Day 4 of 90DaysOfDevOps : Basic Linux Shell Scripting for DevOps Engineers.

Table of contents
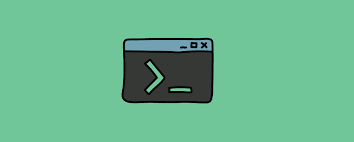
What is Kernel?
The Kernel is the core part of an operating system, acting as a bridge between the hardware and software. It manages system resources and allows programs to interact with the hardware indirectly. The kernel handles tasks like memory management, process scheduling, and device communication. In Linux, the kernel is central to system operation and security, managing tasks that make user commands effective.
What is Shell?
The Shell is an interface that allows users to interact with the operating system. In Linux, it interprets commands and executes them. Common shells include Bash, Zsh, and Sh. Each shell has unique features, but all provide a command-line interface where users can execute commands and scripts.
What is Linux Shell Scripting?
Linux Shell Scripting is writing scripts or commands in a shell (such as Bash or Sh) to automate tasks. Scripts can manage files, automate processes, monitor systems, and configure environments. Shell scripting is widely used in DevOps to streamline deployments, monitor resources, automate repetitive tasks, and manage configurations.
Task 1: What Shell Scripting Means for DevOps
In DevOps, shell scripting is a crucial skill because it enables the automation of manual and repetitive tasks, saving time and reducing errors. For example, with shell scripting, you can:
Automate Deployments: Scripts can package and deploy applications to various environments, ensuring consistent and fast delivery.
Monitor Systems: Write scripts to check server status, disk space, or memory usage and generate alerts.
Configure Environments: Automate configuration of virtual machines or containers with necessary dependencies.
Task 2: What is #!/bin/bash
? Can We Write #!/bin/sh
as Well?
The line #!/bin/bash
at the top of a script tells the system to use Bash (Bourne Again Shell) to interpret the script. This is called a shebang. You can also use #!/bin/sh
, which points to the Bourne Shell. However, bash
is generally more powerful with added features, whereas sh
is more compatible across different Unix-like systems. So, the choice depends on the script requirements.
Task 3: Shell Script to Print a Message
Here’s a simple shell script to print your challenge commitment message.
#!/bin/bash
echo "I will complete #90DaysOfDevOps challenge."
To run this script, save it as challenge.sh
, make it executable with chmod +x
challenge.sh
, and execute it with ./
challenge.sh
.
Task 4: Shell Script to Take User Input and Arguments
Here’s a script that captures user input and arguments, then prints them.
#!/bin/bash
# Taking user input
echo "Enter your name:"
read name
# Taking arguments
arg1=$1
arg2=$2
# Displaying variables
echo "Name: $name"
echo "Argument 1: $arg1"
echo "Argument 2: $arg2"
To run this, save it as input.sh
, make it executable, and run with arguments:
./input.sh argValue1 argValue2
Example of an If-Else Statement in Shell Scripting
Below is a script with an If-Else statement comparing two numbers.
#!/bin/bash
num1=10
num2=20
if [ $num1 -gt $num2 ]; then
echo "$num1 is greater than $num2"
else
echo "$num1 is not greater than $num2"
fi
In this example, the script checks if num1
is greater than num2
. Since num1
is not greater, the script outputs: 10 is not greater than 20
.
This outline and explanation will provide a thorough, practical introduction to shell scripting in DevOps. Let me know if you'd like to dive deeper into any area!
Subscribe to my newsletter
Read articles from Pooja Naitam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pooja Naitam
Pooja Naitam
👋 Hello! I'm Pooja Naitam, a passionate DevOps fresher with a solid foundation in the field. I hold the AWS Certified Cloud Practitioner (CCP) certification, and I'm eager to apply my knowledge to real-world projects while continuously learning cutting-edge technologies. Let's connect and grow together in the exciting world of DevOps!