Master Guide for Responsiveness

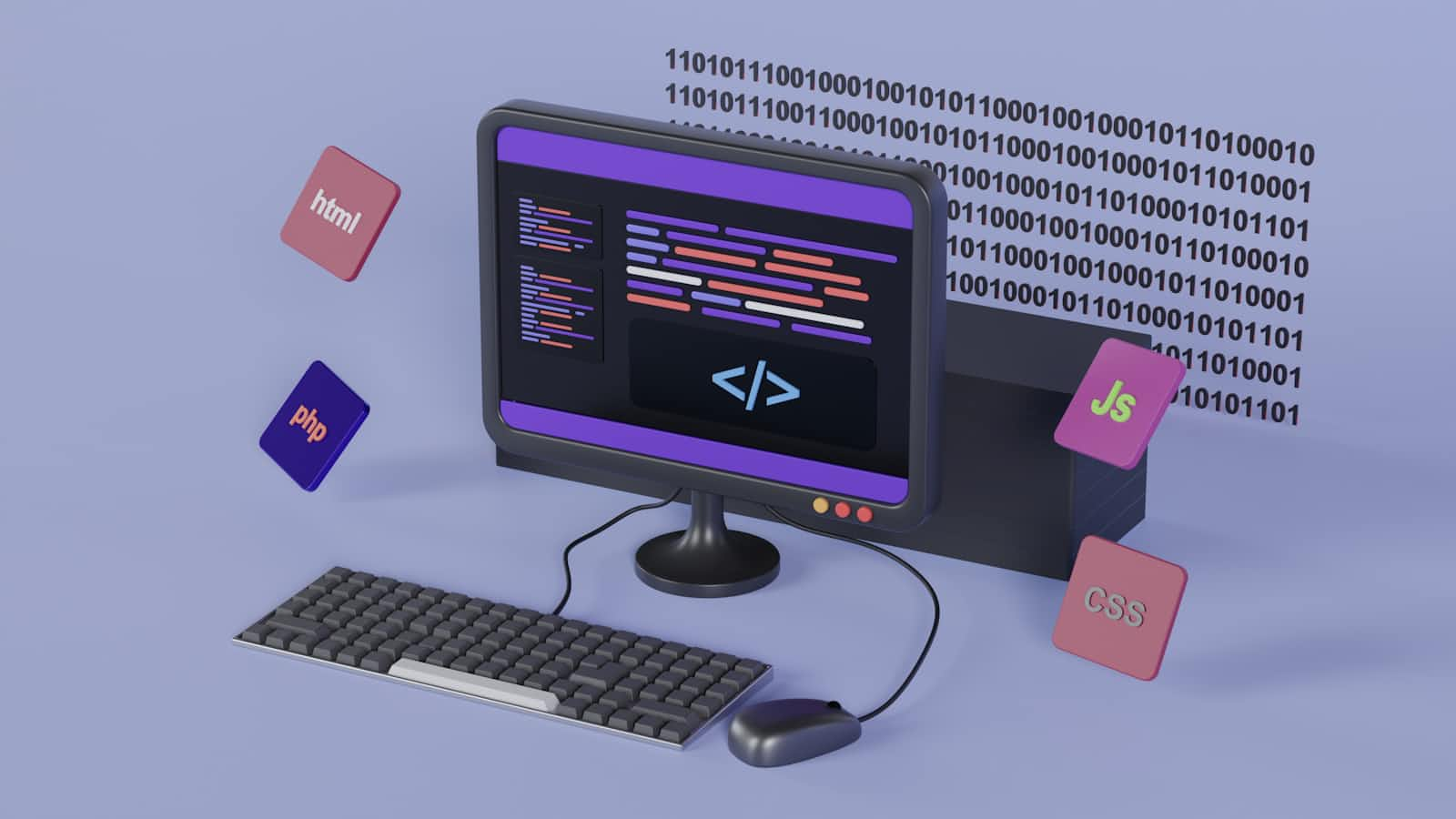
Responsive design ensures your website or app looks (and works!) awesome on any device. It’s more than just shrinking content for smaller screens; it’s about reorganizing and adapting content to fit various screen sizes, orientations, and capabilities. Think of it as making sure your website is dressed for any screen it’s invited to.
Methods to Achieve Responsiveness
Media Queries
Flexible Images
Responsive Typography
Fluid Grids
Breakpoints and Layout Tweaks
Advanced Tips: View Units, REMs, CSS Grid, Flexbox
Let’s dive into each method and see how it helps achieve responsiveness.
1. Media Queries
Media queries allow you to create rules that adjust the layout based on screen width, orientation, and other properties.
@media (max-width: 768px) {
.container {
width: 100%;
}
}
This tells the browser, “If the viewport width is 768px or less, make the container take up the full width.”
You can also combine multiple conditions:
@media (min-width: 768px) and (orientation: landscape) {
/* styles for tablets in landscape mode */
}
2. Fluid Grids: Make It Stretchy!
A fluid grid is a flexible layout that adapts based on screen width. Instead of using fixed pixel sizes, you use percentages. CSS Grid and Flexbox are powerful tools for achieving this.
CSS Grid for responsiveness
CSS Grid is great for creating complex, responsive layouts.
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 16px;
}
This sets up a grid that fits as many 250px
-wide columns as possible, stretching them to fill available space.
Flexbox for Dynamic Alignment
Flexbox is helpful for aligning items, especially for one-dimensional layouts.
.container {
display: flex;
flex-wrap: wrap;
}
.item {
flex: 1 1 200px; /* Grow, shrink, and have a base width */
}
Flexbox and CSS Grid work well together: use Grid for major structure and Flexbox for aligning items within cells.
3. Flexible Images: Avoid Squished Cats
Avoid images that appear stretched or squished by using CSS.
img {
max-width: 100%;
height: auto;
}
The max-width: 100%
rule ensures that images scale within their containers without exceeding their original size, while height: auto
maintains the aspect ratio.
Advanced Tips for Images:
Use the
srcset
attribute for responsive images, letting the browser choose the best size.Use
<picture>
elements for art direction, displaying different images on mobile versus desktop.
4. Responsive Typography: Make It Readable
Fonts that look good on a big screen can become too small on mobile. Here are two methods for responsive typography:
Viewport Units
h1 {
font-size: 5vw; /* scales with viewport width */
}
Be cautious with vw
units, as they may become too small or too large. Combining vw
with rem
can give more control.
Clamp clamp()
clamp()
lets you set a min, preferred, and max size:
h1 {
font-size: clamp(1.5rem, 2vw + 1rem, 3rem);
}
This is ideal for text scaling, setting a minimum, growing based on screen width, and capping at a max size.
5. Breakpoints and Layout Tweaks
Add breakpoints where the content dictates. Instead of sticking to arbitrary sizes, inspect your layout and add breakpoints wherever things start to look awkward.
Common Breakpoints
320px - Small phones
768px - Tablets
1024px - Small laptops
1200px and up - Desktops
Use these as guidelines, but avoid adding too many breakpoints.
Advanced Tips
Viewport Units for Padding and Margins
Use vw
and vh
units for padding and margins, especially for sections that should scale with the screen.
section {
padding: 5vh 5vw;
}
REMs and EMs for Consistency
REMs and EMs maintain proportional sizes across the site. REMs scale based on the root font size, while EMs are relative to the parent element.
Combine Grid and Flexbox
Use CSS Grid for the main layout and Flexbox for smaller items within grid cells. This keeps your code clean and your layout dynamic.
Testing Tools: Don’t Skip This
Test on actual devices if possible! Emulators can only take you so far. Here are some essential testing tools:
Browser DevTools - Every browser has a responsive mode. Use it
Screenfly - A tool for testing different devices and screen sizes
DeviceLab - If you have the resources, set up a DeviceLab with actual phones and tablets
Conclusion
Responsive design can be challenging, but with the right techniques, you’ll be mastering it in no time! Remember:
Use media queries to adapt your styles
Embrace fluid grids and flexible images
Scale typography to keep it readable
Tweak layouts with smart breakpoints
Use advanced techniques like viewport units, REMs, and the clamp function.
The goal? A site that feels seamless, no matter where or how it’s viewed. Get your code editors open, experiment with these tricks, and make your designs work everywhere. Happy coding!
Subscribe to my newsletter
Read articles from Savita Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Savita Verma
Savita Verma
I'm a frontend developer trying to do more than just ship fast code. I care about clarity, purpose, and mindful work. Currently based in Bangalore and working my way toward becoming a senior dev. I write about the technical, the emotional, and everything in between. Feel free to reach out, I’m always excited to chat about frontend, tech, or opportunities. Email Id: svitaverma10@gmail.com