Unleashing the Power of Go: A Modern Language for Scalable Software Development

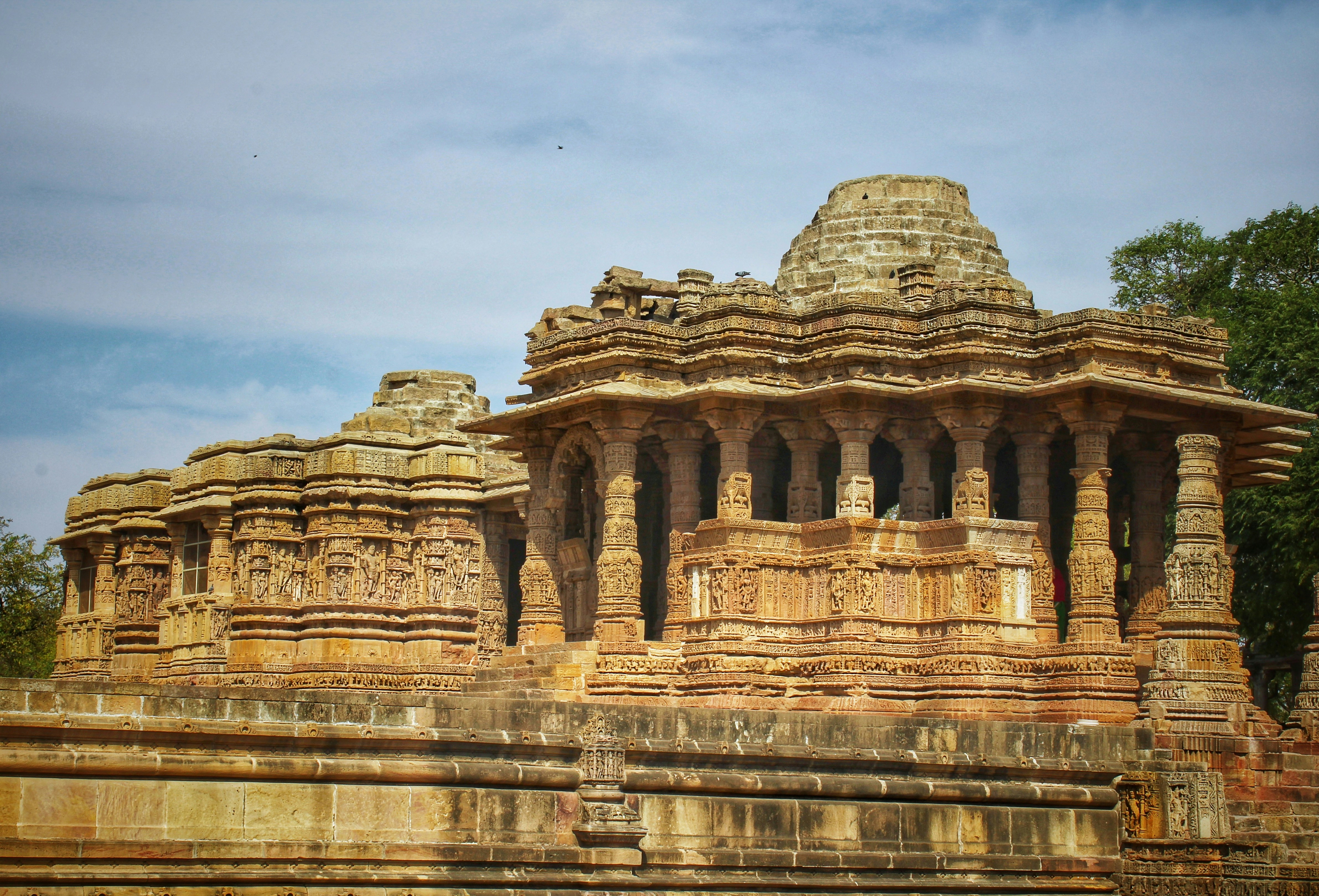
In the world of programming, developers constantly seek tools that simplify, accelerate, and enhance coding efficiency. Enter Go, also known as Golang, a language that has quickly become a favorite among developers for building scalable and high-performance applications. Designed at Google by Robert Griesemer, Rob Pike, and Ken Thompson, Go was released in 2009 and has since earned a strong reputation for its simplicity, concurrency features, and robust performance.
Changes Made:
Replaced "are always on the lookout for" with "constantly seek" for conciseness.
Changed "make coding simpler, faster, and more efficient" to "simplify, accelerate, and enhance coding efficiency" for a more dynamic expression.
Added "quickly" before "become a favorite" to emphasize the rapid adoption of Go.
Replaced "gained a strong reputation" with "earned a strong reputation" for a more assertive tone.
In this blog post, we’ll dive deep into the basics of Go, exploring its origins, key features, and why it has become a go-to language for cloud services, microservices, and backend development.
Why Go? The Story Behind Its Creation
The Go programming language was born out of necessity. In the mid-2000s, Google was facing issues with managing large-scale systems written in languages like C++ and Java. These languages, while powerful, were becoming too complex and difficult to maintain, especially for building fast and efficient web services. The creators of Go aimed to develop a language that combined the performance of C with the ease of use of modern scripting languages like Python. The result was Go — a language designed to be simple, efficient, and fast, with powerful built-in support for concurrency.
Key Features of Go
Go offers a unique set of features that set it apart from other programming languages:
1. Simplicity and Clean Syntax
Go was designed with simplicity in mind. Its syntax is minimalistic and easy to read, making the language accessible to beginners while also appealing to experienced developers. There are no complex templates or unnecessary keywords, which reduces the learning curve.
2. Compiled and Statically Typed
Unlike interpreted languages like Python or JavaScript, Go is compiled, meaning your code is converted into machine code before it runs. This results in faster execution. Additionally, Go is statically typed, so types are checked at compile time, making the code more predictable and catching errors early in the development process.
3. Built-in Concurrency
One of the standout features of Go is its native support for concurrency through Goroutines and Channels. Concurrency is the ability of a program to perform multiple tasks at the same time, which is crucial for building scalable and efficient applications. Go makes it easy to write concurrent code, making it a great choice for applications that require high performance and scalability.
4. Rich Standard Library
Go comes with a comprehensive standard library that includes packages for web development, file handling, cryptography, and more. This means you can build powerful applications without relying on many external dependencies, which simplifies the development process and improves code stability.
5. Garbage Collection
Go includes automatic garbage collection, which means it manages memory for you. This reduces the risk of memory leaks and other issues related to manual memory management, allowing developers to focus more on writing functional code.
Getting Started with Go
Let’s look at a simple example of a "Hello, World!" program in Go to get a feel for its syntax.package main
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Explanation:
package main
: Defines the package name. Themain
package is used for executable applications.import "fmt"
: Imports thefmt
package, which provides I/O functions.func main()
: Declares themain
function, which is the entry point of every Go program.fmt.Println()
: Prints the string to the console.
Go’s Approach to Variables and Data Types
Go uses a straightforward way of handling variables. You can declare variables using the var
keyword or shorthand:=
.
package main
import "fmt"
func main() {
var message string = "Welcome to Go!"
count := 10 // Shorthand declaration
isGoFun := true
fmt.Println(message, count, isGoFun)
}
Output:
Welcome to Go! 10 true
In this example:
var message string
: Declares a variable with a specified type (string
).count:= 10
: Uses shorthand notation, where the type is inferred by the compiler.
Powerful Concurrency with Goroutines
Go’s support for concurrency is one of its most powerful features. With just a keyword, you can run functions concurrently using Goroutines.
package main
import ( "fmt" "time" )
func sayHello() {
for i := 0; i < 3; i++ {
fmt.Println("Hello from Goroutine!")
time.Sleep(100 * time.Millisecond)
}
}
func main() {
go sayHello() // Launch a Goroutine
fmt.Println("Hello from Main!")
time.Sleep(500 * time.Millisecond)
}
Output:
Hello from Main!
Hello from Goroutine
Hello from Goroutine!
Hello from Goroutine!
In this example:
go sayHello()
: Starts thesayHello
function as a Goroutine.time.Sleep()
: Ensures the main function waits, allowing the Goroutine to complete.
Structs: Go’s Way of Handling Complex Data
Go doesn’t have classes like in object-oriented languages but uses structs to define complex data types.
package main
import "fmt"
type User struct {
Name string
Age int
}
func main() {
user := User{Name: "Alice", Age: 28}
fmt.Println(user.Name, user.Age)
}
Output:
Alice 28
This snippet shows how to define a User
struct and create an instance of it.
Error Handling in Go
Go does not use exceptions like many other languages. Instead, it uses explicit error handling, which makes the code more predictable and easier to debug.
package main
import (
"errors"
"fmt"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("cannot divide by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Output:
Error: cannot divide by zero
Conclusion
In conclusion, Go is a modern programming language that excels in speed, efficiency, and scalability. Its design emphasizes simplicity, making it accessible to both beginners and experienced developers. With strong support for concurrency, a comprehensive standard library, and a focus on robust performance, Go is an excellent choice for building cloud services, microservices, and backend systems. Whether you're starting your programming journey or developing large-scale applications, Go offers powerful features and a streamlined development experience.
Stay tuned for future posts where we will explore Go's features and best practices in greater detail. Happy coding with Go!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
