Higher-Order Functions in Javascript
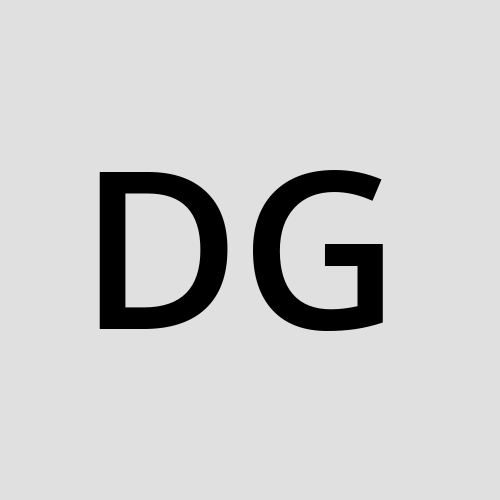
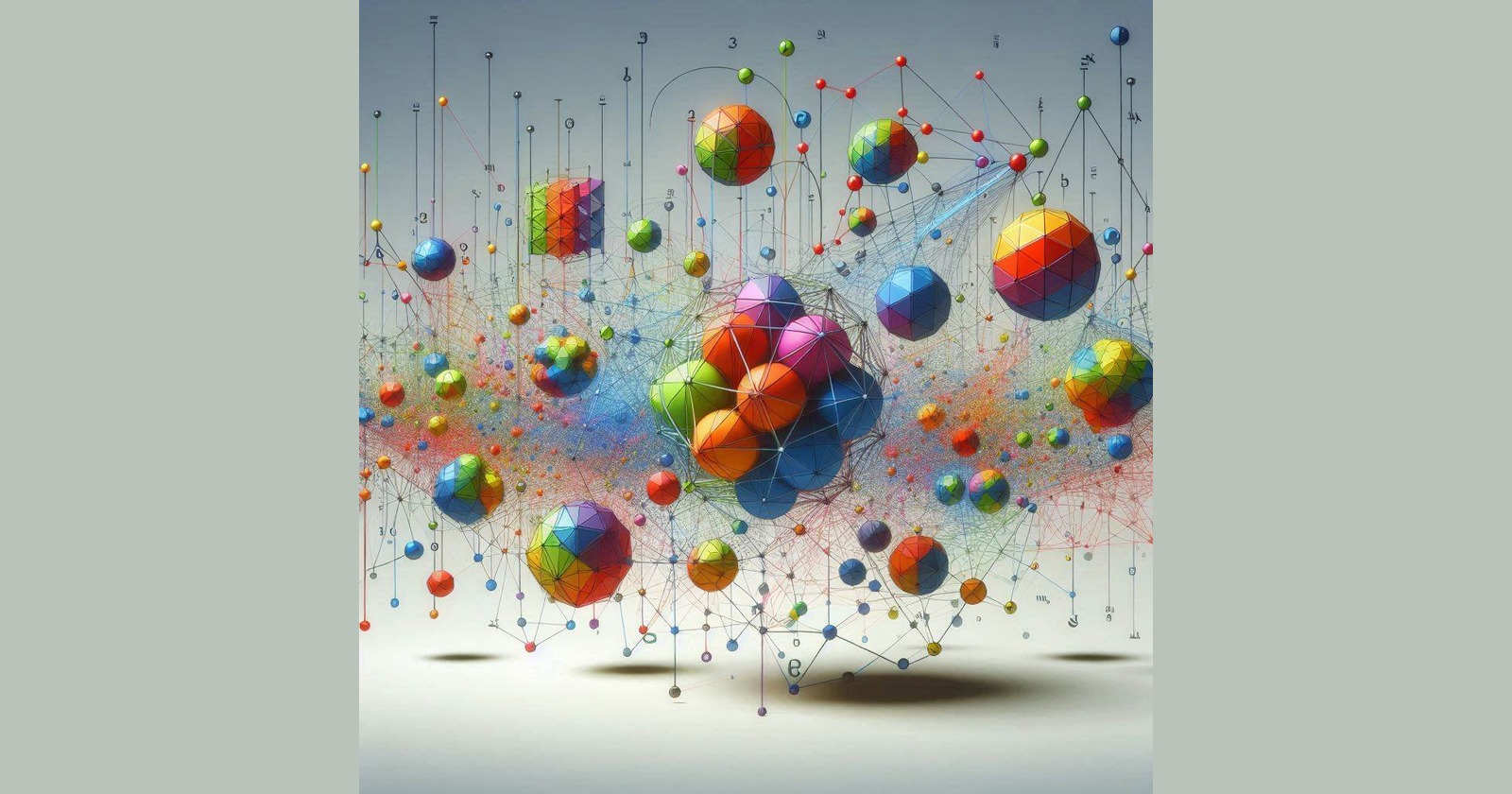
Higher-order functions (HOFs) are functions that either take other functions as arguments, return functions, or both. Here are a few common examples in JavaScript:
1. Using .map()
The .map()
function is a higher-order function because it takes a callback function as an argument. It applies this function to each element in an array and returns a new array with the transformed elements.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
In this example, num => num * 2
is a function passed into .map()
. The map
function iterates over each element in numbers
, applies the callback, and creates a new array with the results.
2. Using .filter()
The .filter()
function is another HOF that takes a function as an argument. This function returns a boolean and filters out elements that do not meet the specified condition.
const numbers = [1, 2, 3, 4, 5];
const evens = numbers.filter(num => num % 2 === 0);
console.log(evens); // Output: [2, 4]
Here, the function num => num % 2 === 0
is passed to .filter()
, which returns only the elements that satisfy the condition.
3. Using .reduce()
The .reduce()
function is a powerful HOF that takes a function as its first argument and an initial value as the second. It applies the function to accumulate values into a single output.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((total, num) => total + num, 0);
console.log(sum); // Output: 15
In this example, (total, num) => total + num
is the callback function, which accumulates the sum of all values in the array.
4. Function that Returns Another Function
A higher-order function can also return another function. Here’s an example of a multiplier function that returns a new function:
function createMultiplier(multiplier) {
return function (num) {
return num * multiplier;
};
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
In this example, createMultiplier
is a higher-order function that returns a function based on the multiplier
parameter. The returned function then multiplies a number by that multiplier.
5. Custom Higher-Order Function
You can create your own HOFs by writing functions that accept other functions as arguments. For example, here’s a forEach
function that mimics the array method:
function forEach(array, callback) {
for (let i = 0; i < array.length; i++) {
callback(array[i], i, array);
}
}
const fruits = ["apple", "banana", "cherry"];
forEach(fruits, fruit => console.log(fruit));
// Output:
// apple
// banana
// cherry
In this example, forEach
is a custom higher-order function that accepts an array and a callback, then applies the callback to each item in the array.
These examples illustrate how higher-order functions can lead to more reusable, modular, and expressive code in JavaScript.
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
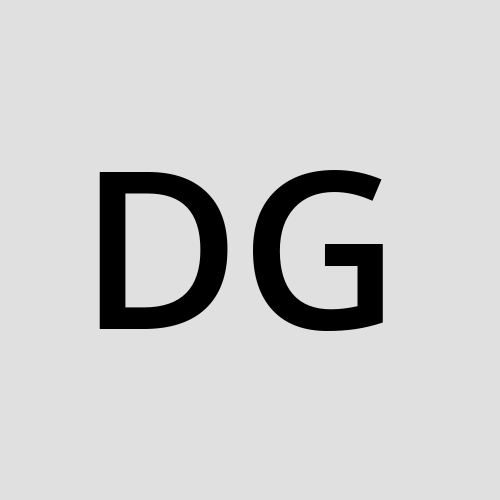
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.