Diving into Docker: Create Your First Container

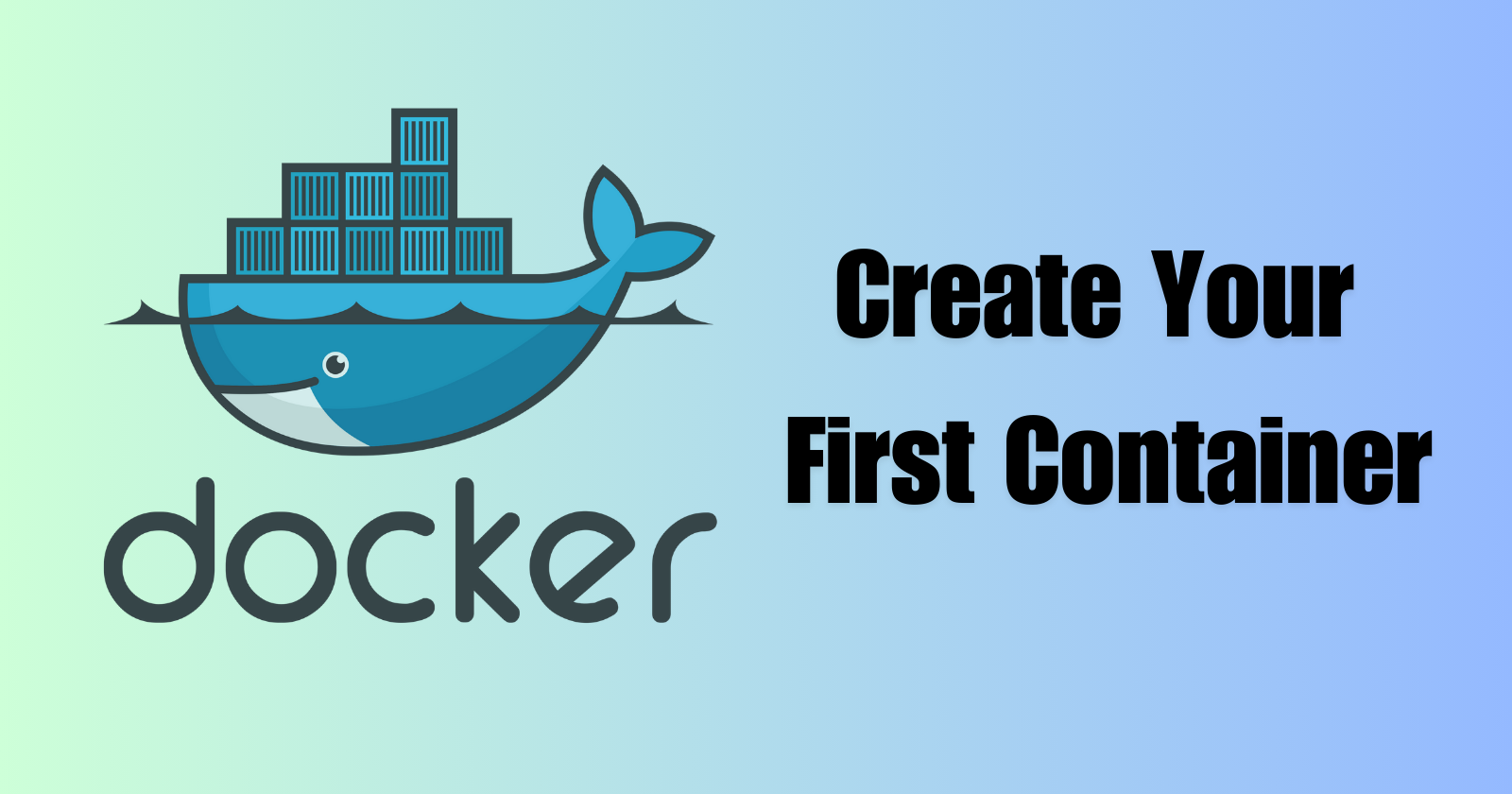
Set Up Your Environment:
Before diving into Docker, you must set up your environment. Follow these steps based on your operating system:
Install Docker:
Download Docker Desktop from the official website.
Linux: Installation methods vary based on the distribution. Here’s the official guide.
*Follow the installation instructions, and once completed, open Docker Desktop to ensure it’s running.
Text Editor:
You’ll need a text editor to create and edit files, including your Dockerfile and index.html. You can use any text editor you’re comfortable with — the two I use most often are VS Code & Vi (or Vim).
Learn the Basics:
If you’re new to Docker, get familiar with basic commands. These commands will be your tools throughout this Docker adventure:
docker ps: Lists running containers.
docker images: Lists available images.
docker build: Builds a Docker image.
docker run: Runs a container from an image.
docker login: Log in to my Docker Hub account.
docker tag: Tags an image.
docker push: Pushes an image to Docker Hub.
Create an Easy Project:
To get experience with Docker, we’ll create a simple web app.
1. Create a new directory for your project:
mkdir hello-docker
cd hello-docker
2. Write a index.html file:
You can use Visual Studio Code or a command-line text editor like Vi to create the index.html file for your web app. Both will work — it just depends on your preference!
Think of the index.html file as the heart of your website in a Docker container. It's what people see when they visit, and it's where you create the look and feel of your site.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Docker</title>
</head>
<body>
<h1>Hello from Docker!</h1>
</body>
</html>
Here's how you can do it with either option:
Using Visual Studio Code:
Open Visual Studio Code.
In Visual Studio Code, go to “File” and click “Open Folder.”
Pop to the directory where you created the hello-docker folder.
Click “Open” to open the folder in Visual Studio Code.
Inside the hello-docker folder, right-click and choose "New File."
Name the new file index.html.
Open the index.html file and paste the HTML code you provided.
Using Vi (or Vim):
Open your terminal or command prompt.
Slide to the directory where you created the hello-docker folder using the cd command.
Use the vi or vim command followed by the filename index.html to create and edit the file. For example:
vi index.html
Press “i” to enter insert mode in Vi/Vim.
Paste the HTML code you provided into the Vi/Vim editor.
Press “Esc” to exit insert mode.
Save the file & quit (exit) Vi/Vim by typing :wq and pressing Enter.
Create Your Dockerfile:
A Dockerfile is like a recipe for making a particular dish. But instead of cooking, it’s for creating a container!
A recipe tells you how to cook a meal > A Dockerfile tells your computer how to build a container.
In the Dockerfile, you list the ingredients (like what type of container to use) and the steps (what software to add). A Dockerfile is a set of instructions at its very basics.
With a Dockerfile, you can make your container unique by adding special software or settings. It’s like adding your own secret sauce to a recipe.
In short, a Dockerfile helps you make a customized container with everything you need for your project. It’s like following a recipe but for containers!
Create your Dockerfile:
Choose a base image: For this example, select the nginx image.
Create a file named Dockerfile (without an extension) in the hello-docker directory.
Write the Dockerfile.
Here’s how you can write a Dockerfile using either Vi (or Vim) and Visual Studio Code:
Using Visual Studio Code:
Open Visual Studio Code.
Go to “File” and select “Open Folder.”
Navigate to the hello-docker directory and click "Open" to open the folder in Visual Studio Code.
Inside the hello-docker folder, right-click and choose "New File."
Name the new file Dockerfile (without an extension).
Open the Dockerfile in Visual Studio Code.
Paste the following Dockerfile content into the Visual Studio Code editor & save the file:
# Use an official Nginx web server image as the base image
FROM nginx:latest
# Copy your HTML file from the host to the web server's document root
COPY index.html /usr/share/nginx/html
# Expose port 80 to allow external access
EXPOSE 80
# Define the command to start the web server
CMD ["nginx", "-g", "daemon off;"]
Using Vi (or Vim):
Open your terminal and navigate to the hello-docker directory where you want to create the Dockerfile.
Use the following command to create and edit the Dockerfile with Vi:
vi Dockerfile
3. Press the “i” key to enter insert mode in Vi/Vim.
4. Copy and paste the following Dockerfile content into the Vi editor:
# Use an official Nginx web server image as the base image
FROM nginx:latest
# Copy your HTML file from the host to the web server's document root
COPY index.html /usr/share/nginx/html
# Expose port 80 to allow external access
EXPOSE 80
# Define the command to start the web server
CMD ["nginx", "-g", "daemon off;"]
5. Press the “Esc” key to exit insert mode.
6. Save the file and exit Vi by typing :wq and pressing Enter.
Build the Docker image:
docker build -t my-custom-image .
\ Replace ‘my-custom-inage’ with the name you want for your Docker image.*
Let’s break down the above Docker command: docker build -t my-custom-image .
docker build: This command tells Docker to build a new image. Think of it as instructing Docker to create a container recipe.
-t my-custom-image: The -t flag stands for "tag," which gives a name or label to the image you're building. In my example, we're naming the image "my-custom-image." It's like naming a dish you're preparing!!
. (period): The period at the end of the command is crucial. It tells Docker to look for the Dockerfile in the current directory.
The whole command tells Docker:: Build a Docker image using the instructions in the Dockerfile found in this folder, and name it ‘my-custom-image’.
Test Your Container:
After the image is built, you can run a container based on this image to view your webpage using the following command:
docker run -p 8080:80 my-custom-image
This command maps port 8080 on your host machine to port 80 in the container, allowing you to access the webpage via http://localhost:8080 in your web browser.
Our simple “Hello Docker” webpage should now be accessible on http://localhost:8080
To break this down more, docker run: This command part tells Docker to run a container based on the specified image.
-p 8080:80: The -p flag is used to map ports between your host machine and the container. It maps port 8080 on your host to port 80 in the container. Any traffic to port 8080 on your computer will be redirected to port 80 inside the running container.
my-custom-image: This is the name of the Docker image from which you want to run a container. It's the image we built earlier.
Why do we use this command?
When you run a Docker container, it tends to run in isolation, and its network ports are not directly accessible from your host machine. By using the -p flag to map ports, you're essentially creating a bridge between your host and the container.
Here's what happens:
Your web application inside the container listens on port 80 (the default HTTP port).
When you access http://localhost:8080 in your web browser, your computer requests to port 8080 on your host.
Docker sees that you’ve mapped port 8080 to port 80 in the container, so it forwards the request to the container’s port 80.
Inside the container, your web application receives the request, processes it, and returns the response to your host, which then displays it in your web browser.
This ^ setup allows you to access your web application running in the Docker container via your web browser on your host machine using http://localhost:8080. You're essentially "proxying" requests from your host to the container.
So, when you run the docker run command with the port mapping as described, your "Hello Docker" webpage should become accessible on http://localhost:8080.
Push Your Docker Image to Docker Hub
If you want to share your custom Docker image with others or make it accessible for deployment on different systems, you can push it to Docker Hub, a cloud-based registry for Docker images. Here’s how you can do it:
Create an Account on Docker Hub:
If you haven’t already, visit the Docker Hub website and sign up for a free Docker Hub account. You’ll need this account to publish and share your Docker images.
Log in to Docker Hub via the Terminal:
Open your terminal or command prompt and enter the following command:
*Note: You’ll be prompted to enter your Docker Hub username and password. Provide the credentials you created when signing up for Docker Hub.
docker login
Tag Your Image:
Before pushing your image, tag it with your Docker Hub username and a repository name. This makes it easier to identify and locate your image on Docker Hub.
\Note: Replace my-custom-image with the name of your Docker image and yourusername with your Docker Hub username.*
docker tag my-custom-image:latest yourusername/my-custom-image:latest
Push Your Image:
Push your tagged image to Docker Hub using the following command:
docker push yourusername/my-custom-image:latest
Once the push process is complete, you should see messages indicating a successful upload. Your Docker image is now hosted on Docker Hub and can be pulled by others using the docker pull command.
Conclusion
Diving into Docker and creating your first container is an exciting and rewarding experience. By setting up your environment, learning the basic commands, and following the step-by-step guide to create a simple web app, you gain hands-on experience with Docker. Building and running your custom Docker image allows you to see the power and flexibility of containerization. Additionally, pushing your Docker image to Docker Hub enables you to share your work with others and deploy it across different systems. With these foundational skills, you're well on your way to exploring more advanced Docker features and integrating containerization into your development workflow.
Connect and Follow:
Like👍 | Share📲 | Comment💭
Subscribe to my newsletter
Read articles from Nikunj Vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikunj Vaishnav
Nikunj Vaishnav
👋 Hi there! I'm Nikunj Vaishnav, a passionate QA engineer Cloud, and DevOps. I thrive on exploring new technologies and sharing my journey through code. From designing cloud infrastructures to ensuring software quality, I'm deeply involved in CI/CD pipelines, automated testing, and containerization with Docker. I'm always eager to grow in the ever-evolving fields of Software Testing, Cloud and DevOps. My goal is to simplify complex concepts, offer practical tips on automation and testing, and inspire others in the tech community. Let's connect, learn, and build high-quality software together! 📝 Check out my blog for tutorials and insights on cloud infrastructure, QA best practices, and DevOps. Feel free to reach out – I’m always open to discussions, collaborations, and feedback!