AWS CodePipeline
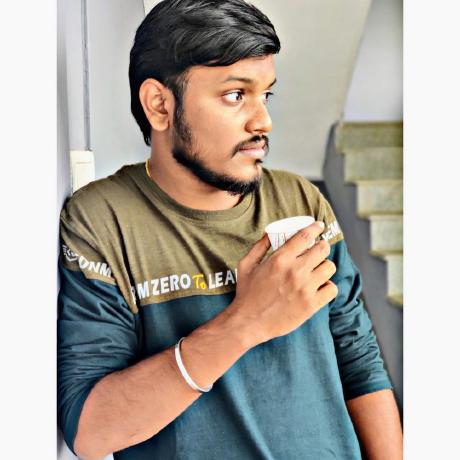
Understanding AWS CodePipeline
AWS CodePipeline is a fully managed continuous integration and continuous delivery service offered by Amazon Web Services (AWS). It automates the build, test, and deployment phases of your release process, allowing for the rapid and reliable delivery of applications and infrastructure updates. Its core strength lies in its flexibility and integration capabilities, enabling seamless orchestration of various tools and services within the AWS ecosystem and beyond.
Advantages of CodePipeline:
- Visual Pipeline Design: CodePipeline provides a user-friendly interface to create, model, and visualize your release pipelines.
- Integration with Multiple Tools: It integrates seamlessly with other AWS services, third-party tools, and even your custom scripts to build and deploy your application.
- Automated and Reliable: CodePipeline automates the entire release process, ensuring that your code is continuously integrated, tested, and deployed without manual intervention.
Key Features and Benefits
Pipeline Creation and Visualization: CodePipeline allows developers to construct customizable pipelines that visualize the workflow stages from source code through to production deployment. This visual representation simplifies understanding and debugging of the delivery process.
Flexibility and Extensibility: Integration with other AWS services like AWS CodeBuild, AWS CodeDeploy, and third-party tools via custom actions allows for a tailored CI/CD pipeline. It supports multiple source code repositories like AWS CodeCommit, GitHub, and Bitbucket, offering flexibility in source control.
Automated Testing and Validation: CodePipeline facilitates automated testing at various stages, ensuring code quality and reliability before deployment. Integration with AWS testing services like AWS CodeBuild and AWS Device Farm streamlines the testing process.
Security and Compliance: AWS CodePipeline ensures security measures by providing encryption options, secure access controls, and compliance with industry standards. It also supports fine-grained permissions using AWS Identity and Access Management (IAM).
Scalability and Performance: Being a fully managed service, CodePipeline can automatically scale based on the workload, ensuring consistent performance even during high-demand periods.
Workflow of AWS CodePipeline
The typical workflow in AWS CodePipeline involves stages such as:
Source: Fetching the source code from a repository (GitHub, AWS CodeCommit, etc.).
Build: Compiling the code, running tests, and producing deployable artifacts (using AWS CodeBuild or similar services).
Deploy: Automating the deployment of artifacts to various environments, integrating with services like AWS CodeDeploy.
Approval: Manual approval steps for human intervention or compliance checks.
Invoke: Triggering subsequent actions or services based on the pipeline's successful completion.
Real-world Applications
AWS CodePipeline finds applications across various industries and use cases:
Software Development: Streamlining the release cycles for web applications, mobile apps, and software updates.
Infrastructure as Code (IaC): Automating the deployment of infrastructure changes using tools like AWS CloudFormation.
DevOps Practices: Facilitating the adoption of DevOps methodologies by automating processes and reducing manual interventions.
Continuous Integration and Deployment: Enabling teams to deliver updates rapidly and consistently while maintaining quality standards.
Scenario:
Let's consider a simple web application built using Node.js and deployed on AWS Elastic Beanstalk. The project is stored in a GitHub repository.
Steps to Set Up AWS CodePipeline:
1. Configuration in AWS Management Console:
AWS CodePipeline:
Go to the AWS Management Console and open the CodePipeline service.
Click on "Create pipeline" and give it a name like "MyWebAppPipeline."
Source Stage:
Choose GitHub as the source provider and connect to the repository containing your Node.js web application code.
Select the branch you want to deploy.
Build Stage:
Use AWS CodeBuild as the build provider.
Configure the build settings to install dependencies, run tests, and package the application.
Deploy Stage:
For deployment, choose AWS Elastic Beanstalk as the deployment provider.
Specify the application and environment details where you want to deploy the code.
2. Pipeline Execution:
Once the pipeline is created, it automatically starts the workflow:
Source Stage:
- CodePipeline fetches the source code from the GitHub repository.
Build Stage:
- CodeBuild triggers the build process. It installs dependencies, runs tests, and generates the deployment artifacts.
Deploy Stage:
- The deployment artifacts are sent to Elastic Beanstalk, which deploys the updated application to the specified environment.
Approval Stage (if included):
- If an approval stage is present, designated personnel can review the changes and manually approve the deployment.
3. Monitoring and Management:
Pipeline Monitoring:
- Track the progress of the pipeline in the AWS CodePipeline console. Monitor each stage's execution and check for any failures or issues.
Logging and Notifications:
- Set up logging and notifications to receive alerts or notifications in case of pipeline failures or successful deployments.
Certainly! In AWS CodePipeline, you can define your pipeline using YAML through AWS CloudFormation templates or AWS CDK (Cloud Development Kit). Here's an example using AWS CDK, which allows you to define infrastructure as code using programming languages like TypeScript or Python. Let's create a simple pipeline using TypeScript and CDK.
TypeScript Example using AWS CDK:
Firstly, make sure you have AWS CDK installed and configured.
MyWebAppPipelineStack.ts
(TypeScript file):
import * as cdk from '@aws-cdk/core';
import * as codepipeline from '@aws-cdk/aws-codepipeline';
import * as codepipelineActions from '@aws-cdk/aws-codepipeline-actions';
import * as codebuild from '@aws-cdk/aws-codebuild';
import * as codecommit from '@aws-cdk/aws-codecommit';
export class MyWebAppPipelineStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Define the CodePipeline
const pipeline = new codepipeline.Pipeline(this, 'MyWebAppPipeline', {
pipelineName: 'MyWebAppPipeline',
});
// Source stage - GitHub repository
const sourceOutput = new codepipeline.Artifact();
const sourceAction = new codepipelineActions.GitHubSourceAction({
actionName: 'GitHub_Source',
output: sourceOutput,
oauthToken: cdk.SecretValue.secretsManager('github-token'), // Replace with your GitHub token
owner: 'YourGitHubUserName',
repo: 'YourGitHubRepositoryName',
branch: 'main',
trigger: codepipelineActions.GitHubTrigger.WEBHOOK,
});
pipeline.addStage({
stageName: 'Source',
actions: [sourceAction],
});
// Build stage - AWS CodeBuild
const buildOutput = new codepipeline.Artifact();
const buildProject = new codebuild.PipelineProject(this, 'MyWebAppBuild', {
buildSpec: codebuild.BuildSpec.fromSourceFilename('buildspec.yml'),
});
const buildAction = new codepipelineActions.CodeBuildAction({
actionName: 'CodeBuild',
input: sourceOutput,
outputs: [buildOutput],
project: buildProject,
});
pipeline.addStage({
stageName: 'Build',
actions: [buildAction],
});
// Deployment stage - AWS Elastic Beanstalk (Sample, replace with your deployment target)
// Assuming Elastic Beanstalk environment is already set up
const deployAction = new codepipelineActions.ElasticBeanstalkDeployAction({
actionName: 'Deploy',
applicationName: 'MyWebApp',
input: buildOutput,
environmentName: 'MyWebAppEnvironment',
});
pipeline.addStage({
stageName: 'Deploy',
actions: [deployAction],
});
}
}
buildspec.yml
(Example Build Specification File):
version: 0.2
phases:
install:
runtime-versions:
nodejs: 14
pre_build:
commands:
- npm install
build:
commands:
- npm run build
artifacts:
files:
- '**/*'
discard-paths: yes
Explanation:
The TypeScript file
MyWebAppPipelineStack.ts
defines a CDK stack that sets up a CodePipeline with three stages: Source (GitHub), Build (CodeBuild), and Deploy (Elastic Beanstalk).The
buildspec.yml
file represents the build specification used by AWS CodeBuild. It defines the commands to install dependencies, build the project, and specify the artifacts to be generated.You would need to replace placeholders like
YourGitHubUserName
,YourGitHubRepositoryName
, GitHub tokens, and AWS deployment details with your actual project information.
This TypeScript code showcases how to define a CodePipeline using AWS CDK, integrating GitHub as a source, CodeBuild for building the application, and Elastic Beanstalk for deployment. You can further expand this example to include additional stages, tests, approvals, and more as per your project requirements.
Some important Questions!
What is AWS CodePipeline?
AWS CodePipeline is a fully managed continuous integration and continuous delivery (CI/CD) service that automates the release process of software applications. It enables developers to build, test, and deploy their code changes automatically and efficiently.
How does CodePipeline work?
CodePipeline orchestrates the flow of code changes through multiple stages. Each stage represents a step in the release process, such as source code retrieval, building, testing, and deployment. Developers define the pipeline structure, including the sequence of stages and associated actions, to automate the entire software delivery lifecycle.
Explain the basic structure of a CodePipeline.
A CodePipeline consists of stages, actions, and transitions. Stages are logical phases of the pipeline, actions are the tasks performed within those stages (e.g., source code checkout, deployment), and transitions define the flow of execution between stages.
What are artifacts in CodePipeline?
Artifacts are the output files generated during the build or compilation phase of the pipeline. These artifacts are the result of successful action and are used as inputs for subsequent stages. For example, an artifacts could be a packaged application ready for deployment.
Describe the role of the Source stage in CodePipeline.
The Source stage is the starting point of the pipeline. It retrieves the source code from a version control repository, such as GitHub or AWS CodeCommit. When changes are detected in the repository, the Source stage triggers the pipeline execution.
How can you prevent unauthorized changes to the pipeline?
Access to CodePipeline resources can be controlled using AWS Identity and Access Management (IAM) policies. By configuring IAM roles and permissions, you can restrict access to only authorized individuals or processes, preventing unauthorized modifications to the pipeline.
Can you explain the concept of a manual approval action?
A manual approval action is used to pause the pipeline and require human intervention before proceeding to the next stage. This action is often employed for production deployments, allowing a designated person to review and approve changes before they are released.
What is a webhook in CodePipeline?
A webhook is a mechanism that allows external systems, such as version control repositories like GitHub, to automatically trigger a pipeline execution when code changes are pushed. This integration facilitates the continuous integration process by initiating the pipeline without manual intervention.
How can you parallelize actions in CodePipeline?
Parallel execution of actions is achieved by using parallel stages. Within a stage, you can define multiple actions that run concurrently, optimizing the pipeline's execution time and improving overall efficiency.
What's the difference between AWS CodePipeline and AWS CodeDeploy?
AWS CodePipeline manages the entire CI/CD workflow, encompassing various stages like building, testing, and deploying. AWS CodeDeploy, on the other hand, focuses solely on the deployment phase by automating application deployment to instances or services.
Describe a scenario where you'd use a custom action in CodePipeline.
A custom action is useful when integrating with third-party tools or services that are not natively supported by CodePipeline's built-in actions. For example, you could create a custom action to integrate with a specialized security scanning tool.
How can you handle different deployment environments (e.g., dev, test, prod) in CodePipeline?
To handle different deployment environments, you can create separate stages for each environment within the pipeline. This allows you to customize the deployment process, testing procedures, and configurations specific to each environment.
Explain how you would set up automatic rollbacks in CodePipeline.
Automatic rollbacks can be set up using CloudWatch alarms and AWS Lambda functions. If the deployment triggers an alarm (e.g., the error rate exceeds a threshold), the Lambda function can initiate a rollback by deploying the previous version of the application.
How do you handle sensitive information like API keys in your CodePipeline?
Sensitive information, such as API keys or database credentials, should be stored in AWS Secrets Manager or AWS Systems Manager Parameter Store. During pipeline execution, you can retrieve these secrets and inject them securely into the deployment process.
Describe Blue-Green deployment and how it can be achieved with CodePipeline.
Blue-Green deployment involves running two separate environments (blue and green) concurrently. CodePipeline can achieve this by having distinct stages for each environment, allowing testing of the new version in the green environment before redirecting traffic from blue to green.
What is the difference between a pipeline and a stage in CodePipeline?
A pipeline represents the end-to-end workflow, comprising multiple stages. Stages are the individual components within the pipeline, each responsible for specific actions or tasks.
How can you incorporate testing into your CodePipeline?
Testing can be integrated into CodePipeline by adding testing actions to appropriate stages. Unit tests, integration tests, and other types of tests can be performed as part of the pipeline to ensure code quality and functionality.
What happens if an action in a pipeline fails?
If an action fails, CodePipeline can be configured to respond in various ways. It can stop the pipeline, notify relevant stakeholders, trigger a rollback, or continue with the pipeline execution based on predefined conditions and actions.
Explain how you can create a reusable pipeline template in CodePipeline.
To create a reusable pipeline template, you can use AWS CloudFormation. Define the pipeline structure, stages, and actions in a CloudFormation template. This enables you to consistently deploy pipelines across multiple projects or applications.
Can you integrate CodePipeline with on-premises resources?
Yes, you can integrate CodePipeline with on-premises resources using the AWS CodePipeline on-premises action. This allows you to connect your existing tools and infrastructure with your AWS-based CI/CD pipeline, facilitating hybrid deployments.
Subscribe to my newsletter
Read articles from Ashwin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
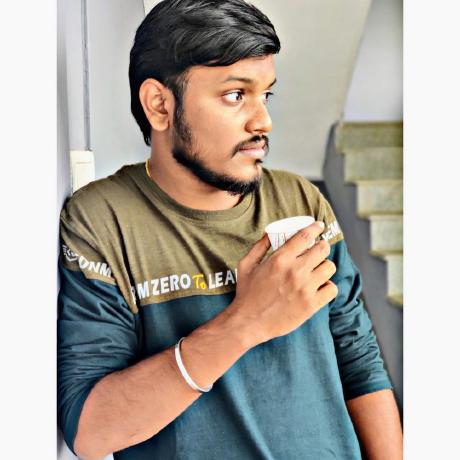
Ashwin
Ashwin
I'm a DevOps magician, conjuring automation spells and banishing manual headaches. With Jenkins, Docker, and Kubernetes in my toolkit, I turn deployment chaos into a comedy show. Let's sprinkle some DevOps magic and watch the sparks fly!