Day 2 of 30 days of JavaScript Challenge
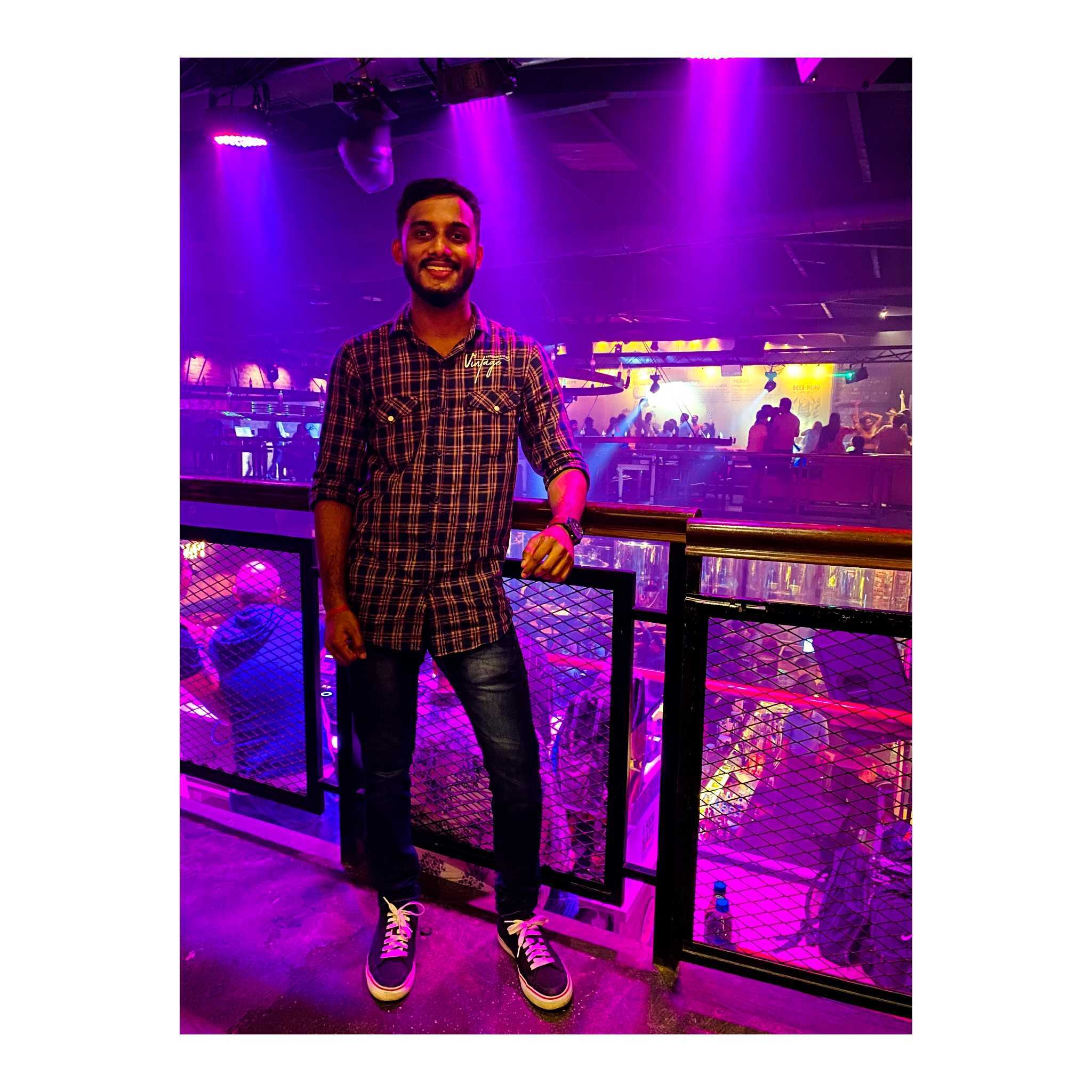
Table of contents
Hello guys welcome back to new series of JavaScript, where we‘ll be solving 30 days of JavaScript Questions from LeetCode.
Here is link of all the problems
In this series, we'll tackle each question one by one. I'll guide you by providing solutions and discussing the approach we should take to solve each problem, so let's dive in.
Prerequisite
For this challenge, you only need a basic understanding of JavaScript, nothing more!
Question
In this challenge, we need to create a “ counter “
which keeps track of count
Approach
To solve this problem, we will require knowledge one of the important JavaScript concept called “ closure
“
Closure is function which is bind or bundled together with it’s hierarchical parent.
Also closure is a function that has access to the variables and parameters of its outer function, even after the outer function has returned or finished executing.
Solution
To solve this problem LeetCode provide basic snippet, which is as below
/**
* @param {number} n
* @return {Function} counter
*/
var createCounter = function(n) {
return function() {
};
};
To solve this, we'll use the JavaScript increment operator “ ++
“ which will increment value of particular variable by 1 every time its been called.
In this createCounter
function return a inner function which will return incremented count of n i.e. “ n++
“ .
The final code snippet will look like below
/**
* @param {number} n
* @return {Function} counter
*/
var createCounter = function(n) {
return function() {
return n++;
};
};
That’s it, it was that simple to create a counter in JavaScript.
Conclusion
I hope you find this article helpful and that it adds some value to your coding career.
Be sure to check out my other articles to gain more knowledge.
In you have any suggestions, ideas or doubts please reach out to me on my social handles.
Until next time, Have a nice coding! 🤙 🤘
Subscribe to my newsletter
Read articles from Vasant Mestry directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
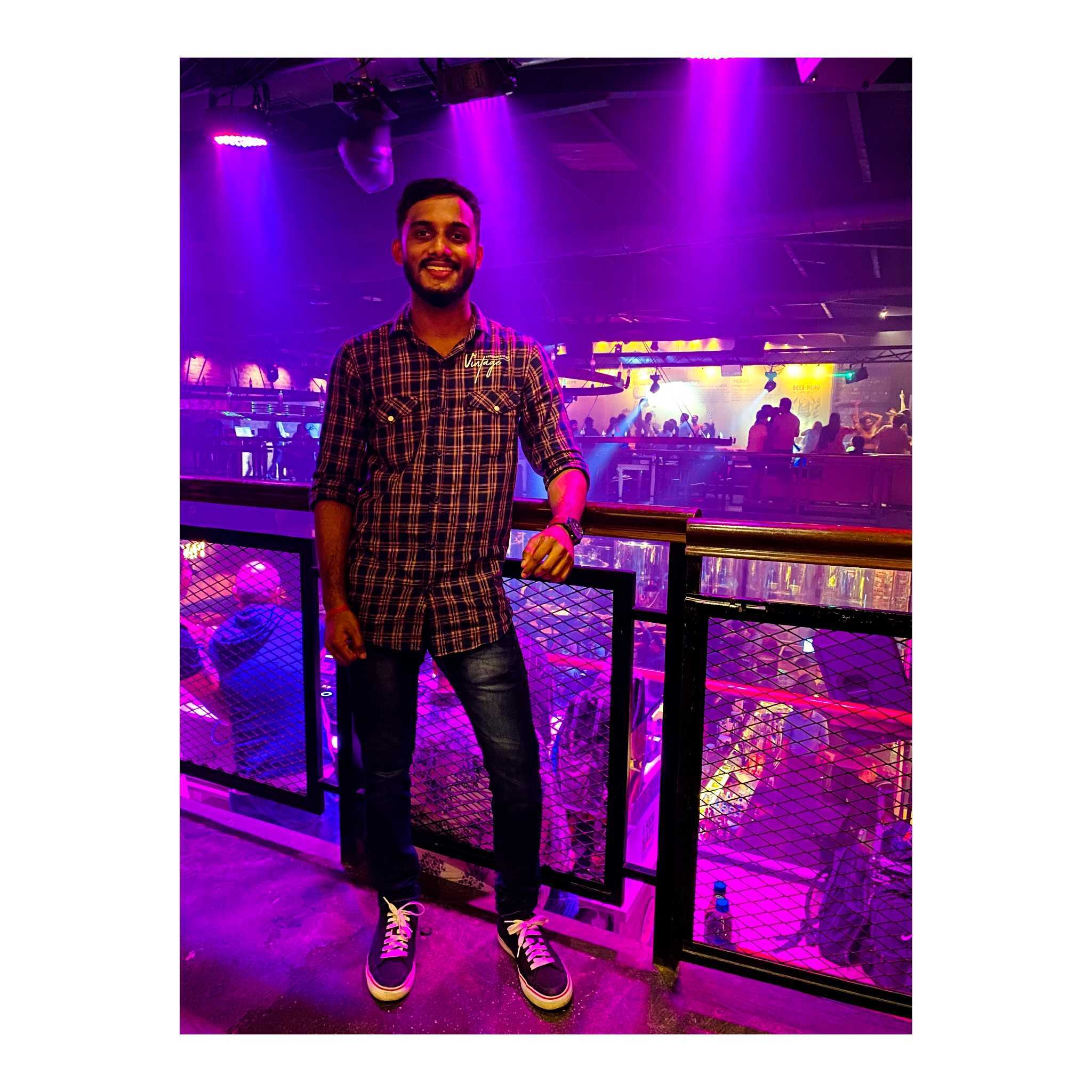