Deployment of tomcat server in AWS EC2 and stream logs to CloudWatch
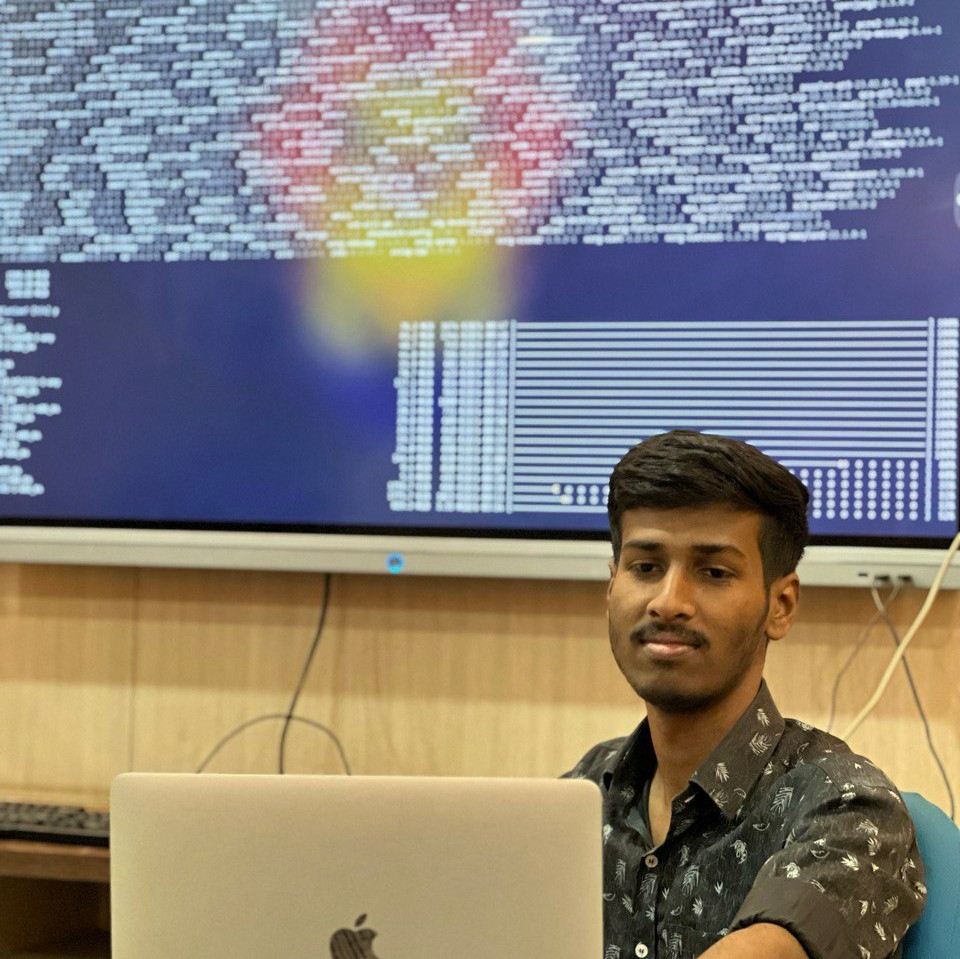

Setup EC2 Instance
1. Create EC2 Instance Using Terraform or AWS Management Console
Create a main.tf
file with the following configuration:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "tomcat" {
ami = "ami-0866a3c8686eaeeba"
instance_type = "t3.micro"
key_name = "tomcat-server" # Replace with your key name
security_groups = [aws_security_group.tomcat_sg.name]
tags = {
Name = "Tomcat-Server"
}
}
resource "aws_security_group" "tomcat_sg" {
name_prefix = "tomcat-sg-"
ingress {
from_port = 8080
to_port = 8080
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
}
Run Terraform commands:
terraform init
terraform plan
terraform apply
Modify IAM Role for EC2 instance
Step 1: Create a New IAM Role
1.1 Log in to AWS Management Console
Open the AWS Management Console.
Navigate to IAM (Identity and Access Management) under the Security, Identity & Compliance section.
1.2 Create a New Role
In the IAM Dashboard, click on Roles from the left-hand navigation pane.
Click the Create role button at the top of the page.
1.3 Select Trusted Entity
In the Select trusted entity step, choose AWS service.
Under Choose a use case, select EC2 (This option allows EC2 instances to assume the role).
Click Next: Permissions.
1.4 Attach Policies
In the Attach permissions policies step, search for CloudWatchAgentServerPolicy.
Check the box next to CloudWatchAgentServerPolicy to select it.
You may also attach additional policies if needed (e.g., AmazonEC2ReadOnlyAccess for read-only EC2 access).
1.6 Review and Create Role
Provide a Role name, such as EC2CloudWatchAgentRole.
Review the role details.
Click Create role.
Step 2: Attach the Role to the EC2 Instance
2.1 Navigate to EC2 Dashboard
Go to the EC2 Dashboard.
Under the Instances section, find the instance that you want to modify.
2.2 Modify IAM Role
Select the EC2 instance.
In the Instance details section at the bottom, click the Actions dropdown button.
Choose Security → Modify IAM Role.
2.3 Assign the New IAM Role
In the Modify IAM role dialog, select the new role you just created (EC2CloudWatchAgentRole).
Click Update IAM role.
Steps to Connect to EC2 via SSH:
Check Security Group:
Ensure the inbound rule for SSH (port 22) is allowed from your IP.
Get Public IP or DNS:
In the EC2 console, find the Public IP or Public DNS of your instance.
Set Permissions on Private Key:
Run:
chmod 400 /path/to/your-key.pem
Connect via SSH:
For Linux/macOS:
Install and Configure Apache Tomcat 10
Apache Tomcat is a web server and servlet container that is used to serve Java applications. It’s an open-source implementation of the Jakarta Servlet, Jakarta Server Pages, and other technologies of the Jakarta EE platform.
Step 1 — Installing Tomcat
Download the latest version, set up a separate user, and appropriate permissions for it. You will also install the Java Development Kit (JDK).
For security purposes, Tomcat should run under a separate, unprivileged user. Run the following command to create a user called tomcat:
sudo useradd -m -d /opt/tomcat -U -s /bin/false tomcat
update the package manager cache by running and install jdk:
sudo apt update
sudo apt install default-jdk
check the version of the available Java installation:
java –version
To install Tomcat, you’ll need the latest Core Linux built for Tomcat 10, which you can get from the downloads page. Select the latest Core Linux build, ending in .tar.gz. At the time of writing, the latest version was 10.0.20.
First, navigate to the /tmp directory:
cd /tmp
Download the archive using wget by running the following command:
wget
https://dlcdn.apache.org/tomcat/tomcat-10/v10.0.20/bin/apache-tomcat-10.0.20.tar.gz
The wget
command downloads resources from the Internet.
Then, extract the archive you downloaded by running:
sudo tar xzvf apache-tomcat-10*tar.gz -C /opt/tomcat --strip-components=1
Since you have already created a user, you can now grant tomcat ownership over the extracted installation by running:
sudo chown -R tomcat:tomcat /opt/tomcat/
sudo chmod -R u+x /opt/tomcat/bin
Step 2 — Configuring Admin Users
Tomcat users are defined in /opt/tomcat/conf/tomcat-users.xml
Step 3 — Creating a systemd service
The systemd service that you will now create will keep Tomcat quietly running in the background. The systemd service will also restart Tomcat automatically in case of an error or failure.
Tomcat, being a Java application itself, requires the Java runtime to be present, which you installed with the JDK in step 1. Before you create the service, you need to know where Java is located. You can look that up by running the following command:
sudo update-java-alternatives –l
store the tomcat service in a file named tomcat.service, under /etc/systemd/system
. Create the file for editing by running:
sudo nano /etc/systemd/system/tomcat.service
Add the following lines:
/etc/systemd/system/tomcat.service
[Unit]
Description=Tomcat
After=network.target
[Service]
Type=forking
User=tomcat
Group=tomcat
Environment="JAVA_HOME=/usr/lib/jvm/java-1.11.0-openjdk-amd64"
Environment="JAVA_OPTS=-Djava.security.egd=file:///dev/urandom"
Environment="CATALINA_BASE=/opt/tomcat"
Environment="CATALINA_HOME=/opt/tomcat"
Environment="CATALINA_PID=/opt/tomcat/temp/tomcat.pid"
Environment="CATALINA_OPTS=-Xms512M -Xmx1024M -server -XX:+UseParallelGC"
ExecStart=/opt/tomcat/bin/startup.sh
ExecStop=/opt/tomcat/bin/shutdown.sh
RestartSec=10
Restart=always
[Install]
WantedBy=multi-user.target
Modify the highlighted value of JAVA_HOME if it differs from the one you noted previously.
Here, you define a service that will run Tomcat by executing the startup and shutdown scripts it provides. You also set a few environment variables to define its home directory (which is /opt/tomcat as before) and limit the amount of memory that the Java VM can allocate (in CATALINA_OPTS). Upon failure, the Tomcat service will restart automatically.
When you’re done, save and close the file.
Reload the systemd daemon so that it becomes aware of the new service:
sudo systemctl daemon-reload
You can then start the Tomcat service by typing:
sudo systemctl start tomcat
Then, look at its status to confirm that it started successfully:
sudo systemctl status tomcat
Install cloudwatch on Ubuntu AWS EC2 instance
Download and install the Cloudwatch agent in your EC2 instance
wget
https://s3.amazonaws.com/amazoncloudwatch-agent/ubuntu/amd64/latest/amazon-cloudwatch-agent.deb
sudo dpkg -i -E ./amazon-cloudwatch-agent.deb
Step 3: Launch the Cloudwatch wizard
sudo /opt/aws/amazon-cloudwatch-agent/bin/amazon-cloudwatch-agent-config-wizard
Step 4: Choose settings so that /opt/aws/amazon-cloudwatch-agent/bin/config.json file will look like this:
{
"agent": {
"metrics_collection_interval": 60,
"run_as_user": "root"
},
"logs": {
"logs_collected": {
"files": {
"collect_list": [
{
"file_path": "/opt/tomcat/logs/catalina.out",
"log_group_class": "STANDARD",
"log_group_name": "tomcatLogs",
"log_stream_name": "{instance_id}",
"retention_in_days": -1
}
]
}
}
},
"metrics": {
"metrics_collected": {
"disk": {
"measurement": [
"used_percent"
],
"metrics_collection_interval": 60,
"resources": [
"*"
]
},
"mem": {
"measurement": [
"mem_used_percent"
],
"metrics_collection_interval": 60
},
"statsd": {
"metrics_aggregation_interval": 60,
"metrics_collection_interval": 60,
"service_address": ":8125"
}
}
}
}
Start the CloudWatch agent
sudo /opt/aws/amazon-cloudwatch-agent/bin/amazon-cloudwatch-agent-ctl -a fetch-config -m ec2 -s -c file:/opt/aws/amazon-cloudwatch-agent/bin/config.json
Tip: Command to check status of the Cloudwatch agent
service amazon-cloudwatch-agent status
Now all you have to do is restart the cloudwatch agent and you can see the logs on cloudwatch console: sudo systemctl restart amazon-cloudwatch-agent
References:
IAM roles for Amazon EC2
https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/iam-roles-for-amazon-ec2.html
https://tomcat.apache.org/download-10.cgi
https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/install-CloudWatch-Agent-on-EC2-Instance.html
Subscribe to my newsletter
Read articles from Shubham Kshetre directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
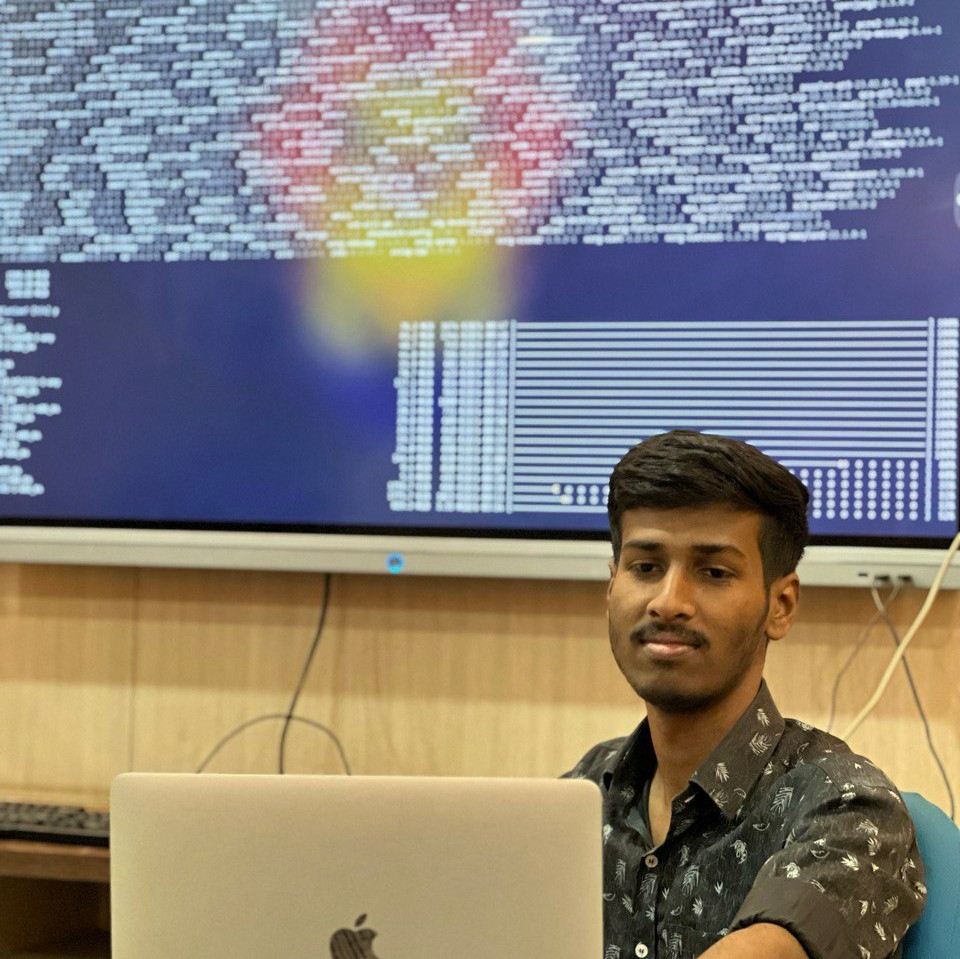