Day 18: Unlocking the Power of JavaScript’s Spread and Rest Operators
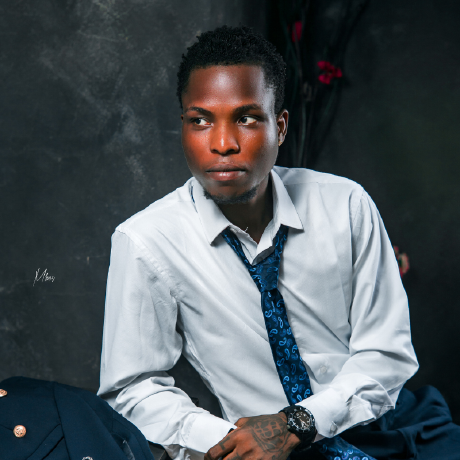
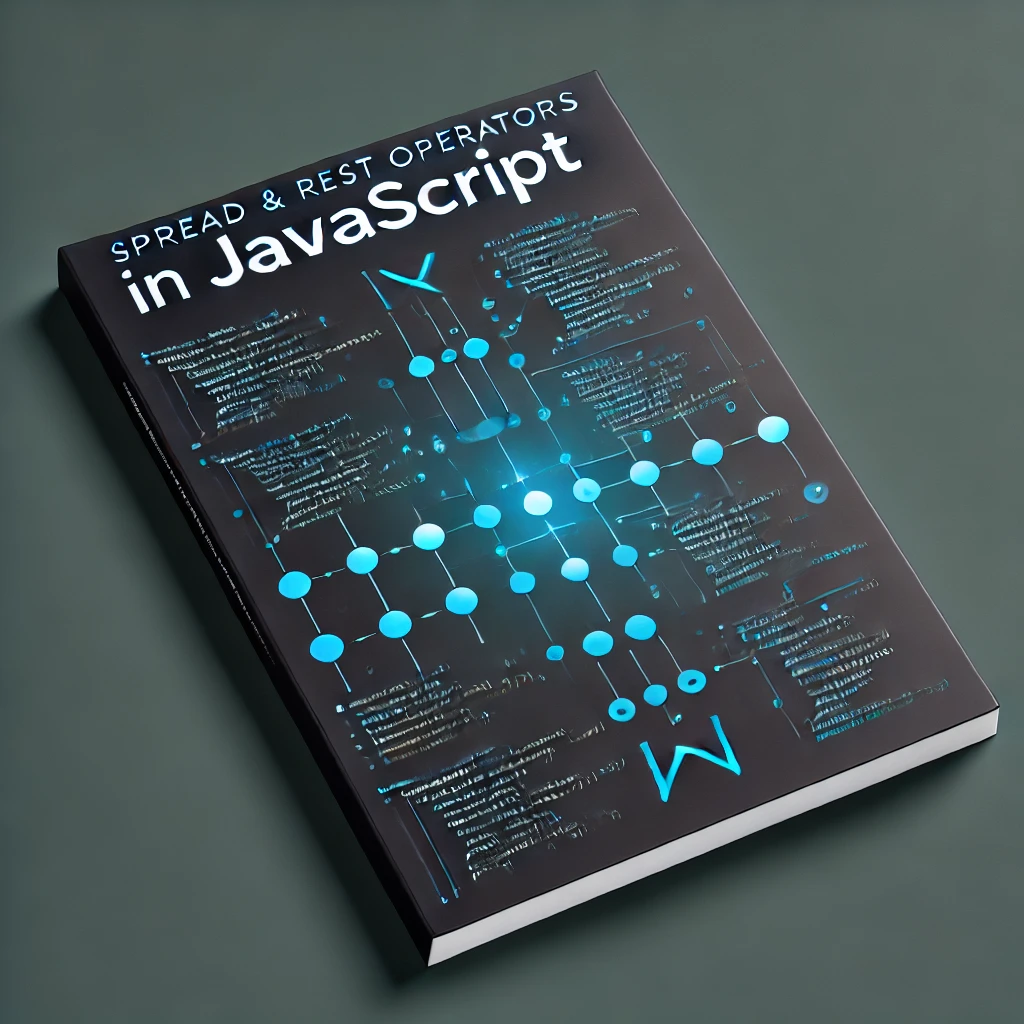
JavaScript is packed with versatile tools that make coding cleaner, more efficient, and more enjoyable. Today, we’re diving into two deceptively simple operators that can transform how you work with data structures: the spread (...
) and rest (...
) operators. They’re two of JavaScript’s most powerful features for handling arrays, objects, and functions.
Let’s explore how they work when to use them, and practical examples that highlight the elegance of these operators.
Understanding the Basics
Though they look identical, the spread and rest operators serve very different purposes:
Spread Operator: Expands elements of an iterable (like an array) into individual elements.
Rest Operator: Gathers multiple elements or arguments into a single array.
Despite this difference, the syntax for both is the same. The distinction lies in where and how we use the ...
syntax in our code.
The Spread Operator: Expanding Values
The spread operator is used when you want to spread or unpack values from an array, object, or string.
1. Combining Arrays
The spread operator makes combining arrays seamless. Gone are the days of using methods like .concat()
.
const fruits = ['apple', 'banana'];
const veggies = ['carrot', 'spinach'];
const food = [...fruits, ...veggies];
console.log(food); // ["apple", "banana", "carrot", "spinach"]
In this example, the spread operator merges
fruits
andveggies
into a newfood
array. Each element fromfruits
andveggies
is unpacked and placed infood
, maintaining the original order.
2. Copying Arrays
Copying an array with the spread operator is not only simple but also ensures you’re working with a new reference, which is important for avoiding unintended mutations in JavaScript.
const original = [1, 2, 3];
const copy = [...original];
copy.push(4);
console.log(original); // [1, 2, 3]
console.log(copy); // [1, 2, 3, 4]
With the spread operator,
copy
is an independent clone oforiginal
. Adding an item tocopy
doesn’t affectoriginal
.
3. Expanding Arguments in Functions
The spread operator also works with function arguments. Imagine you have an array, but you want each element to be a separate argument in a function call.
const numbers = [10, 20, 30];
console.log(Math.max(...numbers)); // 30
Instead of passing each item individually, the spread operator expands the
numbers
array, allowing us to find the maximum value in one line.
4. Spreading Objects
Introduced in ES2018, spreading also works with objects, making it easy to create new objects or merge them.
const person = { name: 'Alice', age: 25 };
const job = { title: 'Developer', company: 'Tech Corp' };
const employee = { ...person, ...job };
console.log(employee);
// { name: "Alice", age: 25, title: "Developer", company: "Tech Corp" }
This approach combines
person
andjob
into a newemployee
object, with all properties included. Notably, if both objects contain the same key, the latter value will overwrite the former.
The Rest Operator: Consolidating Values
The rest operator does the opposite of spreading—it collects elements into a single array, often simplifying function definitions.
1. Handling Variable Arguments in Functions
Before the rest operator, you’d rely on arguments
to handle variable arguments, which is less flexible. With rest, it’s easy to manage dynamic arguments.
function sum(...nums) {
return nums.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4)); // 10
Here,
sum()
can take any number of arguments, thanks to...nums
, which collects all arguments into a single array,nums
.
2. Destructuring Arrays with Rest
Rest also shines in array destructuring, helping you separate primary items from remaining elements.
const colors = ['red', 'green', 'blue', 'yellow', 'pink'];
const [primary, secondary, ...others] = colors;
console.log(primary); // "red"
console.log(secondary); // "green"
console.log(others); // ["blue", "yellow", "pink"]
With this pattern, we can easily grab the first two colors and bundle the rest in the
others
array.
3. Using Rest with Objects
Rest properties with objects allow us to select specific properties and gather the rest.
const car = { brand: 'Toyota', model: 'Corolla', year: 2021, color: 'blue' };
const { brand, ...details } = car;
console.log(brand); // "Toyota"
console.log(details); // { model: "Corolla", year: 2021, color: "blue" }
Common Use Cases for Spread and Rest in Everyday Code
Cloning: Quickly create clones of arrays or objects without affecting the original.
Merging: Merge multiple arrays or objects efficiently.
Destructuring: Pull specific items from arrays and objects, simplifying data extraction.
Dynamic Functions: Handle any number of function arguments, especially useful in math or data processing functions.
When to Use Each Operator
Deciding when to use spread or rest depends on context:
Use spread when you want to expand an array or object.
Use rest when you want to consolidate multiple elements.
Conclusion
Mastering the spread and rest operators can make your code more concise and flexible, saving time and minimizing errors. Whether you’re merging arrays, handling dynamic function arguments, or extracting specific data, these operators will make your life easier.
For more information, check out these excellent resources:
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
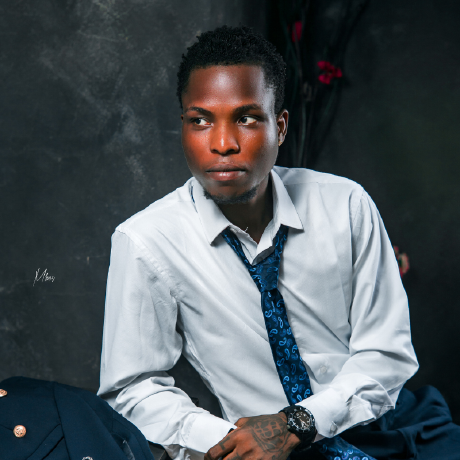
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com