Chapter 46: Dynamic Programming (Part 6) - DSA Series
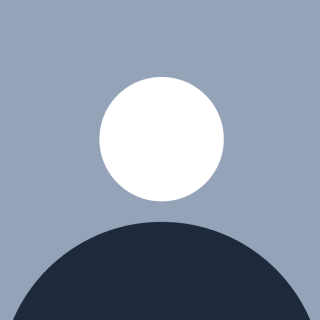
Table of contents
- 1. Basics of Matrices (Math)
- 2. Matrix Chain Multiplication (Recursion)
- 3. MCM with Memoization
- 4. MCM with Tabulation
- 5. Minimum Partitioning
- 6. Minimum Array Jumps (Explanation)
- 7. Minimum Array Jumps (Code Explanation)
- Explanation of Code
- Conclusion
- See Also My Other Posts
- Visit My Other Series Also
- Also Visit My Profiles
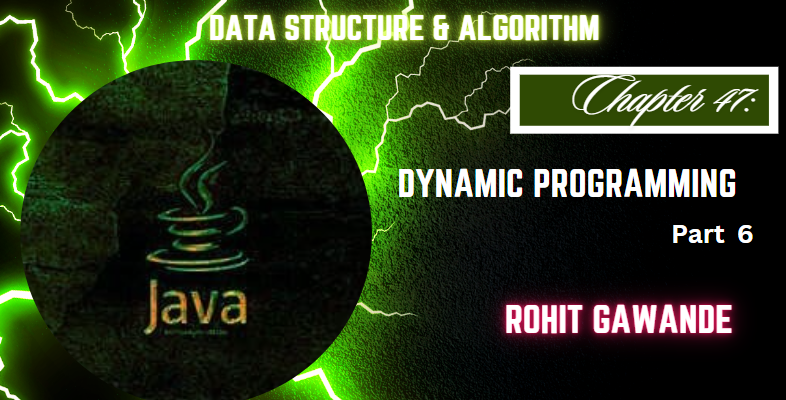
Welcome to Chapter 46 of my Data Structures and Algorithms (DSA) Series! In this chapter, we dive into essential dynamic programming concepts focused on matrix operations and complex problem-solving techniques. Dynamic programming is all about breaking down problems into smaller, manageable subproblems and optimizing them to find the most efficient solution. This chapter covers a range of topics, from matrix basics to advanced applications like the Matrix Chain Multiplication (MCM) problem, Minimum Partitioning, and Array Jump problems. Let's explore these topics in depth and strengthen our problem-solving skills!
1. Basics of Matrices (Math)
Matrices are foundational in many computational tasks, especially those involving multidimensional data. They allow us to represent and manipulate complex structures, making them a crucial tool in algorithms like dynamic programming. A matrix is a two-dimensional array of numbers arranged in rows and columns. Understanding matrix operations, such as multiplication, transposition, and addition, is key to solving many problems efficiently.
2. Matrix Chain Multiplication (Recursion)
Matrix Chain Multiplication (MCM) is a classic dynamic programming problem that involves finding the optimal way to multiply a sequence of matrices. Unlike standard matrix multiplication, where the order is fixed, MCM focuses on determining the most efficient order of multiplications to minimize the number of scalar multiplications. Using recursion, we can break down this problem into smaller subproblems by trying different split points in the chain and computing the cost recursively for each possibility. However, this approach can become inefficient due to overlapping subproblems.
3. MCM with Memoization
Memoization is an optimization technique that improves the recursive MCM solution by storing the results of subproblems to avoid redundant calculations. By using a table to keep track of previously computed values, we can significantly reduce the time complexity of MCM, making it more efficient. Memoization turns the exponential time complexity of plain recursion into a polynomial one, which is crucial for handling larger inputs.
4. MCM with Tabulation
Tabulation is a bottom-up dynamic programming approach that further optimizes the MCM problem by filling a table iteratively. Instead of solving subproblems through recursion, we build solutions starting from the smallest subproblems, working our way up to the original problem. This method ensures that all required subproblems are computed only once and directly referenced when needed, leading to an even more optimized solution for matrix chain multiplication.
5. Minimum Partitioning
The Minimum Partitioning problem involves dividing an array of integers into two subsets such that the absolute difference between their sums is minimized. This problem has real-world applications in load balancing, resource allocation, and more. By leveraging dynamic programming, we can break down the array into subsets and calculate the minimum achievable difference efficiently. This approach relies on a subset-sum style DP array that helps us track the possible sums for different partitions.
6. Minimum Array Jumps (Explanation)
The Minimum Array Jumps problem asks for the minimum number of jumps required to reach the end of an array, where each element represents the maximum jump length from that position. This problem is complex due to the potential variations in jump paths, but a dynamic programming approach allows us to solve it efficiently by calculating the minimum jumps for each position as we progress through the array. By understanding which paths lead to the fewest jumps, we can determine the optimal route to the end.
7. Minimum Array Jumps (Code Explanation)
Here's an example of code to solve the Minimum Array Jumps problem using dynamic programming:
public int minJumps(int[] arr) {
int n = arr.length;
if (n <= 1) return 0;
if (arr[0] == 0) return -1;
int[] jumps = new int[n];
jumps[0] = 0;
for (int i = 1; i < n; i++) {
jumps[i] = Integer.MAX_VALUE;
for (int j = 0; j < i; j++) {
if (i <= j + arr[j] && jumps[j] != Integer.MAX_VALUE) {
jumps[i] = Math.min(jumps[i], jumps[j] + 1);
break;
}
}
}
return jumps[n - 1] == Integer.MAX_VALUE ? -1 : jumps[n - 1];
}
Explanation of Code
In this code, we initialize an array jumps
to store the minimum number of jumps required to reach each position in the array. We then iterate through each element of the array and calculate the minimum jumps needed by considering all possible jumps to the current position. This solution provides a polynomial-time approach to the problem and ensures we achieve the optimal number of jumps.
Conclusion
Dynamic programming is a powerful technique that allows us to solve complex problems by breaking them down into manageable subproblems and storing intermediate results to avoid redundant calculations. In this chapter, we've explored a variety of DP problems, from the basics of matrices to advanced applications like Matrix Chain Multiplication, Minimum Partitioning, and Minimum Array Jumps. Each problem highlights the versatility and efficiency of dynamic programming in optimizing real-world solutions. As we continue to build on these skills, weโll be better equipped to tackle even more challenging problems!
Thank you for reading! Stay tuned for more chapters in my DSA Series.
See Also My Other Posts
To get a complete understanding of Java and Data Structures & Algorithms (DSA), check out these other chapters in my series:
1. Chapter 1: Variables and Data Types
This chapter is the foundation of Java programming. It covers the basics of variables, how to declare them, and the different data types available in Java (primitive and non-primitive).
2. Chapter 25: Queues
In this chapter, we delve into Queues, an essential data structure that follows the First-In-First-Out (FIFO) principle.
3. Chapter 26: Greedy Algorithm
The Greedy Algorithm is a fundamental problem-solving technique in computer science.
Visit My Other Series Also
Expand your knowledge in web development with my dedicated series:
1. Full Stack Java Development
This series walks through the entire journey of becoming a Full Stack Java Developer, from fundamentals like Java Programming and Object-Oriented Programming (OOP) to advanced topics like Spring Boot, REST APIs, and Database Integration. I cover front-end technologies like HTML, CSS, and JavaScript, and show how to integrate them with Java back-end systems.
2. Full Stack JavaScript Development
In this series, I focus on Full Stack JavaScript Development, covering the entire stack from front-end frameworks like React and Angular to back-end technologies such as Node.js, Express.js, and MongoDB. The series provides a comprehensive guide for building full-fledged web applications using JavaScript, the most popular language for web development today.
Also Visit My Profiles
Stay connected and explore my coding journey through my other profiles:
GitHub: Check out my open-source projects, coding exercises, and repositories for Java, JavaScript, and more.
LinkedIn: Connect with me professionally to stay updated on my learning path, projects, and posts.
LeetCode: Follow my progress as I solve coding challenges, optimize algorithms, and prepare for technical interviews.
Feel free to explore these resources for a more comprehensive learning experience!
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
๐ Tech Enthusiast | Full Stack Developer | System Design Explorer ๐ป Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iโm Rohit Gawande! ๐I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iโm Currently Doing ๐น Writing an in-depth System Design Series to help developers master complex design concepts.๐น Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.๐น Exploring advanced Java concepts and modern web technologies. What You Can Expect Here โจ Detailed technical blogs with examples, diagrams, and real-world use cases.โจ Practical guides on Java, System Design, and Full Stack Development.โจ Community-driven discussions to learn and grow together. Letโs Connect! ๐ GitHub โ Explore my projects and contributions.๐ผ LinkedIn โ Connect for opportunities and collaborations.๐ LeetCode โ Check out my problem-solving journey. ๐ก "Learning is a journey, not a destination. Letโs grow together!" Feel free to customize or add more based on your preferences! ๐