned for more updates on my blog series!
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊