Introduction to ViewModel: Simplifying Communication Between View and Data

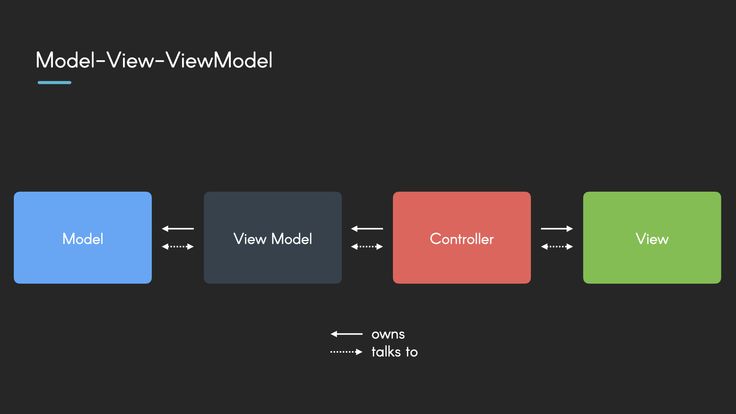
If you’re an Android developer, you’ve likely heard of ViewModel
or even used it before. Today, let’s dive into what a ViewModel
is, why it’s essential, and how it integrates within the architecture of an Android app.
As someone transitioning from web development to mobile, I realized that the learning curve in mobile development can be more complex, especially if you want to do things right. ViewModel
is a central concept to understand for building well-structured applications.
What is the ViewModel, and Why Was It Introduced?
The ViewModel
isn’t just there to preserve app state during screen rotations, though that’s one of its benefits. Its purpose goes beyond that. In a well-architected Android app, we typically see three main layers: the Data Layer
, the Domain Layer
(optional), and the View Layer
. Here’s what each layer does:
Data Layer: This layer manages all data-related tasks in the app, like databases and API calls.
View Layer: This layer includes everything related to the user interface, such as buttons, text, and images – essentially, what the user sees and interacts with.
Domain Layer (optional): If present, this layer handles the core business logic of the app, the logic that is independent of the UI or specific data sources.
The ViewModel
acts as a bridge between the Data Layer
and the View Layer
. Its primary role is to manage UI-related data in a lifecycle-conscious way. In other words, the ViewModel
keeps data available even if the activity or fragment is recreated, like during a screen rotation.
Why Not Access Data Directly from the View?
Although it’s technically possible for the View to directly access the Data Layer
, it’s not recommended. Direct access compromises the separation of concerns and can lead to code that’s harder to maintain and test. In an MVVM architecture, the View should only communicate with the ViewModel
to obtain data. The ViewModel
then handles data and UI logic, allowing the View to stay lightweight and focused solely on display.
The ViewModel’s Role in a Well-Designed Architecture
Simply put, the ViewModel
's main role is to be a bridge between the data and the view layers. It has two primary functions:
Expose Data to the View: The
ViewModel
exposes the final data that the UI needs to display. This data is often represented byLiveData
orStateFlow
objects in Kotlin, allowing the View to observe it and automatically update when changes occur. These are known as "states."Handle User Events: The
ViewModel
provides functions for handling events that trigger data changes. For instance, if a user clicks a button to refresh information, theViewModel
takes care of calling the necessary API, retrieving data, and updating the state so the View reflects the new information.
How Does a ViewModel Work in Practice?
Let’s look at a simple example. Imagine you have an e-commerce app, and you want to display the latest product in stock. With a ViewModel
, the process would look like this:
The View (UI) asks the
ViewModel
to fetch the latest product in stock.The
ViewModel
, acting as a bridge, interacts with theData Layer
to retrieve information about the product.Once the data is retrieved, the
ViewModel
updates the state that the View is observing.The View observes this state and automatically updates to display the new product.
This separation makes the code much easier to read and maintain because each layer has a specific, well-defined role.
Best Practices for Using ViewModel
Avoid Storing UI Elements: The
ViewModel
should contain only app data, not direct references to UI elements (such as anActivity
orFragment
) to avoid memory leaks.Use LiveData or StateFlow: These types allow the View to subscribe to the data in the
ViewModel
and react in real time when the state changes.Centralize Data Management Logic: All logic related to data interaction (like API calls) should be handled by the
ViewModel
to keep the View lightweight and independent of data.
By following these practices, you can build Android applications that are robust, performant, and easy to maintain. The ViewModel
is a powerful tool when understood and used correctly.
Subscribe to my newsletter
Read articles from nathan kayumba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

nathan kayumba
nathan kayumba
I'm a passionate software engineer with a strong focus on backend development and mobile app creation. I specialize in building native Android applications using Kotlin and Jetpack Compose. With a background in software engineering, I enjoy solving complex problems and creating seamless user experiences. When I'm not coding, you'll find me immersed in music, constantly seeking inspiration from new sounds. Let's build the future, one app at a time!