Exploring Dart Records: A Better Way to Group Data
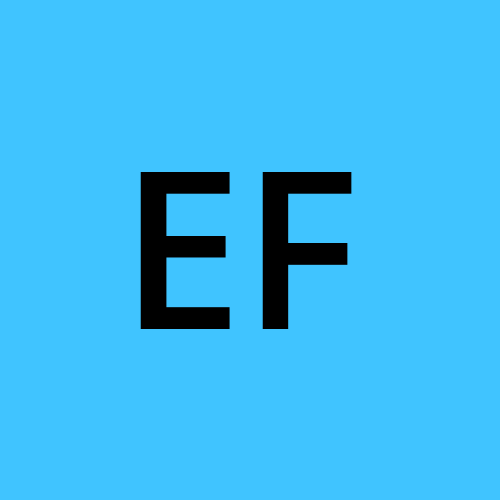
This article explores the use of Dart Records to efficiently group multiple objects. We'll examine how Records compare to other collection types, particularly List
, for grouping data of different types.
Introduction
Imagine you're working on an application that tracks employee information. You need to return multiple data such as name, age, and email from a function, but you don’t want to create a whole class just for that. In situations like these, Dart's Records can be the perfect solution.
Dart is known as a typed language, but until the introduction of Records in version 3.0, there wasn't a straightforward way to group multiple objects or data types into a single object. Unlike other collection types such as List
and Map
, Records are more type-safe and easier to manage for multiple objects. In this guide, we’ll explore why Records offer more advantages over other data grouping methods.
Prerequisite
To follow along, you should have a basic understanding of Dart’s syntax and familiarity with data structures like List
and Map
. You will need Dart version 3.0 or higher.
What is Dart’s Record?
Records are immutable(once they are created, their values cannot be changed) data structures that allow you to group multiple objects into a single object. Unlike other collection types, Records have a fixed number of fields, each with its own specific type, allowing different types of data in one structure.
Records can be stored in variables, passed to and from functions, and stored in data structures such as List
, Map
, and Set
. With Records, Dart provides a structured way to manage multiple objects without needing to define custom classes for every scenario.
How to Create and Use Records
In a Record, each element is called a field. Fields in record expressions mirror how parameters and arguments work in functions. Records are created by listing named or positional fields in a comma-delimited format within parentheses:
// A positional record
(String name, int age, String email) person1 = ('Alice', 30, 'alice@example.com');
// A record with named fields
({String name, int age, String email}) person2 = (name: 'Alice', age: 30, email: 'alice@example.com');
Accessing Record’s Fields
Fields in Records are accessible through built-in getters(a function that returns the value of a field). Records are immutable, so fields do not have setters(a function that allows you change the value of a field). For positional fields, getters are represented by $<position>
. For named fields, getters take the field’s name:
// Accessing fields in a positional record
print(person1.$1); // Outputs 'Alice'
// Accessing fields in a named record
print(person2.name); // Outputs 'Alice'
Use Cases for Records
Returning Multiple Values from a Function
Records are ideal when a function needs to return multiple values, providing a lightweight alternative to creating custom classes.
// Function that returns multiple values about a user Record<String, int, bool> fetchUserDetails(int userId) { // Imagine this is fetched from an API return ('John Doe', 29, true); // name, age, isActive } void main() { var userDetails = fetchUserDetails(1); print('Name: ${userDetails.$1}, Age: ${userDetails.$2}, Active: ${userDetails.$3}'); }
Data Grouping
Records are perfect for grouping different types of data into a single object, like grouping a number and a string without losing track of their individual types.
(int id, String name, double price) product = (101, 'Laptop', 999.99);
When to Use Records Over Collection Types
While Records are excellent for grouping related, typed data, they are not a replacement for collections like List
, Map
, or Set
. Collections are designed for varying numbers of elements of the same type, while Records are fixed in size with specific, typed fields.
Conclusion
Dart’s Records offer a type-safe solution for grouping data of different types into a single, structured object. Records simplify many coding scenarios where custom classes or untyped lists like List<dynamic>
would be used.
References and Resources
Subscribe to my newsletter
Read articles from Ewoma favour directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
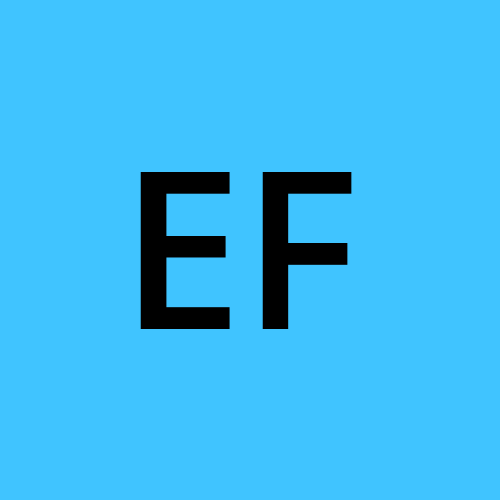