Enhance Office Efficiency: Smart Fridge Water Monitoring with Microsoft Power Automate and SeeedStudio ESP32 XIAO C3
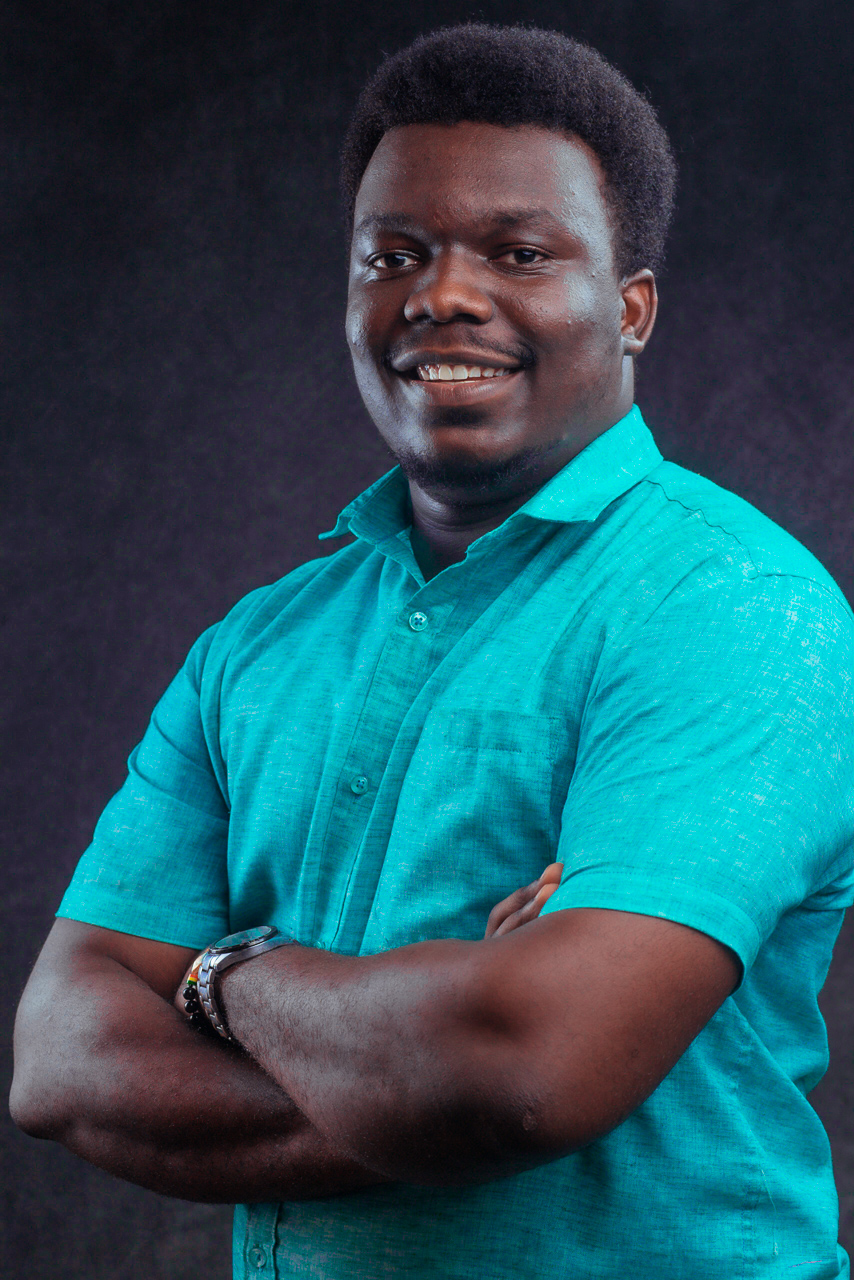
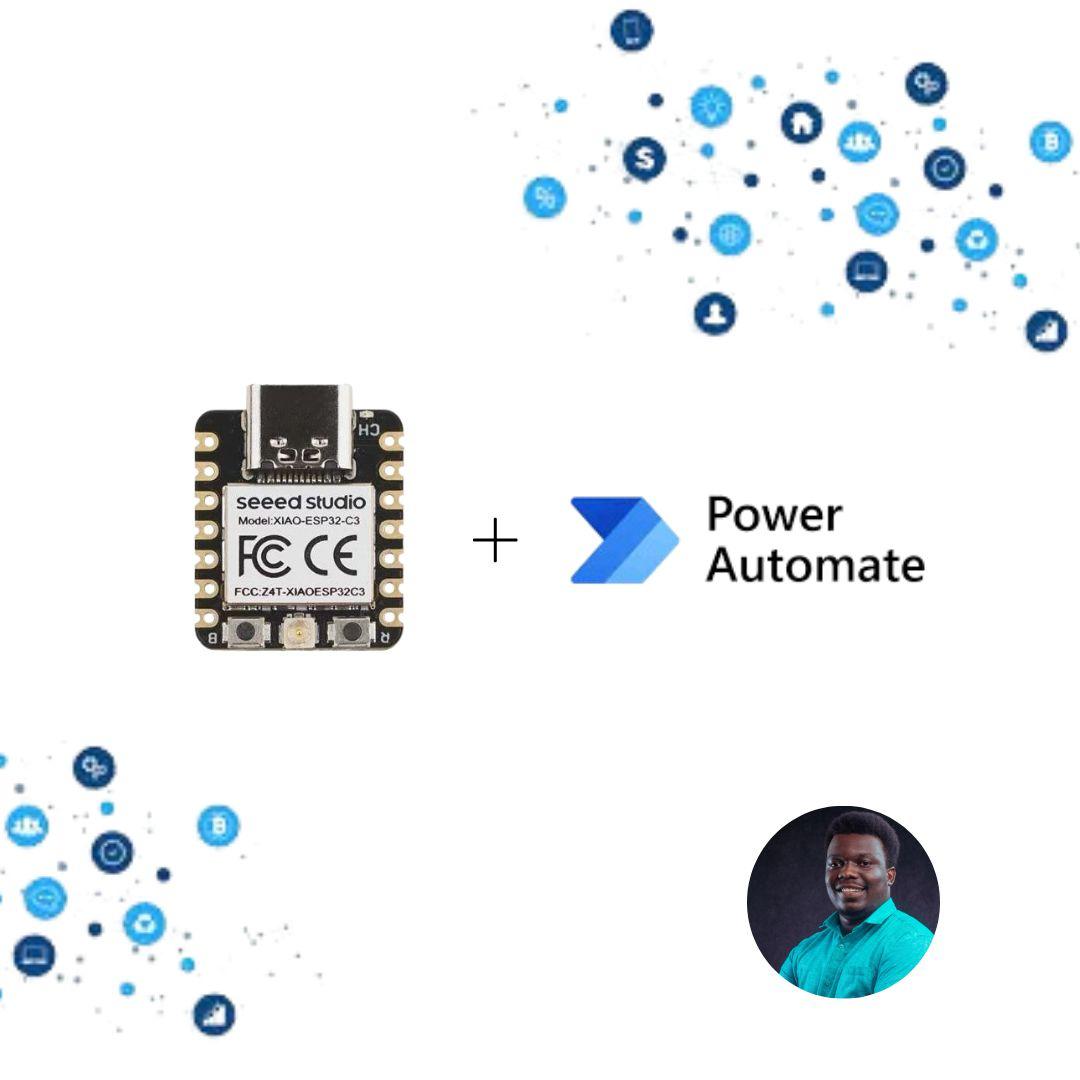
Table of Contents
Introduction
Project Overview
Required Components
Setting Up the Hardware
Circuit Diagram
Pin Connections
Programming the ESP32 XIAO C3
Code for Measuring Water Level
Logic for Notifications
Sending HTTP Requests to Power Automate
Creating a Notification Flow in Power Automate
Code for Measuring Water Level
Logic for Notifications
Sending HTTP Requests to Power Automate
Integrating ESP32 and Power Automate
Sensor Detection and Monitoring
Triggering Notification Flow
Automated Email Alert
Testing and Deployment
Simulation in Wokwi
Real-World Testing and Troubleshooting
Benefits of the Smart Fridge Water Monitoring System
Conclusion
INTRODUCTION
Certain essentials in fast-paced office environments, such as a consistent supply of drinking water, are critical but easy to overlook. At Afrilogic, where our team prioritizes innovation and productivity, we discovered that manually checking the office fridge for water was an inefficient use of time and sometimes overlook it. Frequently, the water supply ran low, leaving team members without water until someone noticed and replenished it. This process was prone to delays and interruptions, which hampered the overall office workflow and reduced productivity.
To address this, I created a Smart Fridge Water Monitoring System, which automates the process. Using an ESP32 XIAO C3 and Power Automate, this system monitors the water packs in the fridge and sends an automated notification to the office manager when the water needs to be replenished. This solution not only eliminates the need for manual checks, but also ensures that we always have access to critical supplies, allowing the team to focus on what is most important. This project demonstrates how IoT can increase workplace efficiency and transform everyday office processes.
PROJECT OVERIEW
The Smart Fridge Water Monitoring System is a useful IoT solution for optimizing essential office tasks by monitoring the supply of bottled or sachet water in the office fridge. In our office, water is kept in a separate bowl inside the fridge that holds bottles or sachets. This system uses a waterproof ultrasonic sensor connected to an ESP32 XIAO C3 microcontroller to detect the height of the items in the bowl rather than directly measuring liquid levels. When the height of the water items falls below a predetermined threshold, indicating a low supply, the system sends an automated alert via Power Automate to notify the office manager, allowing for timely restocking. This method not only eliminates the need for manual checks, but it also improves the reliability of the water supply, ensuring that critical office resources are always accessible.
While we use an ultrasonic sensor for accuracy and non-contact measurements, other sensors, such as infrared distance sensors, could be used for similar applications. The Smart Fridge Water Monitoring System saves time, improves resource management, and allows team members to focus on more important tasks, thereby increasing overall office productivity.
REQUIRED COMPONENTS
SeeedStudio ESP32 XIAO C3: A small, thumb-sized development board that's based on the Espressif ESP32-C3 WiFi/Bluetooth dual-mode chip.
HC-SR04 Ultrasonic Sensor: For measuring the distance between objects.
Microsoft Power Automate: A a cloud-based tool that automates repetitive tasks and business processes.
Wokwi Simulator (if you do not have hardware available): Useful for testing the circuit virtually before implementing it with hardware.
SETTING UP THE HARDWARE
Pin Connections
VCC on the Ultrasonic connects to 3.3V on the ESP32 XIAO C3.
GND connects to GND on the ESP32.
Trigger Pin (TRIG) connects to digital pin D8 on the ESP32.
Echo Pin (ECHO) connects to digital pin D9 on the ESP32.
Make sure to add the following libraries to enable smooth development, ultrasonic library , arduinoJson library.
PROGRAMMING THE ESP32 XIAO C3
Code for Measuring Water Level
This code uses an HC-SR04 ultrasonic sensor to measure the height of water bottles or sachets inside a fridge, checking whether the water supply is low. The SeeedStudio ESP32 XIAO C3 microcontroller is connected to the sensor, and if the water level falls below a certain threshold, the system sends a notification via Power Automate to alert the office manager that the fridge needs to be restocked.
Setup and Initialization
#include <Ultrasonic.h> #include <WiFi.h> #include <HTTPClient.h> #include <ArduinoJson.h> const char* ssid = "Wokwi-GUEST"; //Connects to virtual wifi const char* password = ""; // Define the request URL (replace with your endpoint URL) String requestUrl = ""; #define TRIG 8 #define ECHO 9 int threshold = 20; Ultrasonic water_checker(TRIG, ECHO);
The WiFi credentials (
ssid
andpassword
) are set for the ESP32 to connect to the network.requestUrl
contains the URL of the Power Automate flow trigger that will be called when the water level is low.The HC-SR04 ultrasonic sensor is connected to digital pins 8 and 9 on the ESP32, where pin 8 is the Trigger pin and pin 9 is the Echo pin.
The
threshold
value is set to 20 cm, meaning if the sensor detects a distance greater than 20 cm (indicating a low water level), it will trigger a
- WiFi Connection
void setup() { Serial.begin(115200); WiFi.begin(ssid, password); Serial.println("Connecting"); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.print("Connected to WiFi network with IP Address: "); Serial.println(WiFi.localIP()); }
WiFi.begin() is used to connect the ESP32 to the WiFi network using the provided SSID and password.
The
while
loop checks the connection status and prints dots until the ESP32 is connected to the network. Once connected, the local IP address is printed to the serial monitor.
Measuring Water Level and Sending Email
void loop() { int distance = water_checker.read(); Serial.println(distance); delay(1000); if (distance > threshold) { Serial.println("Water is finished in the fridge is finsihed please fill it"); sendEmail(); delay(5000); } }
The
if (distance > threshold)
condition checks if the measured distance is greater than the threshold value (20 cm in this case). If the distance is greater, it indicates that the water level is low (since the sensor is measuring the height of the water).If the condition is true, a message is printed to the serial monitor, and the
sendEmail()
function is called to notify the office manager or kitchen staff via Power Automate.A
delay(5000)
ensures that multiple notifications are not sent in rapid succession, allowing a 5-second pause between triggers.
- Send Notification/Email Function
void sendEmail() {
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin( requestUrl);
http.addHeader("Content-Type", "application/json");
StaticJsonDocument<200> doc;
doc["message"] = true; // Set the JSON key-value pair
// Serialize JSON document to a string
String message;
serializeJson(doc, message);
// Send the HTTP POST request with the JSON payload
int httpResponseCode = http.POST(message);
if (httpResponseCode > 0) {
Serial.print("HTTP Response code: ");
Serial.println(httpResponseCode);
String response = http.getString();
Serial.println(response);
} else {
Serial.print("Error code: ");
Serial.println(httpResponseCode);
}
http.end();
} else {
Serial.println("WiFi Disconnected");
}
}
The
sendEmail()
function checks if the ESP32 is connected to WiFi using WiFi.status().If connected, an HTTPClient object is created to send a POST request to the Power Automate flow URL (
requestUrl
).A JSON object is created using ArduinoJson, with a key-value pair (
"message": true
), indicating that the water level is low and a notification needs to be sent.The
serializeJson(doc, message)
function converts the JSON document to a string, which is then sent using http.POST().If the HTTP response code is 202 (successful), it prints the response to the serial monitor. If there's an error, the error code is printed.
Logic Summary:
Measure distance: The ultrasonic sensor calculates the distance between the sensor and the water surface.
Check distance: If the distance exceeds the threshold (i.e., the water level is low), a notification is triggered.
Send notification: The system sends a notification to Power Automate, which triggers an email to the relevant personnel to refill the water.
You can get the full code in my wokwi project link.
SENDING HTTP REQUEST TO POWER AUTOMATE
Creating a Notification Flow in Power Automate
Setting Up the HTTP Trigger
In Power Automate, create a new Automated Cloud Flow.
Set the When an HTTP request is received trigger to allow the ESP32 to send a POST request.
Configuring the Email Notification
Add a Send an Email action.
Customize the message to notify the office manager.
You can watch the video to setup the power automate flow
INTEGRATING ESP32 & POWER AUTOMATE
Sensor Detection and Monitoring
The ultrasonic sensor detects water packs and continuously checks if the level falls below the threshold.
Triggering Notification Flow
When the water pack level is low, the ESP32 Xiao sends a POST request to Power Automate.
Automated Email Alert
Power Automate sends an automated notification email to relevant personnel.
TESTING & DEPLOYMENT
Simulation in Wokwi
Before implementing the actual hardware, you can use the Wokwi simulator to test the setup. visit https://wokwi.com/
Real-World Testing and Troubleshooting
After assembling the components, monitor the sensor's distance readings and adjust the threshold if necessary.
Benefits of the Smart Fridge Water Monitoring System
Time-saving: No need for manual water level checks.
Improved Efficiency: Automated alerts ensure timely refilling, preventing shortages.
Scalability: This solution can be adapted for other supply monitoring needs, such as coffee or snacks.
CONCLUSION
The Smart Fridge Water Monitoring System is a practical example of how IoT and automation tools like Power Automate can enhance productivity in the workplace. By using a simple yet effective setup with the ESP32 and ultrasonic sensor, we’ve transformed a manual process into an efficient, automated system. This project is just one way IoT can simplify everyday tasks and make office life smoother.
For developers looking to build innovative solutions, this is a great place to start experimenting with IoT and cloud-based automation. Don’t hesitate to try new things, explore different sensors, or adapt this project to fit other use cases in your environment. Keep an eye out for upcoming blogs and videos on even more ways you can use IoT to improve efficiency in your projects.
Stay tuned, and happy innovating!
Subscribe to my newsletter
Read articles from Kimkpe Arnold Sylvian directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
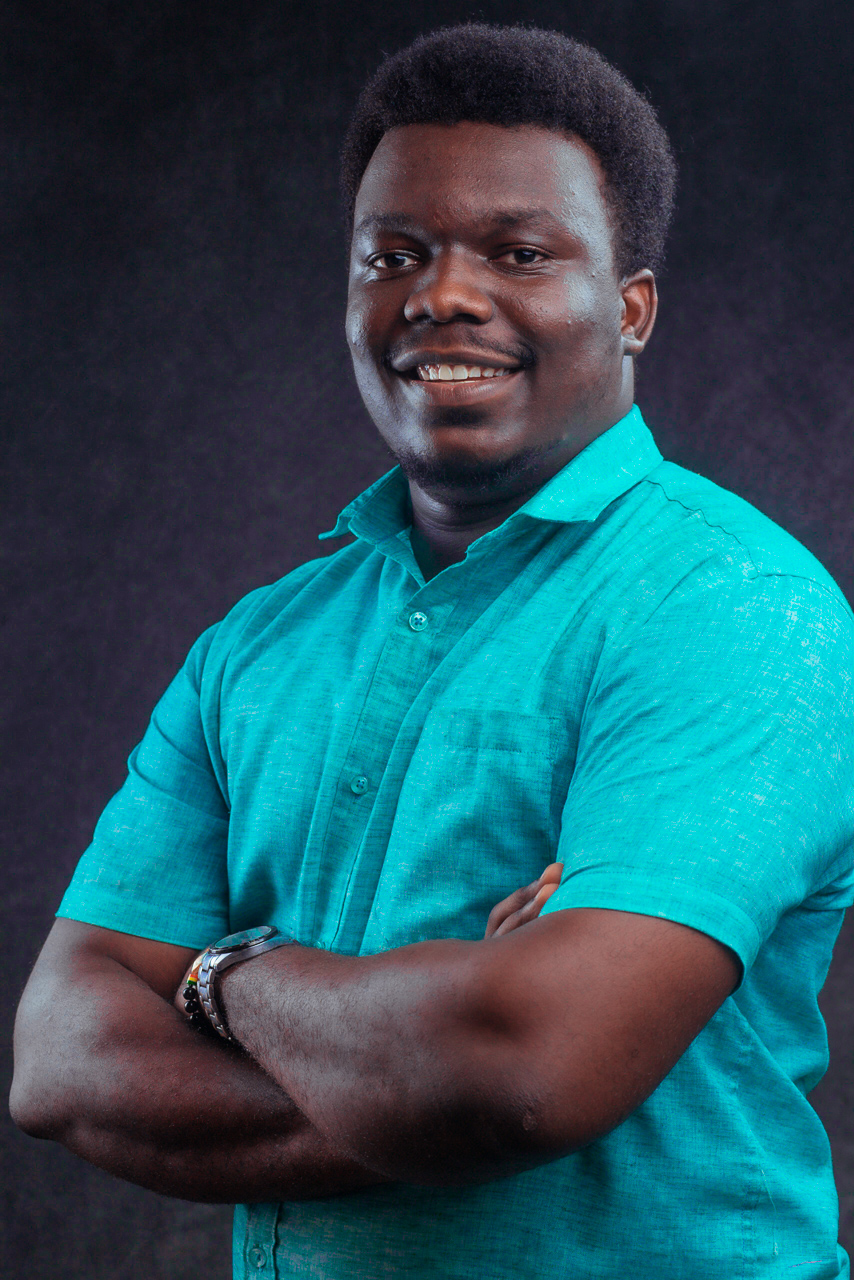
Kimkpe Arnold Sylvian
Kimkpe Arnold Sylvian
I’m an IoT firmware/software engineer who loves bringing ideas to life by designing, building, and maintaining IoT solutions from the ground up. My expertise spans everything from hardware design to full-scale deployment, ensuring every step is seamless and efficient. I’m passionate about robotics and mechatronics engineering, and I have strong skills in ROS and other essential tools. As a full-stack IoT developer, I enjoy tackling complex problems and creating innovative, reliable solutions that make a real impact.