Memory Management in JavaScript: Understanding Scope!
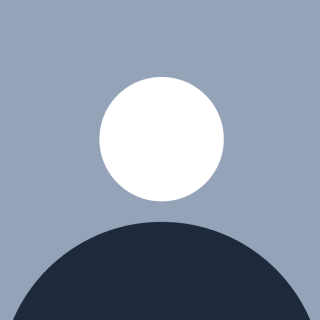
Table of contents
- What is Scope?
- Global vs. Local Scope
- Closures — Unlocking Inner Treasures
- Flowchart: Scope and Closures
- Navigating the Treasure of Scope
- Building the Secret Number Game — A Practical Challenge
- Scope Pitfalls — Avoiding Common Mistakes
- Pitfall 1: Global Variables Overwritten by Local Variables
- Pitfall 2: Closures and Loops
- Mastering Scope and Closures
- Challenge Time: Create Your Own Treasure Hunt
- Navigating Scope and Closures
- Get ready for Asynchronous Programming!
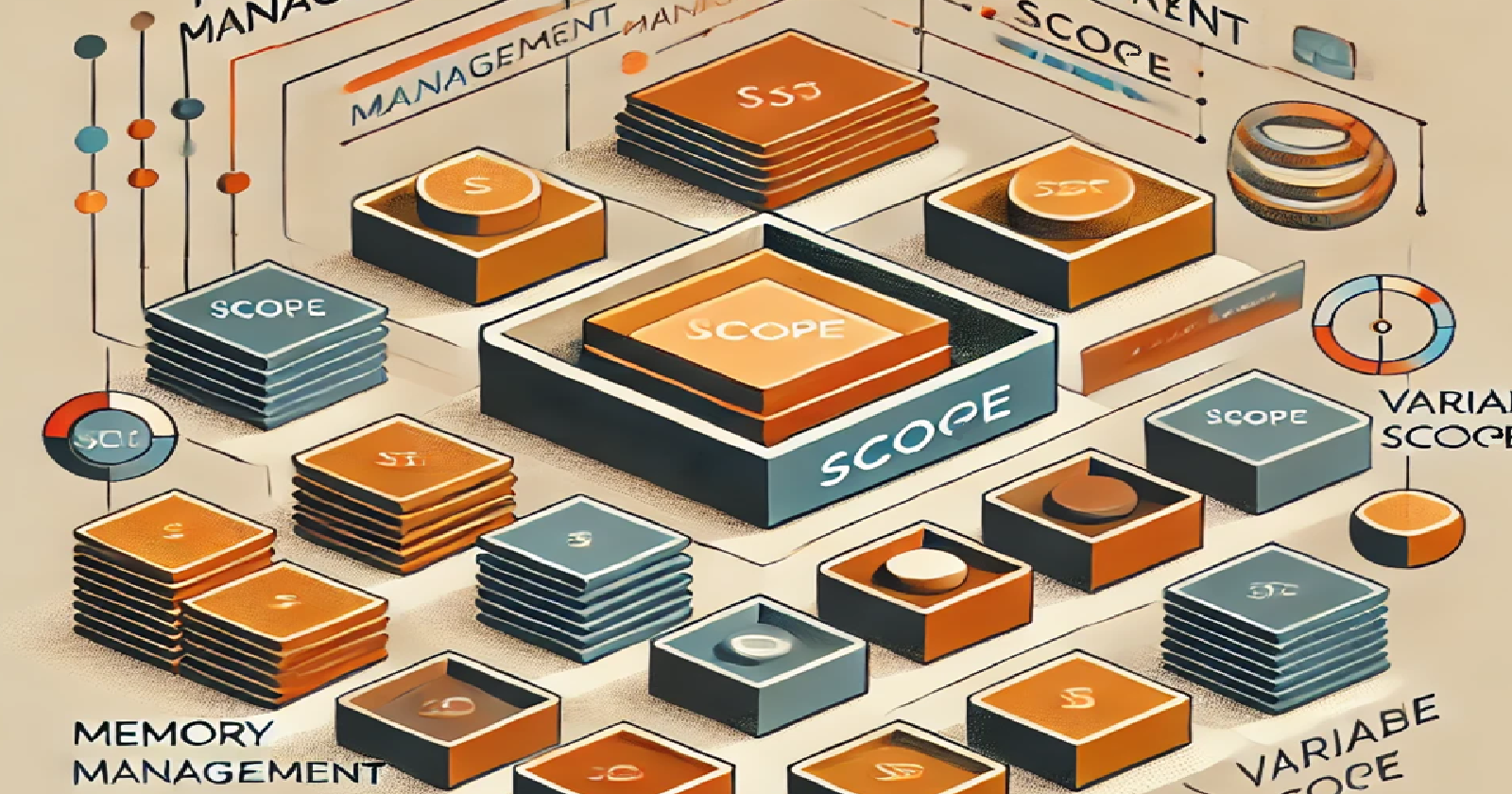
Imagine you’re searching for hidden treasures, and each treasure is locked inside a box. Some boxes are accessible to everyone, while others are hidden deep inside locked rooms. In JavaScript, scope is like these treasure boxes—determining where variables can be accessed or hidden.
Understanding scope is essential for effective memory management in JavaScript. By knowing where variables live and how they’re accessed, you can write more efficient, organized, and bug-free code. In this article, we’ll dive into the different types of scope, how closures work, and how to avoid common pitfalls when working with variables.
Let’s begin our treasure hunt!
What is Scope?
In JavaScript, scope refers to the visibility of variables, determining where in your code a variable can be accessed. There are two main types of scope:
Global Scope: Variables declared in the global scope are accessible from anywhere in the program.
Local Scope: Variables declared within a function or block are only accessible within that specific area, like a treasure locked inside a box.
Think of the global scope as the main treasure room where everyone can access the items inside, while local scope represents locked treasure boxes that can only be accessed from inside a specific room.
Global vs. Local Scope
In JavaScript, variables can be declared with var
, let
, or const
, and these declarations influence their scope. Variables declared outside of any function are part of the global scope, while variables declared inside a function or block are part of the local scope.
Code Snippet: Example of Global vs. Local Scope
Let’s explore how global and local scope work in practice with an example:
let treasure = "gold coins"; // Global variable
function openTreasureBox() {
let hiddenTreasure = "diamonds"; // Local variable
console.log("Inside the box: " + hiddenTreasure);
}
openTreasureBox(); // Output: Inside the box: diamonds
console.log(treasure); // Output: gold coins
console.log(hiddenTreasure); // Error: hiddenTreasure is not defined
Explanation:
The variable
treasure
is global and can be accessed anywhere in the program.The variable
hiddenTreasure
is local to theopenTreasureBox()
function and cannot be accessed outside the function. It’s hidden in the treasure box!
In this way, local scope keeps variables secure and prevents them from interfering with other parts of the program, much like how hidden treasures stay locked in their boxes.
Closures — Unlocking Inner Treasures
A closure is a special feature in JavaScript where an inner function has access to the variables of its outer function, even after the outer function has returned. Closures are like secret keys to treasure boxes that can be passed around, giving the inner function access to variables that were declared in the outer scope.
Closures allow you to create powerful, memory-efficient code by keeping variables alive in the background.
Code Snippet: Creating Closures
Let’s create a closure where an inner function keeps track of a hidden treasure:
function treasureChest() {
let hiddenTreasure = "ancient relic"; // Local variable
return function revealTreasure() {
console.log("Revealed: " + hiddenTreasure);
};
}
let openChest = treasureChest();
openChest(); // Output: Revealed: ancient relic
Explanation:
The
revealTreasure
function is defined insidetreasureChest
, and it closes over the variablehiddenTreasure
.Even though
treasureChest
has finished executing, the inner functionrevealTreasure
still has access to the variablehiddenTreasure
because of the closure.
Closures allow you to store secret information inside your code, ensuring it can only be accessed in specific circumstances.
Flowchart: Scope and Closures
Let’s visualize how scope and closures work in JavaScript:
[ Global Scope ] [ Local Scope ]
| |
treasure hiddenTreasure (accessible only in function)
| |
[ Function Call ] [ Inner Function Closure ]
| |
Can access Can access hiddenTreasure (even after outer function returns)
This flowchart shows how global and local variables are managed, and how closures allow functions to access variables from their outer scope even after the outer function has finished executing.
Navigating the Treasure of Scope
In this part, you’ve learned about global and local scope, and how closures provide a powerful way to manage and access variables in JavaScript. Understanding scope is like learning how to unlock different treasure boxes in your code, allowing you to manage memory efficiently and keep your variables secure.
In the next part, we’ll dive deeper into practical challenges, including creating a secret number game and exploring more complex closures.
Building the Secret Number Game — A Practical Challenge
Now that you understand how scope and closures work, it’s time to put your knowledge to the test with a fun challenge! We’ll create a Secret Number Game using closures to keep track of a hidden number. This game will allow users to guess the number, and they will only get access to the hidden treasure (the number) if they guess correctly.
Challenge: Create a Secret Number Game
In this game, the secret number is stored inside a closure, and the user can guess the number by calling a function. Each guess will be compared to the hidden number, and if the guess is correct, the treasure will be revealed.
Here’s how we can build the Secret Number Game:
function secretNumberGame() {
let secretNumber = Math.floor(Math.random() * 10) + 1; // Generate random number between 1 and 10
return function(guess) {
if (guess === secretNumber) {
return "Congratulations! You've found the treasure: " + secretNumber;
} else {
return "Try again!";
}
};
}
let playGame = secretNumberGame();
console.log(playGame(3)); // Example output: "Try again!"
console.log(playGame(7)); // Example output: "Congratulations! You've found the treasure: 7"
Explanation:
The function
secretNumberGame
creates a random secret number and returns a function that accepts the user’s guess.The inner function has access to the
secretNumber
variable, even though it’s outside the local scope of the function where it was created. This is the power of closures!The user can keep guessing, but the secret number is only revealed if the guess is correct.
This challenge demonstrates how closures can be used to securely store hidden values and only reveal them under certain conditions.
Scope Pitfalls — Avoiding Common Mistakes
While scope and closures are powerful tools in JavaScript, there are a few common pitfalls that developers should be aware of. Understanding these can help you avoid bugs and improve the efficiency of your code.
Pitfall 1: Global Variables Overwritten by Local Variables
If you accidentally declare a variable without let
, const
, or var
, it will automatically become a global variable. This can lead to issues where global variables are unintentionally overwritten.
Example:
function updateTreasure() {
treasure = "silver coins"; // No declaration keyword, becomes global
}
let treasure = "gold coins";
updateTreasure();
console.log(treasure); // Output: "silver coins" (global variable overwritten)
Solution:
- Always declare variables using
let
,const
, orvar
to avoid accidentally creating global variables.
Pitfall 2: Closures and Loops
When using closures inside loops, developers sometimes run into issues with scope, especially when the closure captures a variable that is shared across iterations.
Example:
function treasureHunt() {
for (var i = 1; i <= 3; i++) {
setTimeout(function() {
console.log("Treasure found at level: " + i);
}, 1000);
}
}
treasureHunt(); // Output: "Treasure found at level: 4" (three times)
The problem here is that the variable i
is captured by the closure, but since var
is function-scoped, it only has one value by the time the loop finishes: i = 4
.
Solution:
Use let
, which is block-scoped, to capture the correct value in each iteration:
function treasureHunt() {
for (let i = 1; i <= 3; i++) {
setTimeout(function() {
console.log("Treasure found at level: " + i);
}, 1000);
}
}
treasureHunt(); // Output: "Treasure found at level: 1", "Treasure found at level: 2", "Treasure found at level: 3"
Using let
ensures that each iteration of the loop has its own unique value of i
.
Mastering Scope and Closures
Mastering scope and closures is key to writing efficient, bug-free JavaScript. By understanding where variables live and how closures keep certain variables alive, you gain more control over how memory is managed in your code.
Key Takeaways:
Global Scope: Variables declared outside of any function or block can be accessed anywhere in the program, but use them sparingly to avoid conflicts.
Local Scope: Variables declared inside functions or blocks are only accessible within that specific context, making your code more secure and modular.
Closures: A closure allows an inner function to access variables from an outer function, even after the outer function has finished executing. This is powerful for creating persistent state in your code, like in the Secret Number Game.
Avoiding Pitfalls: Watch out for common scope-related mistakes like unintentional global variables and scope issues in loops. Always declare your variables properly and be mindful of how closures behave.
Challenge Time: Create Your Own Treasure Hunt
Now it’s time for a final challenge! In this treasure hunt, you’ll create a game where players search for hidden treasures. Use closures to store the locations of the treasures, and allow players to guess where the treasure is hidden. If they find it, reveal the treasure!
Challenge Instructions:
Create a closure that stores the location of a hidden treasure (use random numbers or predefined values).
Allow the player to guess the treasure location by calling a function.
If they guess correctly, reveal the treasure. If not, encourage them to keep guessing!
Here’s a simple starting point for your code:
function treasureHunt() {
let treasureLocation = Math.floor(Math.random() * 5) + 1; // Random location between 1 and 5
return function(guess) {
if (guess === treasureLocation) {
return "Congratulations! You found the treasure at location: " + treasureLocation;
} else {
return "No treasure here. Keep searching!";
}
};
}
let playHunt = treasureHunt();
console.log(playHunt(3)); // Example output: "No treasure here. Keep searching!"
console.log(playHunt(1)); // Example output: "Congratulations! You found the treasure at location: 1"
Navigating Scope and Closures
In this article, we’ve unlocked the mysteries of scope and closures in JavaScript, learning how variables are stored, accessed, and managed throughout a program. Scope is like a treasure box that keeps variables secure, and closures provide the keys to access those treasures from inside inner functions.
By mastering scope and closures, you’ll be able to write more secure, memory-efficient, and modular code. Whether you’re building games, managing data, or working on larger applications, understanding how JavaScript handles scope will be invaluable.
Get ready for Asynchronous Programming!
In the next article, we’ll dive into asynchronous programming—how JavaScript handles tasks that take time, such as fetching data from a server or reading a file. You’ll learn how to use callbacks, promises, and async/await to manage asynchronous tasks efficiently!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by