Mastering the Art of Async: Let’s Get Moving!
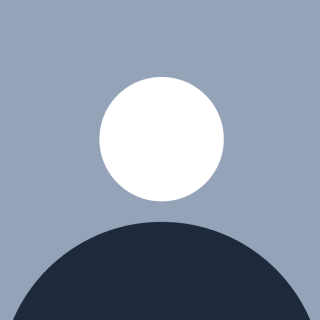
Table of contents
- Why Go Async?
- Synchronous vs. Asynchronous – The Race Track
- Async Functions – Letting the Race Continue
- Promises – The Pit Crew of Async
- Flowchart: The Promise Lifecycle
- Async Functions and Promises
- Introduction to Async/Await – The Smoothest Race Experience
- Using async and await – A New Way to Race
- Handling Errors with Try/Catch
- Practical Example – Creating Multiple Async Pit Stops
- Flowchart: Async/Await Flow
- Challenges: Put Your Async Skills to the Test!
- Mastering Async JavaScript
- Let’s dive into the DOM!
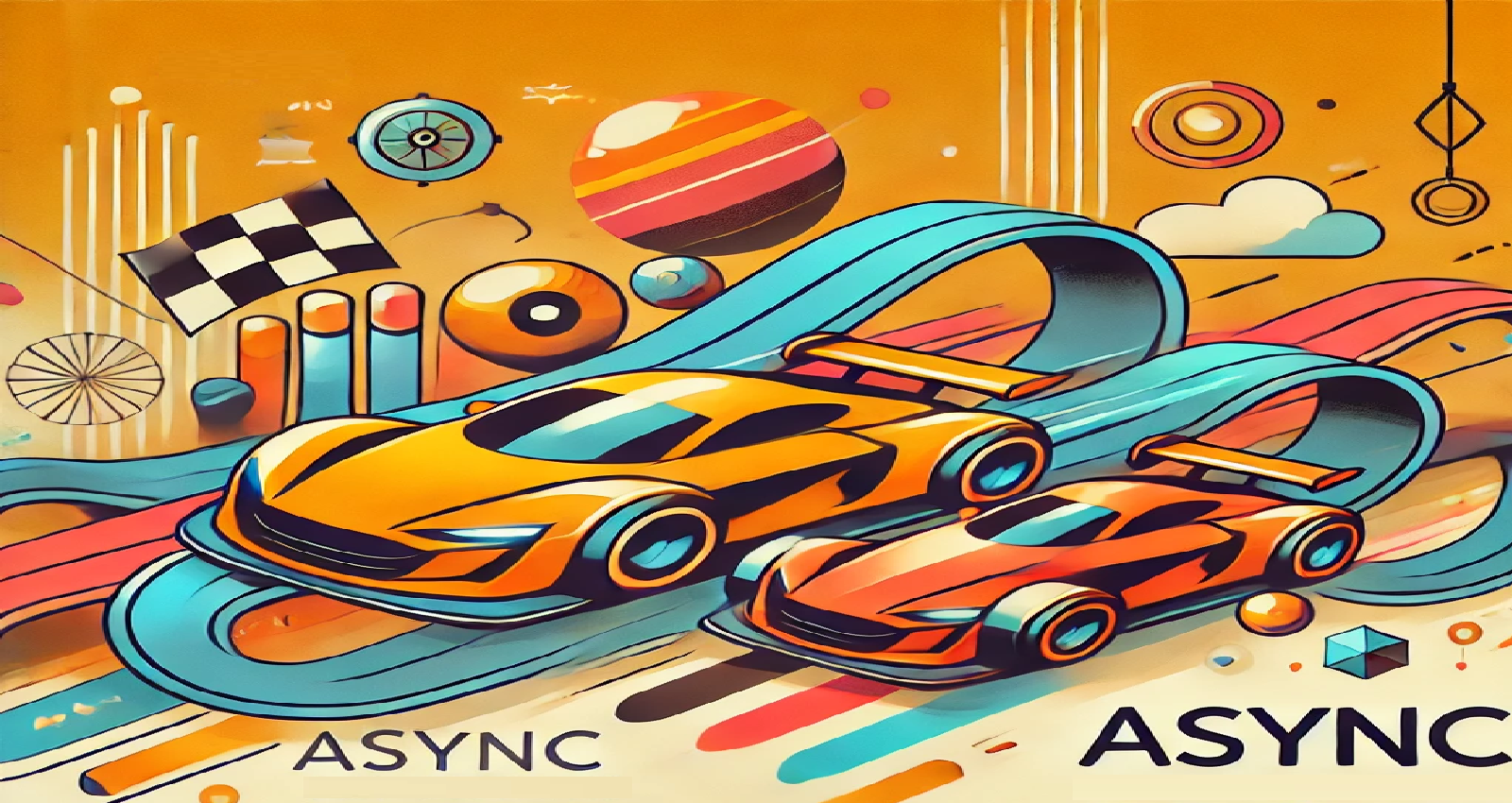
Why Go Async?
Imagine you’re racing a car, but every time your car needs a pit stop, the entire race pauses until it’s finished. This wouldn’t make sense! In a race, every car runs independently, so one car’s pit stop doesn’t stop others. Asynchronous programming works the same way. It lets tasks run without stopping everything else.
In JavaScript, asynchronous programming is the way we can handle multiple things at once, like fetching data from a server while still allowing other code to execute. This is important for keeping applications fast and responsive, especially when dealing with tasks that take time.
Synchronous vs. Asynchronous – The Race Track
Synchronous programming is when tasks are handled one after the other, like a single car driving through each stage of a race without passing. Each task finishes before the next one starts.
Example of synchronous code:
console.log("Start");
console.log("Racing...");
console.log("Finish");
Output:
Start
Racing...
Finish
Here, each line runs one after another in order. But imagine if “Racing…” involved a big, time-consuming task, like waiting for a pit stop to finish. The entire sequence would be blocked until it was done, slowing everything down.
Flowchart: Sync vs. Async Execution
Here’s a visual to show the difference:
[Synchronous]
+-----------+ +----------+ +----------+
| Operation | --> | Operation | --> | Operation |
+-----------+ +----------+ +----------+
[Asynchronous]
+-----------+ +----------+
| Operation | ---> | Operation |
+-----------+ +----------+
\ /
\ +----------+
------> | Operation |
+----------+
Async Functions – Letting the Race Continue
Async functions allow us to tell JavaScript, “Hey, let this task work in the background. Don’t wait around for it.” By adding the async
keyword to a function, we can run tasks independently and keep the race going!
Here’s an example of an async function:
async function raceStart() {
console.log("On your marks...");
return "Go!";
}
raceStart().then((message) => console.log(message));
Output:
On your marks...
Go!
Explanation:
Adding
async
toraceStart
tells JavaScript that this function will handle an asynchronous task.return "Go!"
automatically wraps the result in a Promise (more on that below).then() handles the result when the task is complete.
Promises – The Pit Crew of Async
Imagine your car needs a pit stop. You trust your pit crew to handle it while you keep racing. Sometimes the stop goes well, and you’re back on track. Other times, there’s a delay.
A Promise is JavaScript’s way of managing tasks that might not complete immediately. Think of it like a signal from your pit crew:
Pending: The pit stop is in progress.
Fulfilled: The stop is done, and you’re back in the race.
Rejected: Something went wrong, and you’re delayed.
Code Snippet: A Basic Promise
Let’s see a basic Promise and what each part does.
let pitStop = new Promise((resolve, reject) => {
let pitSuccess = Math.random() > 0.5; // Randomly decide if pit stop is successful
if (pitSuccess) {
resolve("Pit stop successful! Back on track!"); // Task is done successfully
} else {
reject("Pit stop failed. You’re delayed!"); // Task encountered an error
}
});
// Using the Promise
pitStop
.then((message) => console.log(message)) // Runs if resolve is called
.catch((error) => console.log(error)); // Runs if reject is called
Explanation:
resolve: Think of this as the pit crew saying, “The car’s ready! Go!”
reject: This is like the crew saying, “There’s a problem; you’re delayed.”
Example Output: Depending on pitSuccess
, you might see:
Pit stop successful! Back on track!
or
Pit stop failed. You’re delayed!
Why Promises Matter
Promises let JavaScript handle multiple tasks without stopping everything. It’s like letting the pit crew work while the rest of the race continues. If the stop goes smoothly, you keep racing; if not, there’s a plan to handle it.
Chaining with .then()
and .catch()
We can also use .then()
and .catch()
to chain tasks together, handling the success or failure of each step.
pitStop
.then((message) => {
console.log(message);
return "Victory lap!";
})
.then((celebration) => console.log(celebration))
.catch((error) => console.log(error));
If everything succeeds, the car goes on a “victory lap.” If the promise fails at any step, it stops and runs .catch()
.
Flowchart: The Promise Lifecycle
Here’s a simple flowchart showing how a Promise works from start to finish:
+-------------+
| Pending |
+-------------+
|
+-----------|-----------+
| |
+-----------+ +-----------+
| Fulfilled | | Rejected |
+-----------+ +-----------+
| |
+---------------+ +---------------+
| .then() | | .catch() |
+---------------+ +---------------+
With Promises, JavaScript can handle asynchronous tasks while keeping your code organized and clear.
Async Functions and Promises
In this first part, we introduced async functions and Promises using the pit crew and race car analogy. By understanding these tools, you’re better equipped to write responsive, efficient code that doesn’t block everything else.
In Part 2, we’ll simplify asynchronous programming further with async/await, making async code even easier to understand and manage.
Introduction to Async/Await – The Smoothest Race Experience
Promises are a powerful tool for managing asynchronous code, but sometimes, .then()
and .catch()
chains can get long and tricky to read. This is where async/await steps in, letting us write asynchronous code that looks like synchronous code.
Imagine a high-tech race car that can handle every pit stop without needing constant updates from the driver. Async/await gives us this smooth, efficient experience.
Using async
and await
– A New Way to Race
When we use async/await, we don’t need to create long chains of .then()
calls. Instead, await
pauses the code within an async function until the Promise is resolved. Think of await
as the driver knowing they’re in the pit stop without needing to be told when it’s done – they simply wait until everything is ready.
Example: Basic Async/Await
Let’s create a simple async function that waits for a pit stop using async/await.
async function raceDay() {
console.log("The race begins...");
let pitStop = new Promise((resolve) => {
setTimeout(() => resolve("Pit stop completed!"), 2000);
});
let result = await pitStop; // Pauses here until the promise resolves
console.log(result);
console.log("And we're back on track!");
}
raceDay();
Output:
The race begins...
(pause for 2 seconds)
Pit stop completed!
And we're back on track!
Explanation:
await pitStop: This tells JavaScript to wait here until
pitStop
is resolved.Async functions can run smoother because
await
allows us to write code without complex.then()
chains.
Handling Errors with Try/Catch
In async functions, errors are caught with try/catch blocks, making it easier to manage failures. Just like a driver has a backup plan if something goes wrong in the pit stop, we can use try/catch
to handle errors.
async function riskyPitStop() {
try {
let pitStop = new Promise((resolve, reject) => {
let success = Math.random() > 0.5;
success ? resolve("Pit stop successful!") : reject("Pit stop failed!");
});
let result = await pitStop;
console.log(result);
} catch (error) {
console.log(error); // Handles any errors if the pit stop fails
}
}
riskyPitStop();
Explanation:
try: Starts the block that will attempt to execute the async function.
catch: If an error occurs, it’s caught and handled here, keeping the program from crashing.
Practical Example – Creating Multiple Async Pit Stops
Now that we understand async functions and error handling, let’s build a scenario with multiple pit stops. In this example, we’ll create three pit stops, each taking a random time to complete.
async function completeRace() {
console.log("Race starts...");
async function pitStop(number) {
return new Promise((resolve) => {
let time = Math.floor(Math.random() * 3000) + 1000;
setTimeout(() => resolve(`Pit stop ${number} completed in ${time}ms`), time);
});
}
for (let i = 1; i <= 3; i++) {
let result = await pitStop(i);
console.log(result);
}
console.log("Race finished!");
}
completeRace();
Explanation:
await pitStop(i);
: Waits for each pit stop to complete before starting the next.Loop with await: By awaiting each pit stop in a loop, we maintain control of the order, ensuring each step is completed before the next begins.
Output Example:
Race starts...
Pit stop 1 completed in 1200ms
Pit stop 2 completed in 2500ms
Pit stop 3 completed in 1900ms
Race finished!
Flowchart: Async/Await Flow
[ Start Race ]
|
[ Async Function ]
|
+----------------+ +----------------+ +----------------+
| await Pit Stop | ----> | await Pit Stop | ----> | await Pit Stop |
+----------------+ +----------------+ +----------------+
|
[ Race Finished ]
Each pit stop is awaited, allowing our function to proceed only after each Promise is resolved.
Challenges: Put Your Async Skills to the Test!
Here are three challenges to help solidify your understanding of async/await:
Challenge 1: Track Maintenance
Simulate a maintenance check on the track after each lap, ensuring each check completes before moving on to the next lap.
Requirements:
Each maintenance check should take a random amount of time between 1-3 seconds.
After each check, print the time it took, e.g., “Lap 1 check completed in X ms.”
Solution:
async function trackMaintenance() {
for (let lap = 1; lap <= 3; lap++) {
const time = Math.floor(Math.random() * 2000) + 1000; // 1-3 seconds
await new Promise((resolve) => setTimeout(resolve, time)); // Simulate maintenance time
console.log(`Lap ${lap} check completed in ${time} ms`);
}
console.log("All laps checked!");
}
trackMaintenance();
Explanation:
await new Promise
simulates the maintenance time.Each lap check waits to complete before the next one begins, printing the duration of each lap check.
Challenge 2: Team Pit Stops
In this challenge, the driver must go through a series of pit stops. If any stop fails, retry it until successful.
Requirements:
Each pit stop should take between 1-2 seconds.
If a pit stop fails (random chance), log “Pit stop failed. Retrying…” and try again.
Complete three successful pit stops for a successful race.
Solution:
async function teamPitStops() {
for (let stop = 1; stop <= 3; stop++) {
let success = false;
while (!success) {
try {
await pitStop(stop);
success = true; // Stop succeeded
} catch (error) {
console.log(`Pit stop ${stop} failed. Retrying...`);
}
}
}
console.log("All pit stops completed!");
}
async function pitStop(number) {
const time = Math.floor(Math.random() * 1000) + 1000;
const success = Math.random() > 0.5; // 50% success chance
return new Promise((resolve, reject) => {
setTimeout(() => {
if (success) {
console.log(`Pit stop ${number} completed in ${time} ms`);
resolve();
} else {
reject();
}
}, time);
});
}
teamPitStops();
Explanation:
The
while (!success)
loop retries each pit stop until it completes successfully.Each pit stop has a 50% chance of success and retries on failure.
Challenge 3: Async Race Timer
Create a function that tracks the time taken for each async task and calculates the total duration.
Requirements:
Run a series of async tasks, each with a random duration.
Log the duration of each task after completion.
Print the total time taken for all tasks.
Solution:
async function asyncRaceTimer() {
let totalTime = 0;
for (let task = 1; task <= 3; task++) {
const time = Math.floor(Math.random() * 2000) + 1000; // 1-3 seconds
await new Promise((resolve) => setTimeout(resolve, time));
console.log(`Task ${task} completed in ${time} ms`);
totalTime += time;
}
console.log(`Total time: ${totalTime} ms`);
}
asyncRaceTimer();
Explanation:
Each task duration is randomized, and the task pauses until it completes.
The time for each task is added to
totalTime
, which is printed at the end.
Mastering Async JavaScript
Async/await simplifies asynchronous programming, making our code cleaner and easier to manage. With async
functions, you can control async code with the elegance of synchronous code, improving readability without sacrificing performance.
By mastering async programming, you’re now prepared to create fast, responsive applications that handle tasks smoothly, even in complex workflows.
Let’s dive into the DOM!
Now that you've mastered the power of asynchronous programming, it's time to step into the visual world of the DOM (Document Object Model)! In our next article, we’ll explore how JavaScript interacts with HTML elements, bringing your web pages to life. Get ready to control, manipulate, and transform elements on the page, creating dynamic and interactive experiences. Let’s dive into the DOM!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by