Application Security in Android: A Comprehensive Guide [PART 3]
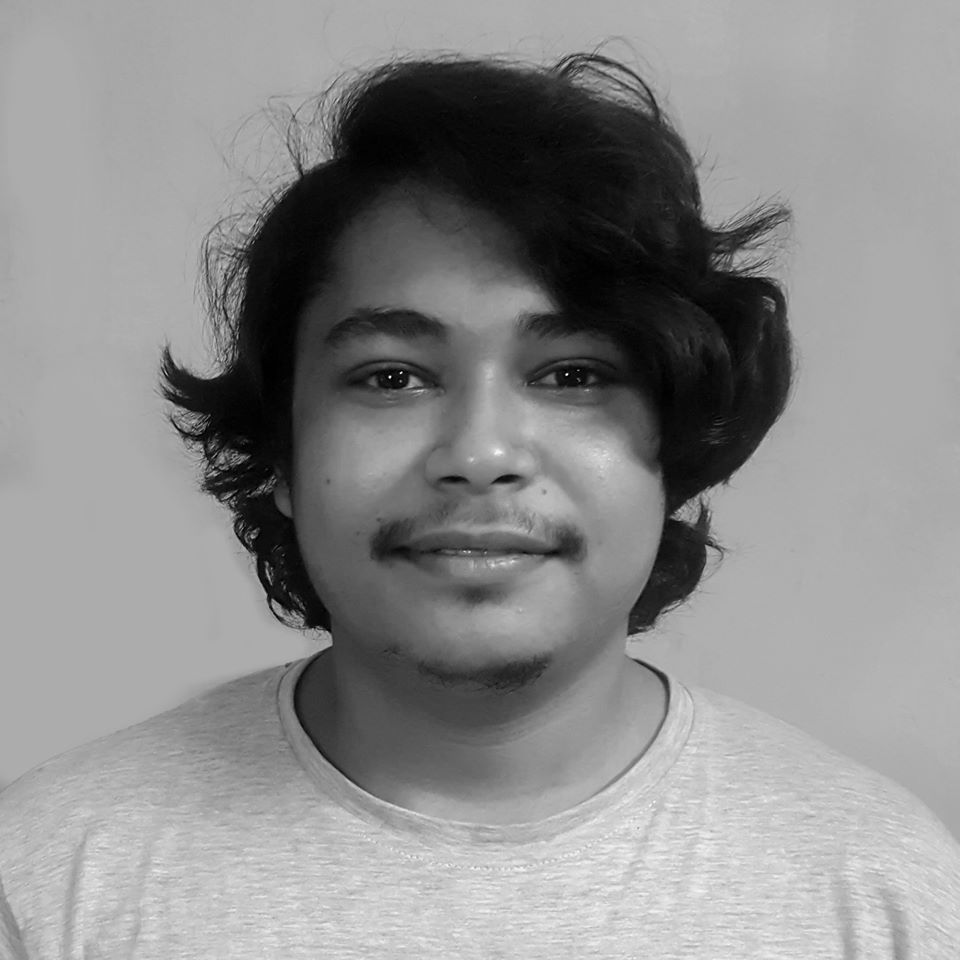
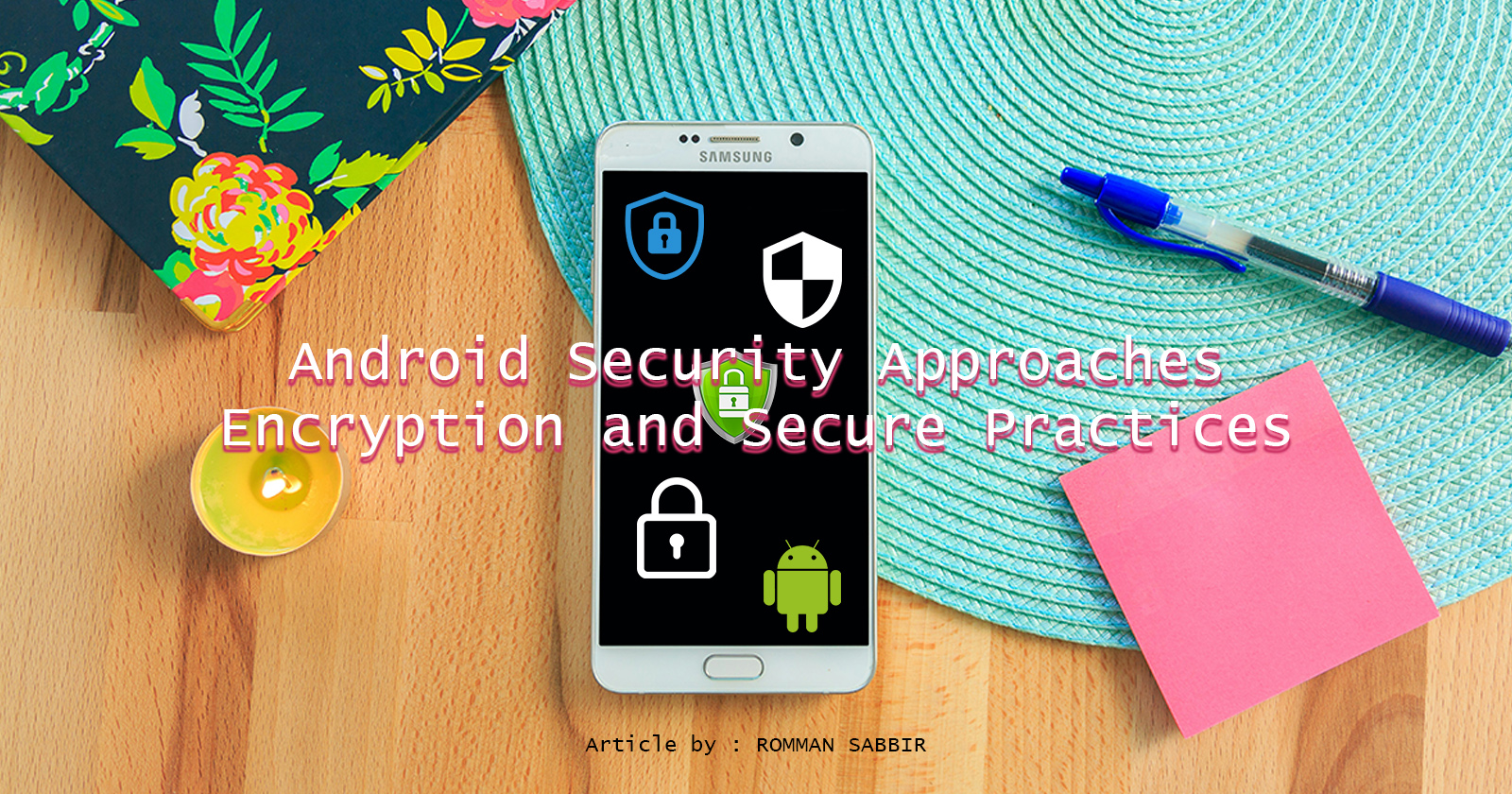
Application security is essential for preventing unauthorized access, reverse engineering, and tampering with your Android apps. There are several strategies and tools that developers can employ to protect their applications from these threats. In this article, we will explore ProGuard/R8 for code obfuscation, App Signing for validating authenticity, the SafetyNet Integrity Check to detect compromised devices, and Google Play App Signing for managing signing keys. Each section provides detailed explanations, real-life examples, and Kotlin code snippets to help you implement these security practices.
ProGuard/R8: Code Obfuscation and Shrinking
ProGuard and R8 are tools that help reduce the size of your APK and obscure your code to prevent reverse engineering. These tools minify, optimize, and obfuscate the code, making it harder for attackers to analyze the logic of your app.
ProGuard: Originally, ProGuard was used to shrink and obfuscate the app's bytecode. It also removes unused code and resources, optimizing the app size.
R8: As of Android Gradle Plugin 3.4.0, R8 replaces ProGuard by default. It combines shrinking, obfuscation, and optimization in a single step, with better performance than ProGuard.
Use Case: Preventing Reverse Engineering
When your Android app contains sensitive code, such as proprietary algorithms, encrypting logic, or API keys, it is crucial to obscure that code to prevent attackers from reverse-engineering it.
Kotlin Example: Configuring R8/ProGuard
- Enable R8 in your project (this is the default in newer versions of Android Studio). You can customize your R8 rules by editing the
proguard-rules.pro
file.
# Preserve class names for debugging purposes
-keep class com.example.myapp.** { *; }
# Remove logging
-assumenosideeffects class android.util.Log {
public static *** d(...);
public static *** v(...);
}
- To enable code shrinking and obfuscation, add the following to your
build.gradle
file:
android {
buildTypes {
release {
minifyEnabled true // Enable shrinking and obfuscation
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
Real-Life Example: Banking Apps
Banking apps often contain sensitive logic for handling transactions, encryption algorithms, and user data. Obfuscating the code using ProGuard or R8 makes it much harder for attackers to decompile the APK and understand how these processes work.
App Signing: Validating the Authenticity of Your App
App Signing is the process of using a cryptographic key to sign your APK or AAB (Android App Bundle) before distributing it. Signing the app ensures its authenticity and integrity, as the signature is used by the system to verify that the APK has not been tampered with.
Use Case: Securing App Distribution
When you release an Android app, signing it is required to publish it on the Google Play Store. This signature verifies the source of the APK, ensuring users are downloading a legitimate version of the app. If the app isn’t signed, the Android operating system won’t install it.
Steps for App Signing in Android Studio
Generate a Signing Key: In Android Studio, go to Build > Generate Signed Bundle / APK and follow the steps to create a keystore file and generate a private key.
Configure App Signing in
build.gradle
:
android {
signingConfigs {
release {
storeFile file("keystore.jks")
storePassword "your-store-password"
keyAlias "your-key-alias"
keyPassword "your-key-password"
}
}
buildTypes {
release {
signingConfig signingConfigs.release
}
}
}
- Sign and Publish: After signing your APK, you can publish it to Google Play or distribute it directly. The system will verify the signature upon installation.
Real-Life Example: App Stores
Every Android app published to the Google Play Store is required to be signed. This prevents malicious actors from modifying apps and re-distributing them without the developer’s consent.
Integrity Check (SafetyNet API): Detecting Rooted or Compromised Devices
Google’s SafetyNet API is a security feature that helps detect whether the device running your app has been compromised, such as through rooting or other tampering methods. SafetyNet provides an attestation API, which you can use to determine whether the device passes security checks. This is especially important for apps dealing with sensitive data, like financial apps or secure messaging apps.
Use Case: Blocking Access on Compromised Devices
You might want to block or limit access to certain features if the device is rooted or compromised. For example, a banking app might not allow a user to initiate transactions if the device is not secure.
Kotlin Example: Implementing SafetyNet Attestation
- Add the necessary dependencies to your
build.gradle
file:
implementation 'com.google.android.gms:play-services-safetynet:18.0.1'
- Use the SafetyNet API to check the device’s integrity:
import com.google.android.gms.safetynet.SafetyNet
import com.google.android.gms.tasks.OnSuccessListener
import com.google.android.gms.tasks.OnFailureListener
fun checkDeviceIntegrity() {
SafetyNet.getClient(this).attest(nonce, getApiKey())
.addOnSuccessListener(OnSuccessListener { response ->
// Process the attestation result
val jwtToken = response.jwsResult
// Verify the JWT response on your server to determine the integrity of the device
})
.addOnFailureListener(OnFailureListener { e ->
// Handle error
})
}
In this example, nonce is a random value generated to ensure that the attestation request is fresh, and getApiKey() returns your SafetyNet API key. The response contains a JWT token that you can verify on your server to determine the integrity of the device.
Real-Life Example: Financial Services
A financial app can use the SafetyNet attestation API to block access to payment features if the device fails the integrity check, ensuring that users cannot perform transactions from compromised devices.
Google Play App Signing: Secure Key Management
Google Play App Signing is a service provided by Google Play that helps manage and secure your app signing keys. Instead of managing your signing keys manually, you can opt into Google Play App Signing, where Google securely stores your signing key and signs your APKs on your behalf when you upload them to the Play Store.
Use Case: Protecting Your Signing Key
Managing your own app signing keys can be risky, especially if they are lost or compromised. With Google Play App Signing, Google manages your signing keys securely, ensuring that even if you lose your local copy of the key, your app can still be updated and maintained.
Steps to Enable Google Play App Signing
When publishing your app to Google Play for the first time, you will be given the option to enroll in Google Play App Signing.
If you opt in, you will upload an unsigned APK or AAB, and Google will take care of signing it with the stored key.
Once enrolled, you don’t need to worry about key storage, and Google provides a way to securely manage keys for updates.
Real-Life Example: App Development Companies
Many app development companies with large app portfolios rely on Google Play App Signing to handle key management securely, especially when multiple teams are working on the same app. This also ensures that the signing key remains safe even if there are changes in the development team or processes.
Conclusion
Securing your Android applications is essential to protect users’ data, ensure the integrity of your app, and prevent tampering. ProGuard/R8 helps to obfuscate your code, making it harder for attackers to reverse-engineer your app. App Signing guarantees the authenticity of your app, while SafetyNet Integrity Check detects if the app is running on a compromised device. Finally, Google Play App Signing simplifies key management by securely storing and managing signing keys.
By implementing these techniques, you can enhance the security of your app and provide a safe experience for your users, ensuring that their data and your app remain protected from malicious actors.
That’s it today. Happy coding…
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
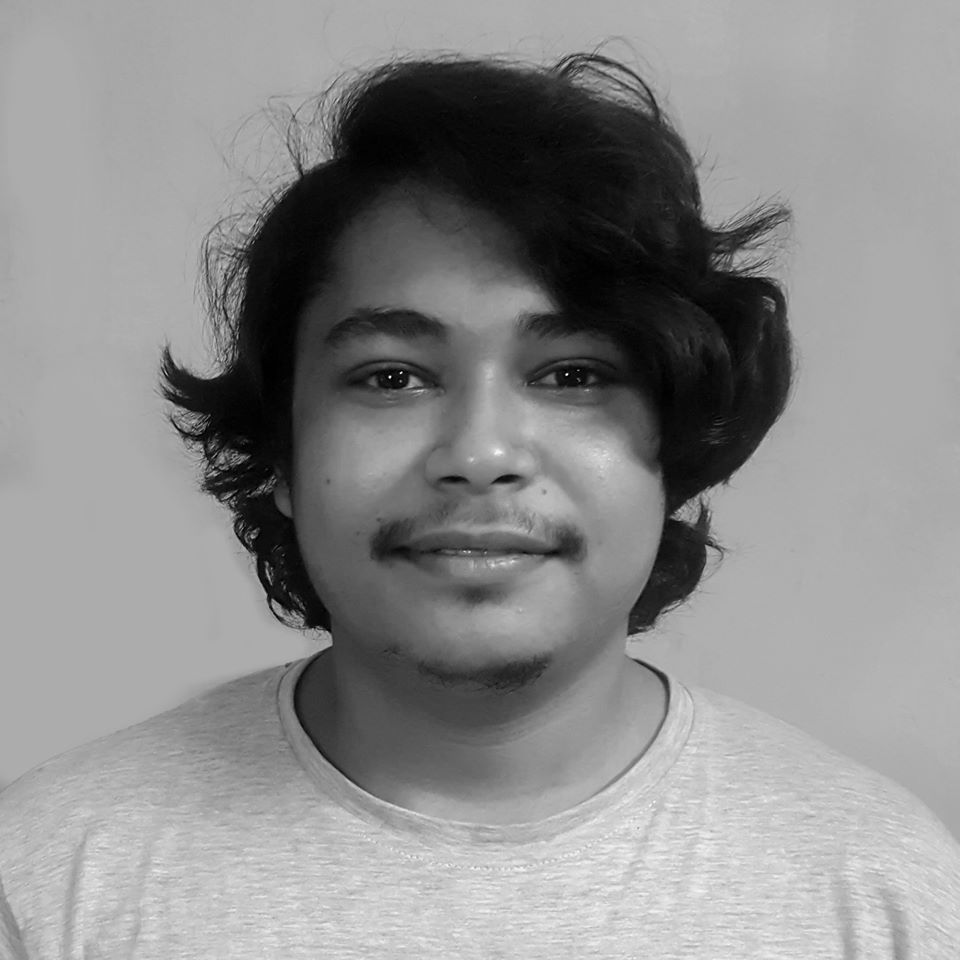
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.