How I integrated Clerk and Threads API: Part 1

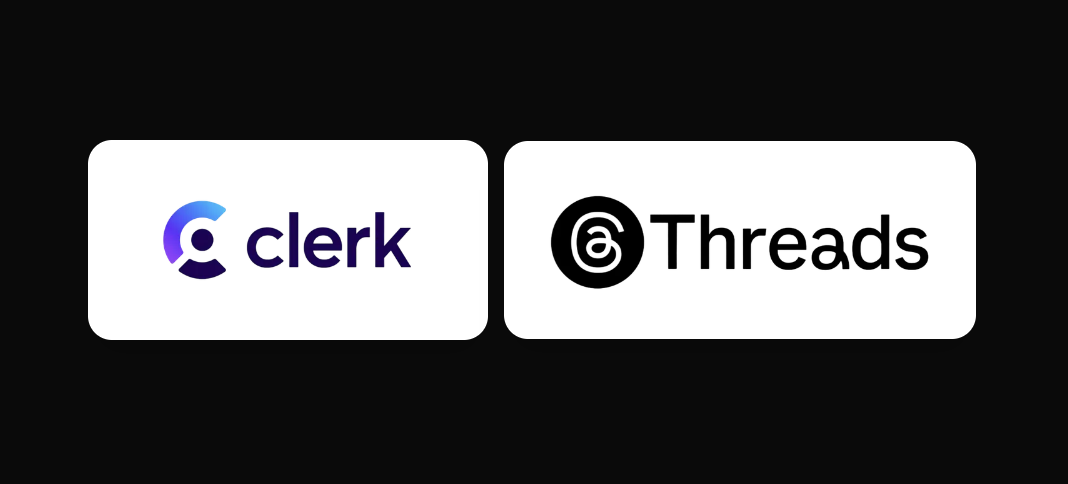
✨ Introduction
Lately I’ve been working on a side-project called DualPosts. It's a tool that lets users cross-post between the X and Threads platforms. The idea came when I found myself constantly copy-pasting the same thing from one site to another, so a tool like this would be handy.
I decided to use Clerk to handle authentication because everyone was talking good about it and I hadn’t used it yet. Also it has a lot of built-in SSO providers, so why not giving it a try?
X integration was easy as it was one of the built-in providers. BUT Threads was not. Luckily Clerk also allows you to configure a custom provider, so let’s go!
I want to show you how I did it because I ran into some technical issues, and I think they're interesting for any developer trying something similar.
👣 Steps
1️⃣ Create the Meta app
Ok so the first thing I needed according to Threads API docs is “A Meta app created with the Threads use case.”
This is pretty straightforward, just go https://developers.facebook.com/apps and create a new app. Follow each step and you’re done.
Here by default the threads_basic
scope is enabled; but for my use case I also needed to add the threads_content_publish
scope since I wanted to use the API to also publish content on Threads.
Go to the settings page and note down the Threads App ID and the Threads App Secret values. You’ll need them in the next step
Attention! 🚧
2️⃣ Add custom SSO connection in Clerk
Now from the Clerk dashboard we’ll add the custom connection to the app we created in the previous step.
Go to Configure → SSO Connections → Add Connection → For All Users. Switch to Custom Provider and put all the information like this:
Use your Threads App ID as the Client ID and your Threads App Secret as the Client Secret.
After adding the connection, there’re a few things more you need to do:
Set Redirect Callback URLs
In the Clerk dashboard you’ll see an Auth Provider Configuration section. Take the Authorized redirect URI from there and paste it in your Meta app settings Redirect Callback URLs value:
Add Threads tester
To be able to use the Threads API while on development we need to also add a Threads user who’s allowed to login. Go to the Roles section in your Meta app dashboard and add your Threads account as a Threads tester:
Set the scopes
We need to tell Clerk which scopes are we going to request while doing the OAuth flow. These are threads_basic
and threads_content_publish
:
Now there’s a little problem here. If you set the scopes like the previous image, it won’t work. I’ll tell you why.
When Clerk makes the request to initiate the OAuth flow, the URL looks something similar to this: https://threads.net/oauth/authorize?client_id=<THREADS_APP_ID>&redirect_uri=<REDIRECT_URI>&scope=threads_basic+threads_content_publish&response_type=code
.
Now, what happens is that this: “threads_basic+threads_content_publish“ is not understood by Threads API. It doesn’t work. It expects a comma-separated list of scopes.
So how do we fix that? Easy:
This is a little “hack” that allows us to form the correct URL that Threads will understand.
Attribute Mapping
When the OAuth flow finishes, a request to the User Info URL will be made to retrieve the data of the user (name, username, profile photo…). We then need to map those values to the corresponding values of the Clerk User object. There’s a specific section in the SSO configuration for this:
Enable connection
This is an easy one. Toggle the switch at the top right corner to enable our custom Threads connection:
3️⃣ Code the “Connect Threads” button!
Ok time to code a little bit, right? Add a simple beautiful button like this one:
And this is all the code needed to initiate the login with Threads functionality:
signIn.authenticateWithRedirect({
strategy: "oauth_custom_threads",
redirectUrl: "/sso-callback",
redirectUrlComplete: "/",
});
That’s it! Click on the button and log into your Threads account. You should use the same account you registered before as “Threads Tester”.
👉🏽 Next steps
Ok so that’s it for this first post! We successfully integrated Threads into our app.
But that’s not all. In the introduction I mentioned that DualPosts is about POSTING. So in the next part of this series we’ll dive deep into how I used Threads API to actually post something to the user’s account
Until then, see you 👋🏽
Subscribe to my newsletter
Read articles from David Fernández Ortiz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Fernández Ortiz
David Fernández Ortiz
I am a developer from Granada, a beautiful city in the south of Spain. I'm passionate about Full-Stack development and DevOps. Want a beer 🍻?