Day 17: Mastering JavaScript Destructuring - Arrays and Objects
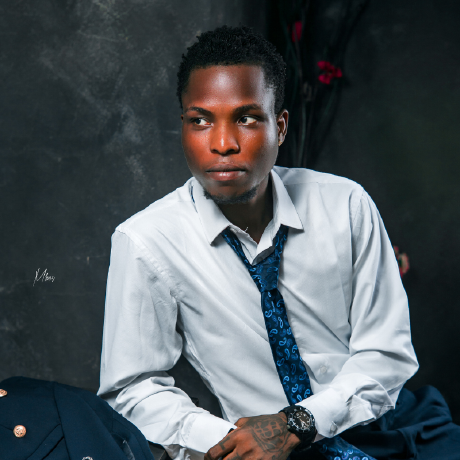
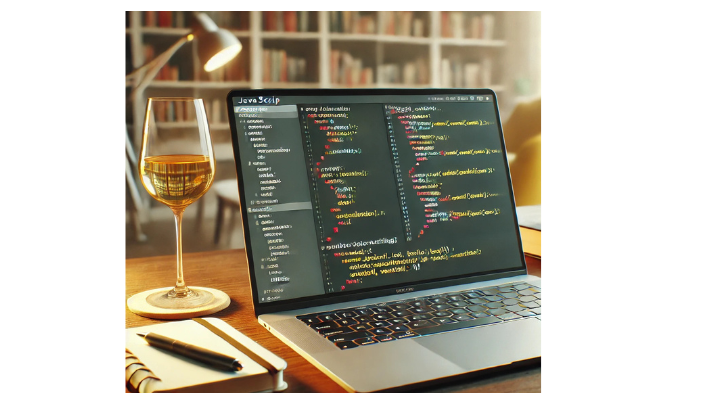
Today’s blog post is brought to you with a glass of white wine in hand – finally! After missing out yesterday due to a last-minute trip to ShopRite, I'm happy to be sipping as I write. 🍷
On Day 17, we’re diving into one of my favorite ES6 features: destructuring in JavaScript! Destructuring makes working with arrays and objects a breeze by allowing us to unpack values and properties into clear, easy-to-read variables. This feature isn’t just about cleaner code; it’s also about making our work more efficient and enjoyable.
So, let’s explore how array and object destructuring can level up our JavaScript skills, all while keeping things refreshingly simple.
1️⃣ Array Destructuring
With array destructuring, we can assign values to multiple variables in one line! This is especially helpful when working with arrays where order matters.
Here's a quick example:
const fruits = ["apple", "banana", "cherry"];
const [first, second, third] = fruits;
console.log(first); // Output: apple
console.log(second); // Output: banana
console.log(third); // Output: cherry
You can even skip items in the array by leaving the corresponding positions empty:
const colors = ["red", "blue", "green", "yellow"];
const [primary, , secondary] = colors;
console.log(primary); // Output: red
console.log(secondary); // Output: green
2️⃣ Object Destructuring
Object destructuring lets us extract specific properties from objects, which is super helpful when working with large objects and only needing a few values. Unlike arrays, with objects, we use the property names.
For instance:
const user = {
name: "Alice",
age: 25,
location: "New York"
};
const { name, location } = user;
console.log(name); // Output: Alice
console.log(location); // Output: New York
We can also rename variables during destructuring, which is especially useful if a property name conflicts with a variable name in our scope:
const user = { name: "Bob", age: 30 };
const { name: userName } = user;
console.log(userName); // Output: Bob
3️⃣ Nested Destructuring
For more complex data, destructuring allows us to reach into nested arrays and objects. Here’s an example:
const person = {
name: "Carol",
details: {
age: 32,
address: {
city: "San Francisco",
zip: 94107
}
}
};
const {
name,
details: {
address: { city }
}
} = person;
console.log(name); // Output: Carol
console.log(city); // Output: San Francisco
4️⃣ Destructuring with Default Values
We can set default values during destructuring, which is great for handling cases where some data might be missing:
const settings = { theme: "dark" };
const { theme, fontSize = "16px" } = settings;
console.log(theme); // Output: dark
console.log(fontSize); // Output: 16px (default value)
5️⃣ Using the Rest Operator with Destructuring
Combining destructuring with the rest operator (...
) allows us to capture any remaining items in an array or properties in an object:
const numbers = [1, 2, 3, 4, 5];
const [first, ...rest] = numbers;
console.log(first); // Output: 1
console.log(rest); // Output: [2, 3, 4, 5]
For objects:
const user = { id: 1, name: "Daisy", role: "Admin" };
const { id, ...otherInfo } = user;
console.log(id); // Output: 1
console.log(otherInfo); // Output: { name: "Daisy", role: "Admin" }
Why Destructuring Matters
Destructuring can save us time and lines of code, especially as our data structures become more complex. By reducing the need for repetitive code, destructuring brings clarity and efficiency to our JavaScript codebase.
If you want to learn more, check out MDN’s official documentation on destructuring, or explore examples on JavaScript.info.
As I explore JavaScript, these features continue to highlight how powerful and adaptable this language is for modern development. If you’re on this journey too, try destructuring and see how it transforms your code! Feel free to share your experiments or challenges on LINKEDIN, and don’t forget to tag me – I’d love to see your progress.
Stay tuned for Day 18 tomorrow as we dive deeper into JavaScript and its most useful features! For more insights, you can always follow along with my JavaScript journey here on Stanley’s JavaScript Blog.
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
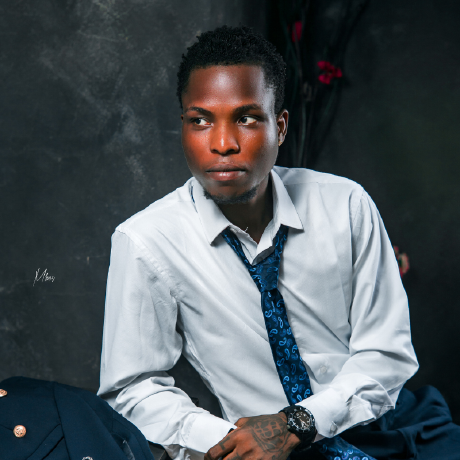
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com