Best Practices For Writing Clean Java Code
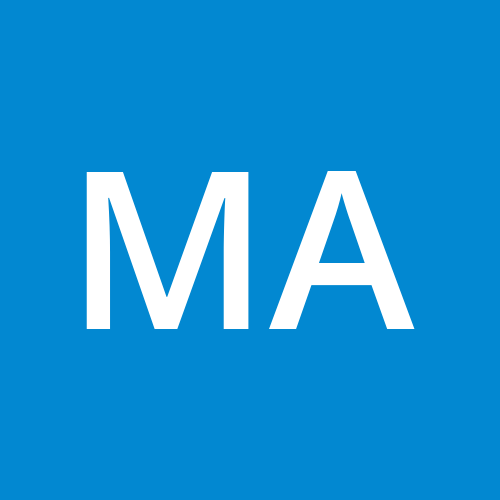
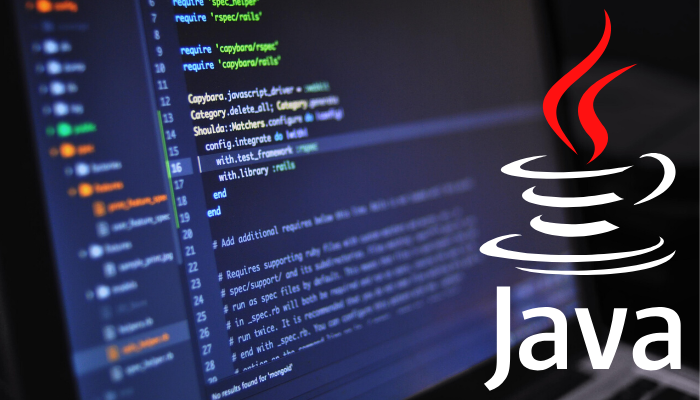
Writing clean, readable, and efficient code is essential for Java developers, enhancing maintainability and making it easier for future developers to understand and work with. Following best practices not only improve code quality but also demonstrates professionalism. If you’re looking to improve your Java skills, consider enrolling in a Java Online Course to dive deeper into these techniques.
Best Practices
1. Use Meaningful Names
One of the key aspects of clean code is choosing names that represent variables, methods, and classes. Meaningful names make it easier to understand the purpose of each component at a glance.
Example:
Naming Element | Bad Example | Good Example |
Variable | x | employee count |
Method | calc() | calculateTotalSalary() |
Class | Mgr | EmployeeManager |
2. Follow Consistent Formatting and Indentation
Uniform indentation and spacing improve code readability. Consistent formatting tools like Checkstyle or Google Java Format can enforce a standard code format automatically.
For developers looking to refine their coding practices, the Java Online Course provides training on best practices, including consistent formatting and coding standards in Java, ensuring that your code remains clean and professional.
3. Limit Class and Method Length
By breaking down code into smaller, single-responsibility classes and methods, you make it more manageable.
For those interested in mastering this skill, the Java Institute in Noida offers comprehensive training, focusing on effective Java development practices, including structuring code for maintainability and performance.
Example:
4. Avoid Hardcoding Values
Use constants or configuration files for values that might change, which makes maintenance easier.
For those looking to deepen their understanding of clean coding practices, Java Institute in Delhi provides training on best practices like avoiding hardcoded values, along with other essential Java development skills.
5. Optimize Error Handling and Logging
Error handling is crucial in Java. Use try-catch blocks appropriately and avoid catching generic exceptions. Additionally, implement logging to aid debugging.
Exception Type | Severity Level | Action |
NullPointer | High | Throw custom exception |
IOExceptions | Medium | Log and retry |
SQLExceptions | Medium | Log and handle gracefully |
6. Write Unit Tests
Testing is essential for reliable code. Unit tests help identify bugs early and ensure that code changes don’t introduce new issues. Using frameworks like JUnit makes unit testing efficient and manageable.
For those interested in mastering testing skills, Java Institute in Noida offers in-depth training in Java development, including best practices for writing and executing unit tests using JUnit and other testing tools.
7. Use Proper Code Documentation
Documenting complex code segments can help others understand the logic. However, avoid unnecessary comments, as self-explanatory code is often preferable.
Documentation Element | Description | Usage Example |
Inline Comments | Describe code logic | // Calculate yearly bonus |
JavaDoc | Class/method explanation | /** Updates employee salary */ |
8. Leverage Design Patterns
Using established design patterns like Singleton, Factory, and Observer makes your code modular, testable, and maintainable.
Recommended Patterns for Different Scenarios
Pattern | Usage Scenario | Benefits |
Singleton | Global instance (e.g., config) | Controlled instance creation |
Factory | Object creation control | Flexible instantiation |
Observer | Event-driven applications | Decouples subject and observer |
Conclusion
Incorporating these best practices into your coding routine will lead to cleaner, more professional Java code. Following these guidelines also prepares you for advanced learning opportunities, like enrolling in an institute in Noida or Delhi, where you can further refine your skills under expert guidance.
Subscribe to my newsletter
Read articles from Manoj Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
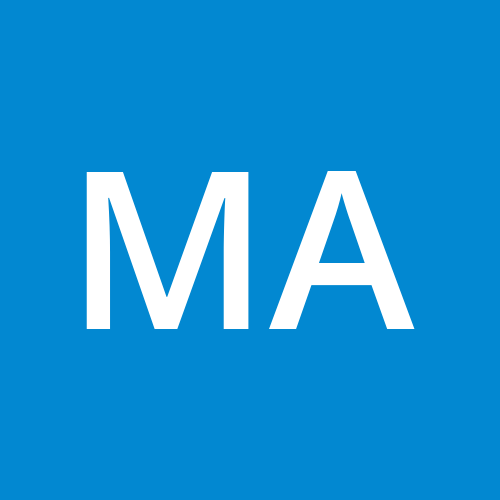