Control Flow Statements in Python
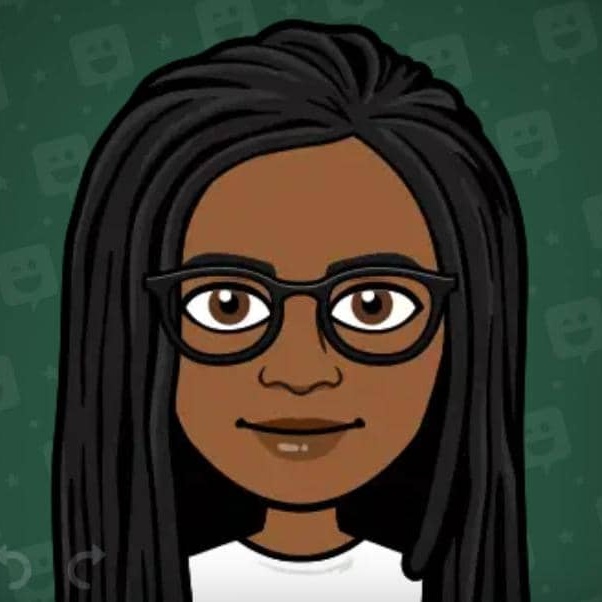
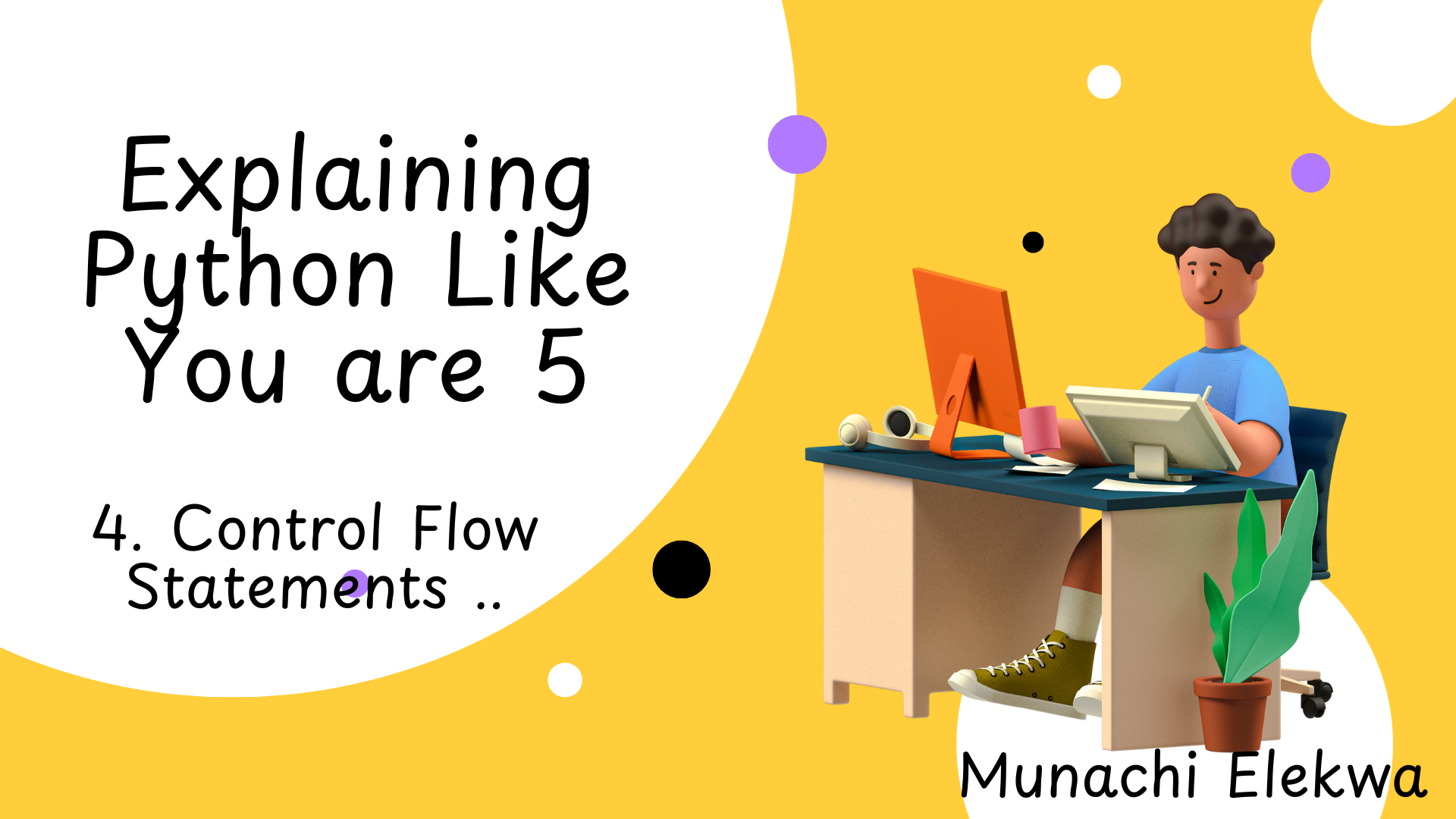
Welcome Back!
If you’re here for the fourth time, fantastic job following along! And if this is your first time, don’t worry—you can quickly catch up with the basics from the previous articles and join us right here. In this lesson, we’re diving into the exciting world of control flow—the part of programming that helps us decide what the program should do based on different conditions.
In Python, control flow statements allow us to:
Make decisions based on certain conditions (Conditional Statements).
Repeat actions as needed (Loops).
Alter the flow inside a loop with special commands (Transfer Statements).
By the end, you’ll know how to make your code a bit smarter by guiding it with conditions and loops.
What Are Control Flow Statements?
Think of control flow as choosing different paths based on your decisions. Imagine if you’re at a traffic light:
If the light is green, you go.
If it’s red, you stop.
If it’s yellow, you prepare to stop.
This is control flow in real life! In programming, control flow statements help the program "decide" what action to take.
Conditional Statements: Making Decisions with if
, else
, and elif
Conditional statements allow your code to ask questions and take different actions based on the answers.
if
Statement: This statement checks a condition. If the condition isTrue
, it runs a specific block of code.else
Statement: This acts as a backup plan. If theif
condition isFalse
, theelse
code block runs.elif
Statement: Short for “else if,” this allows you to check multiple conditions, one after the other.
Let’s see this in action:
Example:
weather = "sunny"
if weather == "rainy":
print("Take an umbrella!")
elif weather == "sunny":
print("Wear sunglasses!")
else:
print("Have a nice day!")
In this code:
If the weather is “rainy,” it prints:
Take an umbrella!
If it’s “sunny,” it prints:
Wear sunglasses!
If neither condition is
True
, it defaults toHave a nice day!
Loops (Iterative Statements): Doing Things Over and Over
Loops allow us to repeat a block of code multiple times without having to write it out again and again. This is useful when we want to process items one by one or repeat actions until a condition is met.
a. The for
Loop
A for
loop lets you repeat an action for each item in a sequence (like a list or a string).
Example: Let’s greet each friend in a list:
friends = ["Muna", "Ella", "Zane"]
for friend in friends:
print("Hello, " + friend + "!")
Output:
Hello, Muna!
Hello, Ella!
Hello, Zane!
This loop goes through each friend in the list and prints a friendly greeting. It’s like saying, “For each friend, say hello!”
b. The while
Loop
A while
loop repeats as long as a certain condition is True
. It’s like saying, “While I’m thirsty, keep drinking water.”
Example: Let’s count up to 3:
count = 1
while count <= 3:
print("Counting:", count)
count += 1
Output:
Counting: 1
Counting: 2
Counting: 3
Here, the code will keep counting up from 1 until it reaches 3.
Transfer Statements: break
and continue
Transfer statements are special commands used inside loops to change the flow.
break
: This statement stops the loop immediately, regardless of its original condition.continue
: This skips the rest of the code in the current loop iteration and moves to the next one.
Example with break
for number in [1, 2, 3, 4, 5]:
if number == 3:
break
print(number)
Output:
1
2
The loop stops when it reaches 3
, so only 1
and 2
are printed.
Example with continue
for number in [1, 2, 3, 4, 5]:
if number == 3:
continue
print(number)
Output:
1
2
4
5
When the loop encounters 3
, it skips printing it but continues with the next numbers.
Common Mistakes to Avoid
Infinite Loops: A
while
loop without a clear exit condition can run forever! Always make sure it has a way to stop.Indentation Errors: In Python, indentation is key. Ensure code blocks under
if
,else
,for
, orwhile
statements are properly indented.Multiple Conditions: Using
elif
is more efficient than writing many separateif
statements.
Mini Challenge: Test Your Control Flow Skills
Try the following exercises to see how well you understand control flow!
Challenge 1: Age Checker
Write a program that asks the user for their age, then:
If the age is 18 or above, prints “You’re an adult!”
If the age is below 18, prints “Still a minor!”
age = int(input("Enter your age: "))
if age >= 18:
print("You're an adult!")
else:
print("Still a minor!")
Challenge 2: Practice with Loops and Transfer Statements
Code Snippet:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num == 4:
break
print(num)
Question: What will the output be? (Hint: The loop stops when it reaches 4
.)
Subscribe to my newsletter
Read articles from Munachi Elekwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
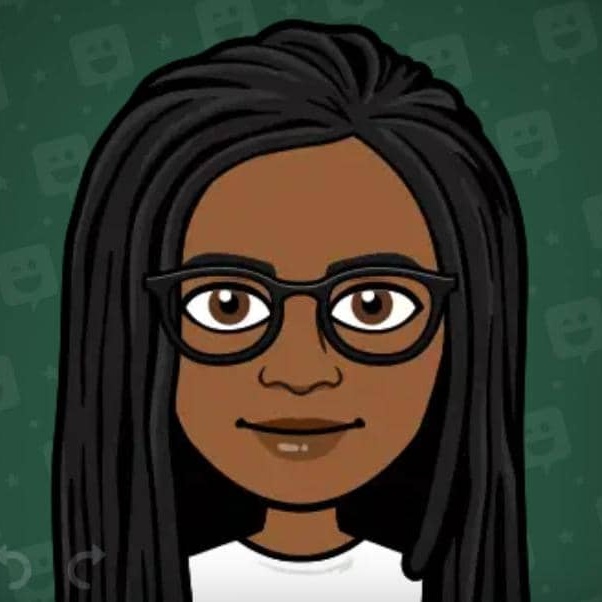
Munachi Elekwa
Munachi Elekwa
I'm Software developer with a sweet tooth.