Project_1.1 : Building a University Website from Scratch: Step-by-Step Guide for Beginners
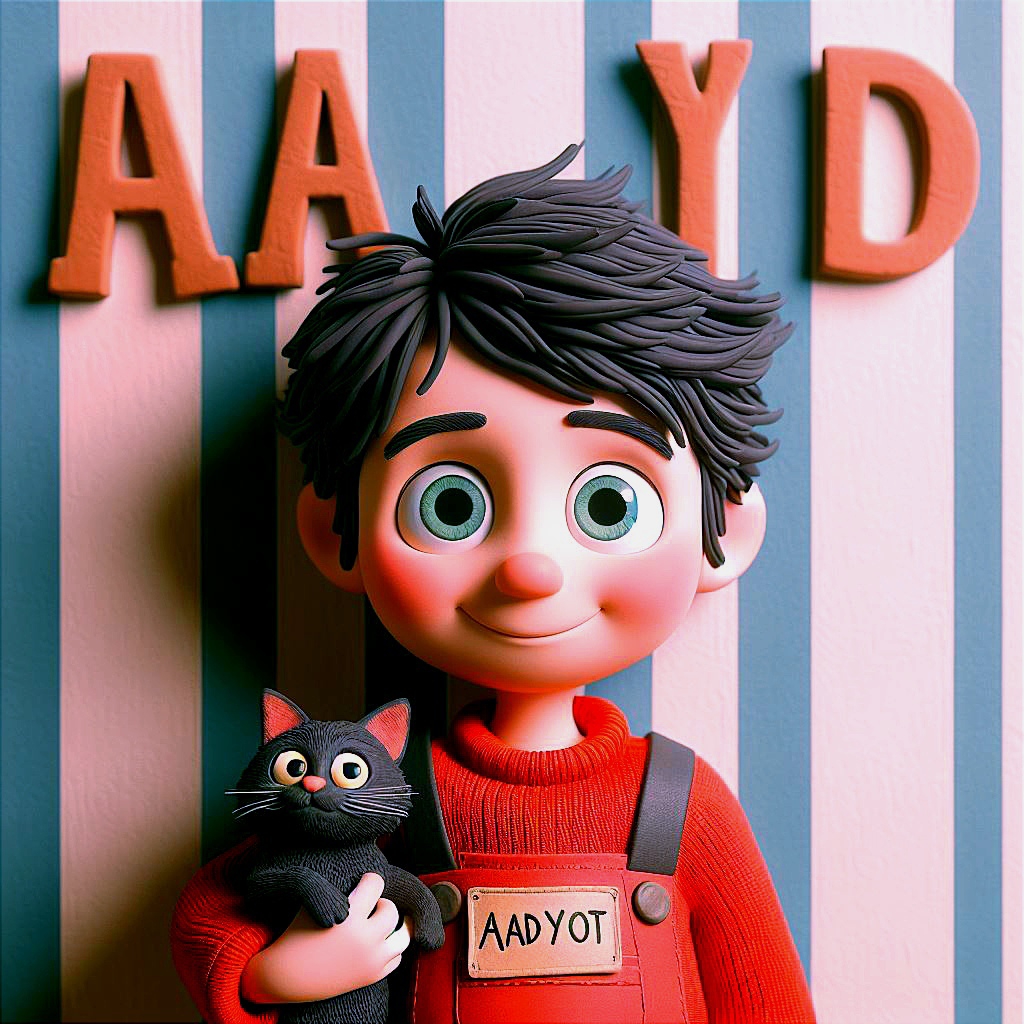
To build this university website project, here’s a list of the essential HTML and CSS concepts you'll need to understand. I've organized them by section to help you follow along step-by-step as you learn.
1. HTML Basics
Start with these foundational HTML concepts to build the structure of the website.
HTML Document Structure: Understanding the skeleton of an HTML file:
htmlCopy code<!DOCTYPE html> <html lang="en"> <head>...</head> <body>...</body> </html>
<html>
tag: The root element for an HTML document.<head>
tag: Contains metadata, such as<title>
, CSS links, and other settings.<body>
tag: Holds all the content displayed on the webpage.
HTML Tags for Structure:
<nav>
: Defines a navigation section for links.<ul>
and<li>
: Unordered lists are used here to create a list of navigation links.<a>
: Anchor tags are used to create clickable links.<img>
: Used for inserting images like logos and banners.
Attributes in HTML Tags:
href
attribute: Used in<a>
tags to link to other pages or sections.src
attribute: Used in<img>
tags to specify the image source.alt
attribute: Adds alternative text for images (important for accessibility).
2. CSS Basics
To style the website, you'll need these fundamental CSS concepts:
Selectors and Properties:
Basic selectors: Target HTML elements directly, like
body
,h1
, ornav
.Class selectors (
.
): Use.
to target specific classes, e.g.,.header
.ID selectors (
#
): Use#
for unique identifiers, like#nav-links
.
CSS Box Model:
Margin: Space outside an element's border.
Padding: Space between an element's content and its border.
Border: Outlines the element.
Width and Height: Controls the element’s size.
Positioning:
Static Positioning (default): Elements follow the natural flow of the page.
Relative Positioning: Offsets the element relative to its original position.
Absolute Positioning: Positions the element relative to its nearest positioned ancestor.
Fixed Positioning: Positions the element relative to the viewport, useful for sticky headers.
Flexbox:
display: flex;: Use this to create flexible and responsive layouts.
justify-content: Aligns items horizontally (e.g.,
justify-content: space-between;
).align-items: Aligns items vertically (e.g.,
align-items: center;
).
Backgrounds:
Background colors: Set solid colors with
background-color
.Background images: Add images with
background-image
, control their size and position withbackground-size
andbackground-position
.Linear gradients: Combine colors with gradients, such as
linear-gradient(...)
.
Typography:
Font styles: Change font size, color, and family.
Google Fonts: Import fonts from Google Fonts, like:
htmlCopy code<link rel="preconnect" href="https://fonts.googleapis.com"> <link href="https://fonts.googleapis.com/css2?family=YourFontName&display=swap" rel="stylesheet">
3. Responsive Design with Media Queries
To make your website responsive (adaptable to different screen sizes), you need to understand media queries.
@media Rule: Sets specific styles for different screen widths.
cssCopy code@media (max-width: 700px) { /* CSS for small screens */ }
Font size adjustments: Make text smaller for mobile.
Hide/show elements: Use display properties (
none
,block
) to control visibility on different screen sizes.Change layout: Adjust
flex-direction
, margins, and paddings to optimize layout for mobile.
4. CSS Animations and Transitions
Animations and transitions add interactivity to buttons and other elements:
CSS Transitions:
transition
property: Smoothens changes over time, like button color changes on hover.Example:
cssCopy code.hero_btn { transition: background 0.5s, border-color 0.5s; }
CSS Animations (Optional, for more advanced effects):
Use
@keyframes
for custom animations.Example for a fade-in effect:
cssCopy code@keyframes fadeIn { 0% { opacity: 0; } 100% { opacity: 1; } }
5. Basic JavaScript for Interactivity
To add functionality to the website, you'll need a few JavaScript concepts.
JavaScript in HTML: Link your JavaScript file with
<script src="script.js" defer></script>
to keep HTML and JavaScript separate.DOM Manipulation:
Selecting elements: Use
document.getElementById()
orquerySelector()
to target elements.Event Listeners: Make elements respond to user actions, like clicks.
javascriptCopy codedocument.querySelector(".fa-bars").onclick = show_menu;
Functions:
Defining functions: To perform specific actions (e.g.,
show_menu()
andhide_menu()
functions to open and close the navigation menu).Changing styles dynamically: Use JavaScript to set CSS properties, such as changing the
right
position of a menu.
Hi everyone! 👋 Welcome to my journey as I create a university website from scratch. As a beginner, I want to explain each step in detail so you can follow along and learn the basics of HTML, CSS, and JavaScript with me.
In this project, we’ll create a modern, responsive website with a navigation bar, a hero section, and interactive elements. Today, we'll focus on setting up the structure, designing the header, and adding a hero section.
1. Setting Up the Project Structure
Let's start by creating a basic folder structure for our website.
Create a Project Folder: Inside your main project folder, add these subfolders:
images
: This is where we’ll keep images like the university logo and banner.css
: For our styling files.js
: For JavaScript code.
Create Files: Inside the project folder, create these essential files:
index.html
: The main HTML file where our content goes.style.css
: The CSS file for styling.script.js
: JavaScript file to add interactivity.
Connect Files in HTML: Now, link these files to
index.html
:<link rel="stylesheet" href="style.css"> <script src="script.js" defer></script>
The defer
attribute in the script tag ensures the JavaScript loads after the HTML, preventing issues with the script running before elements are loaded.
2. Creating the Navigation Bar
Our website needs a navigation bar to guide users to different sections. Here’s the HTML for a basic nav bar with a toggle menu:
<nav>
<a href="index.html"><img src="images/logo.png" alt="University Logo"></a>
<div class="nav-links" id="nav-links">
<i class="fa fa-times" onclick="hide_menu()"></i>
<ul>
<li><a href="#">HOME</a></li>
<li><a href="#">ABOUT</a></li>
<li><a href="#">COURSES</a></li>
<li><a href="#">BLOG</a></li>
<li><a href="#">CONTACT</a></li>
</ul>
</div>
<i class="fa fa-bars" onclick="show_menu()"></i>
</nav>
Explanation:
The
img
tag withsrc="images/logo.png"
is where your logo goes.The
nav-links
div holds the links and toggles with icons for open/close.
We’ll add styles and functionality next.
3. Styling the Header
Now, let’s style the header and navigation bar in style.css
to look more professional.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.header {
min-height: 100vh;
background-image: linear-gradient(rgba(4, 9, 30, 0.7), rgba(4, 9, 30, 0.7)), url(images/banner.png);
background-size: cover;
background-position: center;
}
nav {
display: flex;
padding: 1% 3%;
justify-content: space-between;
align-items: center;
}
nav img {
width: 150px;
}
.nav-links ul li {
display: inline-block;
padding: 8px 12px;
}
.nav-links ul li a {
color: #fff;
text-decoration: none;
font-size: 13px;
}
.text_box {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
text-align: center;
color: #fff;
}
.text_box h1 {
font-size: 62px;
}
.text_box p {
font-size: 14px;
color: #fff;
}
.hero_btn {
border: 1px solid #fff;
color: #fff;
padding: 12px 34px;
text-decoration: none;
transition: background 0.5s, border-color 0.5s;
}
.hero_btn:hover {
background: #f44336;
border-color: #f44336;
}
4. Adding JavaScript for Toggle Menu
We’ll add JavaScript to toggle the menu visibility for smaller screens. Here’s how it works:
Show the menu when the user clicks the "bars" icon.
Hide the menu when the user clicks the "times" icon.
Here’s the code in script.js
:
document.addEventListener("DOMContentLoaded", function() {
const navLinks = document.getElementById("nav-links");
const menuOpen = document.querySelector(".fa-bars");
const menuClose = document.querySelector(".fa-times");
menuOpen.addEventListener("click", () => navLinks.style.right = "0");
menuClose.addEventListener("click", () => navLinks.style.right = "-200px");
});
Final Thoughts
In this post, we created the basic structure, navigation bar, and styled our header. In the next post, we’ll dive deeper into building out other sections of the website. Stay tuned for more as we bring this university website to life!
Subscribe to my newsletter
Read articles from Ranjan Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
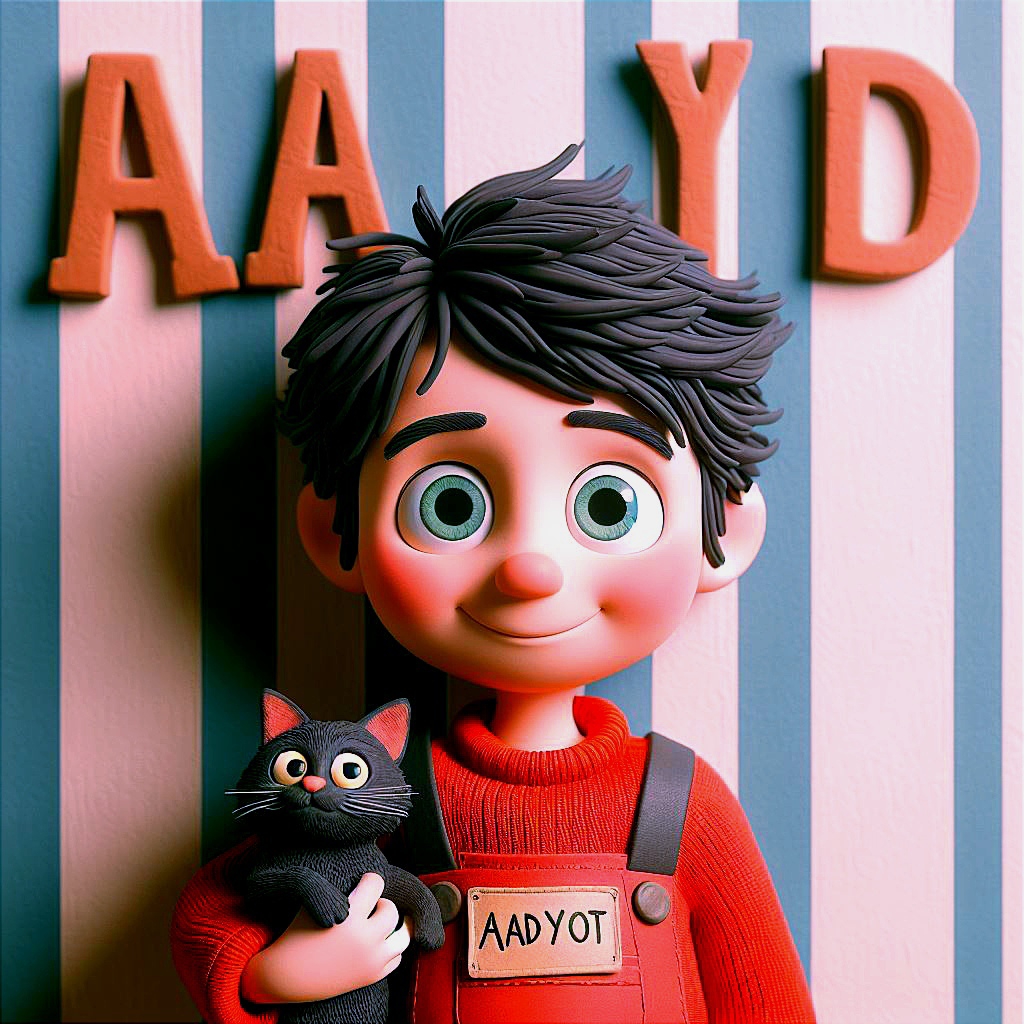