createRoot method

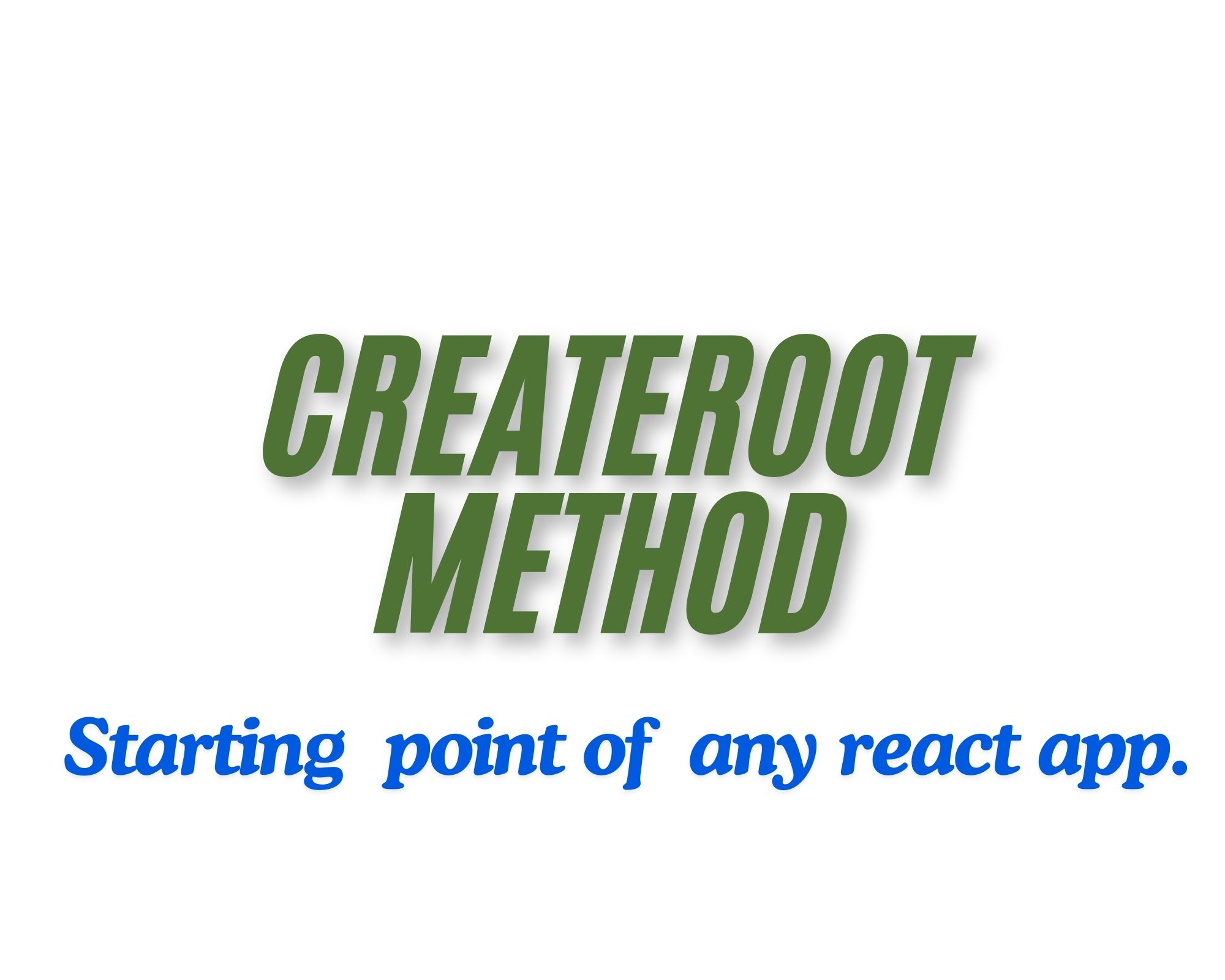
The createRoot
function in React is part of the new React 18 API, replacing the older ReactDOM.render
method. This function helps mount your React application to the HTML DOM more efficiently and provides better support for concurrent rendering features.
Basic explanation:
1.createRoot
Setup: createRoot
is used to create a "root" for your React application. This root is the starting point for rendering React components into the browser.
2.Connecting to the DOM: When we use createRoot
, we specify which part of the HTML should contain our React application. Typically, we select an HTML element with an ID like root
.
3.Rendering Components: After setting up the root, we use the .render()
method to display (render) our main React component (usually <App />
) in that root. This is where the React component tree starts, and all child components will be rendered within this root.
Example Code
Here's how to use createRoot
in a simple example:
Step 1: Setup HTML
In your HTML file (like index.html
), ensure you have an element with an ID root
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>React App</title>
</head>
<body>
<div id="root"></div>
<script src="index.js"></script>
</body>
</html>
Step 2: JavaScript (React Code)
In your main JavaScript file (like index.js
), use createRoot
to render the <App />
component:
import React from 'react';
import { createRoot } from 'react-dom/client';
import App from './App';
createRoot(document.getElementById('root'))
.render(
<App/>
)
Step 1: document.getElementById('root')
What It Does: This part is a standard JavaScript function that searches the HTML document for an element with the ID
'root'
.Purpose: In a React app, the element with ID
'root'
is usually an empty<div>
where we want to render our entire React app.
Step 2: createRoot(...)
What It Does:
createRoot
is a function from React’sreact-dom/client
package.Purpose: It creates a “React Root” on the selected HTML element (here,
document.getElementById('root')
).Why It’s Important: This
createRoot
method sets up a special React container for managing the rendering process. With React 18, this container allows for advanced features like automatic batching and concurrent rendering, which make the app more efficient.createRoot(document.getElementById('root'));
This creates a React root in the
<div id="root"></div>
section of the HTML.
Step 3: .render(<App />)
What It Does:
.render(<App />)
takes the React component<App />
(usually your main or root component) and renders it inside the React root created in Step 2.Purpose: This is where your React application actually appears on the screen. React goes through
<App />
, finds all the child components, and renders them within the#root
HTML element.How It Looks in Context:
createRoot(document.getElementById('root')).render(<App />);
Here, <App />
is the main component. This renders it, along with all its child components, inside the HTML element with the ID root
.
This is starting point of any react app.
Thanks.
Subscribe to my newsletter
Read articles from Patel Nayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Patel Nayan
Patel Nayan
student