The Event Loop and Call Stack in Javascript
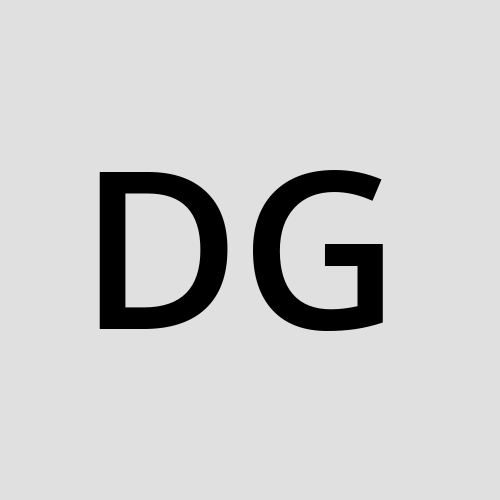

Absolutely! Let’s go through some examples to understand how the event loop and call stack work together in JavaScript.
Key Concepts
Call Stack: Keeps track of functions in execution. It works in a Last In, First Out (LIFO) manner.
Event Loop: Manages the execution of asynchronous code, checking if the call stack is empty before moving functions from the callback queue to the stack.
Callback Queue: Holds asynchronous functions that are ready to run after their main task completes (e.g.,
setTimeout
, promises).
Example 1: Synchronous Code Execution
function first() {
console.log("First function");
}
function second() {
console.log("Second function");
}
function third() {
console.log("Third function");
}
first();
second();
third();
Explanation:
Each function is called one after the other.
The call stack pushes each function, executes it, and then pops it off.
Output will be:
First function Second function Third function
Example 2: Asynchronous Code with setTimeout
Let’s introduce a setTimeout
to see how asynchronous code behaves with the event loop.
function greet() {
console.log("Hello");
}
function delay() {
setTimeout(() => {
console.log("Delayed message");
}, 1000);
}
function goodbye() {
console.log("Goodbye");
}
greet();
delay();
goodbye();
Explanation:
greet()
is called first, so "Hello" is printed immediately.delay()
is called, butsetTimeout
is asynchronous, so it sets a timer and moves the callback to the callback queue to be executed after 1 second.goodbye()
is called, so "Goodbye" is printed immediately.After the stack is empty and 1 second has passed, the event loop moves the callback from
setTimeout
into the call stack, printing "Delayed message."
Output:
Hello
Goodbye
Delayed message
Example 3: Asynchronous Code with Promises
JavaScript treats promises differently—they go into the microtask queue, which has priority over the callback queue.
console.log("Start");
setTimeout(() => {
console.log("Timeout");
}, 0);
Promise.resolve().then(() => {
console.log("Promise resolved");
});
console.log("End");
Explanation:
console.log("Start")
is called first, printing "Start".setTimeout
is called with a 0ms delay, so its callback is scheduled in the callback queue.Promise.resolve().then(...)
schedules its callback in the microtask queue.console.log("End")
is called, printing "End".The call stack is now empty, so the event loop checks the microtask queue first, finding the promise callback and printing "Promise resolved".
Finally, the callback queue is processed, and "Timeout" is printed.
Output:
Start
End
Promise resolved
Timeout
Summary
Synchronous functions go directly into the call stack.
Asynchronous functions (e.g.,
setTimeout
,fetch
,Promise
) are handled by the event loop and wait in the callback or microtask queue.Microtasks (promises) have priority over macrotasks (e.g.,
setTimeout
), so promise resolutions happen beforesetTimeout
callbacks, even with a 0ms delay.
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
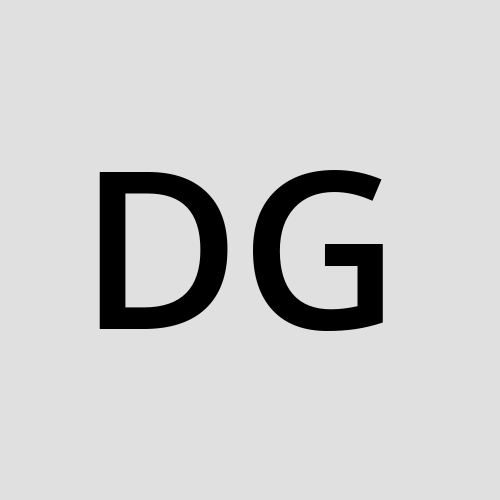
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.