Prototypes and Prototypal Inheritance in Javascript
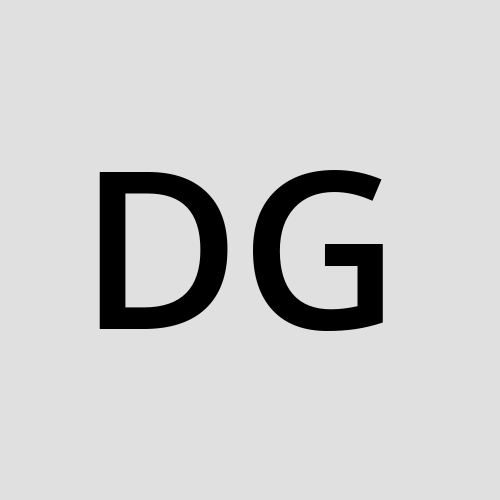
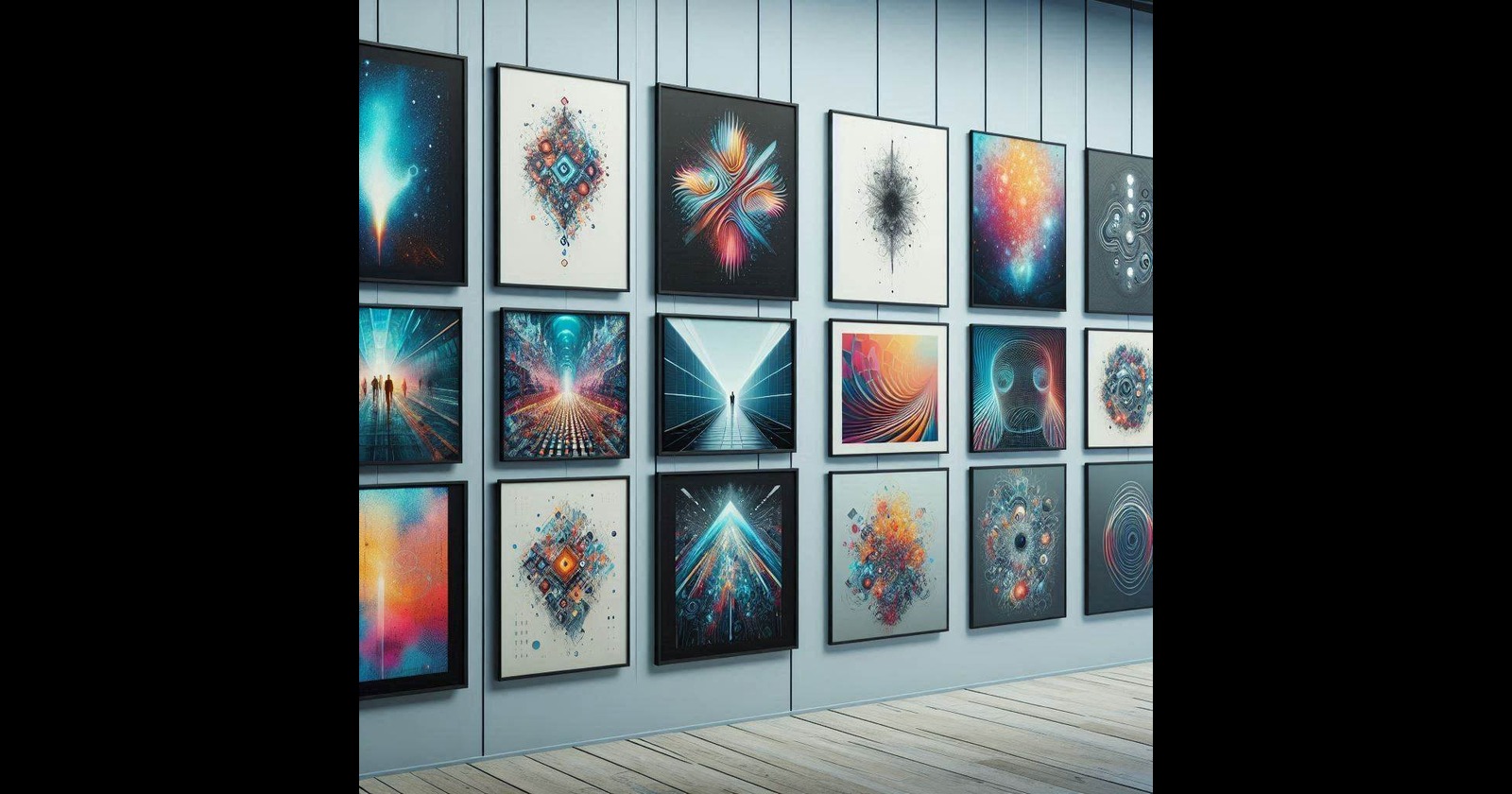
JavaScript uses prototypal inheritance to share properties and methods between objects. Here’s a basic example showing how prototypes and prototypal inheritance work.
Basic Example of a Prototype
// Creating a constructor function
function Person(name, age) {
this.name = name;
this.age = age;
}
// Adding a method to the prototype of Person
Person.prototype.sayHello = function() {
return `Hello, my name is ${this.name}.`;
};
// Creating a new instance of Person
const person1 = new Person("Alice", 30);
console.log(person1.sayHello()); // "Hello, my name is Alice."
In this example:
We define a
Person
constructor function that takesname
andage
as parameters.We add a
sayHello
method toPerson.prototype
, which will be shared by all instances ofPerson
.When we create a new instance
person1
, it has access tosayHello
through the prototype chain.
Prototypal Inheritance Example
Let's say we want to create a Student
that inherits from Person
.
// Constructor for Student
function Student(name, age, grade) {
// Call the parent constructor, Person
Person.call(this, name, age); // Inherit name and age properties from Person
this.grade = grade;
}
// Set up inheritance so that Student inherits from Person
Student.prototype = Object.create(Person.prototype);
// Restore the constructor reference (optional, but good practice)
Student.prototype.constructor = Student;
// Add a method to Student's prototype
Student.prototype.study = function() {
return `${this.name} is studying.`;
};
// Creating an instance of Student
const student1 = new Student("Bob", 20, "A");
console.log(student1.sayHello()); // "Hello, my name is Bob." (inherited from Person)
console.log(student1.study()); // "Bob is studying." (specific to Student)
In this example:
We use
Person.call
(this, name, age)
inside theStudent
constructor to inherit thename
andage
properties.Student.prototype = Object.create(Person.prototype);
sets up inheritance by creating a new object that links toPerson.prototype
.Student.prototype.constructor = Student;
restores theconstructor
property ofStudent
to avoid any issues when identifying its origin.The
sayHello
method is inherited fromPerson
, whilestudy
is unique toStudent
.
Prototype Chain in Action
When student1.sayHello()
is called, JavaScript:
Looks for
sayHello
onstudent1
itself.If it doesn't find it, it looks up the prototype chain to
Student.prototype
, then toPerson.prototype
.It finds
sayHello
onPerson.prototype
, so it calls it.
This is how JavaScript achieves inheritance without traditional class structures, using prototypes instead.
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
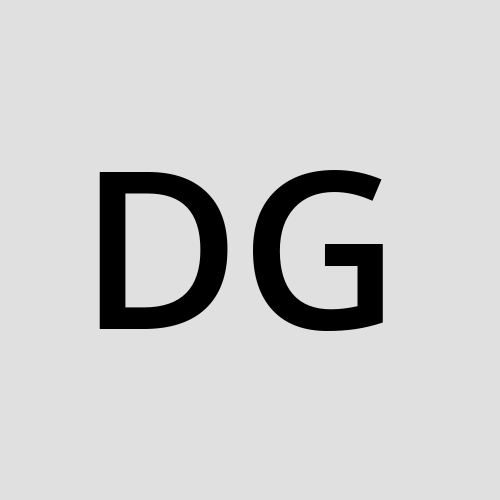
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.