A Beginner's Guide to Shell Scripting in DevOps

Table of contents
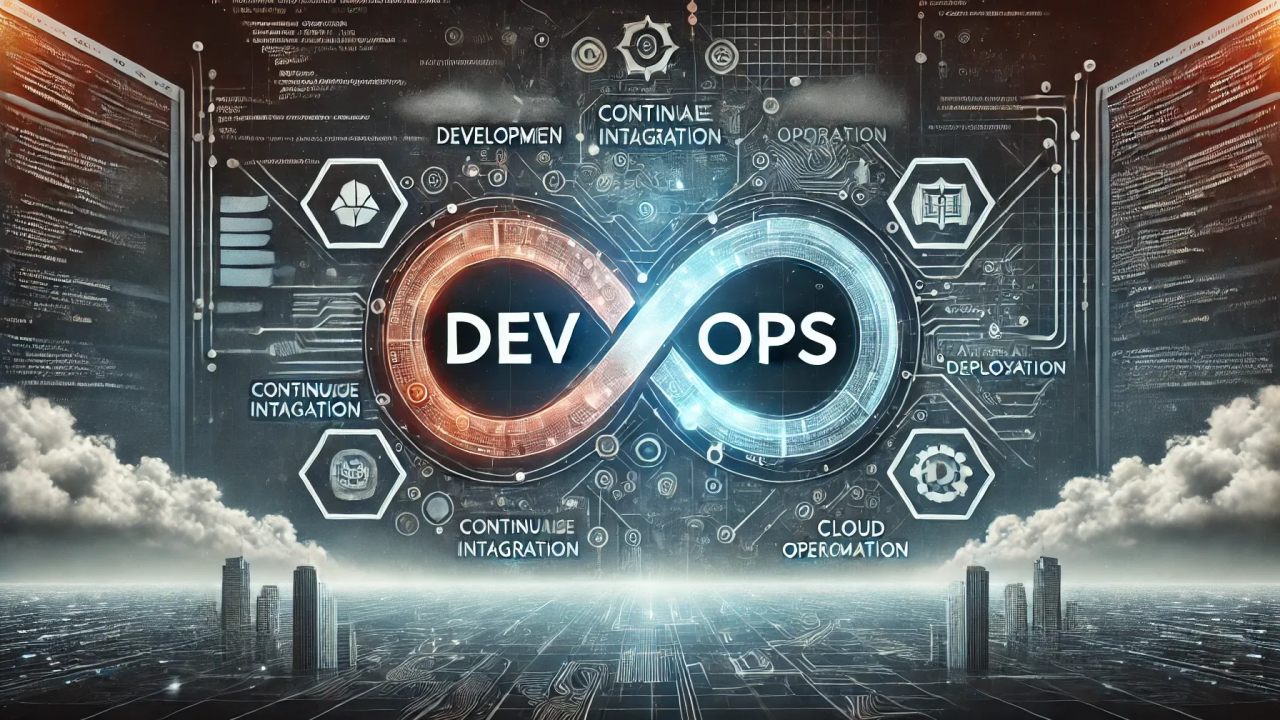
Shell Scripting for DevOps - Automate Your Tasks
Lecture 8 in the DevOps journey dives deep into the essential topic of Shell Scripting. In this blog, we’ll cover what shell scripting is, its purpose, basic commands, script creation, automation using cron jobs, and more. Shell scripting is a crucial skill for DevOps engineers, as it allows for task automation and interaction with the OS kernel.
What is Shell Scripting?
Definition: Shell scripting is essentially a file containing a series of shell commands, which can be executed sequentially.
Purpose: It’s used to automate repetitive tasks, which is valuable in DevOps for saving time and reducing human error.
Shell: A shell is a command-line interface that interacts with the OS kernel. There are multiple types of shells, including
bash
(Bourne Again Shell) andsh
.File Extension: Shell script files typically have the
.sh
extension.Shebang:
#!/bin/bash
is a shebang that specifies the script is for the bash shell.
Creating and Running Shell Scripts
To create a shell script, follow these steps:
Create Script: Use
vim
hello.sh
to create a file.Make Executable: Use
chmod 774
hello.sh
to make it executable.
# Example of a simple shell script
#!/bin/bash
# This is a comment
echo "Hello, World!"
Comments in Shell Scripts
Single-line Comment: Use
#
at the start of the line.Multi-line Comment:
- Use the syntax
<<COMMENT
andCOMMENT
to enclose multiple lines.
- Use the syntax
<<COMMENT
This is a multi-line comment example.
COMMENT
Assignment: Creating a Movie Dialog Script
Here’s a simple script simulating a dialog from the movie Salaar:
#!/bin/bash
# This is Salaar movie dialogue
<<Salaar
Vardha and Deva ki dost
Salaar
echo "Deva: Tu jab bhi bulayega mai aaunga"
echo "Vardha: Vaada kar!"
echo "Deva: Ye vaada hai mera."
echo "Vardha: Deva abb chal sath mai"
echo "Deva: Chal bhai."
echo "Vardha: Ruk jara date dekhlu tuje le jane ka: "
date
Scripting Concepts
Variables and Constants
Print Output:
echo
is used to print anything.User Input:
read
gathers input from the user, andread -p
adds a prompt.#!/bin/bash read -p "Enter your name: " name echo "My name is $name."
Installing Packages via Script
To automate package installation, here’s a script to install NGINX:
#!/bin/bash
echo "***************INSTALLING NGINX**************"
sudo apt update
sudo apt install nginx -y
sudo systemctl start nginx
sudo systemctl enable nginx
echo "**************INSTALLED NGINX******************"
Installing Packages Using a Script
In this section, I learned how to create a script to install any package passed as an argument. Here's the shell script to install any package using a command-line argument:
Script: install_
package.sh
#!/bin/bash
<< note
This script will install any package passed as an argument.
./install_package.sh <arg>
$1 is used to get the first argument (<arg>) which is passed by running the above command to install any package.
note
echo "**************INSTALLING $1**************"
# Updating system
sudo apt update
# Installing the package
sudo apt install $1 -y
# Start and enable the service
sudo systemctl start $1
sudo systemctl enable $1
echo "***************INSTALLED $1***************"
Steps to Execute:
Create the script using
vim install_
package.sh
.Change the file permissions:
chmod 774 install_
package.sh
.Run the script with the desired package as an argument. For example, to install SSH, run:
./install_
package.sh
ssh
.
Output Image:
Creating a Backup of Scripts
A backup script example:
#!/bin/bash
<< note
This scripts takes backup of any destination path given in argument
./backup.sh /home/ubuntu/scripts
note
timestamp=$(date '+%Y-%m-%d_%H-%M-%S')
backup_dir="${timestamp}_backup.zip"
zip -r $backup_dir $1
echo "Backup Completed!"
Execute:
./
backup.sh
/home/ubuntu/scripts
Result: This compresses all scripts in
/home/ubuntu/scripts
into a.zip
file with a timestamp.
Automating Execution with Cron Jobs
Cron is a tool that automates tasks on a schedule.
Editing Cron Jobs: Use
crontab -e
to access and edit the cron file.Schedule Example: The following runs a backup script every minute.
*/1 * * * * bash /home/ubuntu/scripts/backup.sh /home/ubuntu/scripts
Updated
backup.sh
Script: This updated script stores backups in abackups
directory with a timestamp.#!/bin/bash << note This scripts takes backup of any destination path given in argument ./backup.sh /home/ubuntu/scripts note function create_backup { timestamp=$(date '+%Y-%m-%d_%H-%M-%S') backup_dir="/home/ubuntu/backups/${timestamp}_backup.zip" zip -r $backup_dir $1 echo "Backup Completed!" } create_backup $1
To Stop Cron: Comment out or delete the scheduled job in
crontab
.
Conclusion and Next Steps
This blog covered foundational shell scripting concepts, from creating scripts to automating backups using cron jobs. Shell scripting is a powerful tool in DevOps that enables engineers to automate complex tasks efficiently. In the upcoming blog, we’ll explore Backup Rotation—a critical practice for managing space and organizing backups systematically.
Stay tuned for the next blog on Backup with Rotation, where we’ll delve into creating a robust script to handle backups while managing storage space.
Subscribe to my newsletter
Read articles from Amitabh soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amitabh soni
Amitabh soni
DevOps Enthusiast | Passionate Learner in Tech | BSc IT Student I’m a second-year BSc IT student with a deep love for technology and an ambitious goal: to become a DevOps expert. Currently diving into the world of automation, cloud services, and version control, I’m excited to learn and grow in this dynamic field. As I expand my knowledge, I’m eager to connect with like-minded professionals and explore opportunities to apply what I’m learning in real-world projects. Let’s connect and see how we can innovate together!