Using Node.js for Serverless Functions: A Comprehensive Guide

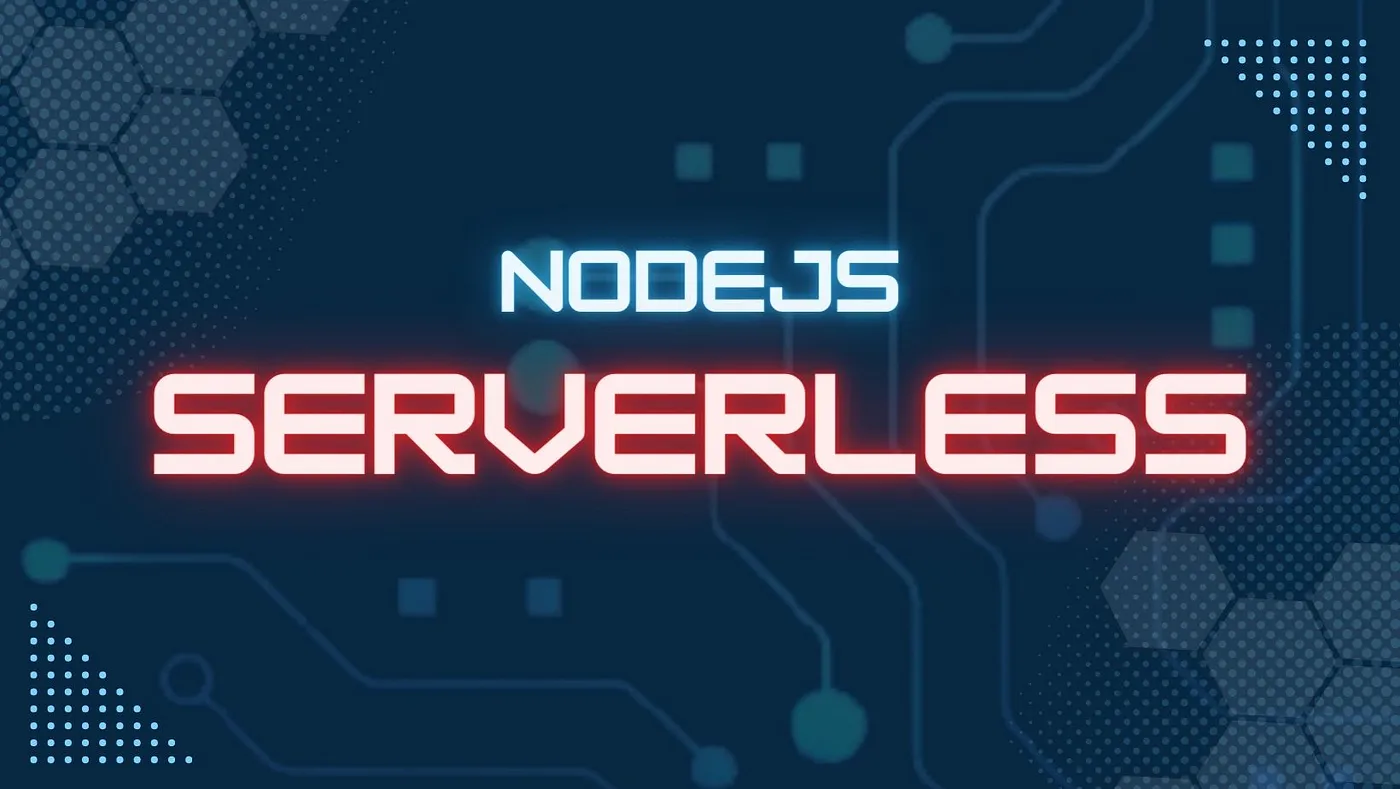
As applications increasingly move towards cloud-native architectures, serverless computing has become a popular solution for its scalability, flexibility, and cost-efficiency. In this guide, we’ll explore how to use Node.js to build serverless functions, understand why Node.js is well-suited for this architecture, and walk through best practices for deploying serverless functions in production environments.
Why Choose Serverless Computing?
Serverless computing allows developers to build and deploy code without managing servers. With serverless, cloud providers manage the underlying infrastructure, scaling resources up or down as needed, so you only pay for the actual computing time your functions use.
Key Benefits:
Automatic Scaling: Serverless functions scale automatically in response to demand.
Cost Efficiency: You only pay for execution time, so there's no need to pay for idle server resources.
Reduced Maintenance: Infrastructure and scaling are managed by the provider, reducing the time and resources spent on server management.
Why Node.js for Serverless Functions?
Node.js is a powerful, lightweight, and efficient runtime for building fast, scalable server-side applications. It's particularly well-suited for serverless functions due to:
Fast Startup Time: Node.js applications can spin up quickly, which reduces cold start times in serverless environments.
Non-blocking I/O: With its asynchronous and event-driven nature, Node.js is optimized for tasks like handling HTTP requests and managing multiple concurrent connections.
Rich Ecosystem: Node.js has a vast ecosystem of libraries and modules, making it easy to integrate with various services and databases.
JavaScript Popularity: JavaScript’s ubiquity in web development makes it accessible to many developers, enabling easy transition from frontend to backend serverless code.
Getting Started with Node.js Serverless Functions
Serverless functions are typically deployed using cloud services like AWS Lambda, Google Cloud Functions, or Azure Functions. Here, we'll focus on AWS Lambda, but the concepts are similar across providers.
Prerequisites
Node.js Installed: Ensure you have Node.js installed on your machine.
AWS Account: For AWS Lambda, create an account and configure the AWS CLI.
Serverless Framework or AWS SAM: Tools like the Serverless Framework or AWS SAM make it easier to develop, deploy, and manage serverless functions.
Step 1: Create a Basic Lambda Function
Initialize the Project Start by creating a new directory and initializing it with
npm
:mkdir my-serverless-app cd my-serverless-app npm init -y
Write the Function Code Create a
handler.js
file. This file will export the function handler for AWS Lambda.// handler.js exports.handler = async (event) => { const name = event.queryStringParameters?.name || 'World'; return { statusCode: 200, body: JSON.stringify({ message: `Hello, ${name}!` }) }; };
This simple function responds to HTTP GET requests, taking an optional
name
parameter and returning a greeting message.Define the Serverless Configuration
If you're using the Serverless Framework, create a
serverless.yml
file:service: my-serverless-app provider: name: aws runtime: nodejs14.x functions: hello: handler: handler.handler events: - http: path: hello method: get
Deploy the Function
To deploy with the Serverless Framework:
npx serverless deploy
After deployment, you'll receive a URL to test your function.
Step 2: Test the Serverless Function
Once deployed, use the provided endpoint URL to test the function:
curl https://<your-api-id>.execute-api.<region>.amazonaws.com/dev/hello?name=Node
You should receive a JSON response like:
{ "message": "Hello, Node!" }
Step 3: Monitor and Debug
AWS CloudWatch provides logging for Lambda functions, which is helpful for debugging:
View Logs: You can view function logs in the CloudWatch Console.
Use Console.log: Debug by adding
console.log
statements in your code.
Step 4: Optimize for Production
To make the function production-ready, consider optimizing for performance, security, and reliability.
Best Practices for Node.js Serverless Functions
1. Minimize Cold Start Times
Use lighter functions by limiting dependencies and reducing package size. Tools like Webpack can bundle and minify your code.
Opt for Provisioned Concurrency in AWS Lambda if you require faster cold start times for critical functions.
2. Limit Dependencies
Only import necessary modules, especially for performance-critical paths.
Consider serverless-optimized libraries, which are designed for lightweight and fast execution.
3. Environment Variables for Configuration
Store configuration settings (like API keys) as environment variables, which can be set in the cloud provider’s console or in configuration files (e.g.,
serverless.yml
).Avoid hardcoding sensitive information in your code.
4. Asynchronous Handling and Error Handling
Use
async/await
to handle asynchronous operations, ensuring cleaner code and better error handling.Implement comprehensive error handling. AWS and other providers automatically retry failed requests, so design your function to be idempotent.
5. Optimize for Memory and Execution Time
Choose an appropriate memory setting for your function, balancing between cost and performance.
Aim to keep execution time under a few seconds. If you require long-running tasks, consider alternatives like AWS Step Functions for orchestration.
6. Monitoring and Logging
Use CloudWatch or similar services to monitor function usage and performance.
Structured logging (JSON format) makes it easier to analyze logs for troubleshooting and metrics.
7. Use Layers for Shared Code
- AWS Lambda supports layers, which allow you to share code (such as libraries) across multiple functions without duplicating them in each function package.
8. Follow Secure Coding Practices
Validate and sanitize input to prevent injection attacks.
Follow the principle of least privilege when assigning IAM roles to functions.
Conclusion
Node.js is an excellent choice for building serverless functions due to its lightweight runtime, asynchronous nature, and vast ecosystem. Leveraging serverless platforms allows you to scale applications seamlessly and control costs effectively. By following best practices around dependency management, error handling, and security, you can deploy reliable, high-performance serverless functions suited to modern cloud-native applications.
With this guide, you have a foundational understanding of how to create and deploy Node.js serverless functions. Whether you're building a simple API or a complex event-driven workflow, serverless functions with Node.js can help you scale with ease. Dive in, experiment, and unlock the power of serverless computing!
Support My Work
If you found this guide on DevOps helpful and would like to support my work, consider buying me a coffee! Your support helps me continue creating beginner-friendly content and keeping it up-to-date with the latest in tech. Click the link below to show your appreciation:
I appreciate your support and happy learning! ☕
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.