Python Basics: Lists, Tuples, Dictionaries
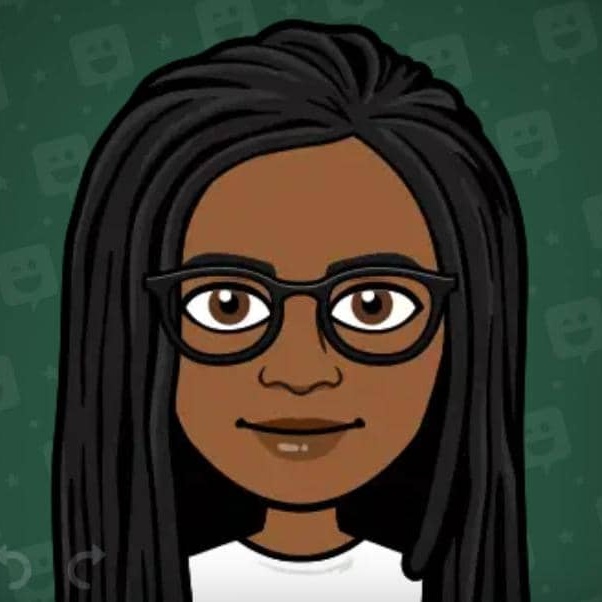
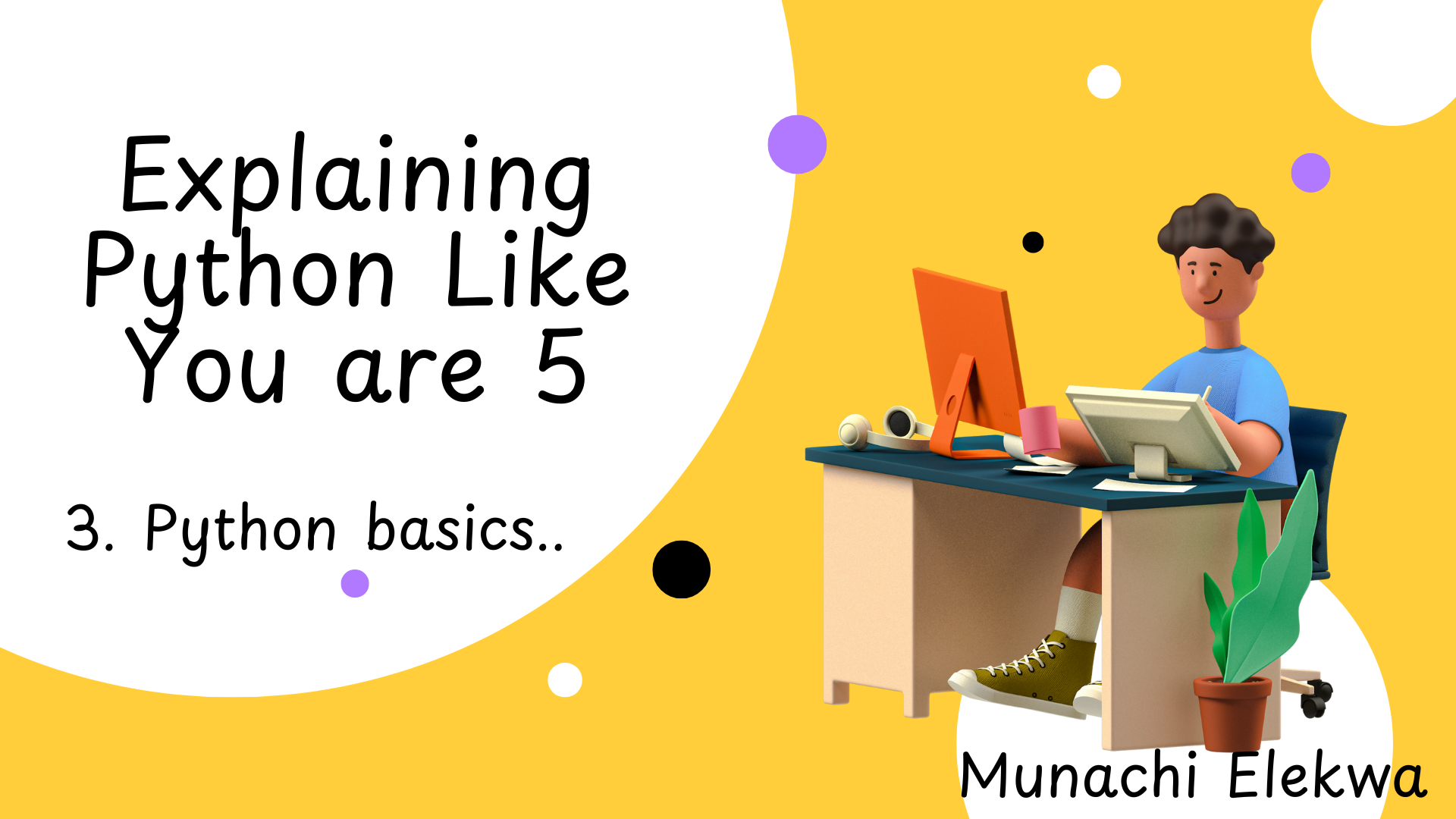
Welcome Back to Explaining Python: Lists, Tuples, and Dictionaries
Welcome back! If you’ve been following along, kudos for sticking with it! If you're just joining, feel free to start from the first lesson to catch up.
In this lesson, we’ll dive into some super useful data structures in Python: lists, tuples, and dictionaries. Each has its strengths, and by the end, you'll have a clear picture of when and why to use them.
What Are Lists, and Why Use Them?
A list in Python is an ordered collection that lets you store multiple items (like numbers, strings, or even other lists) in a single variable. Lists are super handy because you can change them, add to them, or remove items as needed. They’re a go-to for organizing data that you might want to modify later.
Creating a List
To create a list, use square brackets []
and separate items with commas:
fruits = ["apple", "banana", "cherry"]
Here, fruits
is a list containing three string items. You can also mix different data types in a list, like this:
my_list = [5, "hello", True] #first item is an integer, second is a string and the third is a boolean value.
Accessing List Items
You can access items by their index (the position of an item in the list). Remember, Python starts counting from 0!
print(fruits[0]) # Output: apple
print(fruits[2]) # Output: cherry
Modifying Lists: Adding, Removing, and Changing Items
Python provides several methods to make lists dynamic and flexible. Here’s a rundown:
Adding Items
append(item)
: Adds an item to the end of the list.insert(index, item)
: Inserts an item at a specific position.
fruits.append("orange")
print(fruits) # Output: ["apple", "banana", "cherry", "orange"]
fruits.insert(1, "grape")
print(fruits) # Output: ["apple", "grape", "banana", "cherry", "orange"]
Removing Items
remove(item)
: Removes the first occurrence of the item.pop(index)
: Removes the item at a specific index. If no index is specified, it removes the last item.
fruits.remove("banana")
print(fruits) # Output: ["apple", "grape", "cherry", "orange"]
fruits.pop(2)
print(fruits) # Output: ["apple", "grape", "orange"]
Changing Items
- You can change an item in a list by referring to its index.
fruits[0] = "blueberry"
print(fruits) # Output: ["blueberry", "grape", "orange"]
Other Useful List Methods
sort()
: Sorts the list in ascending order.reverse()
: Reverses the order of the list.len(list)
: Returns the number of items in the list.
numbers = [3, 1, 4, 1, 5, 9]
numbers.sort()
print(numbers) # Output: [1, 1, 3, 4, 5, 9]
numbers.reverse()
print(numbers) # Output: [9, 5, 4, 3, 1, 1]
print(len(numbers)) # Output: 6
Tuples: Immutable Lists
A tuple is like a list, but it’s immutable, meaning you can’t change, add, or remove items after you create it. Tuples are perfect when you need a collection of items that won’t change throughout your program (like coordinates or dates).
Creating a Tuple
Tuples use parentheses ()
instead of square brackets []
:
coordinates = (4, 5)
Why Use Tuples?
Tuples are faster than lists and protect data that shouldn’t be modified, which makes them great for fixed collections of items.
Accessing Tuple Items
Just like lists, you can access tuple items by their index:
print(coordinates[0]) # Output: 4
The Key Difference Between Lists and Tuples
Lists are mutable (you can change them).
Tuples are immutable (you can’t change them).
Example Use Case:
Use a list when you want a collection that you may need to modify (like a shopping list).
Use a tuple when you have a collection that should stay the same (like fixed GPS coordinates).
Dictionaries: Key-Value Pairs
A dictionary in Python is a collection of key-value pairs. Think of it as a real dictionary where each word (key) has a definition (value). This is perfect for when you want to store related information but need a fast way to look up specific details.
Creating a Dictionary
Use curly braces {}
to define a dictionary. Each item is a pair: key: value
.
person = {
"name": "Chidi",
"age": 25,
"city": "Abuja"
}
Accessing Dictionary Values
To get the value for a specific key, use square brackets []
:
print(person["name"]) # Output: Chidi
Adding, Changing, and Removing Items
Adding or Updating:
- You can add a new key-value pair or update an existing one by simply assigning it:
person["email"] = "chidi@example.com" # Adds a new key-value pair
person["age"] = 26 # Updates the age
print(person)
Removing:
del person["city"]
: Deletes the item with the specified key.person.pop("email")
: Removes the key and returns its value.
del person["city"]
print(person) # Output: {"name": "Chidi", "age": 26}
Useful Dictionary Methods
keys()
: Returns a list of all the keys.values()
: Returns a list of all the values.items()
: Returns a list of all key-value pairs as tuples.
print(person.keys()) # Output: dict_keys(['name', 'age'])
print(person.values()) # Output: dict_values(['Chidi', 26])
print(person.items()) # Output: dict_items([('name', 'Chidi'), ('age', 26)])
When to Use Dictionaries
Use dictionaries when you want to store data that can be associated with unique keys. For example:
Storing user details (name, email, age) where each piece of information has a clear label.
Building a contact book where names are keys, and phone numbers are values.
Quick Recap
Lists: Ordered, mutable collections; use when data may need updating.
Tuples: Ordered, immutable collections; ideal for fixed sets of values.
Dictionaries: Unordered collections of key-value pairs; perfect for labeled data that’s easy to look up.
Mini Challenge: Practice with Lists, Tuples, and Dictionaries!
Try creating each data structure and experimenting with the methods above. Here are some ideas to get you started:
List Challenge:
- Create a list of your top three favorite foods and add a new one at the end. Then, guess the output before running it!
fav_foods = ["rice", "beans", "yam"]
fav_foods.append("pasta")
print(fav_foods)
Question: What do you think the output will be? (Hint: The new item should appear at the end.)
Tuple Challenge:
- Create a tuple with three numbers that represent your birthdate (day, month, year).
birthdate = (15, 6, 1990)
print(birthdate)
Question: Can you access the month by using its index?
Dictionary Challenge:
- Build a small contact book with names and phone numbers. Then, add a new contact and print the dictionary.
contacts = {
"Sarah": "07037798546",
"James": "08151025649"
}
contacts["Charlie"] = "09018976543"
print(contacts)
Question: What do you expect the output to look like after adding Charlie?
Let’s keep coding and building together! And don’t forget to come back for the next lesson in the series.
Subscribe to my newsletter
Read articles from Munachi Elekwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
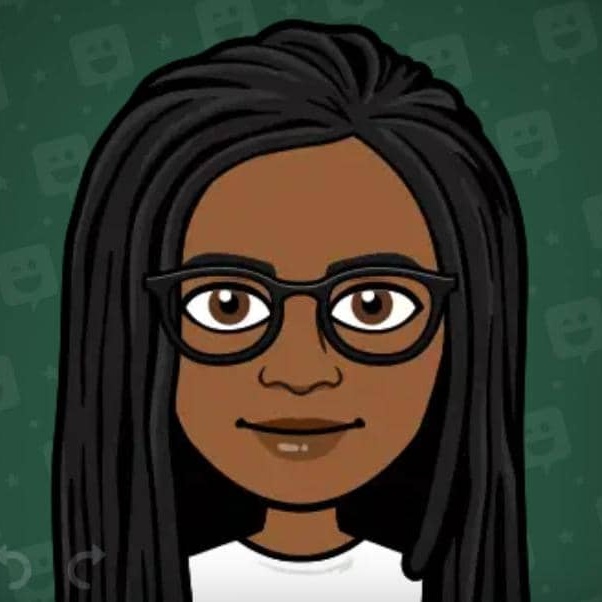
Munachi Elekwa
Munachi Elekwa
I'm Software developer with a sweet tooth.