Variables in Bash Scripting
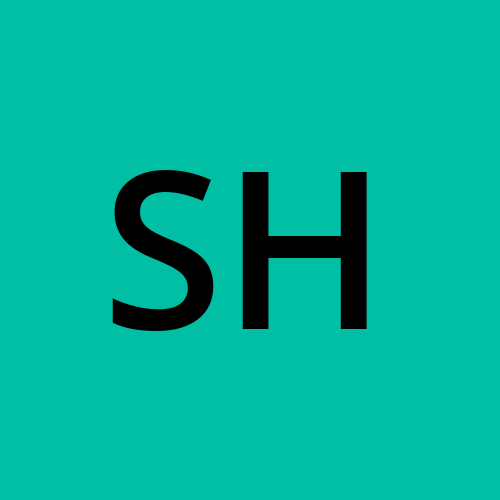
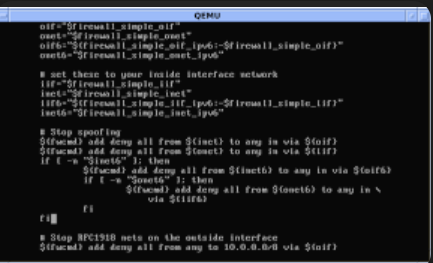
In Bash scripting, variables allow us to store information for later use, minimizing repetition and enable us to save command outputs directly into variables. For example, the command file =$(ls) will execute ls, store its output in the file variable, and allow us to use it later.
Declaring Variables
To declare a variable in Bash, simply type the variable name, followed by an equal sign (=) with no spaces, and assign it a value within quotes if it's text or a number. Example: myname="Sharon". Variables can contain both strings and numbers.
Printing Variables
Use the echo command to print a variable’s contents by including a dollar sign ($) before the variable name. For example, echo $myname will print Sharon. The dollar sign is essential to avoid name collisions and to access the variable’s value rather than its name. Keep in mind that variables in Bash aren’t saved once you exit the terminal.
Example Script to Print Name and Age:
#!/bin/bash
myname="Sharon"
age="27"
echo "My name is $myname."
echo "I am $age years old."
When executed, this script will print:
My name is Sharon. I am 27 years old.
Types of Variables: Manual and Environment Variables
In Bash, there are manually created variables and environment variables. Environment variables are predefined, usually in uppercase, and can be viewed with the env command. For better readability, it’s recommended to declare manually created variables in lowercase.
Handling Single Quotes Inside Single-Quoted Text
If you need to use single quotes within a single-quoted string, Bash will interpret it as the end of the string. To avoid this, you can either:
Escape the single quote with a backslash (\), like so:
echo 'I\'m $age years old'
Output : I'm 27 years old.Use double quotes for the entire string when it contains single quotes:
echo "I'm $age years old"
Output: I'm 27 years old.
Subscribe to my newsletter
Read articles from Sharon Jonallagadda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
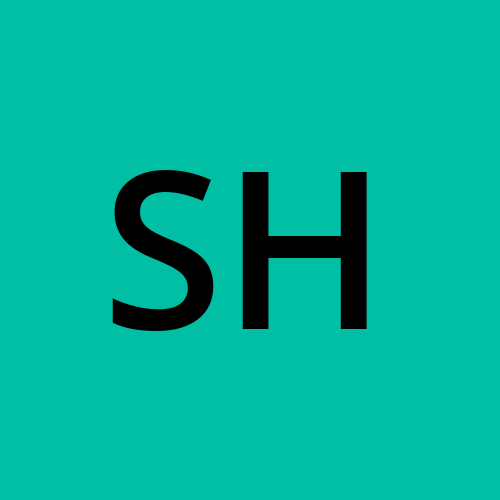
Sharon Jonallagadda
Sharon Jonallagadda
Pursuing my Master’s in IT. Documenting my journey as I work towards securing an internship and a full-time role. Sharing the knowledge and experiences I gain along the way.